Building High-Performance React Applications: Best Practices and Proven Strategies
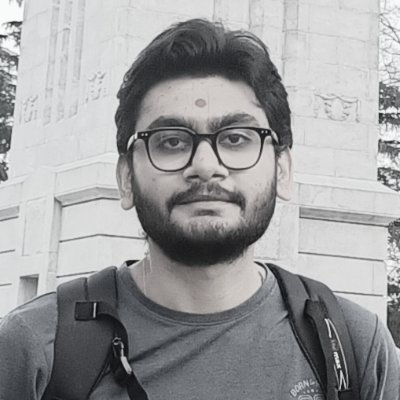
Table of contents
- Introduction
- Step 1: Project Setup
- Step 2: Folder Structure
- Step 3: Component Architecture
- Step 4: State Management with Context
- Step 5: Routing with React Router
- Step 6: Styling with CSS Modules
- Step 7: Data Fetching and API Integration
- Step 8: Managing State with Redux
- Step 9: Adding Routing and Navigation
- Step 10: Integrating APIs and Fetching Data
- Step 11: Error Handling and Loading States
- Step 12: Styling with CSS-in-JS (Styled Components)
- Conclusion
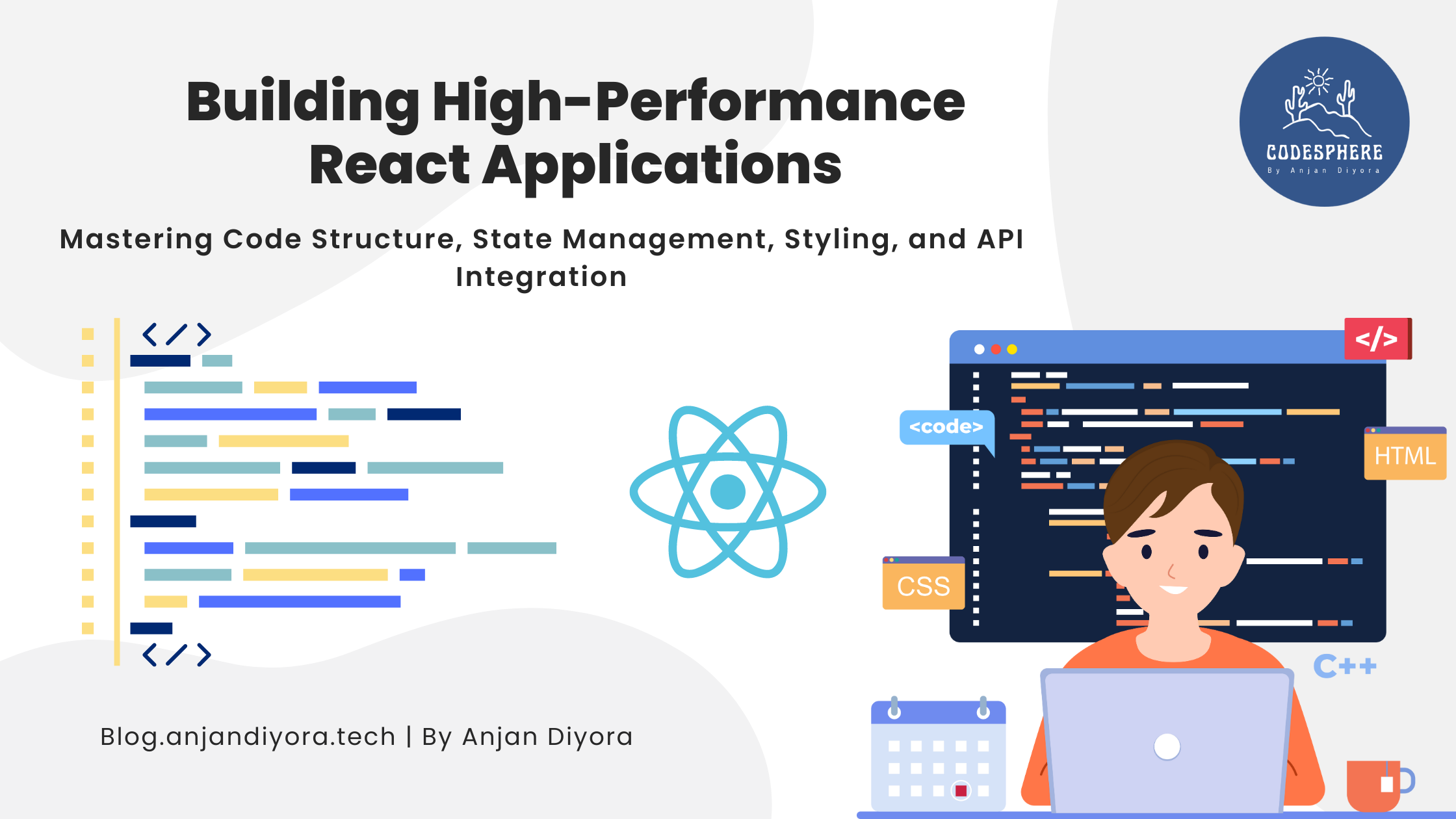
Introduction
ReactJS has revolutionized web development by providing a powerful framework for building dynamic and interactive user interfaces. However, as projects grow in complexity, maintaining a well-organized codebase becomes essential. In this guide, we'll walk you through the step-by-step process of building and structuring an industry-grade React project. We'll cover everything from project setup to component architecture, state management, routing, and more. Let's get started!
