Introducing Django
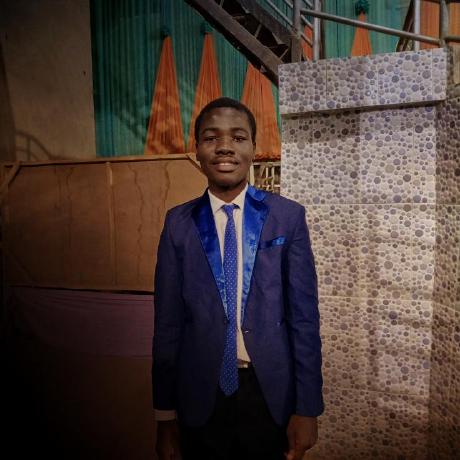
Table of contents
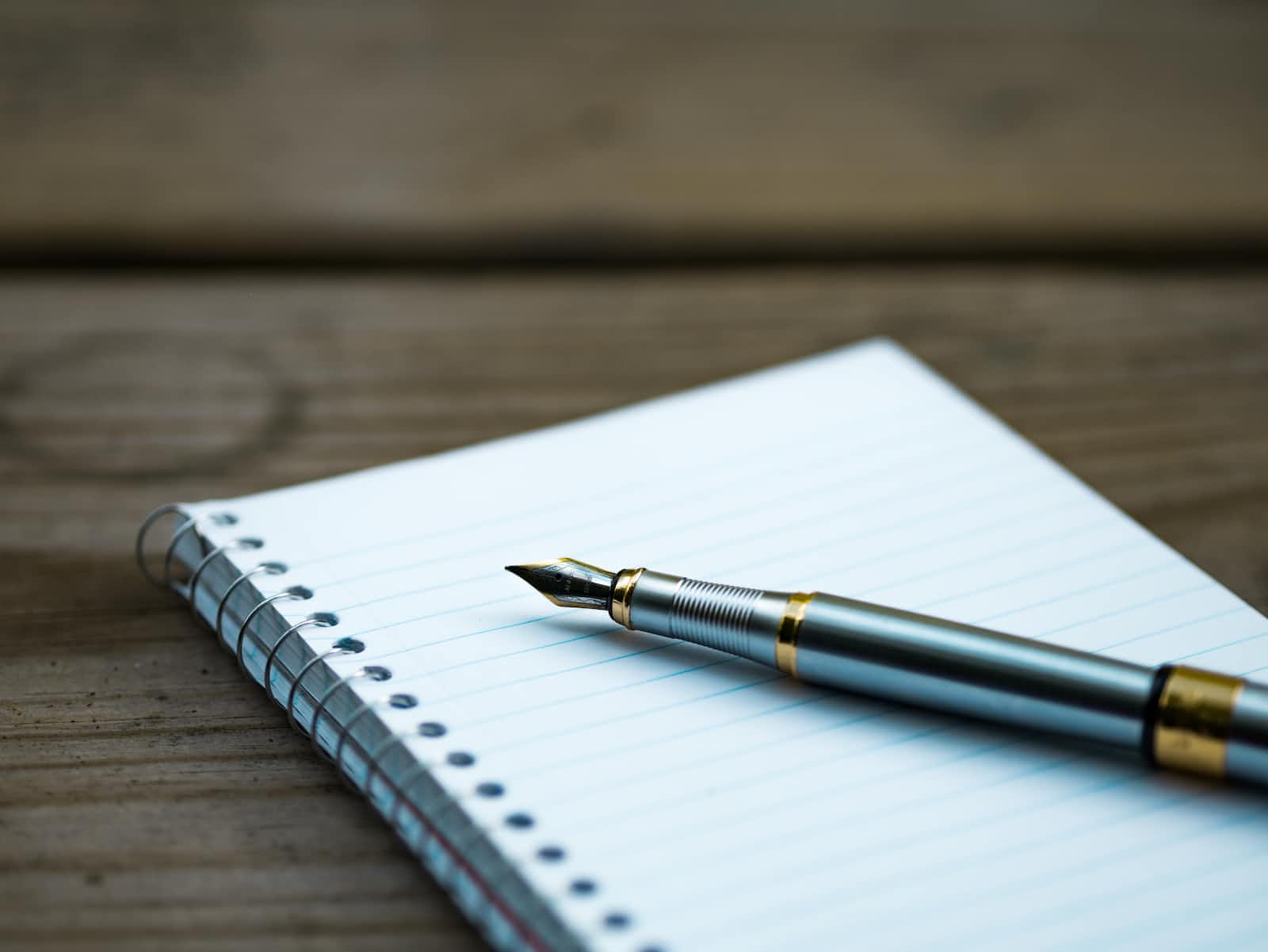
What is Django by the way?
