Creating a Toggle Menu with Show and Hide Functionality using HTML, CSS, and JavaScript
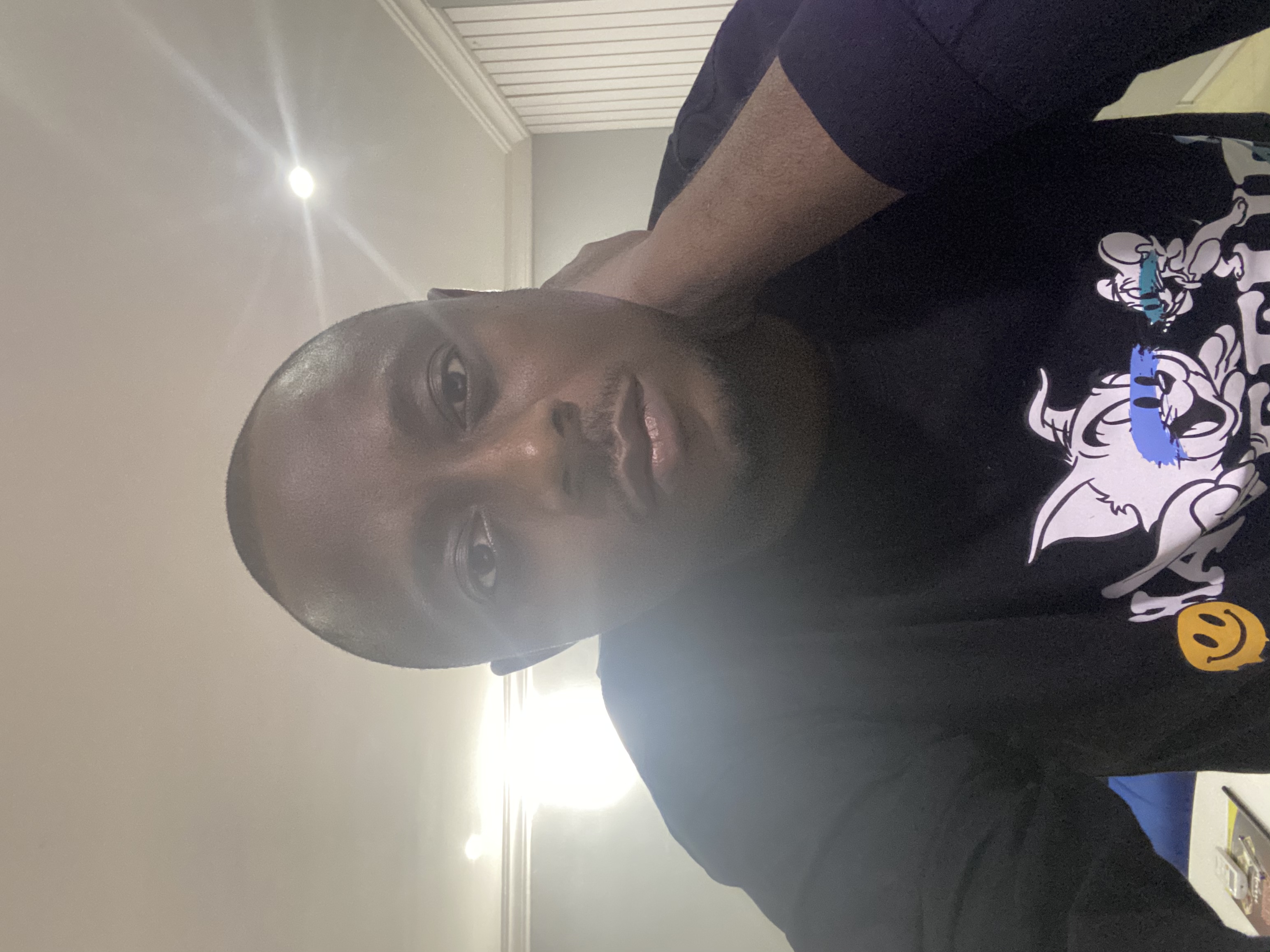
Table of contents
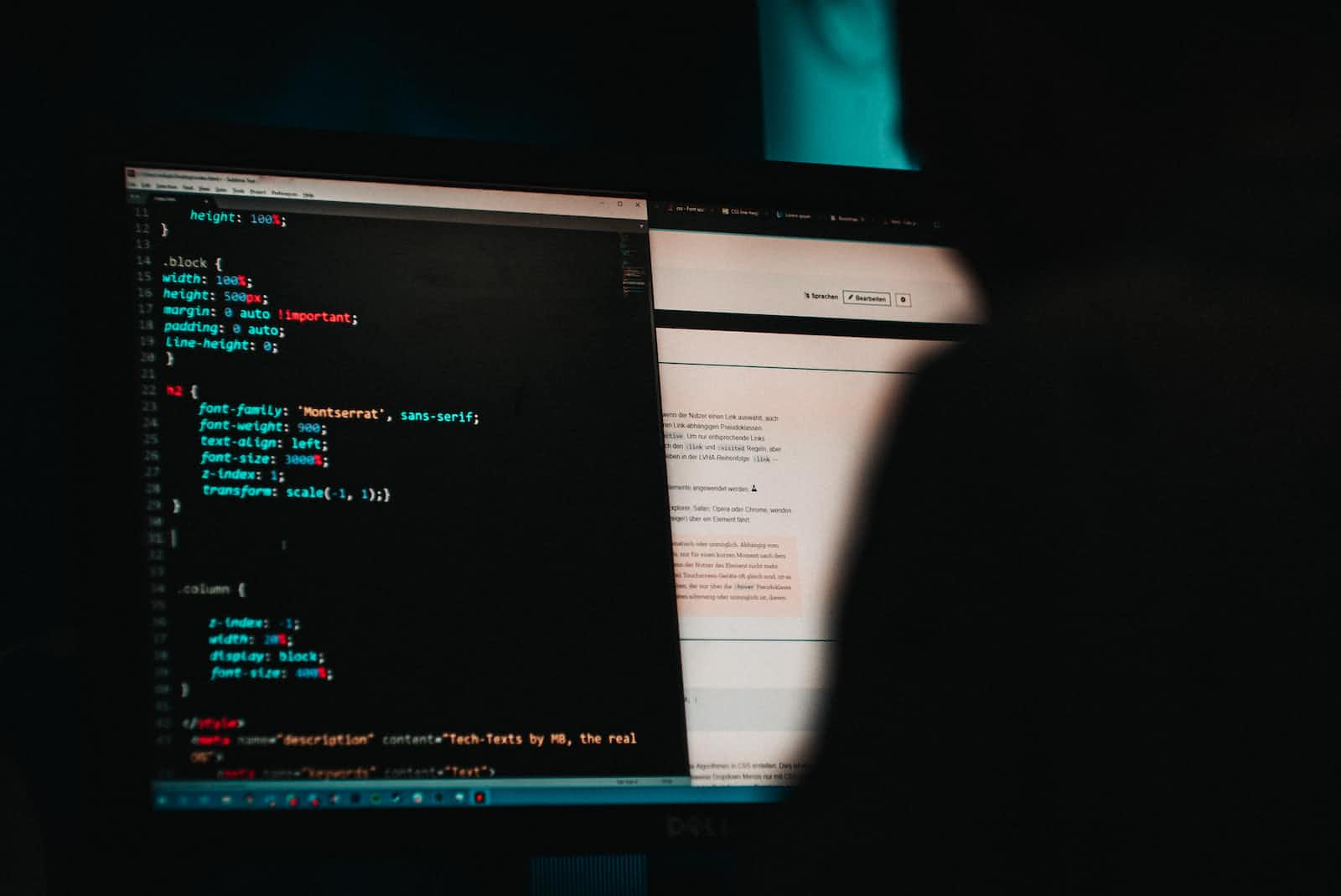
In this tutorial, we're going to create a toggle menu with show and hide functionality using a combination of HTML, CSS, and JavaScript. This interactive element can be a great addition to your web projects, providing a user-friendly way to display additional content without cluttering the main interface. We'll cover each step in detail, ensuring that you have a comprehensive understanding of how the toggle menu works.
Prerequisites:
- Basic knowledge of HTML, CSS, and JavaScript.
Step 1: Setting Up the HTML Structure To start, we'll set up the basic HTML structure of our webpage. This structure will include a header, content section, and a footer containing our toggle menu. You can utilize this structure as a foundation for your projects.