Step 1: Project Setup
1.1. Install Node.js and npm (Node Package Manager) on your machine if you haven't already. You can download them from the official Node.js website.
1.2. Create a new React application using Create React App, a popular tool for bootstrapping React projects. Open your terminal and run the following command:
npx create-react-app industry-project
1.3. Navigate into your project directory:
cd industry-project
Your project is now set up and ready to go.
Step 2: Folder Structure
2.1. Create a clear folder structure to keep your code organized. Here's a suggested structure:
industry-project/
├── src/
│ ├── components/
│ ├── pages/
│ ├── styles/
│ ├── utils/
│ ├── context/
│ ├── hooks/
│ ├── App.js
│ ├── index.js
│ ├── routes.js
│ └── config.js
└── package.json
2.2. Inside the src
folder:
components: Store reusable UI components.
pages: Create page components that represent different views of your app.
styles: Place global styles, variables, and CSS files.
utils: Store utility functions and helper modules.
context: Create React Context providers for managing global state.
hooks: Define custom hooks to encapsulate shared logic.
App.js: Set up the main structure of your app.
index.js: Render your app into the DOM.
routes.js: Configure routes using React Router or other routing libraries.
config.js: Store app-specific configurations.
Step 3: Component Architecture
3.1. Build reusable components that encapsulate specific functionality. For example, let's create a simple Button
component:
// src/components/Button.js
import React from 'react';
import './Button.css';
const Button = ({ text, onClick }) => {
return (
<button className="button" onClick={onClick}>
{text}
</button>
);
};
export default Button;
3.2. Use the Button
component in a page:
// src/pages/Home.js
import React from 'react';
import Button from '../components/Button';
const Home = () => {
const handleClick = () => {
alert('Button clicked!');
};
return (
<div>
<h1>Welcome to My App</h1>
<Button text="Click me" onClick={handleClick} />
</div>
);
};
export default Home;
Step 4: State Management with Context
4.1. Set up a global state using React Context to manage data that needs to be accessed by multiple components:
// src/context/ThemeContext.js
import React, { createContext, useContext, useState } from 'react';
const ThemeContext = createContext();
export const useTheme = () => {
return useContext(ThemeContext);
};
export const ThemeProvider = ({ children }) => {
const [theme, setTheme] = useState('light');
const toggleTheme = () => {
setTheme(prevTheme => (prevTheme === 'light' ? 'dark' : 'light'));
};
return (
<ThemeContext.Provider value={{ theme, toggleTheme }}>
{children}
</ThemeContext.Provider>
);
};
4.2. Wrap your App
component with the ThemeProvider
to provide access to the theme state:
// src/App.js
import React from 'react';
import { ThemeProvider } from './context/ThemeContext';
import Home from './pages/Home';
import './App.css';
const App = () => {
return (
<ThemeProvider>
<div className="app">
<Home />
</div>
</ThemeProvider>
);
};
export default App;
Step 5: Routing with React Router
5.1. Install React Router if not already installed:
npm install react-router-dom
5.2. Configure routing in your routes.js
file:
// src/routes.js
import React from 'react';
import { BrowserRouter, Switch, Route } from 'react-router-dom';
import Home from './pages/Home';
import About from './pages/About';
const Routes = () => {
return (
<BrowserRouter>
<Switch>
<Route path="/" exact component={Home} />
<Route path="/about" component={About} />
</Switch>
</BrowserRouter>
);
};
export default Routes;
5.3. Update your App.js
to include the Routes
component:
// src/App.js
import React from 'react';
import { ThemeProvider } from './context/ThemeContext';
import Routes from './routes';
import './App.css';
const App = () => {
return (
<ThemeProvider>
<div className="app">
<Routes />
</div>
</ThemeProvider>
);
};
export default App;
