Building a Styled Header and Content Layout in HTML and CSS
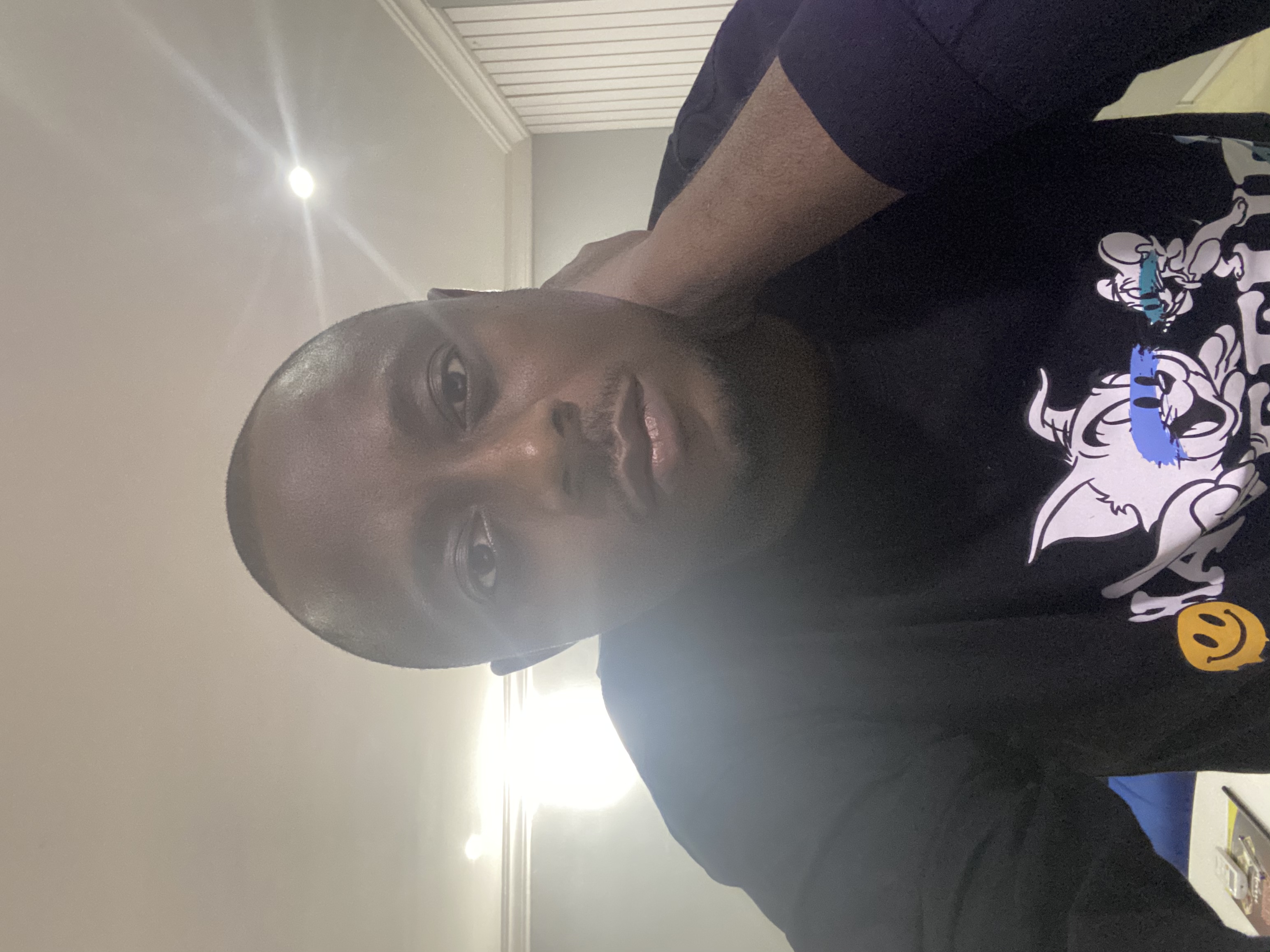
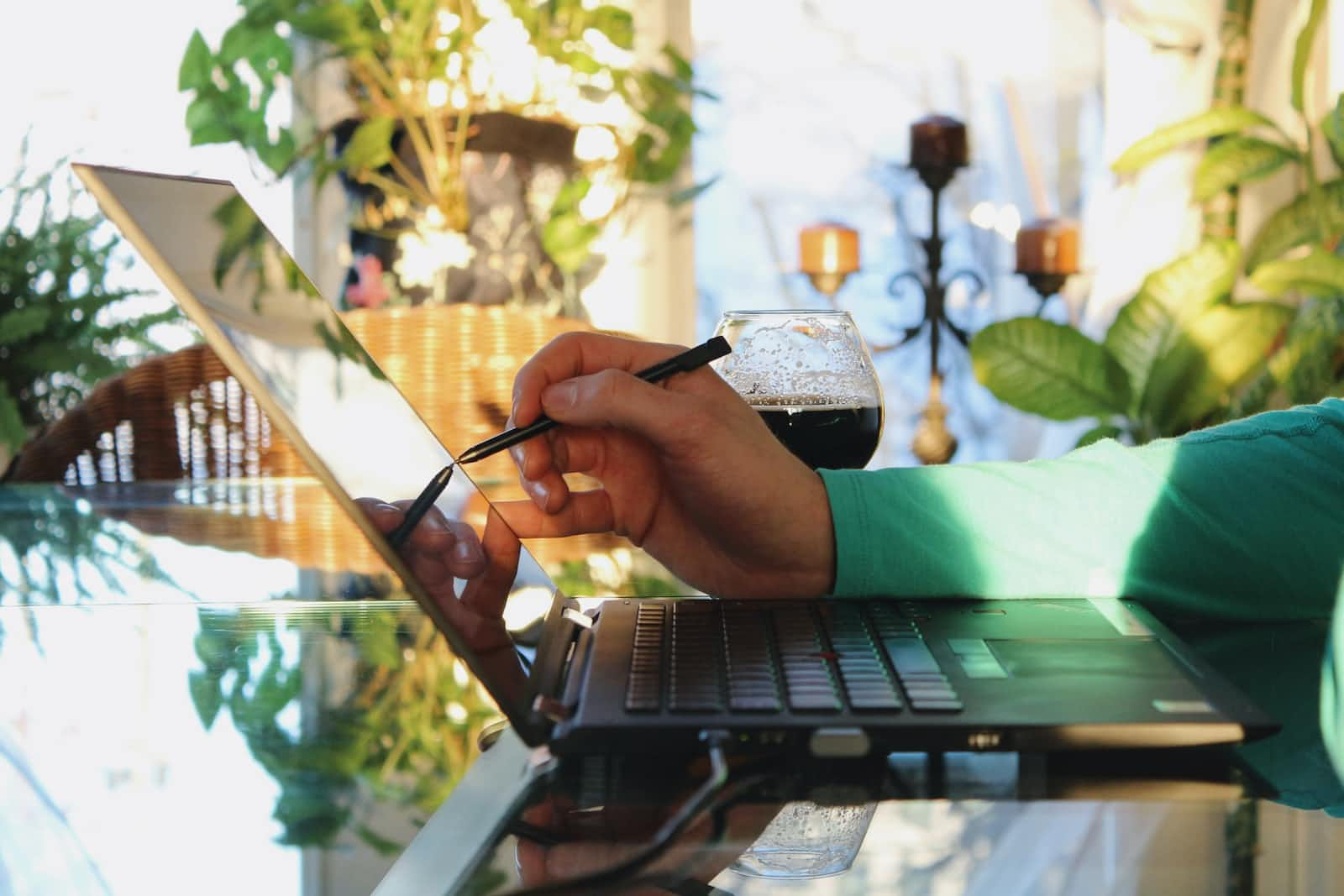
In this tutorial, we will walk through the process of creating a stylish header and content layout for a webpage using HTML and CSS. We will cover how to create a sticky header, style navigation buttons, and organize the content with a fixed footer.
