Java Loop Control: Mastering For, For-each, While, and Do-While Loops
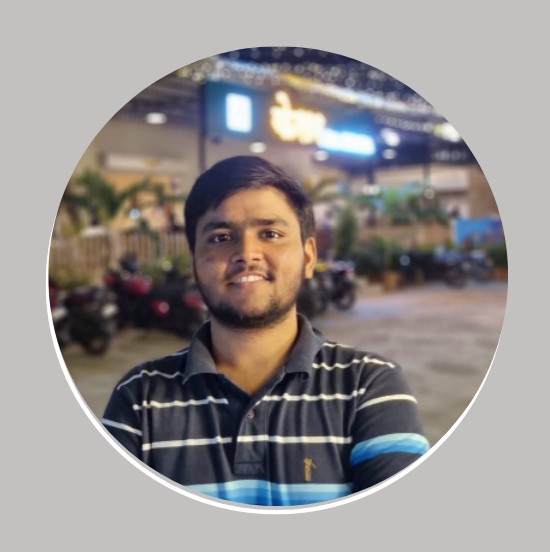
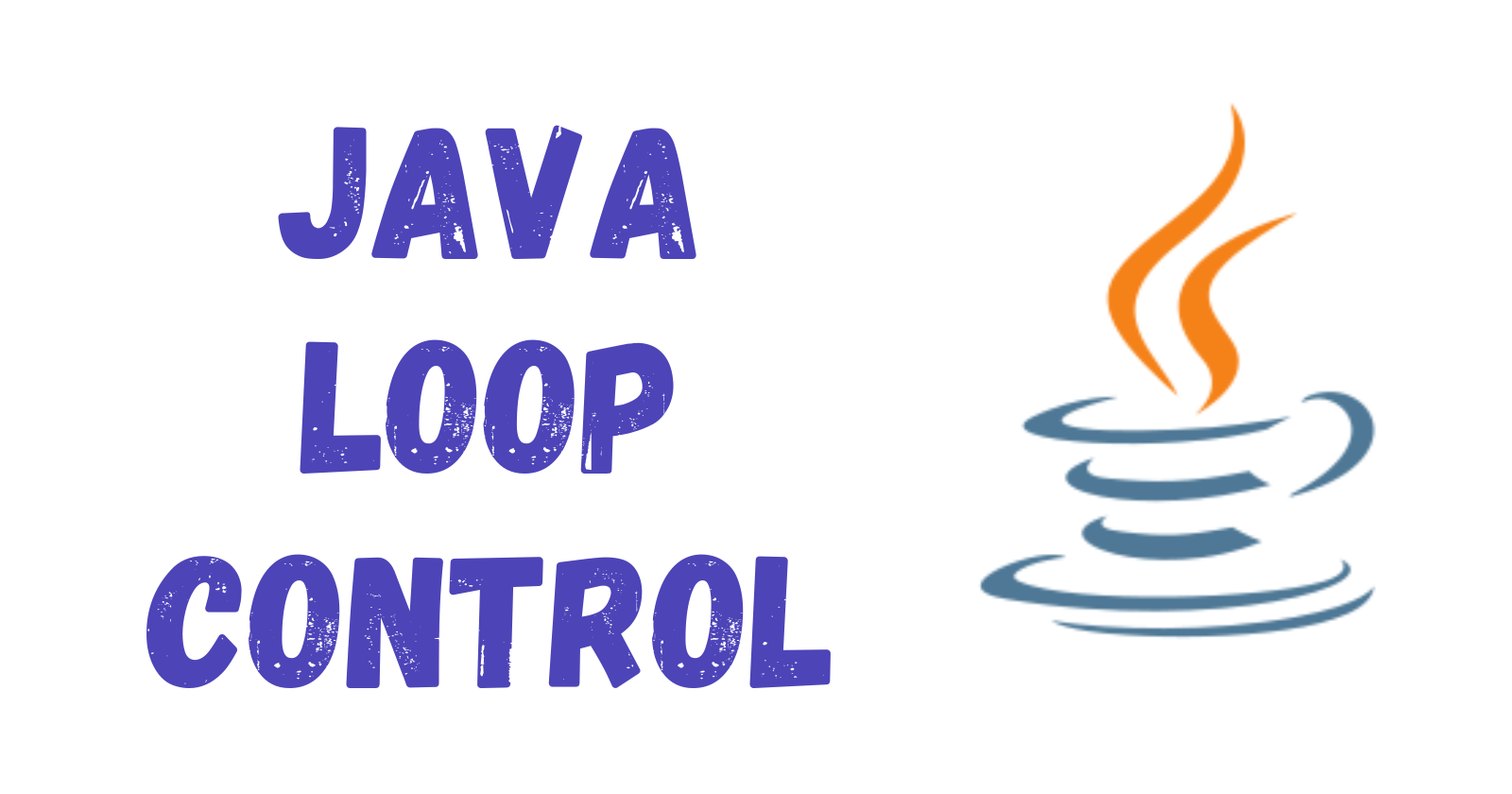
Welcome back, dear readers, to our exciting Java programming series! In our previous articles, we covered topics such as Java Basic Operators and Modifier Types. If you missed any of those articles, we highly recommend giving them a read to strengthen your Java knowledge. In this article, we'll explore an essential aspect of Java programming - loop control. Understanding loop control is vital for repeating code execution, iterating through collections, and handling various scenarios in your Java programs. Let's dive into the world of Java loop control together.
