How To Center a Div
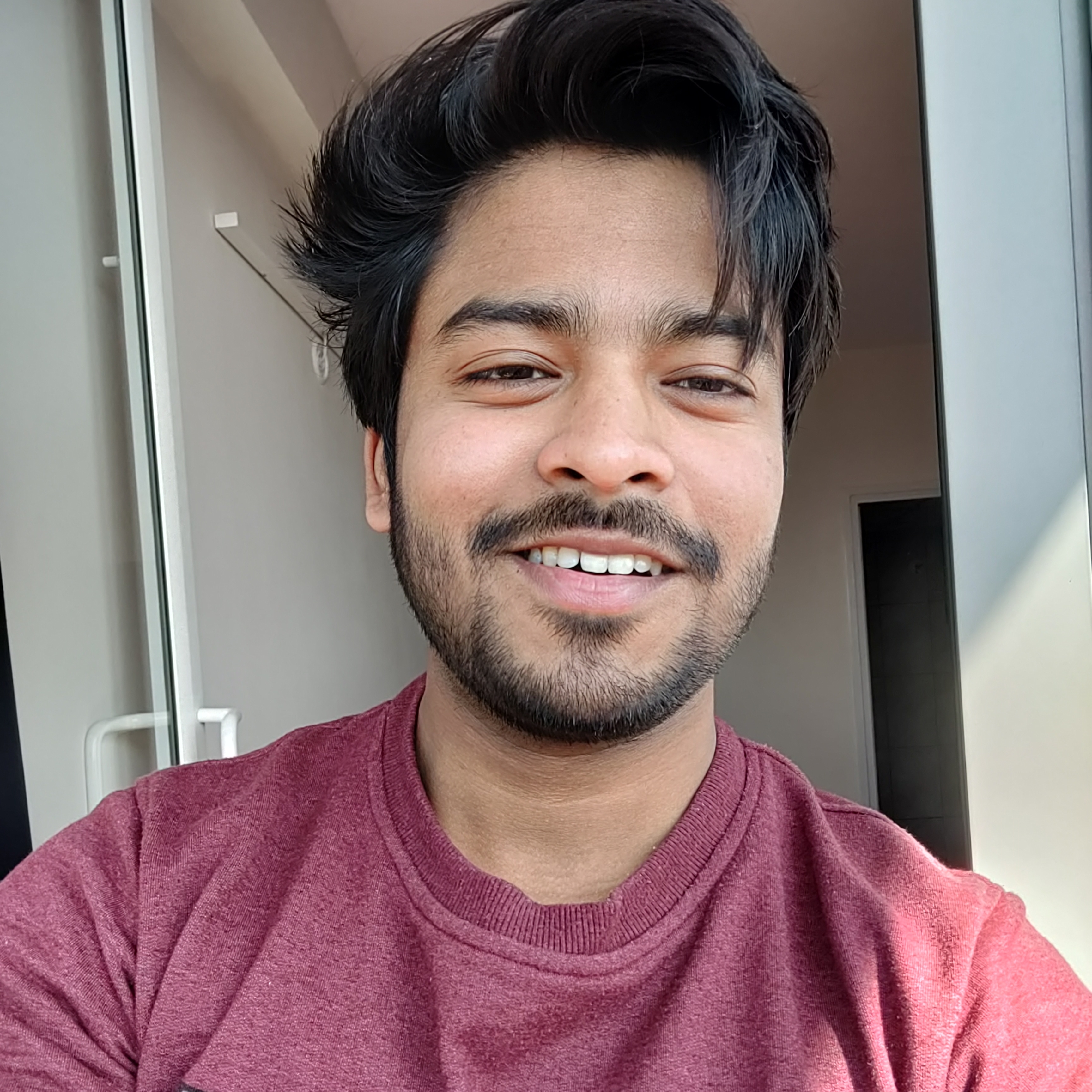
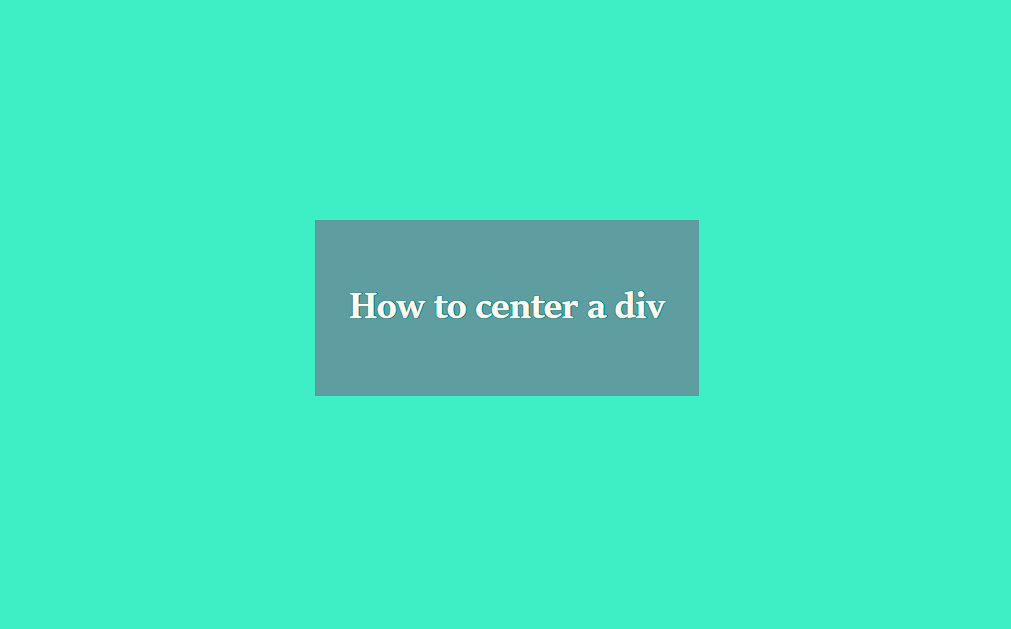
Introduction:
When building web pages, one common challenge developers face is centering a div element both horizontally and vertically on the screen. In this blog post, we'll explore two popular CSS techniques - Flexbox and Grid - to achieve this task. Centering a div is essential for creating aesthetically pleasing and responsive layouts, and we'll learn how to do it effectively using these powerful CSS features.
Method 1: Centering with CSS Flexbox
Flexbox is a CSS layout model that provides a flexible way to distribute and align items within a container. To center a div both horizontally and vertically using Flexbox, we'll follow these steps:
Step 1: Create the HTML structure with a wrapper div and the content div that needs to be centered.
htmlCopy code<!DOCTYPE html>
<html>
<head>
<title>Center Div using Flexbox</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="center-wrapper">
<div class="content">
<!-- Your content goes here -->
</div>
</div>
</body>
</html>
Step 2: Add CSS styles to the stylesheet (styles.css) to center the div using Flexbox.
cssCopy codebody {
margin: 0;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
.center-wrapper {
display: flex;
justify-content: center;
align-items: center;
}
.content {
/* Add styling for your content div */
}
Method 2: Centering with CSS Grid
CSS Grid is another powerful layout model that allows you to create complex grid-based layouts with ease. To center a div using CSS Grid, follow these steps:
Step 1: Create the HTML structure with a wrapper div and the content div.
htmlCopy code<!DOCTYPE html>
<html>
<head>
<title>Center Div using CSS Grid</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="center-wrapper">
<div class="content">
<!-- Your content goes here -->
</div>
</div>
</body>
</html>
Step 2: Add CSS styles to the stylesheet (styles.css) to center the div using CSS Grid.
cssCopy codebody {
margin: 0;
display: grid;
place-items: center;
height: 100vh;
}
.center-wrapper {
display: grid;
place-items: center;
}
.content {
/* Add styling for your content div */
}
Conclusion:
Centering a div both horizontally and vertically is a common requirement in web development. By using CSS Flexbox or CSS Grid, you can easily achieve this layout task without the need for complex calculations or JavaScript. Both techniques offer powerful and flexible ways to create responsive and visually appealing designs. Depending on your specific project needs and layout requirements, you can choose between Flexbox and Grid to center your div elements and create stunning web layouts.
