Real-Life Applications of Stack Data Structure
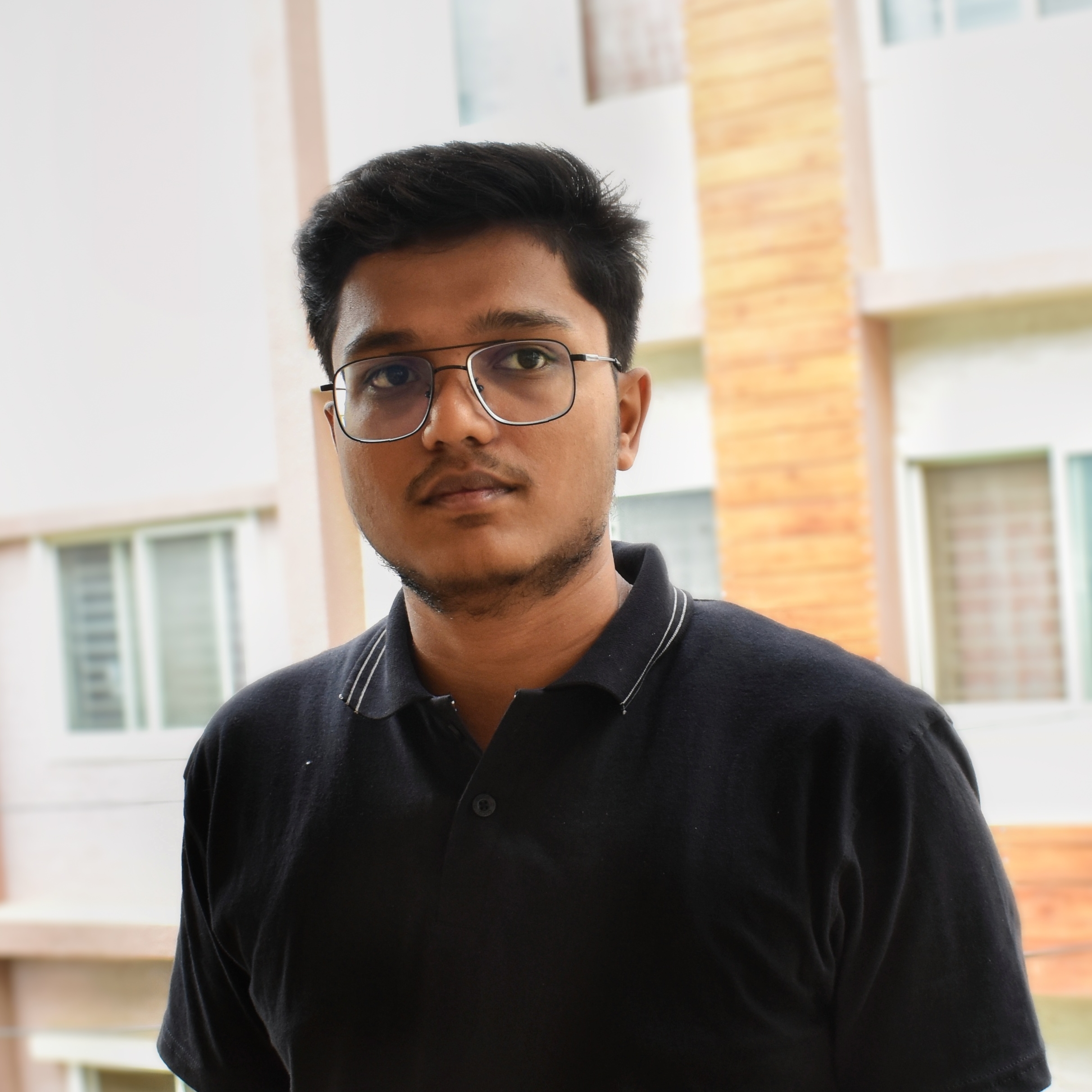
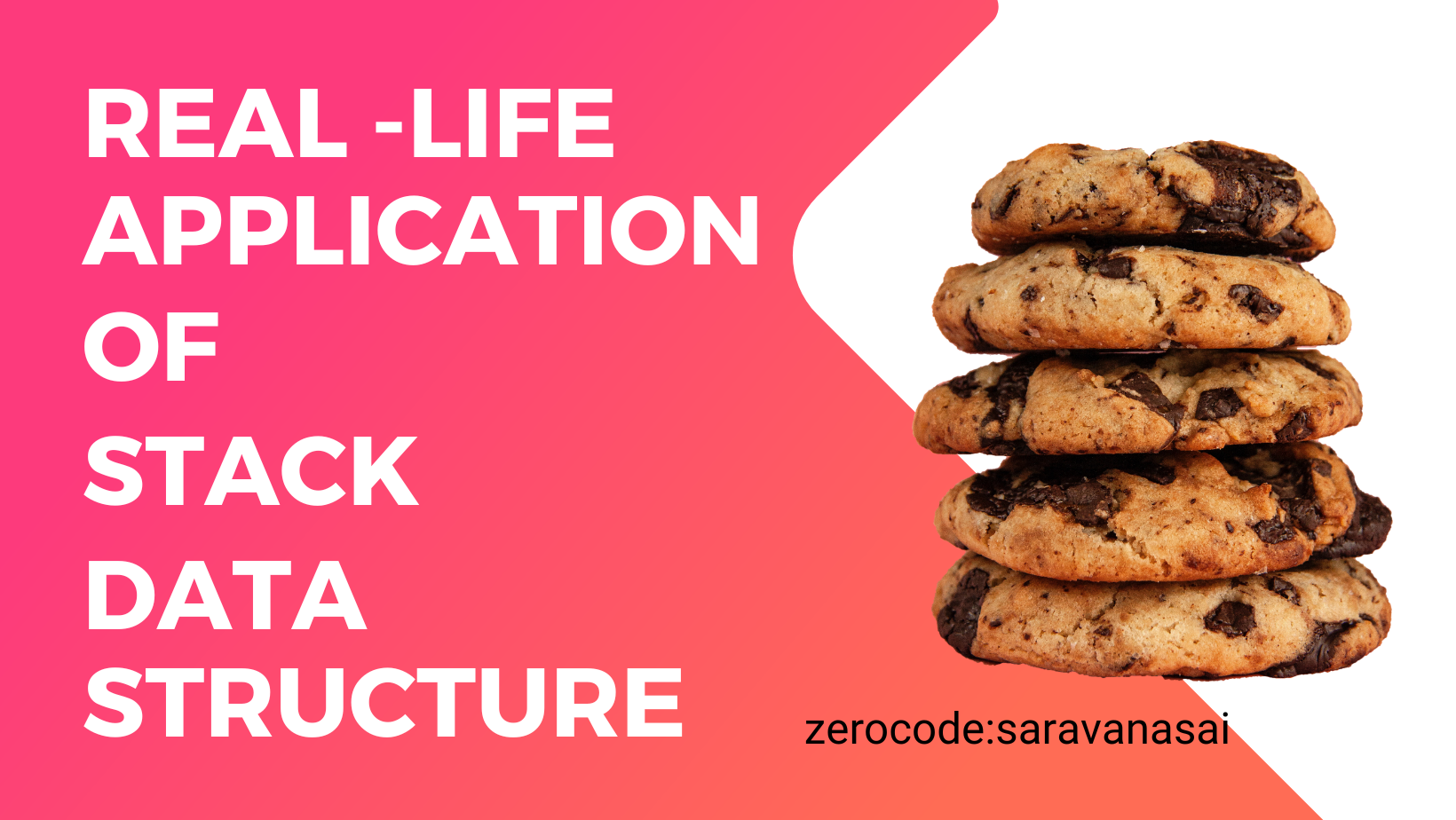
Introduction
Hi, Folks. In this article blog, we will explore the Stack data structure concept, and its underlying mechanisms, and understand how it works. Moreover, we will dive into some practical examples to illustrate its applications in everyday coding & interview scenarios.
Problem to solve
Let me share my experience while attending a front-end interview for a reputed company as React developer. After a warm welcome & intro section. The interviewer threw me a task to build a small text editor where users can type & save the text. The important feature of the editor is to be able to undo & revert back to the old state.
The interviewer shows a demo of the final application should be like a gif below.
For the first time, I have seen this. I am not getting an idea. The interviewer also stated don't focus on the design try to implement the functionality part of it. The interviewer shared with me a stack blitz online editor with a basic react project starter.