Step 6: Styling with CSS Modules
6.1. Use CSS Modules to scope styles to individual components:
/* src/components/Button.module.css */
.button {
background-color: var(--primary-color);
color: white;
padding: 10px 20px;
border: none;
cursor: pointer;
}
6.2. Import and use the styles in your component:
// src/components/Button.js
import React from 'react';
import styles from './Button.module.css';
const Button = ({ text, onClick }) => {
return (
<button className={styles.button} onClick={onClick}>
{text}
</button>
);
};
export default Button;
Step 7: Data Fetching and API Integration
7.1. Fetch data from an API using the fetch
function or a library like Axios:
// src/utils/api.js
export const fetchData = async () => {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
return data;
} catch (error) {
console.error('Error fetching data:', error);
return null;
}
};
7.2. Use the fetched data in your component:
// src/pages/Home.js
import React, { useEffect, useState } from 'react';
import Button from '../components/Button';
import { fetchData } from '../utils/api';
const Home = () => {
const [data, setData] = useState([]);
useEffect(() => {
fetchData().then(result => {
if (result) {
setData(result);
}
});
}, []);
return (
<div>
<h1>Welcome to My App</h1>
<ul>
{data.map(item => (
<li key={item.id}>{item.name}</li>
))}
</ul>
<Button text="Click me" />
</div>
);
};
export default Home;
Step 8: Managing State with Redux
8.1. Install Redux and React Redux libraries:
npm install redux react-redux
8.2. Create a Redux store, reducer, and actions for managing global state:
// src/redux/store.js
import { createStore } from 'redux';
import rootReducer from './reducers';
const store = createStore(rootReducer);
export default store;
// src/redux/reducers.js
const initialState = {
theme: 'light',
data: [],
};
const rootReducer = (state = initialState, action) => {
switch (action.type) {
case 'TOGGLE_THEME':
return { ...state, theme: state.theme === 'light' ? 'dark' : 'light' };
case 'SET_DATA':
return { ...state, data: action.payload };
default:
return state;
}
};
export default rootReducer;
// src/redux/actions.js
export const toggleTheme = () => ({
type: 'TOGGLE_THEME',
});
export const setData = data => ({
type: 'SET_DATA',
payload: data,
});
8.3. Wrap your App
component with the Provider
from react-redux
:
// src/App.js
import React from 'react';
import { Provider } from 'react-redux';
import store from './redux/store';
import Routes from './routes';
import './App.css';
const App = () => {
return (
<Provider store={store}>
<div className="app">
<Routes />
</div>
</Provider>
);
};
export default App;
8.4. Use Redux state and actions in your components:
// src/pages/Home.js
import React, { useEffect } from 'react';
import { useSelector, useDispatch } from 'react-redux';
import Button from '../components/Button';
import { fetchData } from '../utils/api';
import { toggleTheme, setData } from '../redux/actions';
const Home = () => {
const theme = useSelector(state => state.theme);
const data = useSelector(state => state.data);
const dispatch = useDispatch();
useEffect(() => {
fetchData().then(result => {
if (result) {
dispatch(setData(result));
}
});
}, [dispatch]);
const handleThemeToggle = () => {
dispatch(toggleTheme());
};
return (
<div className={`home ${theme}`}>
<h1>Welcome to My App</h1>
<ul>
{data.map(item => (
<li key={item.id}>{item.name}</li>
))}
</ul>
<Button text="Toggle Theme" onClick={handleThemeToggle} />
</div>
);
};
export default Home;
Step 9: Adding Routing and Navigation
9.1. Install React Router if not already installed:
npm install react-router-dom
9.2. Configure routing in your routes.js
file:
// src/routes.js
import React from 'react';
import { BrowserRouter, Switch, Route, Link } from 'react-router-dom';
import Home from './pages/Home';
import About from './pages/About';
const Routes = () => {
return (
<BrowserRouter>
<nav>
<ul>
<li>
<Link to="/">Home</Link>
</li>
<li>
