How to implement the Luhn Algorithm in JavaScript!

2 min read
Table of contents
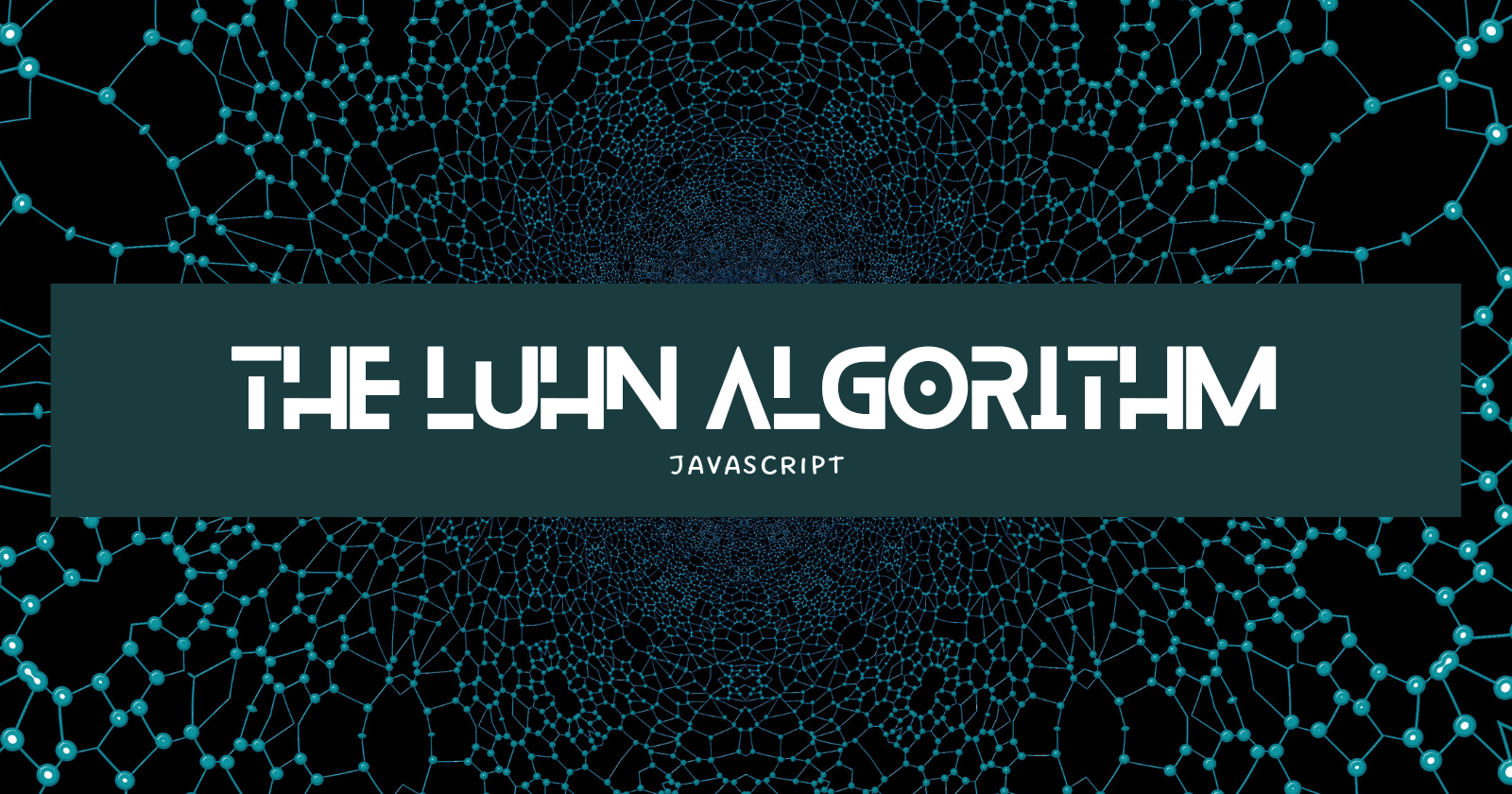
The Luhn Algorithm is a formula that verifies the accuracy of identification numbers. Some of its applications include credit cards, social security numbers, and more.
The formula
The Luhn Algorithm follows the following formula:
1. Starting from the right, multiply every second digit by two.
2. If the multiplication is more than nine, sum up both digits to form one.
3. It then sums up all the digits
4. If the division equals zero, then the card number is correct. Otherwise, it is wrong.
The code
//Create a function whose parameter is an array
function validateCred(cardNum) {
let sum = 0;
for (let i = cardNum.length - 1; i >= 0; i--) {
let digit = cardNum[i];
if ((cardNum.length - i) % 2 === 0) {
digit *= 2;
//if digit is greater than 9, deduct 9 from digit. This is an easier way to implement step 2 in the formula
if (digit > 9) {
digit -= 9;
}
}
sum += digit;
}
return sum % 10 === 0;
}
Thank you for reading, I hope this helped.
💡
Community is the best way to grow. That is why I am looking for other developers who are willing to do coding marathons with me. If grinding projects together seem like a good pathway to improve your skills, please shoot me a DM on Twitter @Armstrong2035.
I'll be seeing you.
0
Subscribe to my newsletter
Read articles from Armstrong Olusoji directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
