Steps to Implement Impersonation in Laravel
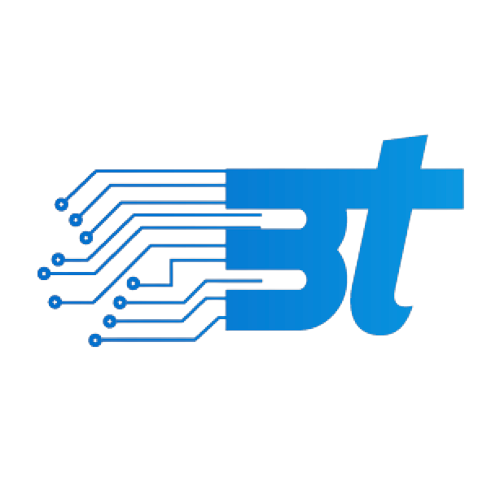
Table of contents
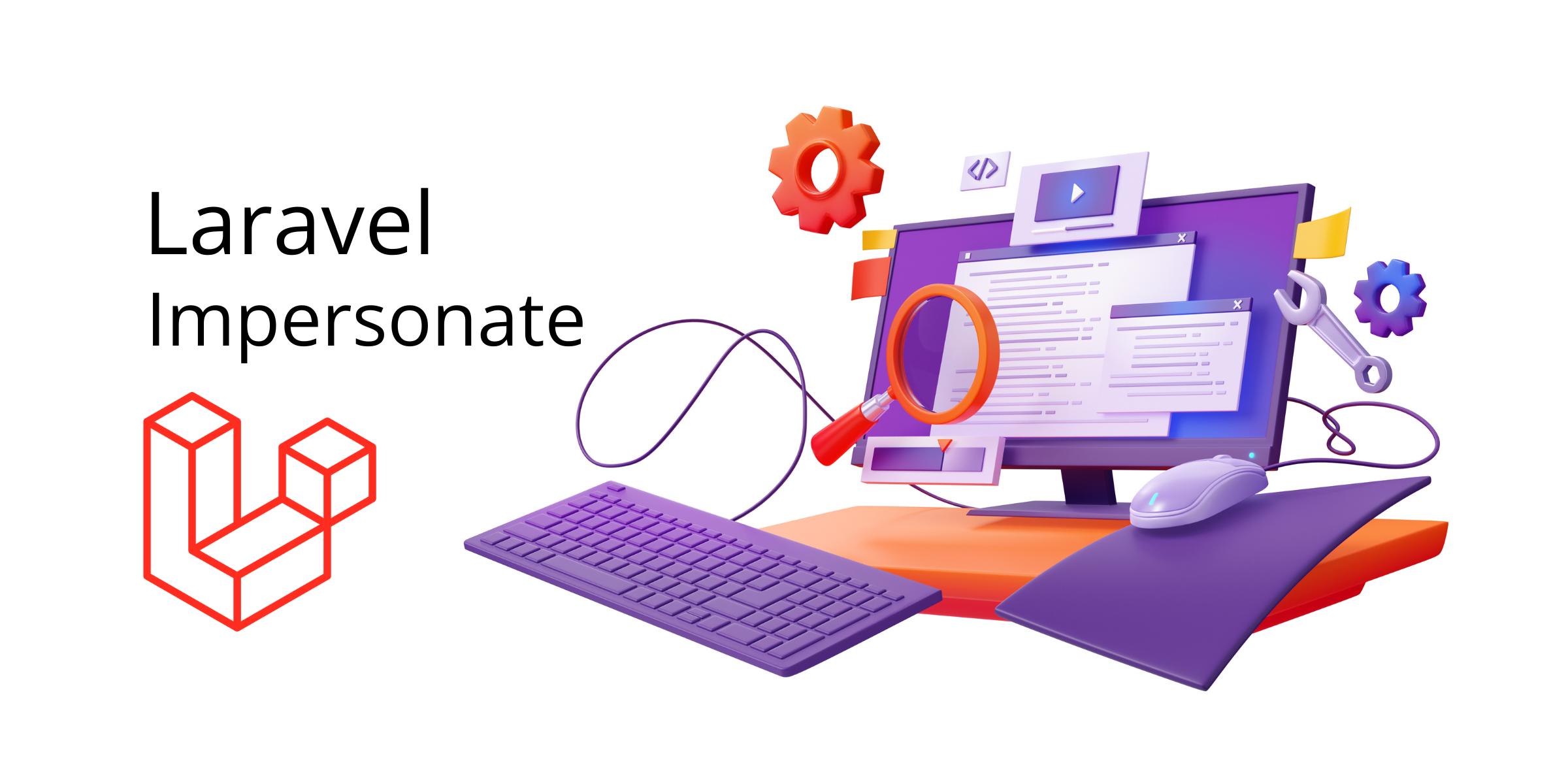
The impersonation in Laravel is similar to playing dress-up but for website administrators or support workers. Assume you are the administrator of a website, and people report problems or want assistance. Impersonation allows you to "dress up" or become that user for a short time to view precisely what they're seeing on the page. It's like putting yourself in their shoes to comprehend what they're going through.