Django is a powerful and versatile web framework that empowers web developers to build feature-rich web applications efficiently and quickly.
Django is an Open source Python web framework, that is, it has various Python codes combined as a structure to aid quick web development from simple to giant web apps.
Web Framework
Open Source
Rather than recreate most web projects from scratch, programmers over the years have created web frameworks in all the major programming languages: Django and Flask in Python, Rails6 in Ruby, and Express7 in JavaScript, Laravel in PHP among many others
Features of the Django Framework
Model-View-Template(MVT) Architecture
The MVT architecture is a way of organizing code in Django. It separates different parts of the web application, making it easier to manage and understand. Models represent data, Views handle user requests, and Templates control how data is displayed to users.
### This is an example models.py file where we ### ..interact with the database class Article(models.Model): title = models.CharField(max_length=200) content = models.TextField() published_date = models.DateTimeField() def __str__(self): return self.title
##This is an example views.py where the functions are written from django.shortcuts import render from .models import Article def article_detail(request, article_id): article = Article.objects.get(id=article_id) return render(request, 'article_detail.html', {'article': article})
###This is an article page where the page is displayed with html <!DOCTYPE html> <html> <head> <title>{{ article.title }}</title> </head> <body> <h1>{{ article.title }}</h1> <p>{{ article.content }}</p> <p>Published on: {{ article.published_date }}</p> </body> </html>
###A urls.py file that designs what is returned based ### ...on the url typed in the browser from django.urls import path from . import views urlpatterns = [ path('articles/<int:article_id>/', views.article_detail, name='article_detail'), ]
Admin-Interface
Django provides an automatic admin interface, which is like a ready-made backend to manage website content without writing extra code. It simplifies content management tasks.
Object-Relational Mapping
ORM helps developers interact with the database using Python code, without directly writing complex database queries. It makes database operations easier to manage. You won't need database query knowledge differently for MySQL, MariaDB, Oracle etc, all you need is a single code and configuration with the data of your choice.
URL Routing
URL routing maps URLs (
like website addresses eg https:google.com/django
) to specific parts of the code that handle user requests. It helps the web application decide what content to show based on the URL.Form Handling
Django's form handling simplifies creating and managing web forms used for user input. It takes care of validating user input and displaying error messages if needed.
Template Engine
The template engine separates the visual appearance of a web page from the underlying code. It makes it easier to create consistent layouts and reuse code for different pages. The template is written in a Django Template and allows you to combine Django codes with regular HTML Syntax.
Built-in Authorization and Authentication System
Authentication ensures users can log in and access personalized content, while authorization controls what users are allowed to do based on their roles and permissions.
Security features
Django includes built-in features to protect web applications from common security vulnerabilities and attacks, ensuring that user data is kept safe.
Internationalization and Localization
Internationalization allows the web application to support multiple languages, while localization tailors the content to specific regions or languages.
Scalability
Scalability means that Django can handle an increasing number of users and traffic without sacrificing its performance.
Be sure to keep a very good grip of this information and explanations, you will need it to understand future concepts.
Django: The Web framework for perfectionists with deadlines
Subscribe to my newsletter
Read articles from Olumide Adeola directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
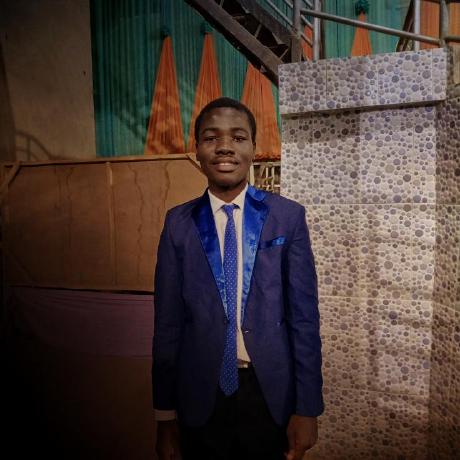
Olumide Adeola
Olumide Adeola
Classical | Health | Academics | Services et Solutions | Finance | Agriculture | Technology This is what my passion revolves round....