Basic Concepts of Programming Using Javascript 1.0
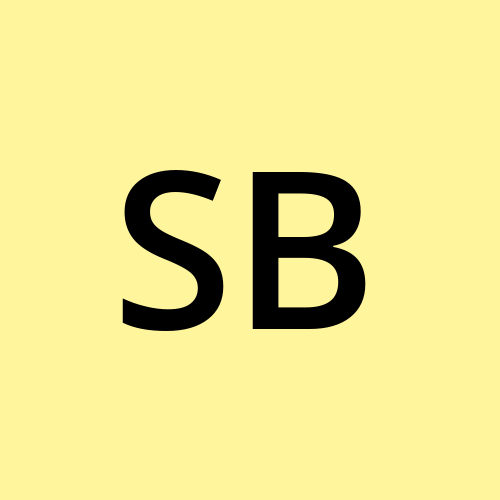
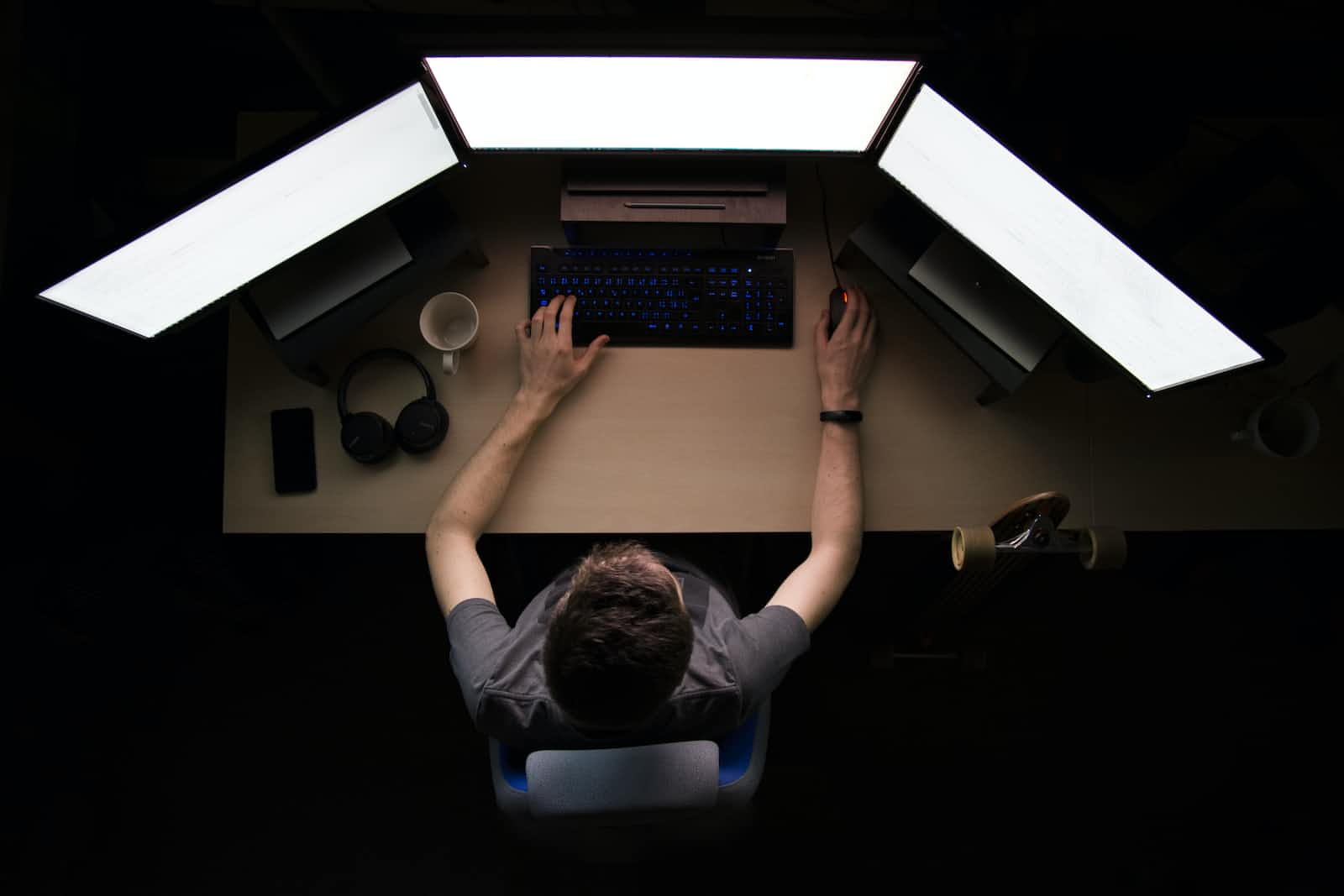
Bindings
How does a program keep an internal state? How does it remember things? So be it any programming language javascript or any other. Mostly language uses bindings to bind a value to a variable so that value can be used by just accessing that variable.
