Adding Large Numbers as Strings in JavaScript: Step-by-Step Guide
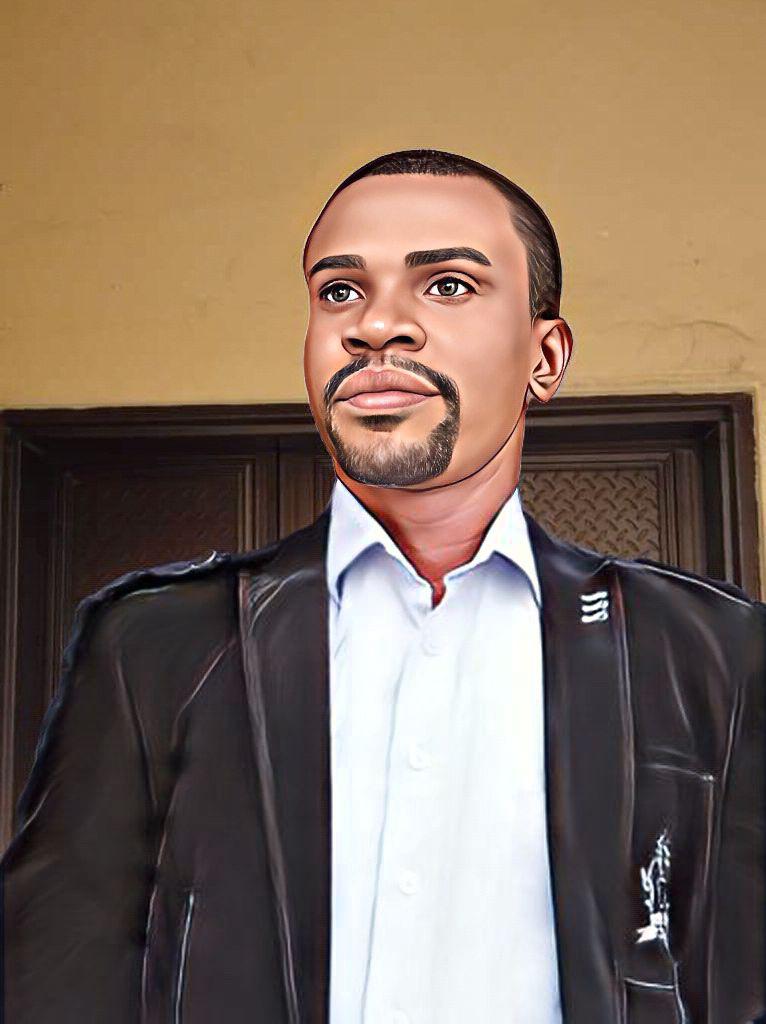
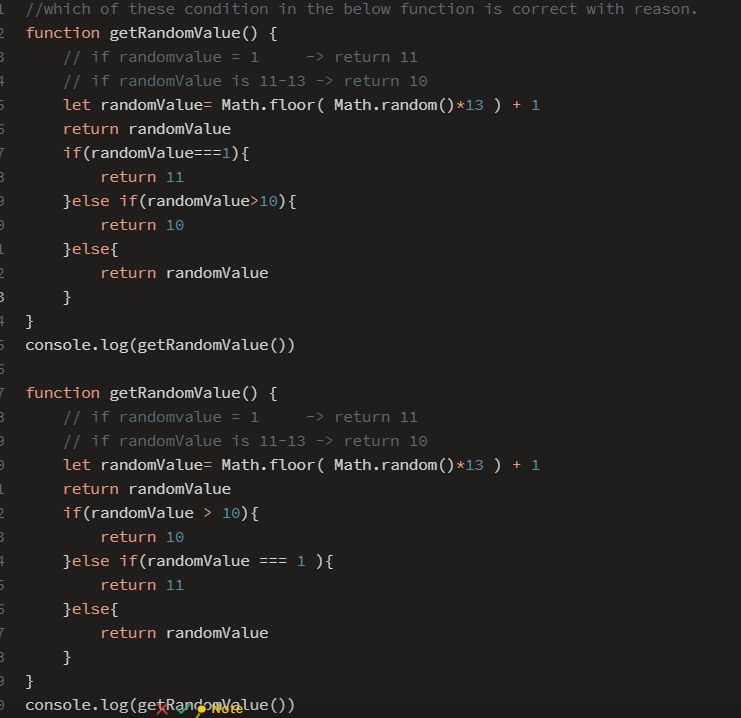
Introduction: Adding two non-negative integers, especially when they are large, can be challenging in programming. In JavaScript, the numbers are represented as strings when they exceed the limits of numerical precision. In this blog post, we'll walk through a step-by-step guide on how to add two non-negative integers represented as strings in JavaScript, providing a solution for the given problem.