Setting up the Basics
First, let's set up the basic structure of our HTML document. We'll include necessary external resources and create placeholders for our header, content, and footer.
<!DOCTYPE html>
<html>
<head>
<title>Styled Header and Content</title>
<!-- Include font and icon resources -->
<link href="https://fonts.googleapis.com/icon?family=Material+Icons" rel="stylesheet">
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/4.7.0/css/font-awesome.min.css">
<!-- Add your custom styles -->
<style>
/* Your CSS styles go here */
</style>
</head>
<body>
<!-- Header goes here -->
<header>
<!-- Navigation and logo -->
<nav>
<div class="logo">
<div>Logo</div>
</div>
<div class="rightme">
<!-- Navigation buttons -->
<div><button class="butt"><i class="fa fa-credit-card"></i></button></div>
<div><button class="butt">Login</button></div>
<div><button class="butt">Register</button></div>
</div>
</nav>
</header>
<!-- Main content -->
<div class="content">
<!-- Your content goes here -->
<!-- ... -->
</div>
<!-- Footer -->
<div class="footers">
<!-- Footer navigation -->
<div class="goat">
<!-- Footer navigation items here -->
</div>
</div>
</body>
</html>
Styling the Header and Navigation
Let's start by styling the header and navigation buttons to achieve a clean and attractive design.
/* Your CSS styles */
body {
margin: 0;
padding: 0;
font-family: Arial, sans-serif;
}
header {
background-color: #333;
color: white;
padding: 14px;
text-align: center;
position: sticky;
top: 0;
z-index: 100;
}
/* ... Other styles ... */
Adding Spacing to Navigation Buttons
To add space between the navigation buttons, we'll utilize the gap
property within the .rightme
class.
.rightme {
display: flex;
flex-direction: row;
justify-content: space-between;
gap: 15px;
}
Creating Stylish Buttons
We'll also style the navigation buttons to make them visually appealing.
.butt {
padding: 10px;
border-radius: 5px;
/* Add more styling as needed */
}
Adding Footer Navigation
We'll create a fixed footer with navigation links.
.footers {
background-color: #333;
color: white;
position: fixed;
bottom: 0;
z-index: 100;
width: 100%;
}
.goat {
display: flex;
flex-direction: row;
justify-content: space-between;
padding: 20px;
}
.bottomflex {
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
gap: 5px;
font-size: 14px;
}
Organizing Content
Lastly, we'll style the content area and ensure it has appropriate margins to accommodate the sticky header and fixed footer.
.content {
padding: 20px;
text-align: center;
margin-top: 120px;
margin-bottom: 100px;
overflow-y: auto;
}
Complete Code
<!DOCTYPE html>
<html>
<head>
<title>Styled Header and Content</title>
<link href="https://fonts.googleapis.com/icon?family=Material+Icons" rel="stylesheet">
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/4.7.0/css/font-awesome.min.css">
<style>
body {
margin: 0;
padding: 0;
font-family: Arial, sans-serif;
}
header {
background-color: #333;
color: white;
padding: 14px;
text-align: center;
position: sticky;
top: 0;
z-index: 100;
/* Ensure header stays on top of content */
}
h1 {
margin: 0;
font-size: 36px;
}
nav {
padding: 0;
display: flex;
justify-content: space-between;
margin-top: 0px;
}
.logo{
display: flex;
justify-content: center;
align-items: center;
}
.rightme {
display: flex;
flex-direction: row;
justify-content: space-between;
gap: 15px;
}
.butt{
padding: 10px;
border-radius: 5px;
}
.texty {
border: 1px solid black;
}
.footers {
background-color: #333;
color: white;
position: fixed;
bottom: 0;
z-index: 100;
/* Ensure header stays on top of content */