Example:
Imagine you're an admin, pretending to be a website administrator with special powers to see and control issues behind the scenes. Users report problems or need assistance, and you can temporarily "put on" the user's clothes to access their account and provide help. After investigating, you can remove the user's clothes and return to being the website admin. An impersonation is a powerful tool, but only for support or administrative purposes, and only trusted admins should have access to it.
Impersonation enhances website administrators' support and understanding of user issues, but comes with responsibility and must be used wisely.
The Steps to Implement Impersonation Manually in Laravel
Step 1: Setup Your Database
Begin by including an is_impersonating column in the user's database. This column will keep track of whether a user is being impersonated at the moment. You may add these columns through the creation of a new migration:
php artisan make:migration add_impersonating_column_to_users_table --table=users
add_impersonating_column_to_users_table php artisan make: migration --table=users
public function up()
{
Schema::table('users', function (Blueprint $table) {
$table->boolean('is_impersonating')->default(false);
});
}
To apply the changes to the database, run the migration:
php artisan migrate
Step 2. Create Impersonate Middleware:
Create a new middleware to handle the impersonation process next. To create a new middleware, which uses the next command:
php artisan make:middleware ImpersonateMiddleware
Update the handle() function in the produced ImpersonateMiddleware file (app/Http/Middleware/ImpersonateMiddleware.php) to incorporate the impersonation logic:
namespace App\Http\Middleware;
use Closure;
use Illuminate\Support\Facades\Auth;
class ImpersonateMiddleware
{
public function handle($request, Closure $next)
{
if (Auth::user() && Auth::user()->is_impersonating) {
Auth::onceUsingId(session('original_user_id'));
}
return $next($request);
}
}
Step 3: Install and activate the Impersonate Middleware:
In the app/Http/Kernel.php file, add the ImpersonateMiddleware to the middleware stack. The middleware may be applied globally or to individual routes or groups.
protected $middlewareGroups = [
'web' => [
.
\App\Http\Middleware\ImpersonateMiddleware::class,
],
];
Step 4: Design an Impersonate Controller
Create a new controller to manage the impersonation process's start and stop:
php artisan make:controller ImpersonateController
Add the following methods to the produced ImpersonateController (app/Http/Controllers/ImpersonateController.php) to start and terminate impersonation:
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Auth;
class ImpersonateController extends Controller
{
public function impersonate(Request $request, $user_id)
{
$user = User::find($user_id);
if ($user) {
session()->put('original_user_id', Auth::id());
Auth::user()->update(['is_impersonating' => true]);
// Log in as the user to impersonate
Auth::login($user);
return redirect('/')->with('success', 'You are now impersonating ' . $user->name);
}
return redirect()->back()->with('error', 'User not found');
}
public function stopImpersonating()
{
Auth::user()->update(['is_impersonating' => false]);
Auth::onceUsingId(session('original_user_id'));
session()->forget('original_user_id');
return redirect('/')->with('success', 'You have stopped impersonating');
}
}
Step 5: Establish Routes
In the routes/web.php file, add the following routes for beginning and halting impersonation:
use App\Http\Controllers\ImpersonateController;
Route::get('impersonate/{user_id}', [ImpersonateController::class, 'impersonate']);
Route::get('stop-impersonating', [ImpersonateController::class, 'stopImpersonating']);
Step 6: Make use of the Impersonate Links
These routes may now be used to generate links or buttons in your views or admin interface. When an administrator hits the imitate link or button for a particular user, they begin imitating that user. They can click the "Stop Impersonating" link to quit impersonating.
Summary
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
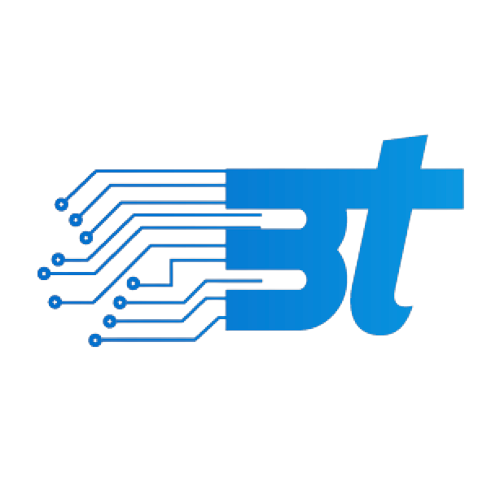
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.