<!DOCTYPE html>
<html>
<head>
<!-- Add your title, links to fonts, and stylesheets here -->
</head>
<body>
<header>
<!-- Header content -->
</header>
<div class="content">
<!-- Your main content goes here -->
</div>
<div class="footers">
<div class="goat">
<!-- Menu button and other menu items -->
</div>
</div>
<div class="gugu">
<div id="brownmenu">
<!-- Menu content goes here -->
</div>
</div>
<!-- JavaScript code for toggle functionality -->
<script>
// JavaScript code will be added here
</script>
</body>
</html>
Step 2: Styling the Header and Content Next, we'll apply some initial styling to the header and content sections using CSS. This ensures that our webpage has a visually appealing layout.
/* Add your CSS styling for header and content sections */
/* ... (Your CSS code) ... */
Step 3: Designing the Toggle Menu In this step, we'll focus on creating the toggle menu's visual appearance. This menu will remain hidden until the user clicks the designated menu button.
/* Styling for the toggle menu and related elements */
#brownmenu {
/* Your styling for the toggle menu container */
}
.gugu {
/* Styling to hide the toggle menu initially */
}
.whiter {
/* Styling for menu text color */
}
.linker {
/* Styling for links within the menu */
}
Step 4: Implementing the Toggle Functionality The most crucial step is implementing the actual toggle functionality using JavaScript. This enables the menu to appear and disappear when the user interacts with the menu button.
// JavaScript code for toggle functionality
function menuclick() {
const gugu = document.querySelector(".gugu");
const body = document.body;
if (gugu.style.display === "" || gugu.style.display === "none") {
gugu.style.display = "block";
body.style.overflow = "hidden";
} else {
gugu.style.display = "none";
body.style.overflow = "auto";
}
}
// Add a click event listener to the document
document.addEventListener("click", function (event) {
const gugu = document.querySelector(".gugu");
const mackButton = document.querySelector(".mack");
if (!gugu.contains(event.target) && !mackButton.contains(event.target)) {
gugu.style.display = "none";
document.body.style.overflow = "auto";
}
});
Complete Code
<!DOCTYPE html>
<html>
<head>
<title>Styled Header and Content</title>
<link href="https://fonts.googleapis.com/icon?family=Material+Icons" rel="stylesheet">
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/4.7.0/css/font-awesome.min.css">
<link rel="stylesheet" href="css/style.css">
<style>
body {
margin: 0;
padding: 0;
font-family: Arial, sans-serif;
}
header {
background-color: #333;
color: white;
padding: 14px;
text-align: center;
position: sticky;
top: 0;
z-index: 100;
/* Ensure header stays on top of content */
}
h1 {
margin: 0;
font-size: 36px;
}
nav {
padding: 0;
display: flex;
justify-content: space-between;
margin-top: 0px;
}
.logo{
display: flex;
justify-content: center;
align-items: center;
}
.rightme {
display: flex;
flex-direction: row;
justify-content: space-between;
gap: 15px;
}
.butt{
padding: 10px;
border-radius: 5px;
}
.texty {
border: 1px solid black;
}
.footers {
background-color: #333;
color: white;
position: fixed;
bottom: 0;
z-index: 100;
/* Ensure header stays on top of content */
width: 100%;
}
.goat {
display: flex;
flex-direction: row;
justify-content: space-between;
padding: 20px;
}
.bottomflex {
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
gap: 5px;
font-size: 14px;
}
.content {
padding: 20px;
text-align: center;
margin-top: 120px;
/* Add margin to the top of content to allow space for the sticky header */
margin-bottom: 100px;
/* Add margin to the bottom of content */
overflow-y: auto;
/* Add vertical scroll if content overflows */
}
</style>
</head>
<body>
<header>
<nav>
<div class="logo">
<div>Logo</div>
</div>
<div class="rightme">
<div><button class="butt"><i class="fa fa-credit-card"></i></button></div>
<div><button class="butt">Login </button></div>
<div><button class="butt">Register </button></div>
</div>
</nav>
</header>
<div class="content">
<!-- Your content goes here -->
<!-- ... -->
<h2>Welcome to My Website</h2>