1) Introduction to Java Loop Control
Loop control structures in Java enable repetitive execution of a block of code based on a specified condition. Loops are powerful tools for automating tasks and processing collections of data. In this article, we'll explore the different types of loops available in Java, including for loop, for-each loop, while loop, and do-while loop.
2) For Loop in Java
The for loop is a widely used loop structure in Java. It allows you to execute a block of code a fixed number of times. The loop has three parts: initialization, condition, and update. Here's an example:
for (int i = 1; i <= 5; i++) {
System.out.println("Iteration: " + i);
}
In the above example, the for loop will execute five times, displaying the iteration number each time.
3) For-each Loop in Java
The for-each loop is used to iterate over elements in an array or collections such as ArrayList. It simplifies the process of traversing through elements. Here's an example:
String[] names = {"Alice", "Bob", "Charlie", "David"};
for (String name : names) {
System.out.println(name);
}
In the above example, the for-each loop will print each name in the array names
.
4) Pitfalls of Loops
While loops are powerful tools for repetitive execution, they can also lead to potential pitfalls if not used carefully. Let's explore some common pitfalls associated with loops and best practices to avoid them:
a) Infinite Loops: One of the most critical pitfalls is creating an infinite loop, where the loop's exit condition is never met. This can cause your program to hang or become unresponsive. For example:
while (true) {
// Code that should eventually break the loop
}
To prevent infinite loops, ensure that the loop's exit condition is reachable and will eventually be met during execution.
b) Inadequate Exit Conditions: Another pitfall is providing exit conditions that don't account for all possible scenarios. If the exit condition is not adequately defined, the loop may terminate prematurely or not at all. Carefully consider the loop's conditions to avoid unintended behavior.
c) Modifying Loop Variables Inside Loop: Modifying loop control variables inside the loop can lead to unpredictable results. It can cause the loop to terminate unexpectedly or not execute as intended. For example:
int i = 0;
while (i < 5) {
System.out.println(i);
i++;
// Avoid modifying i inside the loop
i = i + 2; // Modifying loop variable can lead to issues
}
To avoid this, it's essential to ensure that the loop control variable is modified only outside the loop.
d) Loops with No Exit Condition: If a loop does not have an exit condition, it will run indefinitely, leading to infinite execution. It's crucial to define a clear exit condition to ensure the loop terminates at the appropriate time.
e) Accidental Infinite Loops: Sometimes, during coding, developers may accidentally introduce conditions that inadvertently create infinite loops. This can happen due to logical errors, incorrect initialization of loop control variables, or improper exit conditions. It's essential to carefully review loops and verify their conditions to avoid accidental infinite loops.
f) Inefficient Looping: In some cases, loops can be inefficient if not optimized correctly. For instance, if a loop iterates over a large dataset repeatedly, it can impact the program's performance. Consider using appropriate data structures or algorithms to optimize loops for efficiency.
g) Incorrect Loop Termination: The loop termination condition should be accurate to ensure the loop exits at the right time. If the termination condition is incorrect, it may lead to an incorrect number of iterations or unexpected behavior.
To avoid these pitfalls, follow these best practices:
Use Clear and Concise Exit Conditions: Ensure that the loop's exit condition is explicit, easy to understand, and accounts for all possible scenarios.
Initialize Loop Control Variables Properly: Initialize loop control variables before entering the loop to avoid unexpected behavior.
Verify Loop Conditions During Testing: Always test your loops with various input scenarios to verify that they function as intended and terminate appropriately.
Use for-each Loop When Appropriate: If you are iterating through collections, consider using the for-each loop, as it reduces the chances of making errors related to loop control variables.
Break Up Complex Loops: If you find a loop becoming too complex, consider breaking it down into smaller, more manageable loops or consider using separate methods to improve readability and maintainability.
Avoid Hardcoding Exit Conditions: Avoid hardcoding exit conditions and, instead, use variables or constants to improve code flexibility and maintainability.
By being mindful of these pitfalls and implementing best practices, you can ensure that your loops are reliable, efficient, and free from unintended issues. Loops are powerful constructs, and with proper use, they can significantly enhance your Java programs' functionality.