Problem Statement: Given two non-negative integers num1
and num2
, both represented as strings, our goal is to return the sum of num1
and num2
as a string.
Example: Input: num1 = "12345678901234567890", num2 = "9876543210987654321" Output: "22222222113222222211"
Solution Approach: To solve this problem, we'll perform addition digit by digit, starting from the least significant digit (rightmost) and moving towards the most significant digit (leftmost). We'll use pointers to traverse the input strings from right to left, ensuring that we add digits at corresponding positions, just like how we perform addition manually.
Step-by-Step Iteration:
Initialize variables:
i
andj
as pointers for the last digits ofnum1
andnum2
, respectively.carry
to keep track of any carryover that occurs during addition.result
as an empty string, where we'll store the sum as we build it digit by digit.
Example: num1 = "12345678901234567890", num2 = "9876543210987654321"
Sure! Let's illustrate the step-by-step iteration of the solution using the example from the blog post. We will add the two non-negative integers represented as strings: num1 = "12345678901234567890"
and num2 = "9876543210987654321"
. We'll walk through each iteration and show how the result is concatenated with the existing result.
Title: Adding Large Numbers as Strings in JavaScript: Step-by-Step Guide
Introduction: Adding two non-negative integers, especially when they are large, can be challenging in programming. In JavaScript, the numbers are represented as strings when they exceed the limits of numerical precision. In this blog post, we'll walk through a step-by-step guide on how to add two non-negative integers represented as strings in JavaScript, providing a solution for the given problem.
Problem Statement: Given two non-negative integers num1
and num2
, both represented as strings, our goal is to return the sum of num1
and num2
as a string.
Example: Input: num1 = "12345678901234567890", num2 = "9876543210987654321" Output: "22222222113222222211"
Solution Approach: To solve this problem, we'll perform addition digit by digit, starting from the least significant digit (rightmost) and moving towards the most significant digit (leftmost). We'll use pointers to traverse the input strings from right to left, ensuring that we add digits at corresponding positions, just like how we perform addition manually.
Step-by-Step Iteration:
Initialize variables:
i
andj
as pointers for the last digits ofnum1
andnum2
, respectively.carry
to keep track of any carryover that occurs during addition.result
as an empty string, where we'll store the sum as we build it digit by digit.
Example: num1 = "12345678901234567890", num2 = "9876543210987654321"
javascriptCopy codelet i = num1.length - 1; // 19 (index of last digit in num1)
let j = num2.length - 1; // 18 (index of last digit in num2)
let carry = 0;
let result = ""; // Start with an empty result string
- Iterate through the strings using a loop:
javascriptCopy codewhile (i >= 0 || j >= 0 || carry > 0) {
// ... Steps inside the loop ...
}
- Extract the current digits:
javascriptCopy code// Extract the current digits at pointers i and j
const digit1 = i >= 0 ? parseInt(num1[i]) : 0; // 0 (since i is less than 0)
const digit2 = j >= 0 ? parseInt(num2[j]) : 1; // 1 (digit at index 18 in num2)
- Perform addition with carry:
javascriptCopy code// Calculate the sum of the current digits and the carry from the previous step
const sum = digit1 + digit2 + carry; // 1 + 0 + 0 = 1
- Update the result:
javascriptCopy code// The modulo 10 operation gives us the last digit of the sum (single-digit result).
// Concatenate this digit to the left of the result string
result = (sum % 10) + result; // "1" + ""
- Calculate the carry for the next iteration:
javascriptCopy code// The integer division by 10 gives us the carry for the next step.
carry = Math.floor(sum / 10); // Math.floor(1 / 10) = 0
- Move the pointers to the left:
javascriptCopy codei--; // Move the pointer i to the left (to index 18)
j--; // Move the pointer j to the left (to index 17)
Continue the loop:
- The loop will run for each digit, adding the corresponding digits of
num1
andnum2
, considering any carryover from the previous step.