<p>This is an example of a blank HTML document with top and bottom menus. You can customize the content of this
page as needed.</p>
<h2>Welcome to My Website</h2>
<p>This is an example of a blank HTML document with top and bottom menus. You can customize the content of this
page as needed.</p>
<h2>Welcome to My Website</h2>
<p>This is an example of a blank HTML document with top and bottom menus. You can customize the content of this
page as needed.</p>
<h2>Welcome to My Website</h2>
<p>This is an example of a blank HTML document with top and bottom menus. You can customize the content of this
page as needed.</p>
<h2>Welcome to My Website</h2>
<p>This is an example of a blank HTML document with top and bottom menus. You can customize the content of this
page as needed.</p>
<h2>Welcome to My Website</h2>
<p>This is an example of a blank HTML document with top and bottom menus. You can customize the content of this
page as needed.</p>
<h2>Welcome to My Website</h2>
<p>This is an example of a blank HTML document with top and bottom menus. You can customize the content of this
page as needed.</p>
<h2>Welcome to My Website</h2>
<p>This is an example of a blank HTML document with top and bottom menus. You can customize the content of this
page as needed.</p>
<h2>Welcome to My Website</h2>
<p>This is an example of a blank HTML document with top and bottom menus. You can customize the content of this
page as needed.</p>
<h2>Welcome to My Website</h2>
<p>This is an example of a blank HTML document with top and bottom menus. You can customize the content of this
page as needed.</p>
<h2>Welcome to My Website</h2>
<p>This is an example of a blank HTML document with top and bottom menus. You can customize the content of this
page as neededy.</p>
</div>
<div class="footers">
<div class="goat">
<a class="bottomflex whiter linker mack" id="button1" onclick="menuclick()">
<span><i class="fa fa-align-justify" style="font-size:16px"></i></span>
<span> Menu </span>
</a>
<a class="bottomflex">
<span><i class="fa fa-bookmark" style="font-size:16px"></i></span>
<span> MyBets </span>
</a>
<a class="bottomflex">
<span><i class="fa fa-gamepad" style="font-size:16px"></i></span>
<span> BetSlip </span>
</a>
<a class="bottomflex">
<span><i class="fa fa-lastfm-square" style="font-size:16px"></i></span>
<span> Casino </span>
</a>
<a class="bottomflex">
<span><i class="fa fa-wechat" style="font-size:16px"></i></span>
<span> Chat </span>
</a>
</div>
</div>
<div class="gugu">
<div id="brownmenu">
</div>
</div>
<script>
function menuclick() {
const gugu = document.querySelector(".gugu");
const body = document.body;
if (gugu.style.display === "" || gugu.style.display === "none") {
gugu.style.display = "block";
body.style.overflow = "hidden";
} else {
gugu.style.display = "none";
body.style.overflow = "auto";
}
}
// Add click event listener to the document
document.addEventListener("click", function (event) {
const gugu = document.querySelector(".gugu");
const mackButton = document.querySelector(".mack");
// Check if the click target is not within gugu or mackButton
if (!gugu.contains(event.target) && !mackButton.contains(event.target)) {
gugu.style.display = "none";
document.body.style.overflow = "auto";
}
});
</script>
</body>
</html>
style.css
#brownmenu{
position: fixed;
bottom: 10%;
left: 0;
width: 100%;
height: 60vh;
background-color: #262626;
display: flex;
flex-direction: column;
z-index: 999;
border-top-left-radius: 10px;
border-top-right-radius: 10px;
gap: 10px;
}
.gugu{
display: none;
}
.whiter{
color: white;
}
.linker{
text-decoration: none;
cursor: pointer;
}
Conclusion: By following these steps and using the provided code snippets, you've successfully created a toggle menu with show and hide functionality. This interactive feature enhances user experience and provides an organized way to display additional content. Feel free to customize the styling and content according to your project's requirements. With this newfound knowledge, you can easily incorporate toggle menus into your web projects to create more intuitive and user-friendly interfaces.
