Beyond the Code: How Python is Making Cars Safer and Smarter
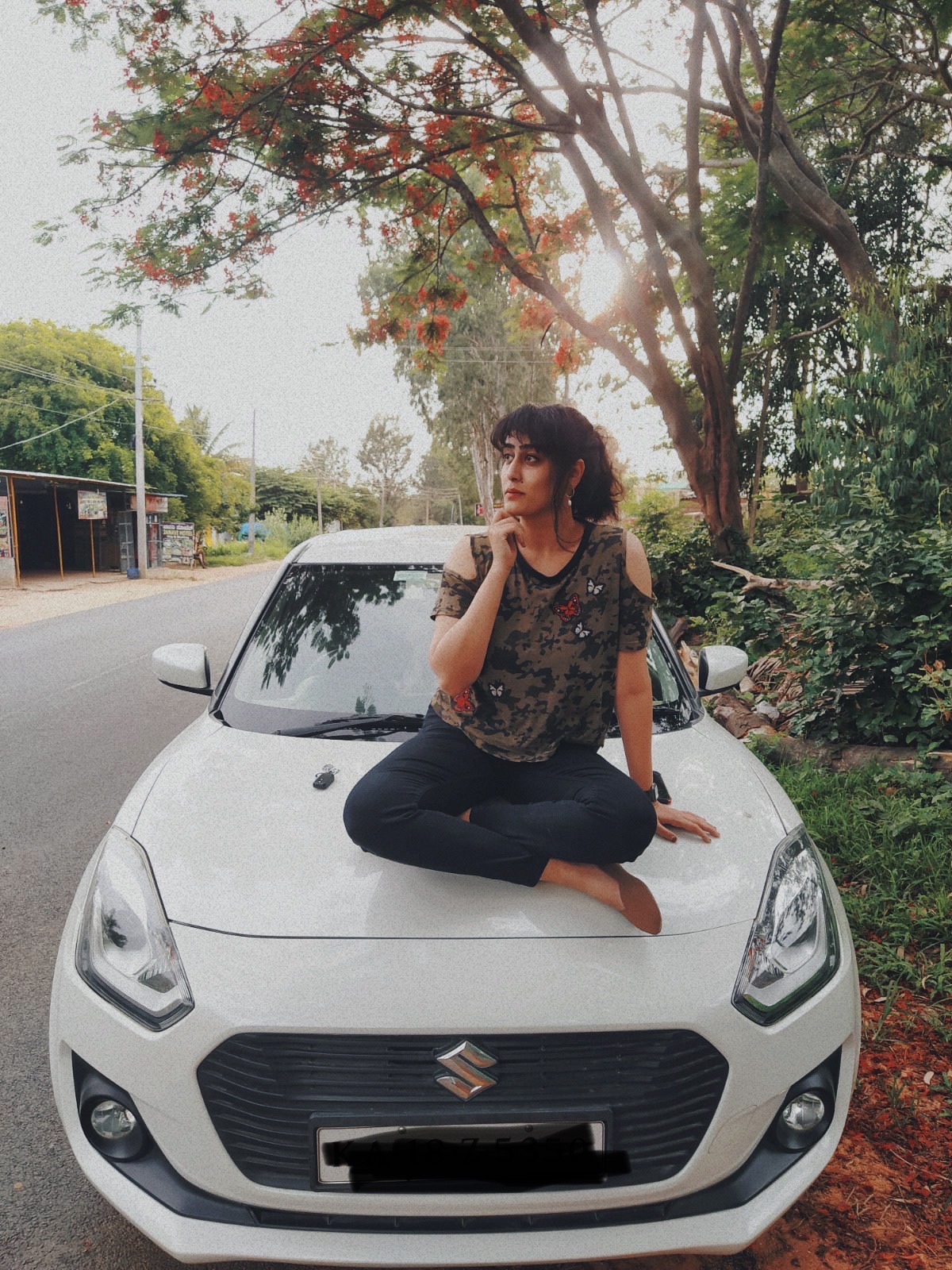
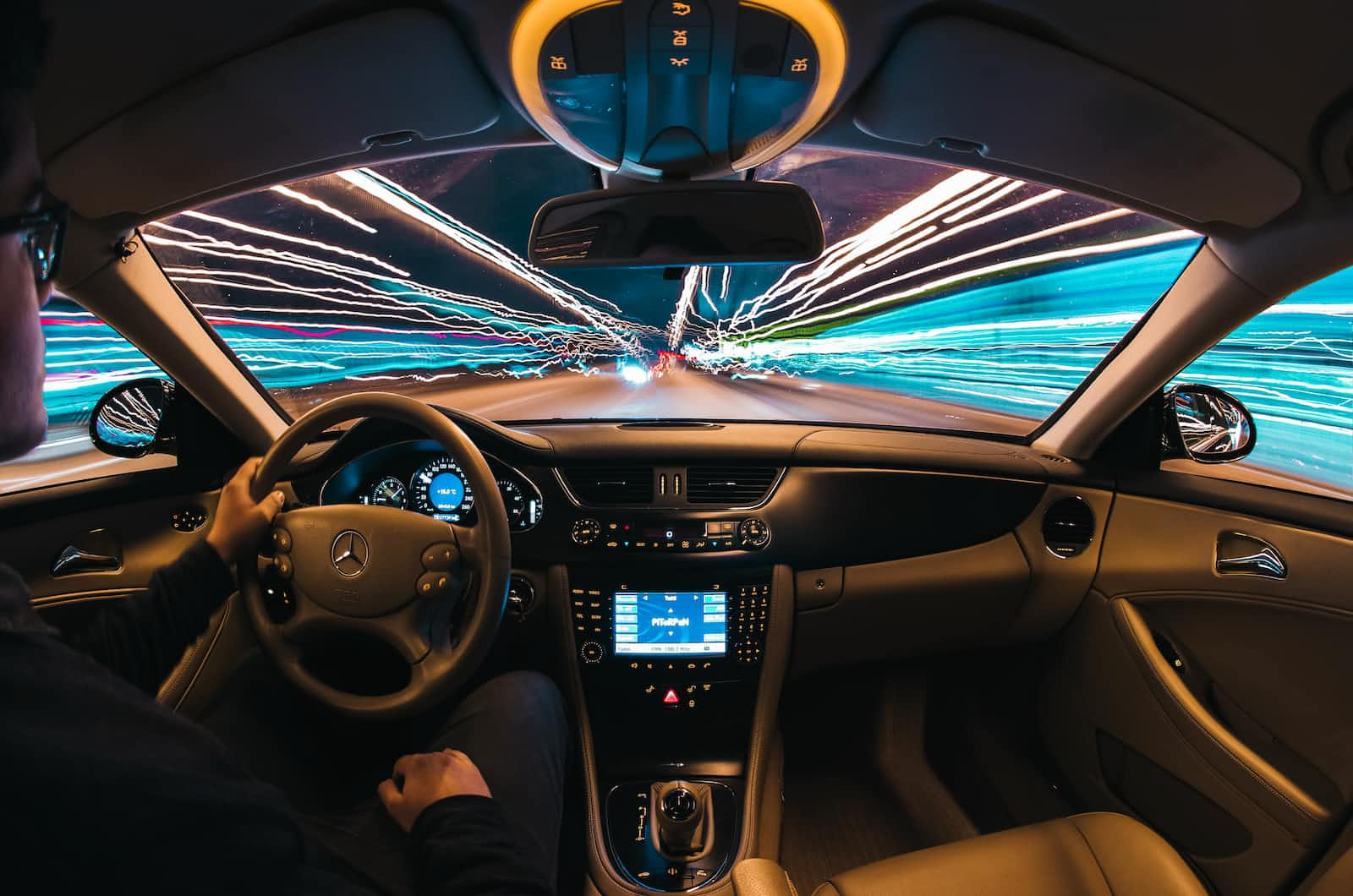
The automotive industry is on the verge of a technological revolution, with automobiles becoming sophisticated, safety-conscious machines. As the world moves toward self-driving cars and increased safety measures, Python's role in making automobiles smarter and safer becomes increasingly important. Python, the flexible and powerful programming language, is not just used for web development and data research; it is also transforming the automobile industry. In this post, we'll look at Python's role in allowing better safety features in automobiles, with an emphasis on collision avoidance, adaptive cruise control, and lane departure alerts. We'll look at some code samples to see how Python is helping us get to a safer future on the road.
Python and Collision Avoidance
Collision avoidance is an important safety element in contemporary automobiles that detects possible crashes and takes remedial steps to avoid them. Python, with its extensive library and real-time data processing capabilities, is critical in the implementation of collision avoidance systems. With a code snippet, let's look at how Python is used for collision avoidance.