<Link to="/about">About</Link>
</li>
</ul>
</nav>
<Switch>
<Route path="/" exact component={Home} />
<Route path="/about" component={About} />
</Switch>
</BrowserRouter>
);
};
export default Routes;
9.3. Update your App.js
to include the Routes
component:
// src/App.js
import React from 'react';
import { Provider } from 'react-redux';
import store from './redux/store';
import Routes from './routes';
import './App.css';
const App = () => {
return (
<Provider store={store}>
<div className="app">
<Routes />
</div>
</Provider>
);
};
export default App;
Step 10: Integrating APIs and Fetching Data
10.1. Install Axios, a popular library for making API requests:
npm install axios
10.2. Create a utility function to fetch data using Axios:
// src/utils/api.js
import axios from 'axios';
export const fetchData = async () => {
try {
const response = await axios.get('https://api.example.com/data');
return response.data;
} catch (error) {
console.error('Error fetching data:', error);
return null;
}
};
10.3. Use the fetched data in your component:
// src/pages/Home.js
import React, { useEffect, useState } from 'react';
import Button from '../components/Button';
import { fetchData } from '../utils/api';
const Home = () => {
const [data, setData] = useState([]);
useEffect(() => {
fetchData().then(result => {
if (result) {
setData(result);
}
});
}, []);
return (
<div>
<h1>Welcome to My App</h1>
<ul>
{data.map(item => (
<li key={item.id}>{item.name}</li>
))}
</ul>
<Button text="Click me" />
</div>
);
};
export default Home;
Step 11: Error Handling and Loading States
11.1. Implement error handling and loading states when fetching data:
// src/pages/Home.js
import React, { useEffect, useState } from 'react';
import Button from '../components/Button';
import { fetchData } from '../utils/api';
const Home = () => {
const [data, setData] = useState([]);
const [loading, setLoading] = useState(true);
const [error, setError] = useState(null);
useEffect(() => {
fetchData()
.then(result => {
if (result) {
setData(result);
}
})
.catch(err => {
setError(err);
})
.finally(() => {
setLoading(false);
});
}, []);
if (loading) {
return <p>Loading...</p>;
}
if (error) {
return <p>Error: {error.message}</p>;
}
return (
<div>
<h1>Welcome to My App</h1>
<ul>
{data.map(item => (
<li key={item.id}>{item.name}</li>
))}
</ul>
<Button text="Click me" />
</div>
);
};
export default Home;
Step 12: Styling with CSS-in-JS (Styled Components)
12.1. Install Styled Components:
npm install styled-components
12.2. Create a styled component for the Button
:
// src/components/Button.js
import React from 'react';
import styled from 'styled-components';
const StyledButton = styled.button`
background-color: var(--primary-color);
color: white;
padding: 10px 20px;
border: none;
cursor: pointer;
`;
const Button = ({ text, onClick }) => {
return <StyledButton onClick={onClick}>{text}</StyledButton>;
};
export default Button;
Conclusion
Congratulations! You've successfully continued your journey of building and structuring an industry-grade React project. In this section, you learned how to integrate APIs, fetch data, handle errors, and implement loading states. You also explored styling with CSS-in-JS using Styled Components, enhancing the visual appeal of your components.
As you continue to refine your React skills, remember that practice, experimentation, and continuous learning are key to becoming a proficient React developer. Each step you take brings you closer to mastering React and creating remarkable web applications that meet industry standards. Keep exploring, building, and pushing the boundaries of your knowledge. Happy coding!
Subscribe to my newsletter
Read articles from Anjan Diyora (AD) directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
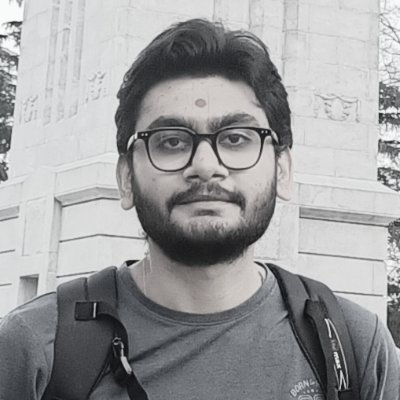
Anjan Diyora (AD)
Anjan Diyora (AD)
🚀 Software Engineer 🌐 Crafting cutting-edge software and network solutions that integrate AI seamlessly. Let's innovate together! 💻🧠🌟