#day14-Python Data Types and Data Structures for DevOps🐍.

Table of contents
- 🗼Datatypes in Python:
- 💻Data Structures in Python
- 🪐Choosing the Right Data Type and Data Structure
- 🧩Give the Difference between List, Tuple and set with a hands-on example.
- 🙌Hands-on Examples
- 👨🔧Create a dictionary and use Dictionary methods to print your favorite tool just by using the keys of the dictionary.
- 🍂Create a List of cloud service providers.
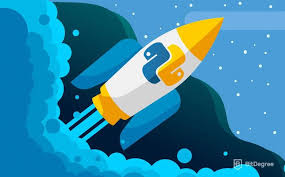
🗼Datatypes in Python:
Data types define the kind of data that a variable can hold. Python is a dynamically typed language, which means you don't need to explicitly specify the data type of a variable. Instead, Python infers the data type based on the value assigned to the variable. Here are some commonly used data types in Python:
Numeric Types:
int: Integer values, ex., 5, -10, 1000.
float: Floating-point values with decimal points, ex., 3.14, -0.5, 2.0.
complex: Complex numbers with real and imaginary parts, ex., 3 + 4j.
Boolean Type:
- bool: Represents a Boolean value, either True or False.
Sequence Types:
str: Represents a string of characters, ex., "Hello, World!", 'Python'.
list: Ordered collection of items, mutable, ex., [1, 2, 3], ['apple', 'banana', 'cherry'].
tuple: Ordered collection of items, immutable, ex., (1, 2, 3), ('red', 'green', 'blue').
range: Represents an immutable sequence of numbers, commonly used in loops, ex., range(5).
Set Types:
set: Unordered collection of unique items, mutable, ex., {1, 2, 3}, {'apple', 'banana', 'cherry'}.
frozenset: Similar to sets but immutable.
Mapping Type:
- dict: Collection of key-value pairs, mutable, ex., {'name': 'John', 'age': 30}.
None Type:
- None: Represents the absence of a value or a null value.
💻Data Structures in Python
Data structures are containers that allow you to organize and store data in different ways, depending on your needs. Python provides several built-in data structures:
List: Lists are ordered collections that can hold elements of different data types. They are mutable, meaning you can add, remove, or modify elements. Example:
numbers = [1, 2, 3, 4, 5]
Tuple: Tuples are similar to lists, but they are immutable, meaning their elements cannot be changed after creation.
Example:
coordinates = (10, 20)
Set: Sets are unordered collections of unique elements. They do not allow duplicates.
Example:
fruits = {'apple', 'banana', 'cherry'}
Dictionary:
Dictionaries are collections of key-value pairs. Each key in a dictionary maps to a corresponding value.
Example:
person = {'name': 'John', 'age': 30, 'city': 'New York'}
Strings: Though not a separate data structure, strings are sequences of characters represented using single or double quotes.
Example:
message = "Hello, Python!"
🪐Choosing the Right Data Type and Data Structure
Selecting the appropriate data type and data structure is crucial for efficient and effective programming. Consider the following when making your choice:
Use integers for whole numbers, floats for decimal numbers, and complex numbers for mathematical calculations involving imaginary components.
Strings are suitable for text manipulation and handling textual data.
Lists are useful when you need an ordered collection of elements, while sets are preferable when uniqueness is important.
Tuples are beneficial when you need an immutable sequence, and dictionaries are great for key-value pair representations.
🧩Give the Difference between List, Tuple and set with a hands-on example.
Lists:
Lists are ordered collections of elements.
Elements in a list are mutable, meaning they can be added, removed, or modified.
Lists are defined using square brackets
[ ]
.
Tuples:
Tuples are similar to lists, but they are immutable, meaning their elements cannot be changed after creation.
Tuples are defined using parentheses
( )
.
Sets:
Sets are unordered collections of unique elements.
Sets do not allow duplicate elements, and they are mutable, allowing elements to be added or removed.
Sets are defined using curly braces
{ }
or theset()
function.
🙌Hands-on Examples
Lists:
# Create a list of numbers
numbers_list = [1, 2, 3, 4, 5]
# Add an element to the list
numbers_list.append(6)
# Modify an element in the list
numbers_list[0] = 10
# Remove an element from the list
numbers_list.remove(3)
# Display the list
print(numbers_list)
Tuples:
# Create a tuple of coordinates
coordinates_tuple = (10, 20)
# Attempt to modify an element in the tuple (will raise an error)
# coordinates_tuple[0] = 5
# Display the tuple
print(coordinates_tuple)
Sets:
# Create a set of fruits
fruits_set = {'apple', 'banana', 'cherry'}
# Add an element to the set
fruits_set.add('orange')
# Remove an element from the set
fruits_set.remove('banana')
# Attempt to add a duplicate element (will not be added)
fruits_set.add('cherry')
# Display the set
print(fruits_set)
👨🔧Create a dictionary and use Dictionary methods to print your favorite tool just by using the keys of the dictionary.
# Create the fav_tools dictionary
fav_tools = {
1: "Linux",
2: "Git",
3: "Docker",
4: "Kubernetes",
5: "Terraform",
6: "Ansible",
7: "Chef"
}
# Use the keys to print my favorite tool
my_favorite_tool_key = 4
if my_favorite_tool_key in fav_tools:
my_favorite_tool = fav_tools[my_favorite_tool_key]
print("My favorite tool is:", my_favorite_tool)
else:
print("Key not found in the dictionary.")
In this example, I've used the key 4
to access the value "Kubernetes"
from the fav_tools
dictionary and printed it as my favorite tool. You can replace my_favorite_tool_key
with any other key from the dictionary to get the corresponding tool.
🍂Create a List of cloud service providers.
# Create the cloud_providers list
cloud_providers = ["AWS", "GCP", "Azure"]
# Display the list
print(cloud_providers)
This code will create a list called cloud_providers
containing three cloud service providers: "AWS" (Amazon Web Services), "GCP" (Google Cloud Platform), and "Azure" (Microsoft Azure). The print()
function will display the contents of the list as follows:
['AWS', 'GCP', 'Azure']
Subscribe to my newsletter
Read articles from Sneha Falmari directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