Intuition & Thoughts
I have started thinking about key features. Then start to write a sudo code on the stack blitz editor.
// Need a piece of state to track a text editor
// Then a handler function to handle the changes on text editor
// Then need a state to save a previous state of text editor
After writing this sudo code. I have started to write a basic component structure for the editor & done with my first 2 sudo code points. After that I reached up to now I can track text editor text changes.
const SimpleCodeEditor = () => {
const [isSaved, setIsSaved] = React.useState(true);
const [editorText, setEditorText] = React.useState('');
const textArea = React.useRef(null);
const handleSave = () => {
setEditorText(textArea.current.value)
setIsSaved(true);
};
return (
<div className="card">
<div className="card-body">
<h5 className="card-title">Code Editor</h5>
<h6 className="card-subtitle mb-2 text-muted">
{isSaved ? 'File Saved' : 'File UnSaved'}
</h6>
<p className="card-text">
<div className="form-floating">
<textarea
ref={textArea}
onFocus={() => setIsSaved(false)}
onBlur={() =>
textArea.current.value != editorText
? setIsSaved(false)
: setIsSaved(true)
}
className="form-control"
placeholder="Write text here"
id="floatingTextarea2"
style={{ height: 200 }}
></textarea>
</div>
</p>
</div>
<div className="card-footer ">
<div className="btn-group" role="group" aria-label="Basic example">
<button type="button" className="btn btn-danger">
Undo
</button>
<button
type="button"
className="btn btn-success"
onClick={handleSave}
>
Save
</button>
</div>
</div>
</div>
);
};
After that, I got an idea. We need to keep the history of previously saved text to get that previous value back on undo operation.
Stack Data Structure Kicks In
I have learned to solve some leet code problems on stacks of the classic valid parenthesis. I got an idea to use because I applies the functionality that I need. In a stack, we can push the current value. Later we can pop to go back previous value.
The idea behind this is to push the new text to stack on save & to pop out on undo operation.
Stack
The key idea behind using a stack is that it allows for easy access and removal of the most recently added element, which can be useful in situations when we need to keep track of a history of actions or reverse actions.
A Stack is a linear data structure that follows the LIFO (Last-In-First-Out) principle. Stack has one end, whereas the Queue has two ends (front and rear).
The element that was inserted last will be removed first.
Solution
So, I have used a stack to implement the text editor to build the desired functionality.
For live solutions check the stack blitz Code Editor.
import * as React from 'react';
import './style.css';
import 'bootstrap/dist/css/bootstrap.css';
const SimpleCodeEditor = () => {
const [isSaved, setIsSaved] = React.useState(true);
const [stack, setStack] = React.useState([]);
const textArea = React.useRef(null);
const handleSave = () => {
setStack((prev) => {
return [...prev, textArea.current.value];
});
setIsSaved(true);
};
const handleUndo = () => {
setStack((prev) => {
return prev.filter((_, index) => index != stack.length - 1);
});
};
React.useEffect(() => {
textArea.current.value = stack[stack.length - 1] ?? '';
}, [stack]);
return (
<div className="card">
<div className="card-body">
<h5 className="card-title">Code Editor</h5>
<h6 className="card-subtitle mb-2 text-muted">
{isSaved ? 'File Saved' : 'File UnSaved'}
</h6>
<p className="card-text">
<div className="form-floating">
<textarea
ref={textArea}
onFocus={() => setIsSaved(false)}
onBlur={() =>
textArea.current.value != stack[stack.length - 1]
? setIsSaved(false)
: setIsSaved(true)
}
className="form-control"
placeholder="Leave a comment here"
id="floatingTextarea2"
style={{ height: 200 }}
></textarea>
</div>
</p>
</div>
<div className="card-footer ">
<div className="btn-group" role="group" aria-label="Basic example">
<button type="button" className="btn btn-danger" onClick={handleUndo}>
Undo
</button>
<button
type="button"
className="btn btn-success"
onClick={handleSave}
>
Save
</button>
</div>
</div>
</div>
);
};
export default function App() {
return (
<div className="row mt-5">
<div className="col-sm-12 col-md-8 offset-md-2">
<div className="container">
<SimpleCodeEditor />
</div>
</div>
</div>
);
}
Application of Stack
Back and forward buttons in a web browser
UNDO/REDO functionality in text editors and image editing software
Delimiter checking
Expression conversion and evaluation
Matching HTML tags in web development
Conclusion
Thank you for reading the article and understanding the stack data structure's real-world usage. Happy coding!
