Function and Case (in Shell Script)

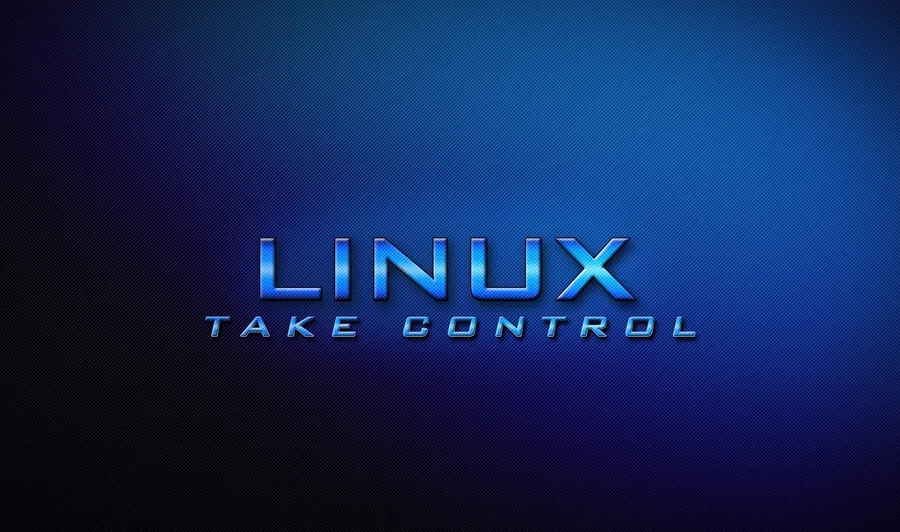
Hey, What's up guys, Sarthak here, Welcome back to another blog. In this blog, I will cover all the relevant points that have been covered in today's Linux Workshop, carried out byPranav Jambare at Dr.Babasaheb Ambedkar Technological University, Lonere. Those who didn't check my previous blogs please check it out first.
On the last day of the workshop, the following points were covered.
Functions
Cases
Functions
In shell scripting, a function is a reusable block of code that performs a specific task or set of tasks.
Functions help to organize code, make it more readable, and avoid redundancy. They can accept arguments and return values, providing a way to pass data in and out of the function.
They enable you to group related commands together, making it easier to manage and maintain your shell scripts.
Functions can be a powerful tool in shell scripting, allowing you to modularize your code and make it more maintainable. They can be called from other functions or directly from the main script, just like any other command.
In most shell scripting languages like Bash, you can define a function using the following syntax:
#Syntax
function_name() {
# function body or commands and statements here
}
- Example 1:
#Example
#!/bin/sh
# Defining function
first_call () {
echo "This is the first function speaking..."
}
second_call () {
echo "This is now the second function speaking..."
}
# Calling function
first_call
second_call
- Example 2
#Example to find the average of three numbers
#!/bin/sh
find_avg(){
echo "Enter the three numbers :"
read n1
read n2
read n3
sum=$(( $n1 + $n2 + $n3 ))
average=$(( sum / 3 ))
echo "Average of three numbers is ${average}"
}
find_avg
Cases
In a shell script, the "case" statement is used for conditional branching based on the value of a variable or an expression.
It provides a way to execute different blocks of code based on the pattern match of the given variable or expression.
The "case" statement is an alternative to using multiple "if-elif-else" statements and can make the code more readable when you have many possible conditions.
The syntax of the "case" statement in shell scripts is as follows:
#Syntax
#!/bin/bash
variable=value
case "$variable" in
pattern1)
# Code to be executed
;;
pattern2)
# Code to be executed
;;
pattern3)
# Code to be executed
;;
*)
# Code to be executed if none of the patterns above match
;;
esac
- Example 1
#!/bin/bash
echo "Enter the score"
read score
case ${score} in
100)
echo "You are on bronze"
;;
500)
echo "You are on Silver"
;;
1000)
echo "You are on Gold"
;;
1500)
echo "You are on Platinum"
;;
2000)
echo "You are on Diammond"
;;
*)
echo "You are on higher rank or you are not a valid player"
;;
esac
- Example 2
#!/bin/bash
first_func () {
echo "This is first function "
}
second_func () {
echo "This is second function "
}
third_func () {
echo "This is third function "
}
fourth_func () {
echo "This is fourth function "
}
echo "1. To call Function 1"
echo "2. To call Function 2"
echo "3. To call Function 3"
echo "4. To call Function 4"
read fun
case ${fun} in
1)
first_func
;;
2)
second_func
;;
3)
third_func
;;
4)
fourth_func
;;
*)
echo "Not a valid function.."
;;
esac
That's it for day 9 (last day)...
Hope you have Enjoyed the blog, Thank You for reading.
Keep Supporting, Learning and growing with each other
Subscribe to my newsletter
Read articles from SARTHAK SHINDE directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

SARTHAK SHINDE
SARTHAK SHINDE
Hey guys, I am Sarthak Shinde . Currently studying Diploma in Computer Engineering at Dr. Babasaheb Ambedkar Technological University, Lonere, Raigad.