Code Snippet: Collision Avoidance System using Python and OpenCV
For our example, we'll look at a rudimentary collision avoidance system that detects objects in the car's path using a single forward-facing camera. To analyze the camera stream and detect potential obstructions, we'll use the OpenCV library, a prominent Python computer vision toolkit.
import cv2
def detect_objects(image):
# Use OpenCV to detect objects in the image.
# For simplicity, let's assume the detection algorithm outputs a list of bounding boxes
# representing the positions of detected objects (e.g., pedestrians, vehicles).
detected_objects = object_detection_algorithm(image)
return detected_objects
def apply_brakes():
# Function to apply the brakes when a potential collision is detected.
print("Collision detected! Applying brakes.")
def sound_warning_alarm():
# Function to sound an audible warning to alert the driver.
print("Warning! Potential collision ahead. Please take evasive action.")
def release_brakes():
# Function to release the brakes when no potential collision is detected.
print("No obstacles detected. Releasing brakes.")
def collision_avoidance_system():
# Initialize the camera (or use pre-recorded video for simulation purposes)
camera = cv2.VideoCapture(0)
while True:
# Capture a frame from the camera feed.
ret, frame = camera.read()
# Perform object detection on the frame.
detected_objects = detect_objects(frame)
if detected_objects:
# If any objects are detected, take collision avoidance measures.
apply_brakes()
sound_warning_alarm()
else:
# If no objects are detected, release the brakes.
release_brakes()
# Display the camera feed with detected objects (for visualization purposes).
cv2.imshow("Collision Avoidance System", frame)
# Exit the loop when the user presses the 'q' key.
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# Release the camera and close all OpenCV windows.
camera.release()
cv2.destroyAllWindows()
# Start the collision avoidance system.
collision_avoidance_system()
Using Python and OpenCV, we created a rudimentary collision avoidance system in this code sample. To identify possible impediments (e.g., pedestrians, automobiles) in the video stream, the 'detect_objects' function employs an object identification method (not explicitly stated in the excerpt for clarity). If any objects are identified, the system applies the brakes and sounds a warning to inform the driver.
The system continually scans frames from the camera stream in real time, allowing it to adapt quickly to environmental changes. When no obstructions are identified, the system automatically releases the brakes, enabling the vehicle to continue regular operation.
Real-world collision avoidance systems are significantly more complicated and use many sensors, including as radar and LiDAR, to improve accuracy and dependability. Furthermore, thorough testing and validation are required to verify that such technologies are safe and effective in production-grade cars.
We can create the groundwork for more complex collision avoidance systems that contribute to making our roads safer and lowering the likelihood of accidents by utilizing Python and OpenCV.
Python and Adaptive Cruise Control (ACC)
Adaptive Cruise Control (ACC) is a sophisticated driver assistance technology that improves safety and comfort by automatically altering a vehicle's speed to maintain a safe following distance from the vehicle in front. ACC measures the distance and relative speed of the leading vehicle using sensors such as radar or lidar and then changes the car's speed appropriately. Python is an ideal choice for constructing ACC systems due to its versatility and interoperability with machine-learning technologies.
Python may be used in an ACC system to handle sensor data, create control algorithms, and even machine learning models to anticipate distances and alter speed accordingly. Let's look at some code that shows how Python may be used to construct an adaptive cruise control system.
import time
# Function to simulate capturing sensor data (distance and relative speed of the lead vehicle).
def capture_sensor_data():
distance_to_vehicle_ahead = 50 # Initial distance in meters
relative_speed_of_vehicle_ahead = 0 # Relative speed in meters per second
return distance_to_vehicle_ahead, relative_speed_of_vehicle_ahead
# Function to simulate controlling the vehicle's speed.
def control_speed(speed_difference):
# In a real-world implementation, this function would interface with the car's throttle control.
print(f"Adjusting speed by {speed_difference} m/s")
def adaptive_cruise_control():
while True:
# Capture sensor data (simulated for demonstration purposes).
distance_to_vehicle_ahead, relative_speed_of_vehicle_ahead = capture_sensor_data()
# Calculate the desired following distance based on the current speed of the car.
desired_following_distance = 2 * relative_speed_of_vehicle_ahead
# Calculate the speed difference between the current speed and the desired speed.
speed_difference = relative_speed_of_vehicle_ahead - desired_following_distance
if speed_difference > 0:
# The car is too close to the vehicle ahead; slow down to maintain a safe following distance.
control_speed(-speed_difference)
else:
# The car is at a safe following distance or farther away; maintain the current speed.
control_speed(0)
# Add a short delay to simulate real-time behavior (simulated for demonstration purposes).
time.sleep(0.5)
# Run the adaptive cruise control system.
adaptive_cruise_control()
We have a rudimentary simulation of an ACC system in this code snippet. The capture_sensor_data() method replicates the sensors that measure the lead vehicle's distance and relative speed. The adaptive_cruise_control() function determines the safe following distance based on the relative speed of the lead vehicle and then changes the car's speed to maintain that safe following distance.