For Example:- let ticketPrice = 100
In this example, the ticket price is a variable that is storing the price of the ticket and can be accessed throughout the program.
For example:- console.log(ticketPrice + "$")
Output will be:- 100$
So by using let it is not the case that we have assigned 100 forever. it can be changed as per our requirements.
For Example:- ticketPrice = 120
The =
operator can be used at any time on existing bindings to disconnect them from their existing values and have them point to a new value.
A Single let Statement may define multiple bindings.
For Example:- let single = 10, double = 20
We can create bindings with var
and const
. We won't use var. It has some bad properties that make it not used in production.
For Const
it keeps bindings constant. There can be many scenarios where we do not want to change our values so we go with const.
Bindings Names
Bindings Names can be any word. Digits can be part of the name but at the same time, we can not start the name with a digit. we can have a dollar sign but we can not start the name with that. it can include _
an underscore sign but no other punctuation or other special character.
There are some reserved words that we can not use as binding names. for example let
, var
and const
are reserved keywords.
So There is The List of reserved bindings names that we can not use. Do not try to remember.
await, break, case, catch, class, const, continue, debugger, default, delete, do, else, enum, export, extends, false, finally, for, function, if, implements, import, in, instanceof, interface, let, new, null, package, private, protected, public, return, static, super, switch, this, throw, true, try, typeof, var, void, while, with, yield
Functions
A function is a piece of program wrapped into a parenthesis that can be invoked and executed. The prompt
binding also holds a function.
For Example:- prompt("Enter Passcode")
Control Flow
When your program contains more than one statement. The Statements are get executed as if they are a story, from top to bottom.
For Example:- let theNumber = Number(prompt('Enter a Number')); console.log("Square root of your number is" + theNumber * theNumber)
The Flow of this statement is taking a number from the user and converting that into a number because that would be a string or to be sure that we are acting on a number otherwise it can throw an error. On the Next line, we are multiplying that to produce a square of that number. The Flow is top to bottom but in complex programming, the flow is never going to be top to bottom so it brings us to conditional execution.
Conditional Execution
Not all programs are straight roads. we need to take routes based on condition and that is conditional execution
For Example:- let iHaveApple = true; if(iHaveApple) console.log("I have apple")
This code may get executed from top to bottom. But imagine if I reset the binding to false then it won't print anything on the console because the only thing it will enter into the if statement if I have apple is true. Imagine getting this input from the user and then giving output.
In False conditions, we can perform operations depending on our requirements. If the user does not have Apple then you can offer him/her to buy from you. These are the real-world scenarios nothing else.
If we have more than one condition we can change them through if else
While and Do Loop
Imagine we need to print numbers from 1 to 100. One way of printing them is to console.log all of them.
For Example:- console.log(1); console.log(2); console.log(3); console.log(4); console.log(5) and so on until 100th
If you are assuming it is a good way then you are wrong it is the dirtiest way of doing the task. For the repetitive task, we have loops in which we can provide an entering condition and an exit condition.
When we combine it with the do and while loop. For Example:-
let number = 1 ; while(number > 100) { console.log(number); number++ }
We can achieve this task just in three lines of code. You can increase the console.log to whatever amount you want it would just stay three lines. You Literally can do same task using For Loop.
Switch Statements.
It just Switches the output based on input or a result that has been calculated. Imagine taking input from the user and then displaying the data based on his input. It provides an alternative to long chains of if...else
statements for handling multiple possible outcomes.
function getDayName(dayNumber) { let dayName;
switch (dayNumber) { case 1: dayName = "Sunday"; break; case 2: dayName = "Monday"; break; case 3: dayName = "Tuesday"; break; default: dayName = "Invalid day"; break; }
return dayName; }
console.log(getDayName(1)); Output: "Sunday"; console.log(getDayName(8)) // Output: "Invalid day"
That's it for the basics in javascript I would like to hear comments on this if you are reading this. PLEASE RECOMMEND what I can add more to this.
I Would Like to Bring a 2.0 article on this topic with some good examples.
Subscribe to my newsletter
Read articles from Sumit Bhardwaj directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
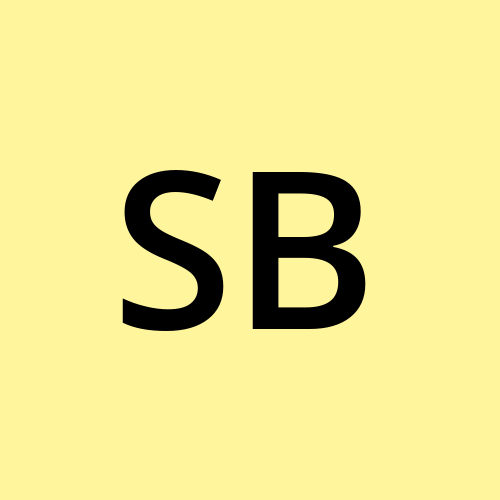