- The loop will run for each digit, adding the corresponding digits of
Return the result:
- The final result will be the
result
string, containing the sum of the two input strings as a string.
- The final result will be the
here is the solution without any explanation
function addStrings(num1, num2) {
let i = num1.length - 1;
let j = num2.length - 1;
let carry = 0; let result = "";
while (i >= 0 || j >= 0 || carry > 0) {
const digit1 = i >= 0 ? parseInt(num1[i]) : 0;
const digit2 = j >= 0 ? parseInt(num2[j]) : 0;
const sum = digit1 + digit2 + carry;
result = (sum % 10) + result; carry =
Math.floor(sum / 10);
i--; j--;
}
return result;
}
const num1 = "12345678901234567890";
const num2 = "9876543210987654321";
const sum = addStrings(num1, num2);
console.log(sum); // Output: "22222222113222222211"
Conclusion: By following this step-by-step guide, we've successfully solved the problem of adding two non-negative integers represented as strings in JavaScript. This approach handles large numbers gracefully and efficiently, ensuring correct results while preserving the original representation as strings. Implementing this solution will enable you to perform addition on large numbers, which is useful in various programming scenarios. Happy coding!
Subscribe to my newsletter
Read articles from Engr Ohazulike Stanley directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
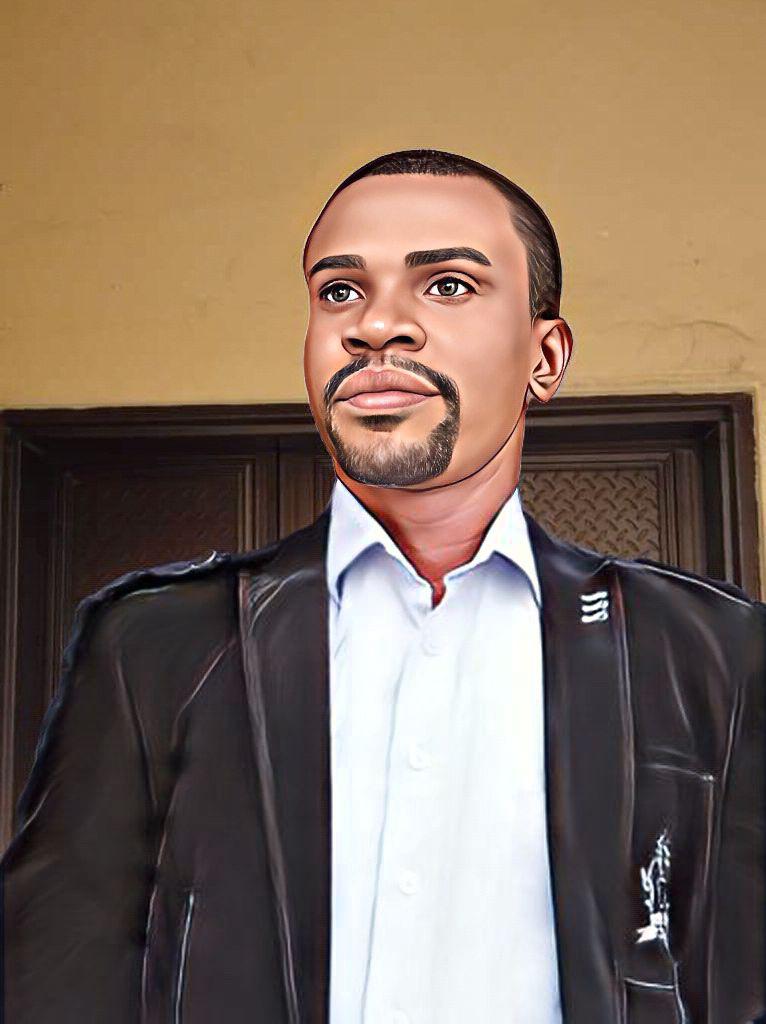
Engr Ohazulike Stanley
Engr Ohazulike Stanley
Frontend developer. HTML | CSS | JS | React. Frontend developer, with experience developing websites and web applications