Build and Deploy a GraphQL API to the Edge with Fauna โ Part 6
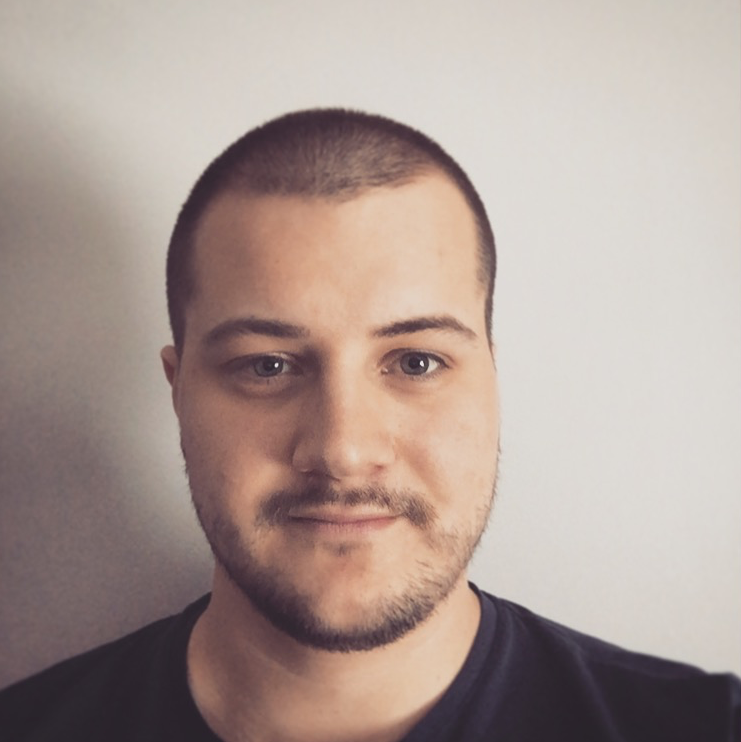
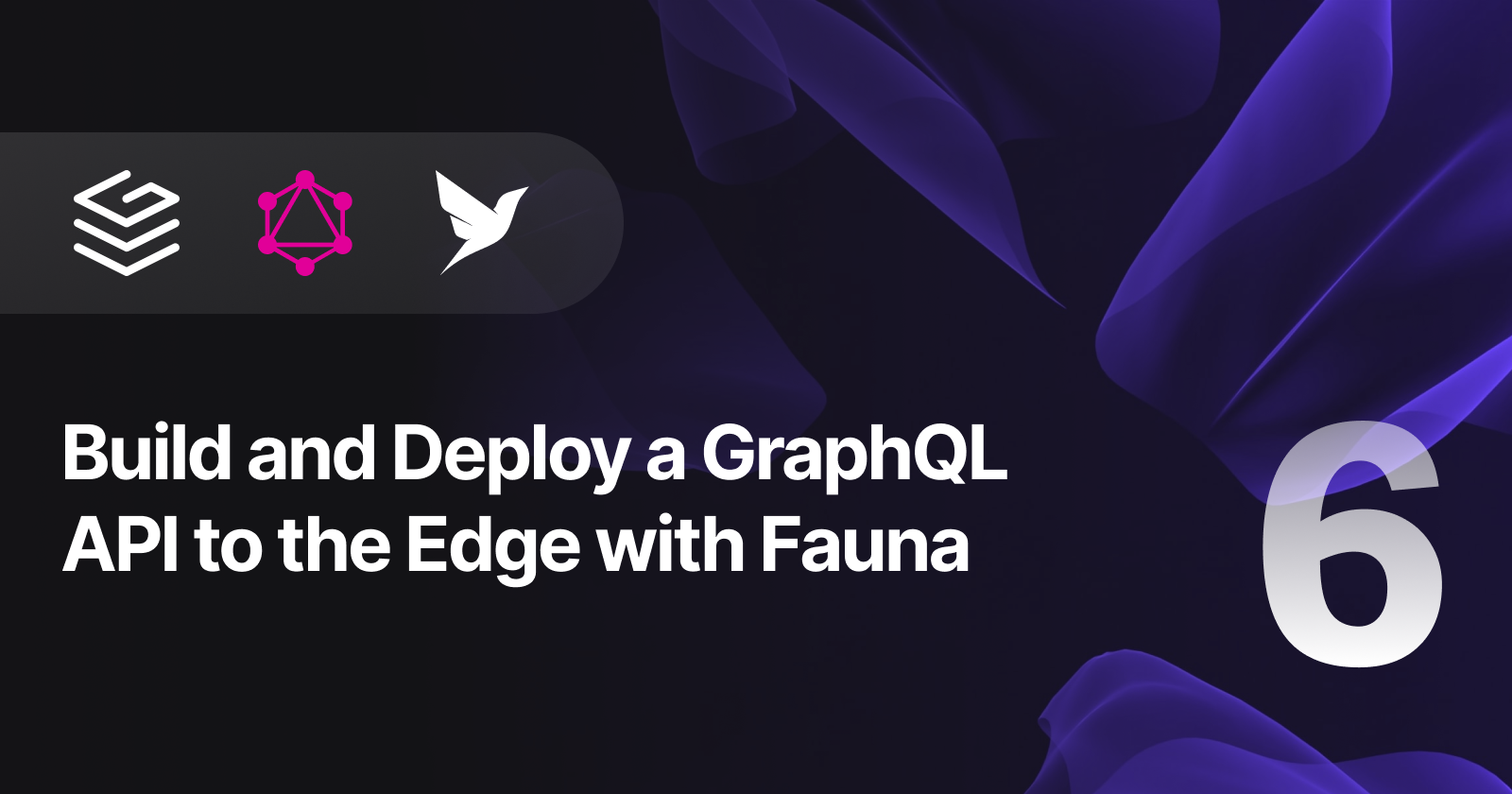
In this step, we'll create a GraphQL mutation to delete products from the Fauna collection. To do this, update the grafbase/grafbase.config.ts
file to include the following:
const productDeletePayload = g.type('ProductDeletePayload', {
deleted: g.boolean()
})
g.mutation('productDelete', {
args: {
by: g.inputRef(productByInput)
},
resolver: 'products/delete',
returns: g.ref(productDeletePayload).optional()
})
We don't need to add anything else to the configuration since we're reusing productByInput
.
Now create the resolver file grafbase/resolvers/products/delete.ts
and add the following:
import { Client, fql, FaunaError } from 'fauna'
const client = new Client()
export default async function ProductsDelete(_, { by }) {
const { id } = by
try {
const documentQuery = fql`
products.byId(${id}).delete()
`
