Transitioning to Doubly Linked Lists: A Balanced Approach
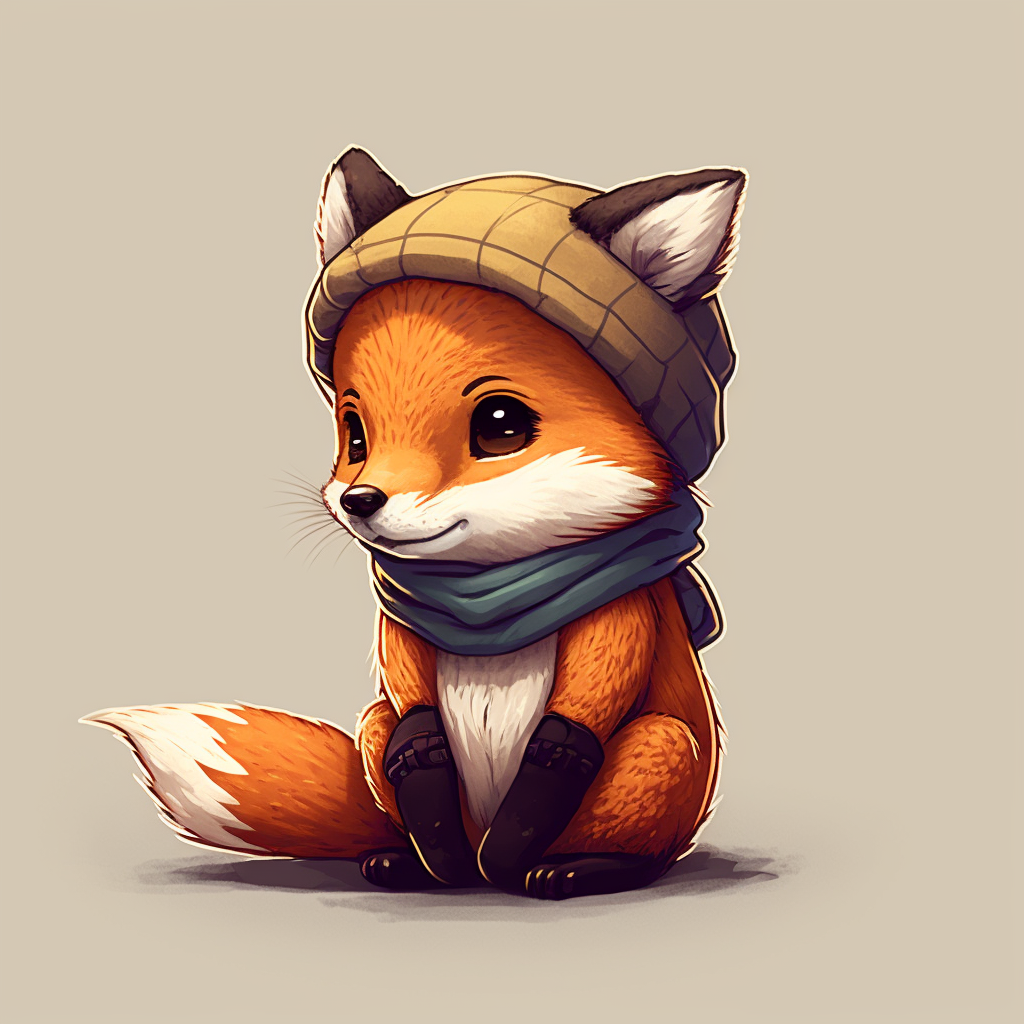
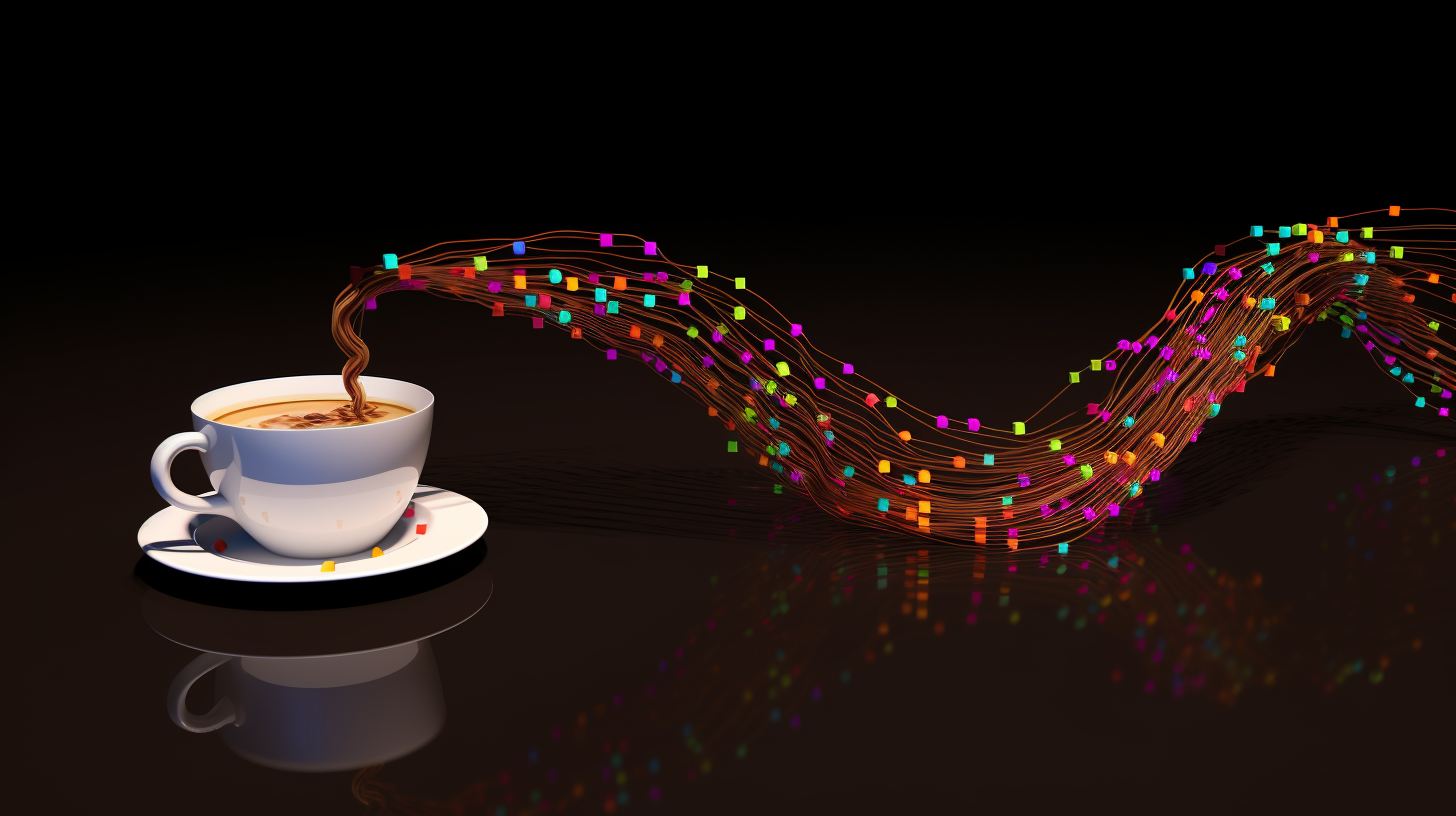
In previous posts, we delved into the fascinating world of singly linked lists. While singly linked lists are a valuable data structure, there are instances where a more robust solution is needed. Enter the doubly linked list.
What is a Doubly Linked List?
A doubly linked list is similar to a singly linked list, with the main distinction being that each node in a doubly linked list has a reference to both its next node and its previous node. In a singly linked list, each node only references its next node.
Here's a basic structure for a node in a doubly linked list:
class DoubleNode {
int value;
DoubleNode next;
DoubleNode prev;
public DoubleNode(int value) {
this.value = value;
this.next = null;
this.prev = null;
}
}
Why Use a Doubly Linked List?
Advantages:
Bidirectional Navigation: In a doubly linked list, you can traverse both forwards and backwards. This can be particularly useful in applications where such flexibility is required, like implementing certain algorithms or navigation within a user interface.
Easier Deletion of Nodes: Deleting nodes, especially from the middle of the list, is more straightforward, since you have direct access to the previous node.
Enhanced Flexibility: The additional reference to the previous node opens up new possibilities for manipulation, making certain operations more efficient.
Disadvantages:
Increased Memory Consumption: Storing an additional reference for each node (the previous node) does take up more memory. This might not be ideal in memory-constrained environments.
Complexity: The implementation of insertion, deletion, and other operations becomes slightly more complex due to the need to maintain two pointers.