In practice, the control_speed() method would communicate with the car's throttle control to change the speed correctly.
It should be noted that the above code is a simplified example for demonstration purposes only. Real-world ACC systems require more sophisticated sensor data processing, control algorithms, and connection with the vehicle's control systems.
Python's strengths, including as its data processing tools and ease of interaction with machine learning frameworks such as TensorFlow, enable engineers and developers to design advanced ACC systems that improve road safety and user experience. ACC systems will become increasingly more complex as Python evolves and researchers push the bounds of machine learning and sensor technology, contributing to the continuous revolution of the automotive industry.
Python and Lane Departure Warnings
Lane departure alerts are an important safety feature in contemporary automobiles since they assist avoid unintentional lane departures and potential crashes with other vehicles. This technology detects lane markers on the road using computer vision techniques and informs the driver if the car begins to wander out of its lane without using turn signals. Python's simplicity of installation and data processing skills are critical in developing accurate and dependable lane departure warning systems.
How Lane Departure Warnings Work
Lane departure alerts identify lane markings on the road using information from the car's cameras and image processing algorithms. Several steps are involved in the process:
Image Capture: The cameras on the automobile record real-time photos of the road ahead.
Image Processing: Python is used to analyze these photos and extract the necessary information for detecting lane markers.
Lane Marking Detection: Python recognizes lane markers such as lane lines or road margins in images using computer vision techniques.
Lane Tracking: The technology then uses the detected lane markers to identify the vehicle's location within the lane over time.
Lane Departure Warning: If the car begins to drift out of its lane without utilizing turn signals, the lane departure warning system alerts the driver with a visual alarm on the dashboard or haptic input via the steering wheel.
Code Snippet: Lane Departure Warning using Python and OpenCV
import cv2
import numpy as np
def detect_lane_markings(image):
# Convert the image to grayscale
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Apply Gaussian blur to reduce noise
blurred_image = cv2.GaussianBlur(gray_image, (5, 5), 0)
# Detect edges using Canny edge detection
edges = cv2.Canny(blurred_image, 50, 150)
# Define a region of interest (ROI) to focus on the road area
height, width = edges.shape
mask = np.zeros_like(edges)
roi_vertices = np.array([[(0, height), (width / 2, height / 2), (width, height)]], dtype=np.int32)
cv2.fillPoly(mask, roi_vertices, 255)
masked_edges = cv2.bitwise_and(edges, mask)
# Detect lines using Hough Transform
lines = cv2.HoughLinesP(masked_edges, rho=2, theta=np.pi/180, threshold=50, minLineLength=100, maxLineGap=50)
return lines
def lane_departure_warning(image):
lines = detect_lane_markings(image)
if lines is not None:
for line in lines:
x1, y1, x2, y2 = line[0]
# Calculate the slope of the line
slope = (y2 - y1) / (x2 - x1 + 1e-6)
# Define a threshold for considering a line as a lane marking
slope_threshold = 0.5
if abs(slope) > slope_threshold:
# Lane departure warning: Display an alert on the image
cv2.putText(image, 'Lane Departure Warning', (50, 50), cv2.FONT_HERSHEY_SIMPLEX, 1, (0, 0, 255), 2, cv2.LINE_AA)
break
return image
# Example: Lane Departure Warning in a video stream
cap = cv2.VideoCapture('road_video.mp4')
while cap.isOpened():
ret, frame = cap.read()
if not ret:
break
frame_with_warning = lane_departure_warning(frame)
cv2.imshow('Lane Departure Warning', frame_with_warning)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
Python uses the OpenCV package to construct a rudimentary lane departure warning system in a video stream in the code sample above. The detect_lane_markings function examines the picture for lane markings and finds them using Canny edge detection and the Hough Transform. When the slope of a line (representing a lane marker) reaches a preset threshold, the lane_departure_warning function displays a warning message on the picture, signaling a potential lane departure.
This is only a simple implementation of a lane departure warning system; real-world systems would have more features and resilience, such as filtering and smoothing techniques to increase accuracy and dependability.
Finally, Python's image processing and computer vision skills enable the creation of improved lane departure warning systems, which contribute to making automobiles smarter and safer on the road. We should expect far more advanced safety features that will impact the future of driving as Python evolves and is adopted by the car industry.