width: 100%;
}
.goat {
display: flex;
flex-direction: row;
justify-content: space-between;
padding: 20px;
}
.bottomflex {
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
gap: 5px;
font-size: 14px;
}
.content {
padding: 20px;
text-align: center;
margin-top: 120px;
/* Add margin to the top of content to allow space for the sticky header */
margin-bottom: 100px;
/* Add margin to the bottom of content */
overflow-y: auto;
/* Add vertical scroll if content overflows */
}
</style>
</head>
<body>
<header>
<nav>
<div class="logo">
<div>Logo</div>
</div>
<div class="rightme">
<div><button class="butt"><i class="fa fa-credit-card"></i></button></div>
<div><button class="butt">Login </button></div>
<div><button class="butt">Register </button></div>
</div>
</nav>
</header>
<div class="content">
<!-- Your content goes here -->
<!-- ... -->
<h2>Welcome to My Website</h2>
<p>This is an example of a blank HTML document with top and bottom menus. You can customize the content of this
page as needed.</p>
<h2>Welcome to My Website</h2>
<p>This is an example of a blank HTML document with top and bottom menus. You can customize the content of this
page as needed.</p>
<h2>Welcome to My Website</h2>
<p>This is an example of a blank HTML document with top and bottom menus. You can customize the content of this
page as needed.</p>
<h2>Welcome to My Website</h2>
<p>This is an example of a blank HTML document with top and bottom menus. You can customize the content of this
page as needed.</p>
<h2>Welcome to My Website</h2>
<p>This is an example of a blank HTML document with top and bottom menus. You can customize the content of this
page as needed.</p>
<h2>Welcome to My Website</h2>
<p>This is an example of a blank HTML document with top and bottom menus. You can customize the content of this
page as needed.</p>
<h2>Welcome to My Website</h2>
<p>This is an example of a blank HTML document with top and bottom menus. You can customize the content of this
page as needed.</p>
<h2>Welcome to My Website</h2>
<p>This is an example of a blank HTML document with top and bottom menus. You can customize the content of this
page as needed.</p>
<h2>Welcome to My Website</h2>
<p>This is an example of a blank HTML document with top and bottom menus. You can customize the content of this
page as needed.</p>
<h2>Welcome to My Website</h2>
<p>This is an example of a blank HTML document with top and bottom menus. You can customize the content of this
page as needed.</p>
<h2>Welcome to My Website</h2>
<p>This is an example of a blank HTML document with top and bottom menus. You can customize the content of this
page as neededy.</p>
</div>
<div class="footers">
<div class="goat">
<div class="bottomflex">
<span><i class="fa fa-align-justify" style="font-size:16px"></i></span>
<span> Menu </span>
</div>
<div class="bottomflex">
<span><i class="fa fa-bookmark" style="font-size:16px"></i></span>
<span> MyBets </span>
</div>
<div class="bottomflex">
<span><i class="fa fa-gamepad" style="font-size:16px"></i></span>
<span> BetSlip </span>
</div>
<div class="bottomflex">
<span><i class="fa fa-lastfm-square" style="font-size:16px"></i></span>
<span> Casino </span>
</div>
<div class="bottomflex">
<span><i class="fa fa-wechat" style="font-size:16px"></i></span>
<span> Chat </span>
</div>
</div>
</div>
</body>
</html>
And that's it! By following these steps and using the provided HTML and CSS code snippets, you can create a beautifully styled header and content layout for your webpage.
Feel free to customize the styles and layout further to match your design preferences.
