Linked List Series

Table of contents
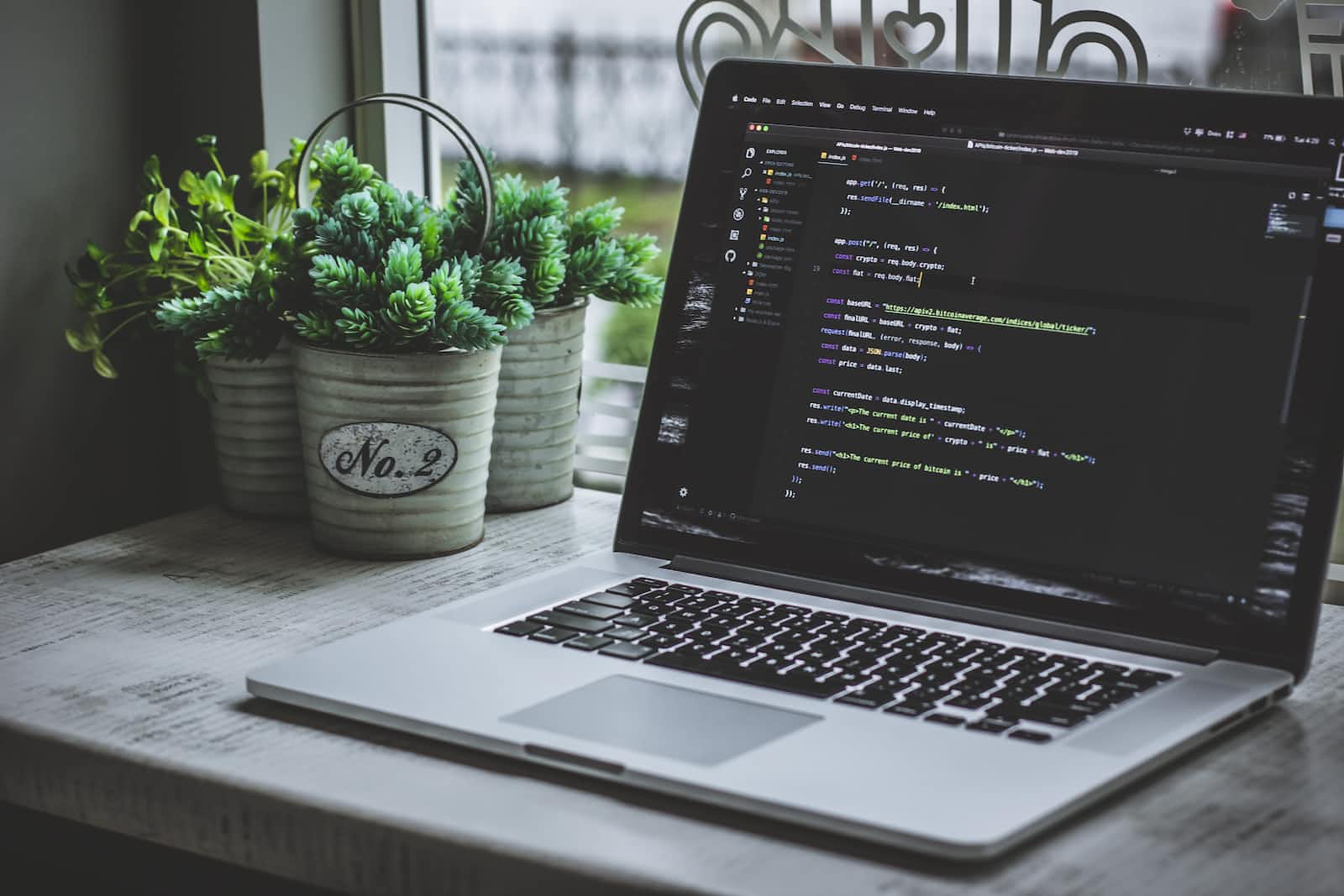
So, what are the drawbacks of using sequential storage to represent stacks and queues?
One major drawback is that a fixed amount of storage remains allocated to the stack or queue even when the structure is using a smaller amount or possibly no storage at all.
A Linked List is a linear data structure that consists of a sequence of nodes, where each node contains two components: the data and a reference (or pointer) to the next node in the sequence. The first node is called the head, and the last node in the list contains a special value, known as null, which is not a valid address. This null pointer is used to signal the end of a list. This chain of nodes enables a dynamic and flexible way to organize data, unlike arrays, which have a fixed size.
Advantages of using Linked Lists:
Dynamic Memory Allocation: Linked lists allow for dynamic memory allocation, meaning that memory for elements is allocated on-demand as new nodes are added. This flexibility enables efficient memory usage, as only the required memory is utilized, unlike arrays that have a fixed size.
Efficient Insertions and Deletions: Linked lists excel at insertions and deletions, especially when performed in the middle of the list. These operations typically have a time complexity of O(1) when dealing with nodes with known references (e.g., doubly linked lists) since no shifting of elements is necessary.
Variable Size: Unlike arrays, linked lists can grow or shrink in size during program execution, accommodating changing data requirements. This adaptability is particularly valuable when dealing with data of unknown or varying sizes.
No Memory Wastage: In linked lists, memory fragmentation is less of an issue since each element can be scattered in memory, and the data structure itself does not require contiguous memory blocks like arrays.
Scalability: Linked lists perform well even with a large number of elements. As long as the references between nodes are properly maintained, the time complexity of accessing elements remains constant.
There are also some disadvantages to using a Linked list.
Inefficient Random Access: Unlike arrays, accessing elements in a linked list requires traversing the list from the head (or tail) until the desired element is reached. This results in a linear time complexity of O(n) for accessing elements at arbitrary positions, making linked lists inefficient for random access.
Lack of Cache Locality: Linked lists do not exhibit good cache locality due to scattered memory locations of individual nodes. This can result in increased cache misses during traversal, leading to slower performance compared to contiguous data structures like arrays.
Memory Fragmentation: Over time, linked lists can lead to memory fragmentation, as nodes are scattered throughout the memory. This can cause memory inefficiency and increase the risk of memory leaks in some cases.
Not Suitable for Index-Based Operations: Linked lists are not ideal for index-based operations (e.g., getting or setting elements at a specific index) since accessing elements by index requires traversing the list from the beginning.
Types of Linked Lists:
Singly Linked List: In a singly linked list, each node points to the next node in the sequence, forming a unidirectional chain. The last node's reference points to null, indicating the end of the list.
Doubly Linked List: Unlike a singly linked list, a doubly linked list has nodes with two references – one to the next node and another to the previous node. This bidirectional linkage allows for easier traversal in both directions.
Circular Linked List: A circular linked list is similar to a singly linked list, except that the last node's reference points back to the first node, creating a circular structure. This circular connection facilitates continuous traversal.
Conclusion:
Linked Lists serve as the backbone of many essential data structures and algorithms, and a solid understanding of their principles is crucial for any programmer or software developer. We have explored the definition of linked lists, their advantages, and the various types they come in, including singly linked lists, doubly linked lists, and circular linked lists. As you progress in your coding journey, you will encounter linked lists in numerous real-world applications, and mastering this data structure will undoubtedly enhance your problem-solving capabilities. So, keep practicing, experimenting, and embracing the power of linked lists in your coding endeavors!
In the next blog, I will write about the various operation on Linked List.
Subscribe to my newsletter
Read articles from Ankur kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Ankur kumar
Ankur kumar
Storyteller || Technology || Software Developer || Code.