Subscribe to my newsletter
Read articles from Ogunuyo Ogheneruemu B directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
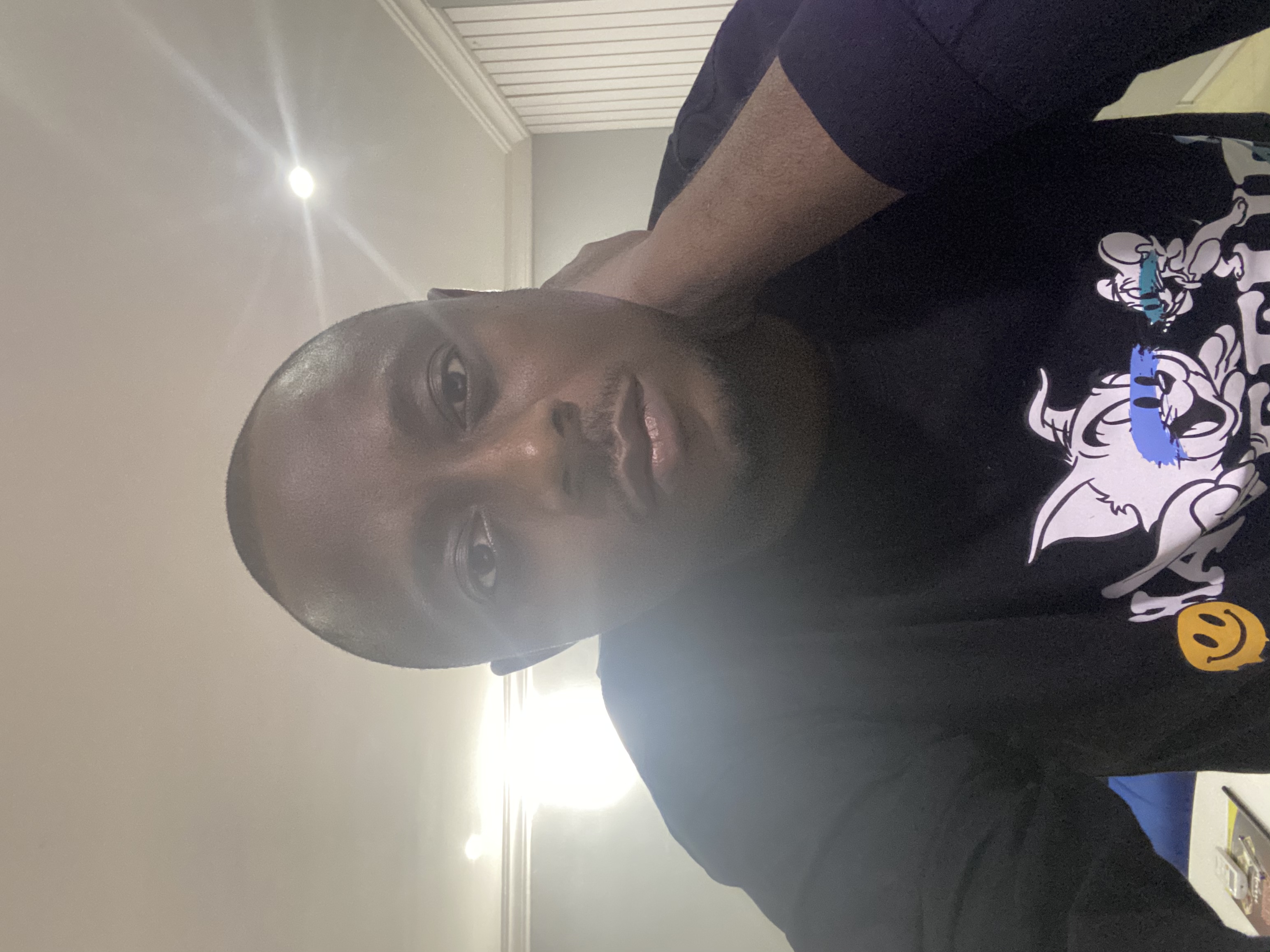
Ogunuyo Ogheneruemu B
Ogunuyo Ogheneruemu B
I'm Ogunuyo Ogheneruemu Brown, a senior software developer. I specialize in DApp apps, fintech solutions, nursing web apps, fitness platforms, and e-commerce systems. Throughout my career, I've delivered successful projects, showcasing strong technical skills and problem-solving abilities. I create secure and user-friendly fintech innovations. Outside work, I enjoy coding, swimming, and playing football. I'm an avid reader and fitness enthusiast. Music inspires me. I'm committed to continuous growth and creating impactful software solutions. Let's connect and collaborate to make a lasting impact in software development.