await client.query(documentQuery)
return { deleted: true }
} catch (error) {
if (error instanceof FaunaError) {
console.log(error)
}
return { deleted: false }
}
}
That's it! Fauna will throw an error if the document by ID does not exist. You can forward these errors on by doing something like this inside the catch
block:
if (error instanceof FaunaError) {
throw new GraphQLError(error?.message)
}
Open Pathfinder at http://127.0.0.1:4000
and execute the following GraphQL mutation:
mutation {
productDelete(by: {
id: "372397409740783822"
}) {
deleted
}
}
id
of a product that you created earlier.You should now see something like this if the request was successful:
{
"data": {
"productDelete": {
"deleted": true
}
}
}
That's it! You can now create, update and delete products inside the Fauna Database using GraphQL.
Subscribe to my newsletter
Read articles from Jamie Barton directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
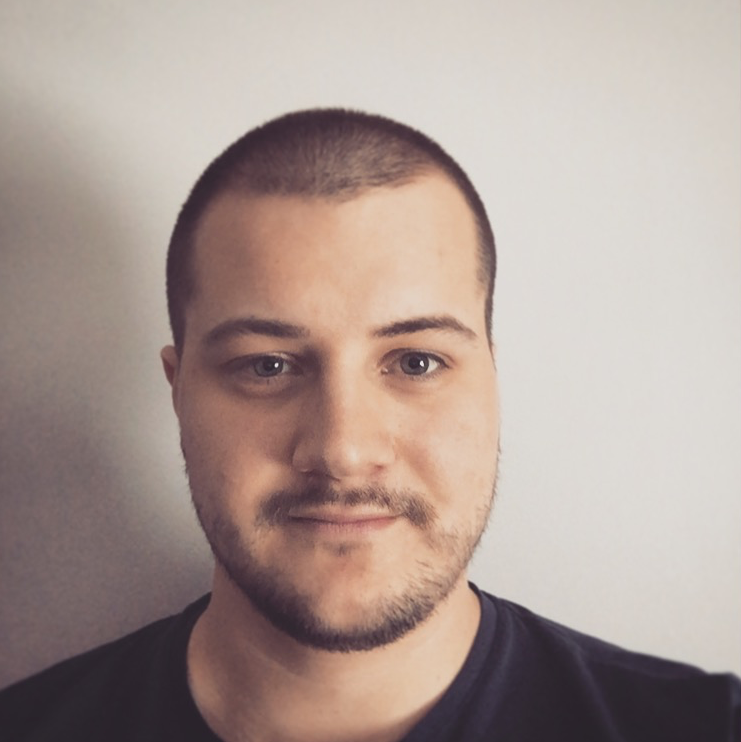