Example of Doubly Linked List in Java:
Here's an abbreviated example of a doubly linked list implementation in Java:
public class DoublyLinkedList {
private DoubleNode head;
private DoubleNode tail;
public DoublyLinkedList(int value) {
DoubleNode newNode = new DoubleNode(value);
head = newNode;
tail = newNode;
}
public void append(int value) {
DoubleNode newNode = new DoubleNode(value);
newNode.prev = tail; // Set the previous reference
tail.next = newNode; // Set the next reference
tail = newNode; // Update the tail
}
// Additional methods like insert, delete, etc.
}
Conclusion:
Doubly linked lists are a powerful extension of singly linked lists, offering greater flexibility at the cost of increased memory and complexity. The decision to use a doubly linked list over a singly linked list depends on specific use-cases and requirements. Understanding both types of linked lists and their respective advantages and disadvantages helps in choosing the right data structure for a given problem.
Next time, we'll dive into specific algorithms and techniques that leverage doubly linked lists. Stay tuned!
Subscribe to my newsletter
Read articles from Jose Ramirez directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
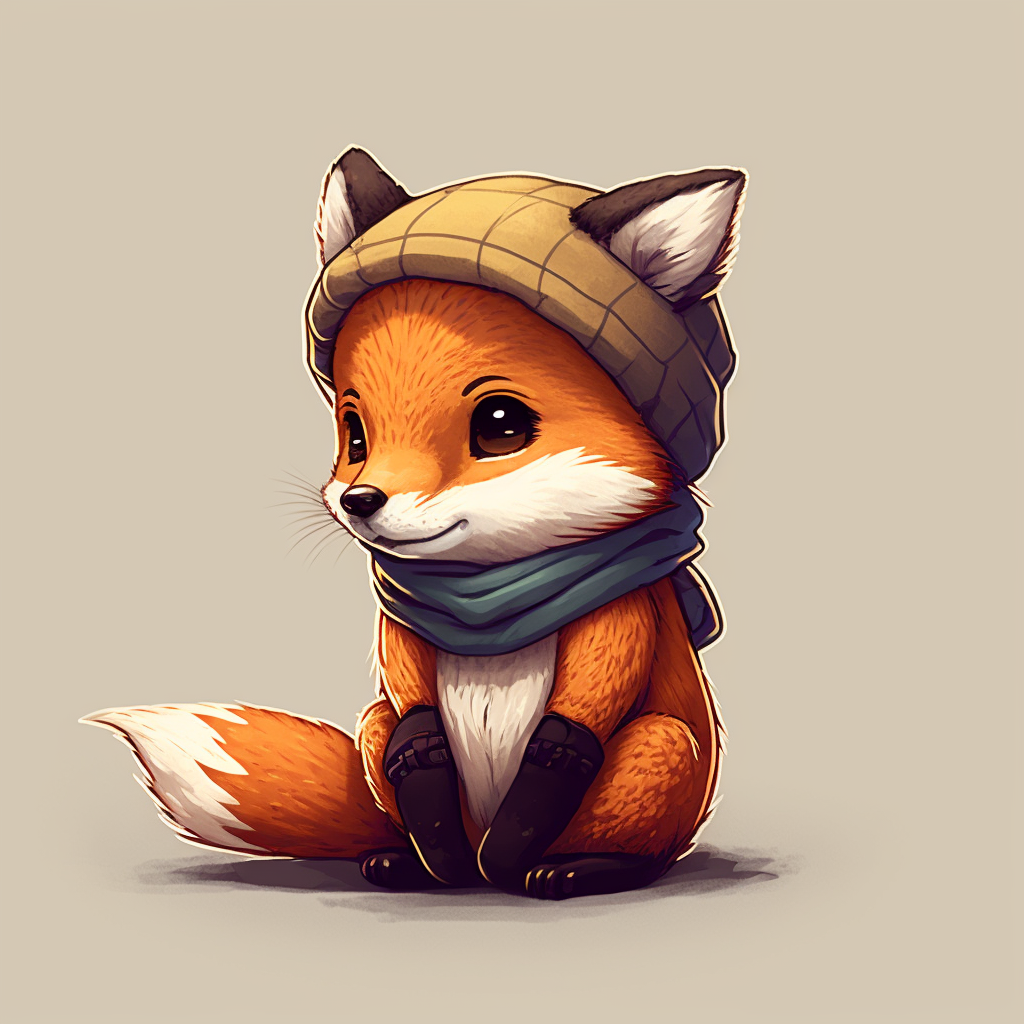