Build and Deploy a GraphQL API to the Edge with Fauna โ Part 4
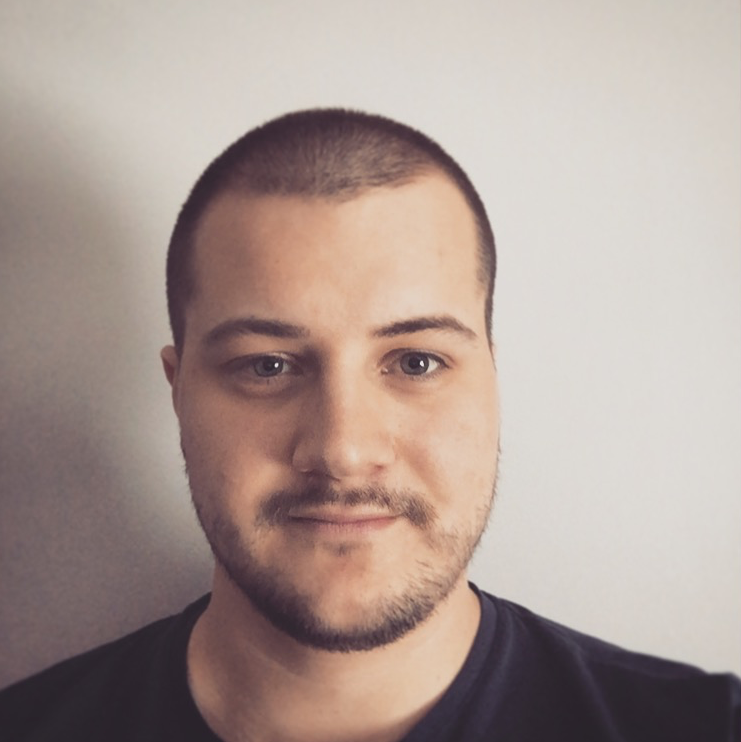
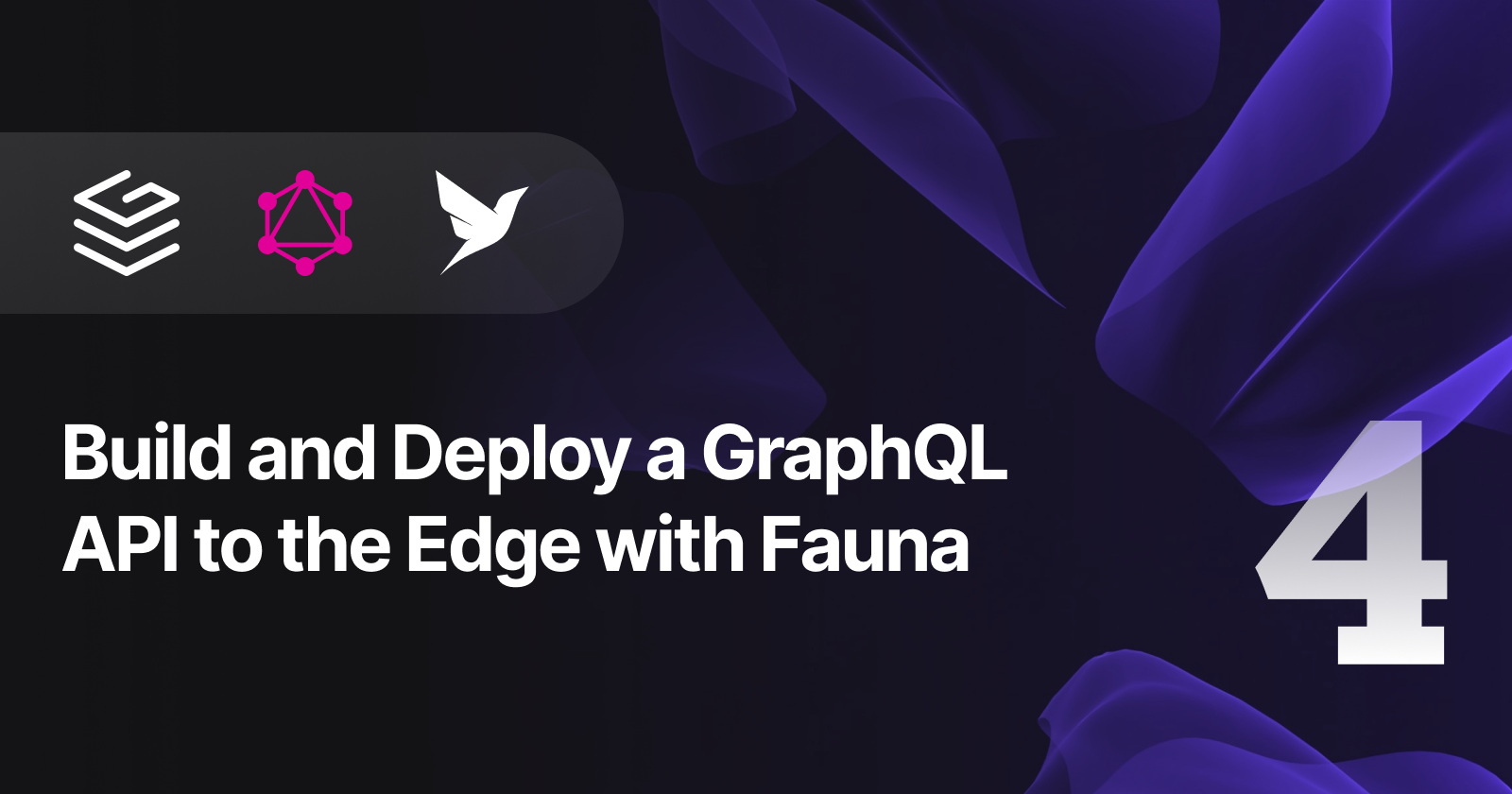
We're now ready to create a GraphQL mutation that we will use to add to our products collection inside the Fauna database.
