How do you fill a PHP array dynamically (PHP, array, development)?

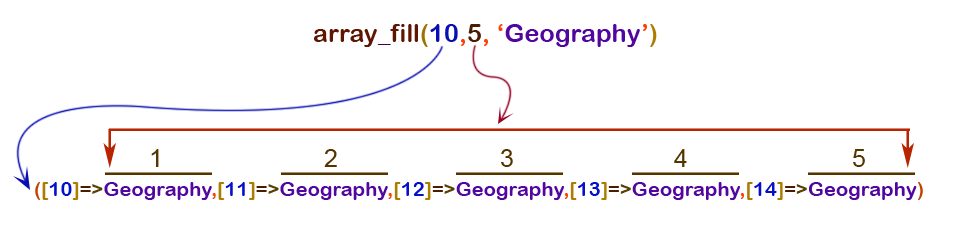
Filling a PHP array dynamically involves adding elements to the array at runtime. This is typically done using a loop or by fetching data from a database or another data source. Here's a basic explanation of how you can accomplish this:
1. Define an empty array
First, you need to define an empty array to which you'll add elements dynamically. Here's how you do that:
$myArray = array();
2. Add elements to the array
Next, you can add elements to the array. Here are several common ways to do this:
Direct assignment: You can add an element to the array using direct assignment.
$myArray[] = 'value1'; // This will add 'value1' to the array $myArray[] = 'value2'; // This
Inside a loop: If you want to fill the array with multiple values (say from 1 to 10), you could use a loop.
for ($i = 1; $i <= 10; $i++)
{ $myArray[] = $i; }
Now
$myArray
would contain the numbers from 1 to 10.From a database: If you're retrieving data from a database (let's say with MySQLi), you could add the results to an array.
$dbConnection = new mysqli('localhost', 'username', 'password', 'database');
if ($dbConnection->connect_error)
{ die("Connection failed: " . $dbConnection->connect_error);
}
$sql = "SELECT column FROM table"; $result = $dbConnection->query($sql);
if ($result->num_rows > 0)
{ while($row = $result->fetch_assoc()) { $myArray[] = $row['column'];
}
}
$dbConnection->close();
In this case,
$myArray
would be filled with the values from thecolumn
oftable
in your database.3. Use the array
Once the array is filled, you can use it however you want in your code. For example, you can print out all the elements:
foreach ($myArray as $value) { echo $value . "\n"; }
Remember that PHP arrays are dynamic, meaning that they can grow and shrink as needed during the execution of a script. This allows you to add elements to the array whenever you need to, without having to define its size ahead of time.
Please note that this is a simplified explanation and actual implementation can vary based on your use case and the state of your PHP environment.
Subscribe to my newsletter
Read articles from Vinh Jacker directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vinh Jacker
Vinh Jacker
Hello, I'm the Chief Technology Officer of Mageplaza, and I am thrilled to share my story with you. My deep love and passion for technology have fueled my journey as a professional coder and an ultra-marathon runner. Over the past decade, I have accumulated extensive experience and honed my expertise in PHP development. 100 Church St, Manhattan, New York, United States