5) While Loop in Java
The while loop executes a block of code as long as the specified condition is true. It is useful when the number of iterations is unknown or determined at runtime. Here's an example:
int count = 1;
while (count <= 5) {
System.out.println("Count: " + count);
count++;
}
In the above example, the while loop will execute five times, displaying the count each time.
6) Do-While Loop in Java
The do-while loop is similar to the while loop, but it guarantees that the block of code will be executed at least once before checking the condition. Here's an example:
int num = 1;
do {
System.out.println("Number: " + num);
num++;
} while (num <= 5);
In the above example, the do-while loop will execute five times, displaying the number each time.
7) Break and Continue Statement in Java
Java provides two loop control statements - break
and continue
- to modify the normal flow of loops. These statements offer more control over loop execution and help improve code readability and efficiency. Let's explore these statements in detail:
a) Break Statement: The break
statement is used to terminate the execution of a loop prematurely. When the break
statement is encountered inside a loop, the loop is immediately exited, and the program continues executing the code after the loop. The break
statement is typically used to stop a loop when a certain condition is met or to exit a loop early based on specific requirements.
Here's an example of using the break
statement:
for (int i = 1; i <= 10; i++) {
if (i == 5) {
break; // Exit the loop when i becomes 5
}
System.out.println(i);
}
Output:
1
2
3
4
In this example, when the value of i
becomes 5, the break
statement is encountered, and the loop is terminated, preventing the execution of the remaining iterations.
b) Continue Statement: The continue
statement is used to skip the rest of the current iteration and continue with the next iteration of the loop. When the continue
statement is encountered, the loop immediately proceeds to the next iteration, skipping the remaining code inside the loop for that particular iteration. The continue
statement is often used to avoid executing certain parts of the loop based on specific conditions.
Here's an example of using the continue
statement:
for (int i = 1; i <= 5; i++) {
if (i == 3) {
continue; // Skip iteration when i is 3
}
System.out.println(i);
}
Output:
1
2
4
5
In this example, when the value of i
becomes 3, the continue
statement is encountered, skipping the code inside the loop for i = 3
, and the loop proceeds to the next iteration.
c) Nested Loops and Break: When using break
in nested loops (loops inside loops), the break
statement will only terminate the innermost loop in which it is encountered. If you want to break out of multiple loops simultaneously, you can use labeled break
statements. Here's an example:
outerLoop: for (int i = 1; i <= 3; i++) {
for (int j = 1; j <= 3; j++) {
if (i == 2 && j == 2) {
break outerLoop; // Exit both loops when i is 2 and j is 2
}
System.out.println("i: " + i + ", j: " + j);
}
}
Output:
i: 1, j: 1
i: 1, j: 2
i: 1, j: 3
In this example, when i
becomes 2 and j
becomes 2, the labeled break outerLoop;
statement is encountered, exiting both the inner and outer loops simultaneously.
8) Conclusion
Congratulations on reaching the end of our Java Loop Control article! You've gained valuable knowledge about for, for-each, while, and do-while loops in Java. Loops are indispensable tools for automating tasks and processing data in your Java programs.
Remember, practice is key to mastering loop control in Java. Experiment with different scenarios, iterate through arrays, and handle various scenarios with loops. Happy coding!
Subscribe to my newsletter
Read articles from Mayank Bohra directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
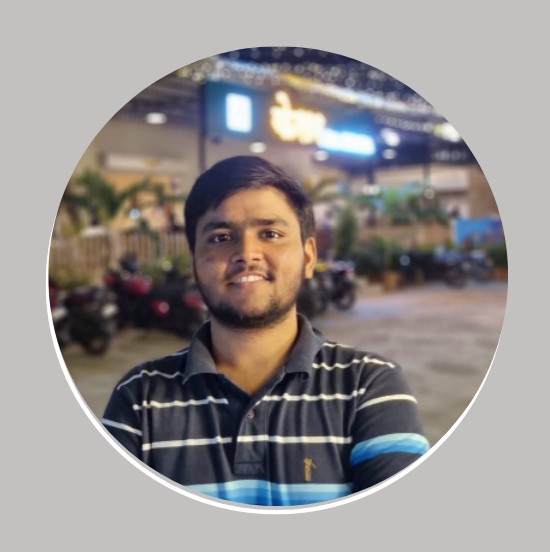
Mayank Bohra
Mayank Bohra
I'm a passionate Computer Science Engineering student, currently pursuing my degree and expected to graduate in 2025. With a focus on web development, database engineering, and machine learning, I'm enthusiastic about exploring the fascinating world of data science. My programming skills include Java and Python, which I utilize to create innovative solutions and contribute to the tech community. As a dedicated learner, I actively share my knowledge and insights as a contributor @ GeeksforGeeks. Join me on this exciting journey as we explore the intersection of technology and creativity.