PHP for Beginners: A Simple Guide to Learning the Language

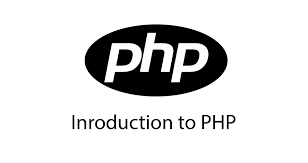
What is PHP?
PHP is a server-side scripting language that is used to create dynamic and interactive web pages. It is a powerful tool that can be used to do a variety of things, such as:
Process form data
Generate dynamic content.
Connect to databases
Create web applications.
Why learn PHP?
There are many reasons why you might want to learn PHP. Here are a few of the most common reasons:
PHP is a popular language. It is used by millions of websites and web applications around the world.
PHP is a versatile language. It can be used to create a wide variety of web applications.
PHP is a relatively easy language to learn. It has a simple syntax that is easy to understand for beginners.
Getting Started With PHP
If you are interested in learning PHP, there are a few things you will need to do:
Install a PHP development environment. There are many different PHP development environments available, such as XAMPP and MAMP.
Learn the basics of PHP syntax. There are many resources available online that can teach you the basics of PHP syntax.
Start writing PHP code. The best way to learn PHP is by writing code. Start by creating simple PHP scripts, and then gradually work your way up to more complex projects.
Where to learn PHP
There are many resources available online that can teach you PHP. Here are a few of the most popular resources:
W3Schools: https://www.w3schools.com/php/
PHP Tutorial: https://www.php.net/manual/en/tutorial.php
Learn PHP: https://www.learnphp.com/
PHP for Beginners: https://www.codecademy.com/learn/learn-php
Examples
Here are a few examples of PHP code that beginners can use to get started:
- A simple "Hello World" script
<?php
echo "Hello World!";
?>
- A script that gets the user's name and age
<?php
$name = $_POST["name"];
$age = $_POST["age"];
echo "Your name is $name and you are $age years old.";
?>
- A script that connects to a database and retrieves a list of users
<?php
$db = new mysqli("localhost", "username", "password", "database");
$query = "SELECT * FROM users";
$results = $db->query($query);
foreach ($results as $row) {
echo $row["name"] . " " . $row["age"] . "<br>";
}
?>
VS Code Extensions for PHP
Several VS Code extensions can be helpful for PHP developers. Here are a few of the most popular:
PHP IntelliSense: This extension provides autocompletion and code linting for PHP.
PHP Debugger: This extension allows you to debug PHP code in VS Code.
PHPStorm Remote Development: This extension allows you to connect to a remote PHP development environment from VS Code.
Tips for Learning PHP
Here are a few tips for learning PHP:
Start with the basics. Learn the syntax of PHP and how to create simple PHP scripts.
Find a good tutorial or course. There are many great resources available online that can teach you PHP.
Practice, practice, practice. The best way to learn PHP is by writing code. Start by creating simple PHP scripts and then gradually work your way up to more complex projects.
Don't be afraid to ask for help. There are many online forums and communities where you can ask questions and get help from other PHP developers.
PHP is a powerful and versatile language that can be used to create a wide variety of web applications. If you are interested in learning PHP, there are many resources available online that can help you get started.
Here are some additional resources that you may find helpful:
PHP Documentation: https://www.php.net/manual/en/
PHP Stack Exchange: https://stackoverflow.com/questions/tagged/php
PHP Reddit: https://www.reddit.com/r/php/
I hope this article has helped you learn more about PHP. If you have any questions, please feel free to ask me.
Subscribe to my newsletter
Read articles from TGC Insights directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