Subscribe to my newsletter
Read articles from Keshav Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
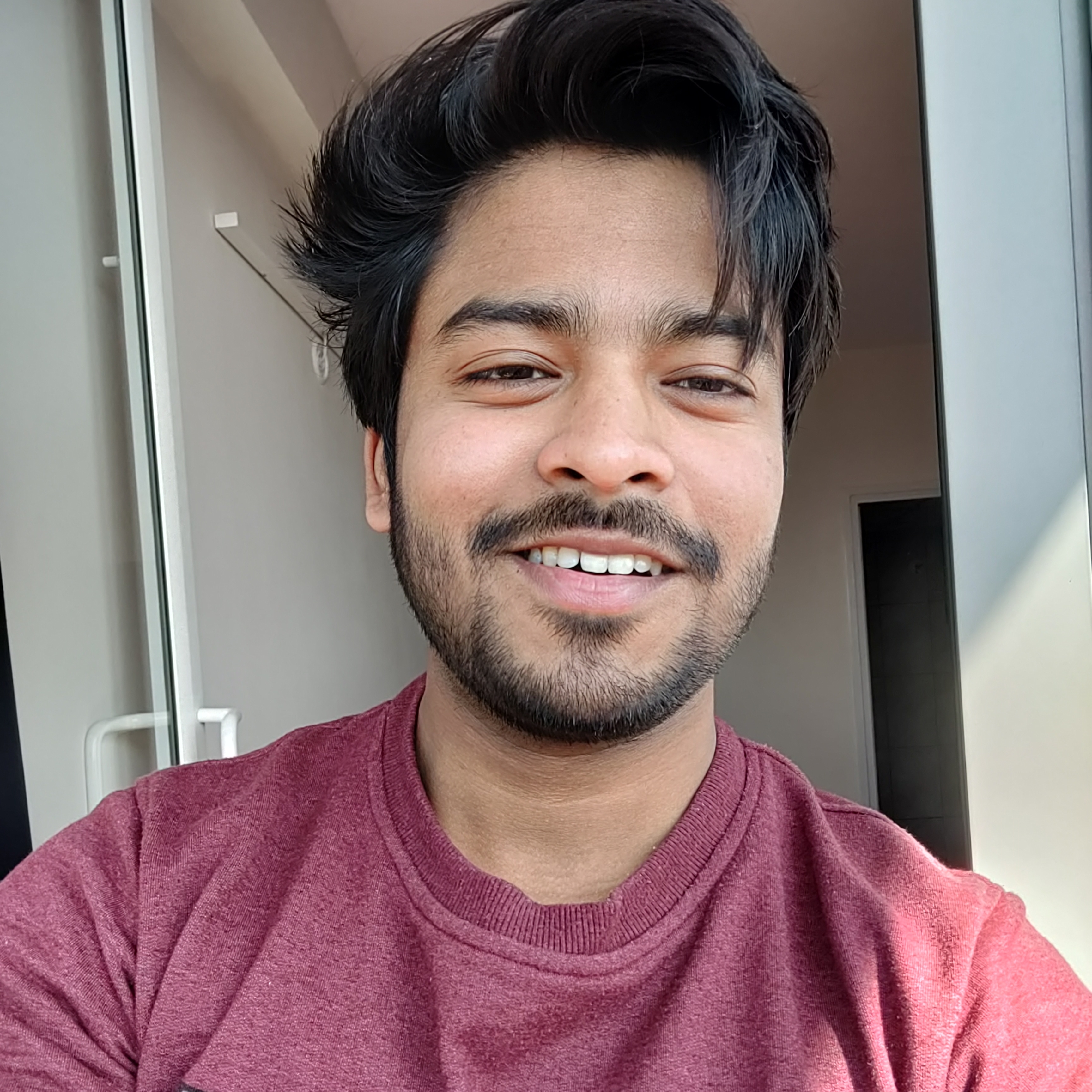
Keshav Kumar
Keshav Kumar
Tech Writer | Open Source