Add and Use Custom Fonts in Next.js
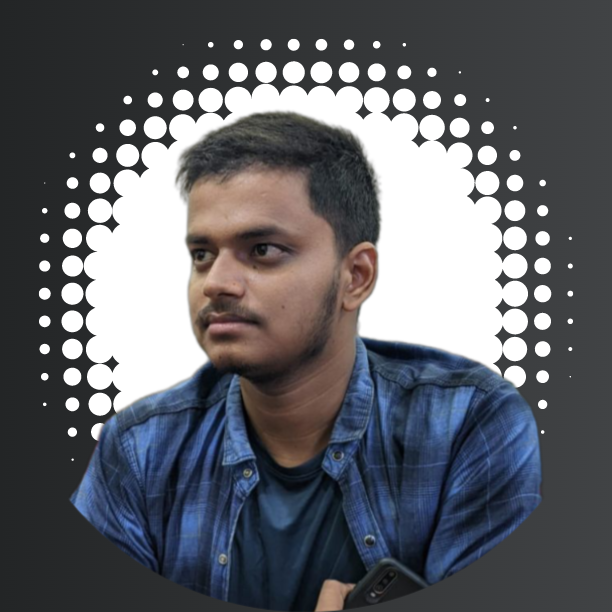
Table of contents
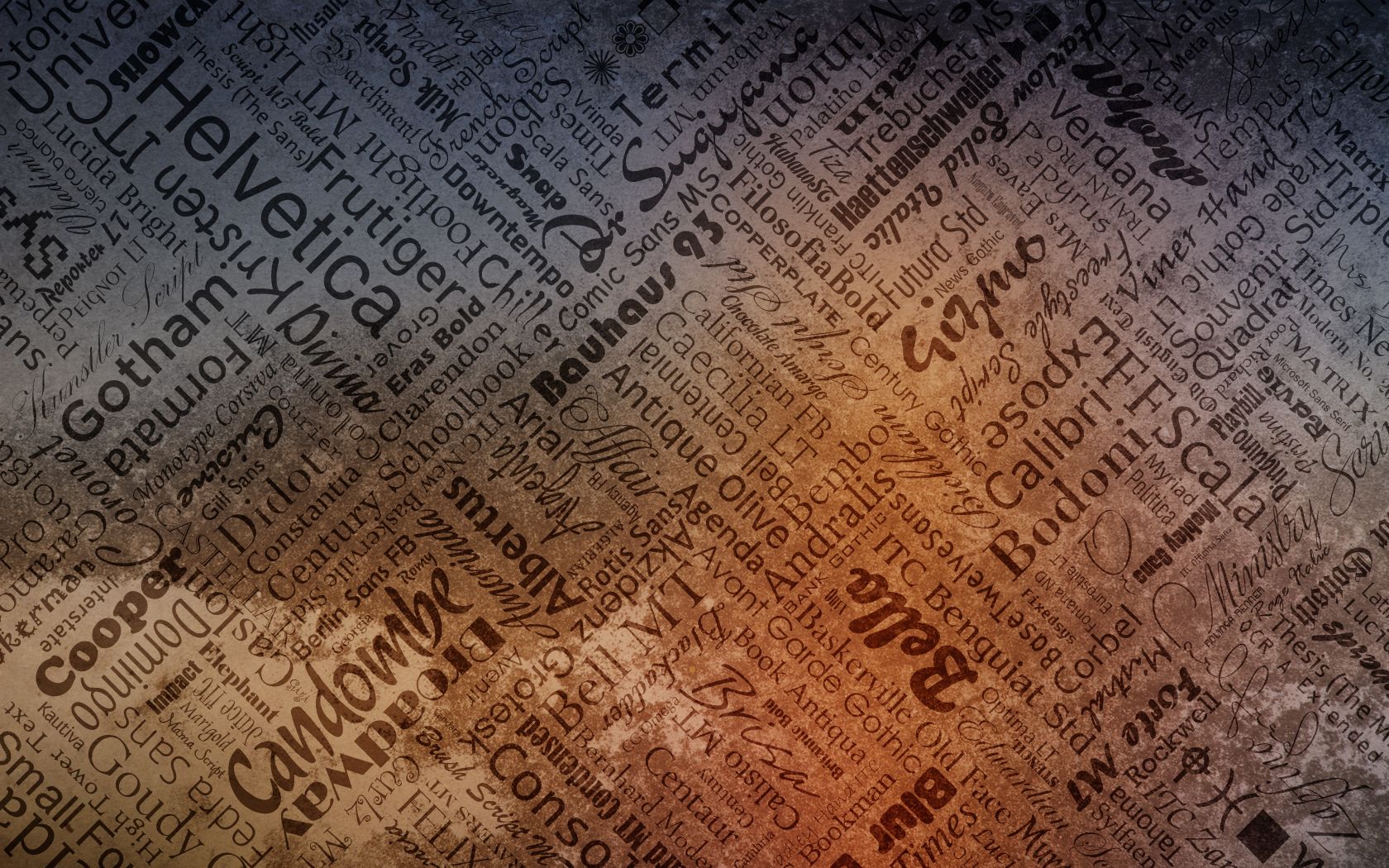
How to Add and Use Custom Fonts in Next.js
To enhance the appearance of your Next.js project, you can add custom fonts from external sources like dafont.com. Follow these steps to download, install, and use custom fonts in your Next.js project:
Step 1: Download and Install the Custom Font
Go to dafont.com and search for the font you want to use. For example, let's consider the "cfour" font: cfour font link.
