Building a React Native App with AWS Amplify.
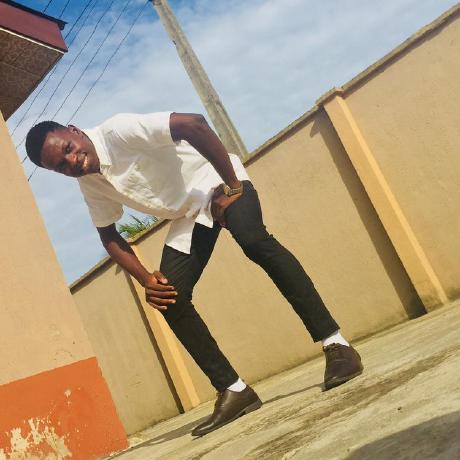
Why do you need AWS Amplify?
Aws Amplify is a Service that helps you build, deploy and scale your app quickly and easily. AWS Amplify is a complete solution that lets frontend web and mobile developers easily build, connect, and host fullstack applications on AWS.
It consists of Amplify CLI to configure your App, Amplify Libraries to add certain features to your App like Authentication, data and file storage, Amplify UI components that supports various frameworks like Angular, Vue, React, Swift etc. to help you build you UI and Amplify hosting to simlify the deployment of your app.
Create Account
First and Foremost before, you start building your App with AWS Amplify you will have to create an AWS account with this link:
If you already have an account, then you can move on to the Next Step.
Configure Amplify CLI
To set up the Amplify CLI on your local machine, you have to configure it to connect to your AWS account. You can do that by typing "aws configure" in your terminal, then you will be taken to your aws account where you will create a new User. Make sure you select "Attach policies directly" and select "AdministratorAccess-Amplify" as the Permissions policy. On the user details page, navigate to the Security credentials tab, scroll down to Access keys and select Create access keys. Follow the instructions and create both Access ID and secret access key. Enter these values into the corresponding CLI prompts.
If you are having issues with this process you can click on the link below to watch a youtube video guide that can help you:
Set up React Native Project
Let us initialize a new project. We will be using expo cli for this app. Create a new App with the following command.
command
command
Install the necessary dependencies with the following command:
command
Start the App with the following command:
Command
Setting up Backend
With the App running let us set up amplify and create our backend to support the App. Let us start up amplify in our app by typing this in our terminal.
Command
When you initialize Amplify you'll be prompted for some information about the app, with the option to accept recommended values:
? Enter a name for the project (movie_app)
The following configuration will be applied:
Project information
| Name: amplified_todo
| Environment: dev
| Default editor: Visual Studio Code
| App type: javascript
| Javascript framework: react-native
| Source Directory Path: /
| Distribution Directory Path: /
| Build Command: npm run-script build
| Start Command: npm run-script start ?
Initialize the project with the above configuration? Yes
Using default provider awscloudformation ?
Select the authentication method you want to use: AWS profile ?
Please choose the profile you want to use default
Set up Frontend
Configure your react native app to interact with the backend services. Open App.js and add the following code block:
import { Amplify } from 'aws-amplify';
import awsExports from './src/aws-exports';
Amplify.configure(awsExports);
In our movie_app we created components folder for our Episodes, Homecontent, Movieitem and videoplayer , constants folder for our styles, hooks folder for our most used functions or helpers, navigation folder for our bottomtab navigation, screens for Homescreen, moviedetail screen and notfound screen. When you are done you should have this structure: https://github.com/Dkingofcode/ReactNative-netflix
Add API and database
Now that you’ve created and configured a React app and initialized a new Amplify project, you can add a feature. The first feature you will add is an API.
The Amplify CLI supports creating and interacting with two types of API categories: REST and GraphQL.
The API you will be creating in this step is a GraphQL API using AWS AppSync (a managed GraphQL service) and the database will be Amazon DynamoDB (a NoSQL database). To add a graphql API to your app you can use the following command:
Command
Accept the default values which are highlighted below:
Select from one of the below mentioned services: GraphQL ?
Here is the GraphQL API that we will create.
Select a setting to edit or continue Continue ?
Choose a schema template: Single object with fields
To deploy this API, write the following:
Command
? Are you sure you want to continue? Yes ... ?
Do you want to generate code for your newly created GraphQL API Yes ?
Choose the code generation language target javascript ? Enter the file name
pattern of graphql queries, mutations and subscriptions src/graphql/**/*.js
? Do you want to generate/update all possible GraphQL operations - queries, mutations and subscriptions Yes
? Enter maximum statement depth [increase from default if your schema is deeply nested] 2
Now the API is live and you can start interacting with it!
Next, run the following command to check Amplify's status:
Command
To view the API in the AppSync console you can run the following command:
Command
Add Authentication
Amplify uses Amazon Cognito as its authentication provider. Amazon Cognito is a robust user directory service that handles user registration, authentication, account recovery & other operations.
To add authentication to your app, run this command:
Command
Select the defaults for the following propmts:
Do you want to use the default authentication and security configuration?Default configuration
Warning: you will not be able to edit these selections. ?
How do you want users to be able to sign in? Username ?
Do you want to configure advanced settings? No, I am done.
To deploy this service you can run the following command:
amplify push
To view the deployed services in your app at any time on the Appsync console run the command:
amplify console
Create Login UI
To create login UI install the following command:
Command
Add the Authenticator login component like this:
import { withAuthenticator, useAuthenticator } from '@aws-amplify/ui-react-native';
Create a custom signout button component:
// retrieves only the current value of 'user' from 'useAuthenticator'
const userSelector = (context) => [context.user]
const SignOutButton = () => {
const { user, signOut } = useAuthenticator(userSelector);
return (
<Pressable onPress={signOut} style={styles.buttonContainer}>
<Text style={styles.buttonText}>Hello, {user.username}! Click here to sign out!</Text>
</Pressable>
)
};
Add the Signup button component to your App
Wrap your App export with withAuthenticator component:
export default withAuthenticator(App);
For more info check out aws website on:
To write your own blogs go to hashnode at:
Subscribe to my newsletter
Read articles from David Oladepo directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
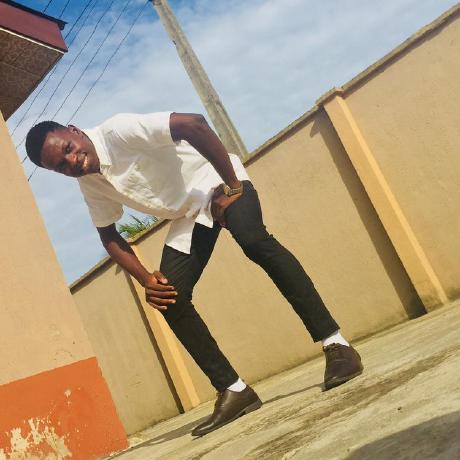
David Oladepo
David Oladepo
I am a software developer from Nigeria, with a passion for building quality products for customers.