Embracing a Safer and Smarter Future on the Roads
The notion of a safer and smarter future on the roads is at the forefront of technology breakthroughs in the automobile industry. Python's role in crafting this future becomes increasingly important as demand for better safety features and autonomous driving capabilities develops. Let's look at how Python, with its code and capabilities, contributes to this idea.
1. Safer Roads with Collision Avoidance:
Python is crucial to the development of collision avoidance systems, which are critical for reducing accidents and improving road safety. These systems use real-time data from sensors and cameras to identify possible collisions and respond quickly to prevent them.
# Collision Avoidance System using Python and OpenCV
import cv2
def detect_objects(image):
# Use OpenCV to detect objects, pedestrians, and other vehicles in the image.
# Perform image processing and object detection.
return detected_objects
def collision_avoidance_system():
while True:
image = capture_image_from_camera() # Function to capture live images from the car's cameras.
detected_objects = detect_objects(image)
if detected_objects:
# Analyze the detected objects and make real-time decisions to avoid collisions.
apply_brakes()
sound_warning_alarm()
else:
release_brakes()
collision_avoidance_system()
Python is used with the OpenCV package in this code sample to recognize objects from the car's cameras in real-time. If any objects, such as pedestrians or automobiles, are recognized within a dangerous distance, the collision avoidance system reacts immediately, applying brakes or sounding warning bells to avoid a collision.
2. Smarter Driving with Adaptive Cruise Control (ACC):
Python is an ideal choice for constructing adaptive cruise control (ACC) systems due to its flexibility and simplicity of interaction with machine learning frameworks. By automatically altering the car's speed, ACC maintains a safe following distance from the vehicle ahead.
# Adaptive Cruise Control using Python and TensorFlow
import tensorflow as tf
def predict_distance_to_vehicle(image):
# Use a pre-trained machine learning model (e.g., CNN) to predict the distance to the vehicle ahead.
model = tf.keras.models.load_model('acc_model.h5')
distance = model.predict(image)
return distance
def adaptive_cruise_control():
while True:
image = capture_image_from_camera() # Function to capture live images from the car's cameras.
distance_to_vehicle_ahead = predict_distance_to_vehicle(image)
if distance_to_vehicle_ahead < safe_distance_threshold:
reduce_speed()
else:
maintain_speed()
adaptive_cruise_control()
Python is used in this example in conjunction with the TensorFlow package to forecast the distance to the vehicle ahead based on pictures recorded by the car's cameras. If the estimated distance is less than a safe threshold, the adaptive cruise control system slows the vehicle down to maintain a safe following distance.
3. Lane Departure Warnings for Enhanced Safety:
Lane departure alerts are critical safety elements that aid in the prevention of unintentional lane departures and accidents with other cars. Python's ease of installation and data processing skills aid in the development of accurate lane departure warning systems.
# Lane Departure Warning using Python and NumPy
import numpy as np
def detect_lane_departure(image):
# Use computer vision techniques to detect lane markings in the image.
lane_markings = detect_lane_markings(image)
return lane_markings
def lane_departure_warning():
while True:
image = capture_image_from_camera() # Function to capture live images from the car's cameras.
lane_markings = detect_lane_departure(image)
if lane_markings is None:
no_lane_detected_warning()
else:
if not lane_within_safe_limits(lane_markings):
lane_departure_warning_alert()
lane_departure_warning()
Python recognizes lane markers from the car's camera feed in this code sample using NumPy and machine vision methods. The lane departure warning system warns the driver if no lane markings are detected or if the vehicle deviates from the lane.
Conclusion:
Python's capacity to analyze data, interact with strong libraries, and handle real-time applications is propelling us toward a more secure and intelligent future on the road. Python is revolutionizing the automotive scene and contributing to a driving experience that prioritizes safety and economy, from collision avoidance to adaptive cruise control and lane departure alerts.
Python will play an increasingly more important part in determining the future of smart and safe driving as technology advances. Accepting these advances will pave the way for a future in which accidents are reduced, traffic is properly handled, and cars become more than simply forms of transportation—they become intelligent companions on our trips.
Disclaimer: The code samples in this article are provided solely for illustrative reasons and may not be directly applicable to production-grade systems. Real-world safety-critical systems need extensive testing, validation, and compliance with industry standards.
Let us all work together to make the future safer and smarter!