Leave your comments & share them with your friends for more content like this.
Subscribe to my newsletter
Read articles from Saravana Sai directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
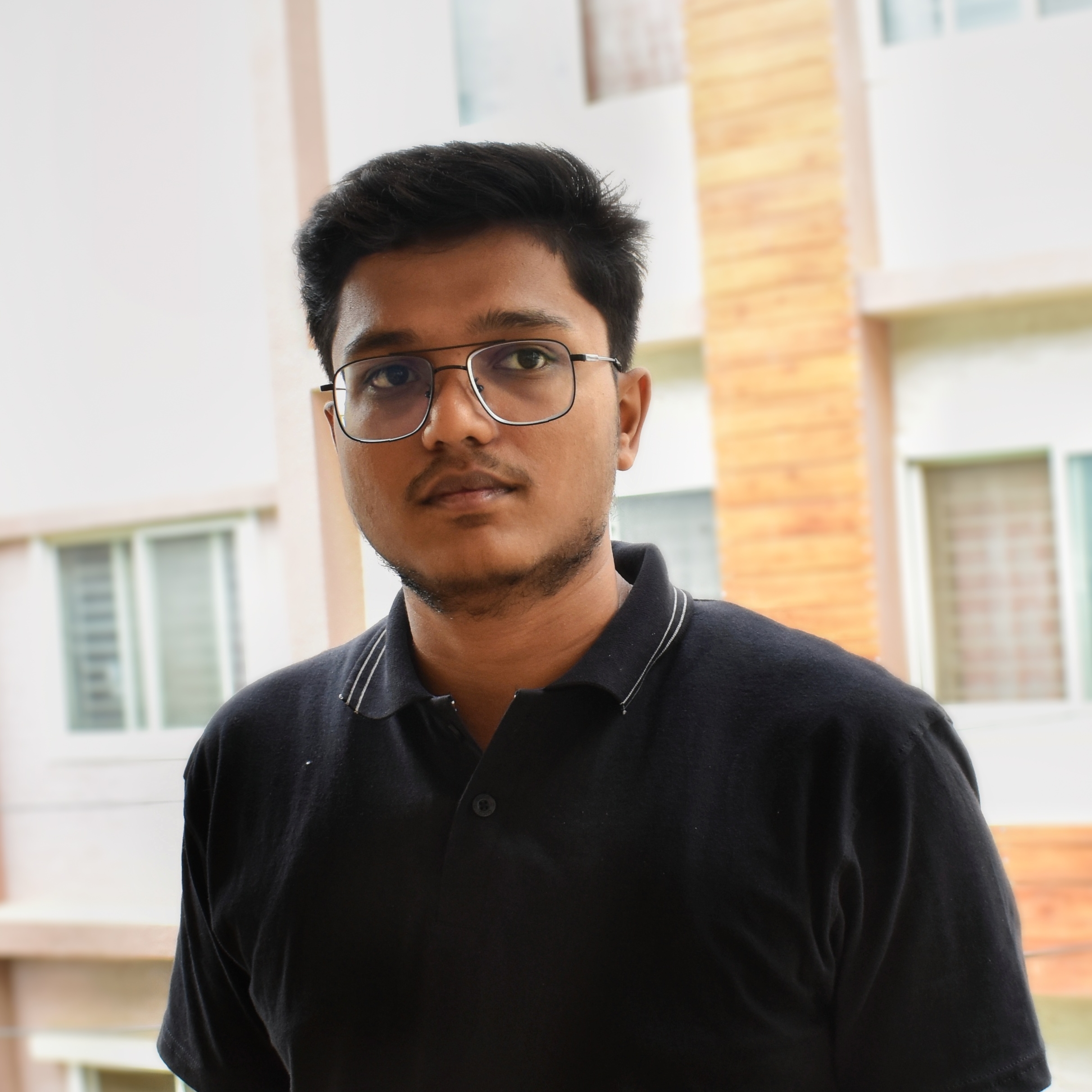
Saravana Sai
Saravana Sai
I am a self-taught web developer interested in building something that makes people's life awesome. Writing code for humans not for dump machine