Subscribe to my newsletter
Read articles from Ogunuyo Ogheneruemu B directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
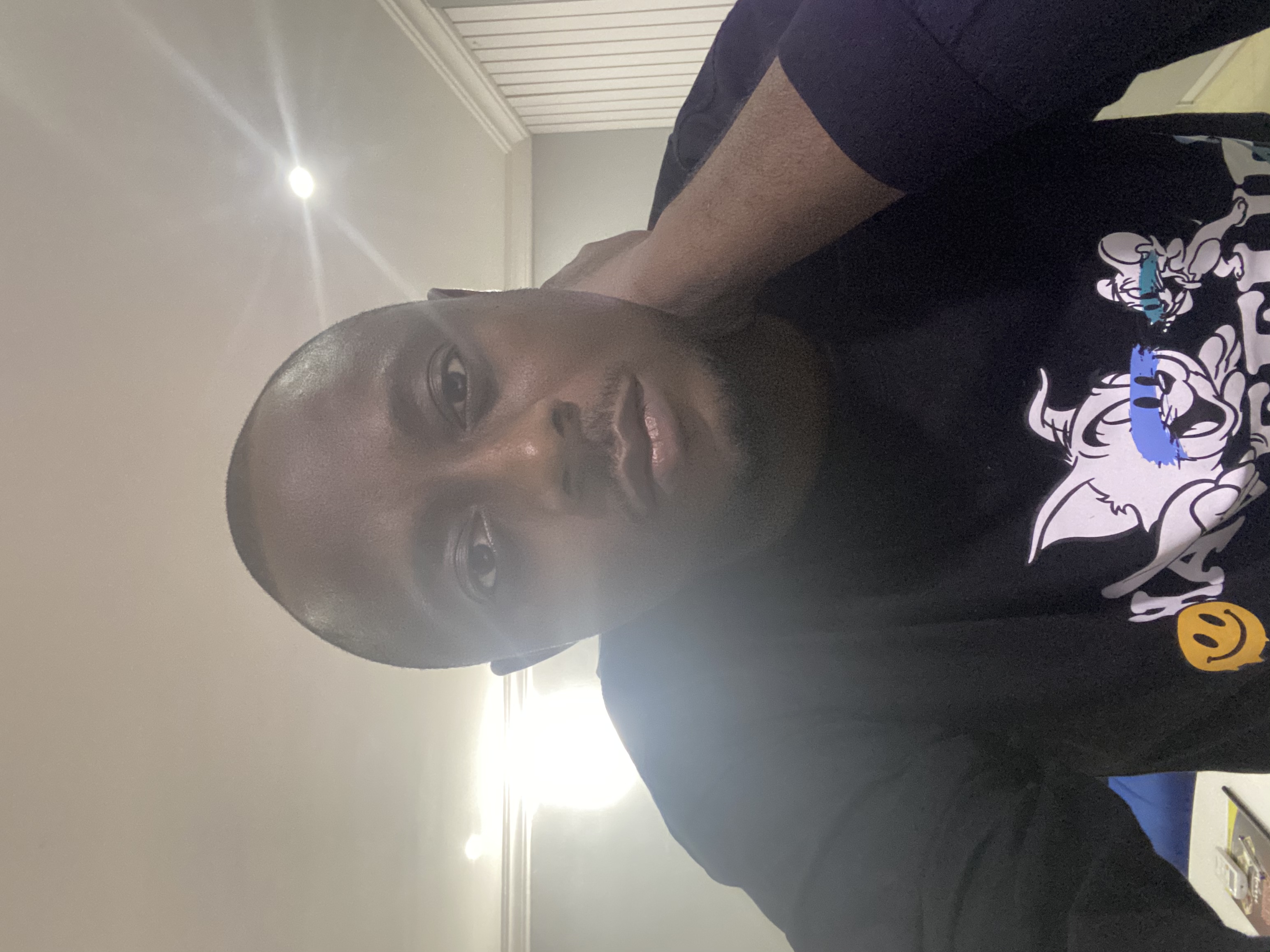
Ogunuyo Ogheneruemu B
Ogunuyo Ogheneruemu B
I'm Ogunuyo Ogheneruemu Brown, a senior software developer. I specialize in DApp apps, fintech solutions, nursing web apps, fitness platforms, and e-commerce systems. Throughout my career, I've delivered successful projects, showcasing strong technical skills and problem-solving abilities. I create secure and user-friendly fintech innovations. Outside work, I enjoy coding, swimming, and playing football. I'm an avid reader and fitness enthusiast. Music inspires me. I'm committed to continuous growth and creating impactful software solutions. Let's connect and collaborate to make a lasting impact in software development.