A GraphQL mutation is a type of operation in GraphQL that modifies data on the server and typically look something like this:
mutation {
doSomething(a: String, b: Int) {
someField
}
}
doSomething
is the name of the mutationa
andb
are names of the arguments passed to mutationsString
andInt
are the data types of the argumentssomeField
is the name of a field returned by the mutation
If you selected TypeScript as the configuration type when using grafbase init
you will have the file grafbase/grafbase.config.ts
. Inside here we will add the type for Product
with the following fields:
id
name
price
import { config, g } from '@grafbase/sdk'
const product = g.type('Product', {
id: g.id(),
name: g.string(),
price: g.int()
})
export default config({
schema: g
})
Now we have the Product type created, we can now use that for the createProduct
mutation. We'll need to create a input
type used by the mutation and configure the mutation itself, which links the resolver file create.ts
:
const productCreateInput = g.input('ProductCreateInput', {
name: g.string(),
price: g.int()
})
g.mutation('productCreate', {
args: { input: g.inputRef(productCreateInput) },
resolver: 'products/create',
returns: g.ref(product).optional()
})
Now let's create the code that runs when the GraphQL mutation productCreate
is executed. This code is known as a GraphQL resolver.
In the previous step, we created the file grafbase/resolvers/create.ts
. This is the file we will use to export a default async
function that invokes a Fauna FQL statement to insert into the database.
Let's update the file create.ts
to contain a new default export:
import { Client, fql, FaunaError } from "fauna";
const client = new Client();
export default async function ProductsCreate(_, { input }) {
// ...
}
Since Fauna is a document based database, we don't need to give it a structure upfront like you would with MySQL or Postgres.
Since we have the collection products
we can invoke products.create()
and pass it the input
type and return the id
field (managed by Fauna) and the name
/price
fields:
export default async function ProductsCreate(_, { input }) {
try {
const documentQuery = fql`
products.create(${input}) {
id,
name,
price
}
`
const { data } = await client.query(documentQuery)
return data
} catch (error) {
if (error instanceof FaunaError) {
console.log(error)
}
return null
}
}
That's all we need to successfully create a product inside the Fauna products collection using a GraphQL mutation. There's minimal error handling, you'll want to add to that.
Now run the Grafbase CLI:
npx grafbase dev
Next open Pathfinder at http://127.0.0.1:4000
and execute the following mutation:
mutation {
productCreate(
input: { name: "Shoes", price: 1000 }
) {
id
name
price
}
}
You should get a response back that looks something like this:
{
"data": {
"productCreate": {
"id": "372390645805875406",
"name": "Shoes",
"price": 1000
}
}
}
That's it! You can repeat this mutation as many times as you like with unique content!
Subscribe to my newsletter
Read articles from Jamie Barton directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
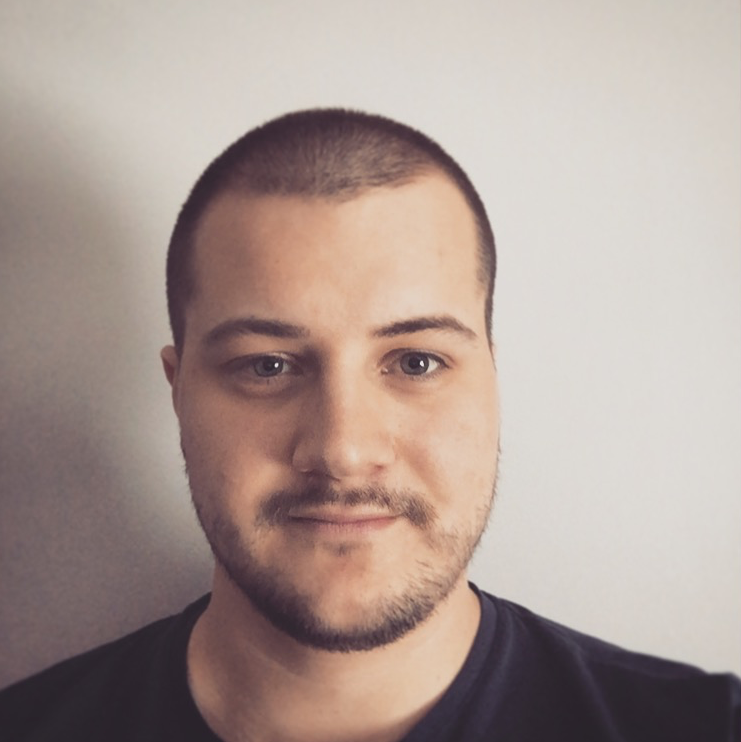