Download the font file (usually in .otf or .ttf format).
Unzip the downloaded file and place it in the
public/fonts
directory of your Next.js project.
Step 2: Install and Configure next-fonts
in Next.js
Install the
next-fonts
package in your project using npm:npm install next-fonts
Open the
next.config.js
file and add the following lines of code:const withFonts = require('next-fonts'); module.exports = withFonts({ enableSvg: true, webpack(config, options) { return config; }, });
These configurations enable the use of external fonts in your Next.js application.
Step 3: Apply the Custom Font in _app.js
To make the custom font available throughout your Next.js project, you need to add it to your _app.js
file in the src/pages
directory. You can do this in two ways:
Method 1: Using <style>
Tag
used and verified by us
import localFont from 'next/font/local';
const myFont = localFont({
src: [
{
path: '../../public/fonts/cfour/Cfour.otf',
weight: '400',
},
],
});
export default function MyApp({ Component, pageProps }) {
return (
<>
<style jsx global>{`
html {
font-family: ${myFont.style.fontFamily};
}
`}</style>
<Component {...pageProps} />
</>
);
}
This approach applies the custom font to the entire html
tag. If you want to use another system font in a specific component, you can use !important
in the CSS as shown below:
.new {
font-family: Sans !important;
}
Method 2: Using className
Recommended by NextJs
import localFont from 'next/font/local';
const myFont = localFont({ src: './my-font.woff2' });
export default function MyApp({ Component, pageProps }) {
return (
<main className={myFont.className}>
<Component {...pageProps} />
</main>
);
}
This method is recommended by Next.js. It allows you to apply the custom font to specific components using the className
attribute.
Link : NextJs
Step 4: Using the Custom Font in Specific Components
If you have used the second method in Step 3 (using className
), and you want to apply the custom font in a specific component (e.g., src/pages/about.js
), you can follow this approach:
import React from 'react';
import { myFont } from '../_app';
export default function About() {
return (
<div>
<h1>About</h1>
<p className={myFont}>
This is a paragraph that uses the "my-font" font family.
</p>
</div>
);
}
By applying the myFont
className to the paragraph, the custom font will be used in that specific component.
Remember that these steps assume you've followed the directory structure and file naming conventions of Next.js projects. With these instructions, you can now successfully download, install, and apply custom fonts to your Next.js project, making it more visually appealing and unique.
References
Subscribe to my newsletter
Read articles from Kumar Ashish Ranjan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
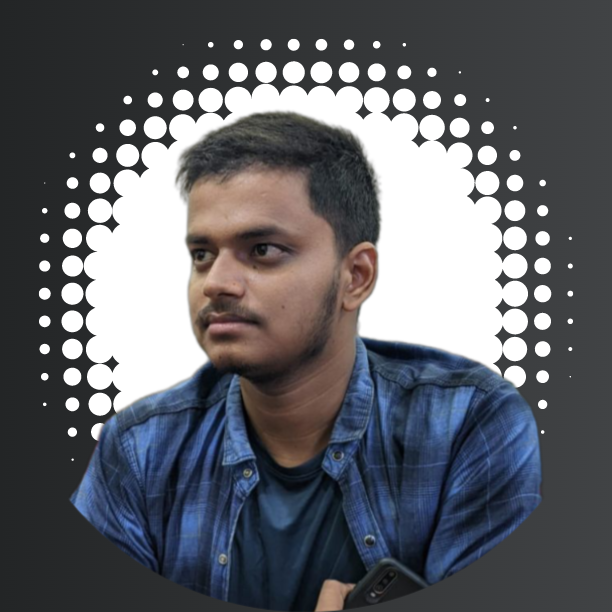
Kumar Ashish Ranjan
Kumar Ashish Ranjan
Hello, fellow tech enthusiasts! I'm Ashish Ranjan, a passionate Web Developer and Frontend Technician. My journey in the world of technology has been a thrilling adventure, where I've delved deep into the realms of CSS, Web Development, and Web Design. Let's connect and collaborate on all things tech. Looking forward to engaging discussions and learning from each other. Cheers! ๐ Crafting the Digital Experience | Exploring the Boundless Horizons of Code