Subscribe to my newsletter
Read articles from Ayesha Siddiqha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
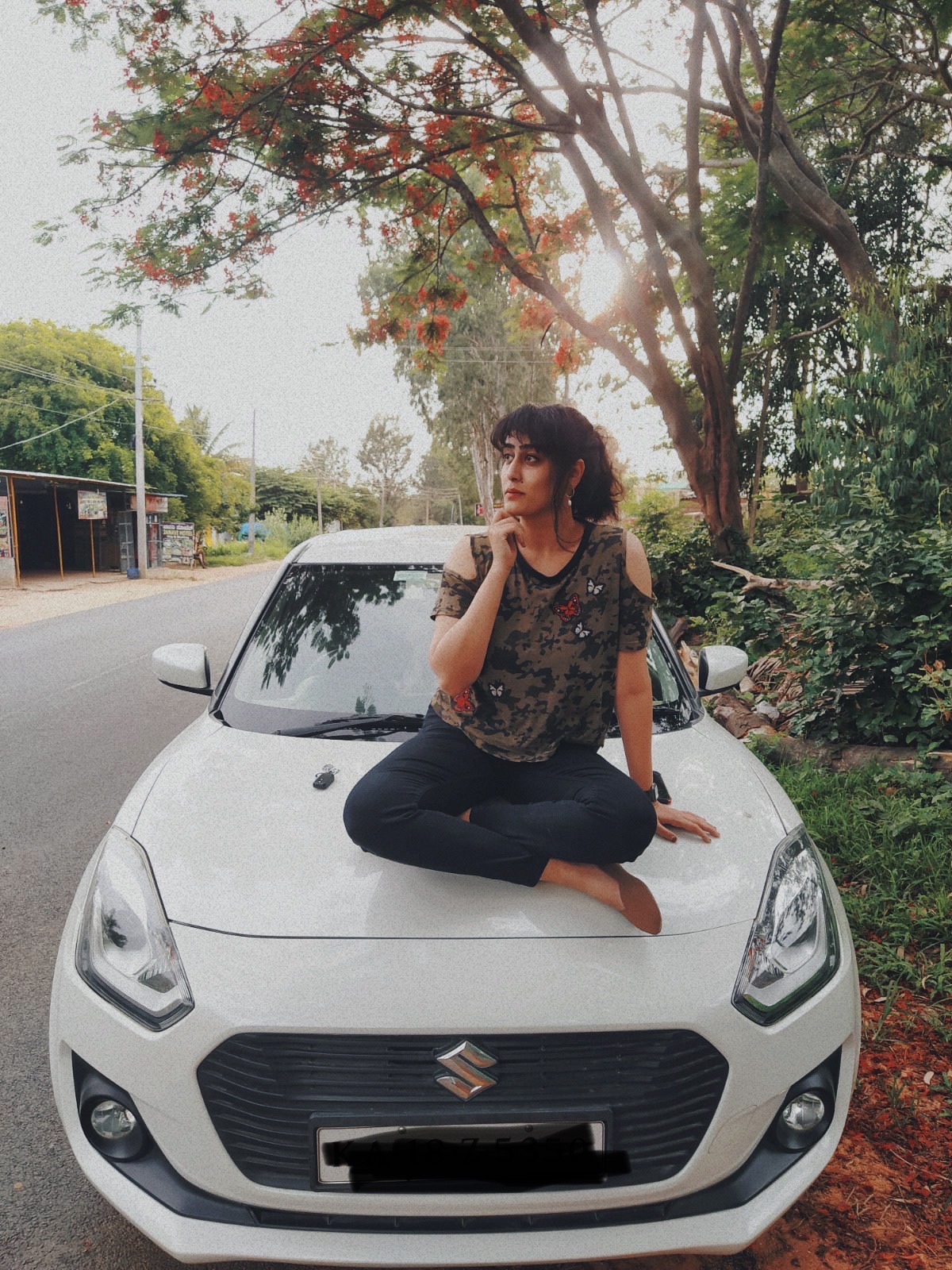
Ayesha Siddiqha
Ayesha Siddiqha
Results-driven Python Developer with a background in data analysis and machine learning. Skilled in utilizing Python libraries such as NumPy, Pandas, Matplotlib, Psycopg2, seaborn and sqlAlchemy I have experience in developing data pipelines and implementing predictive models. I thrive in data-driven environments, where I can leverage my strong analytical skills to extract insights and make informed decisions. Committed to continuous improvement, I stay updated on the latest advancements in Python and machine learning techniques. Let's harness the power of data and drive impactful outcomes.