Activity and the activity life cycle

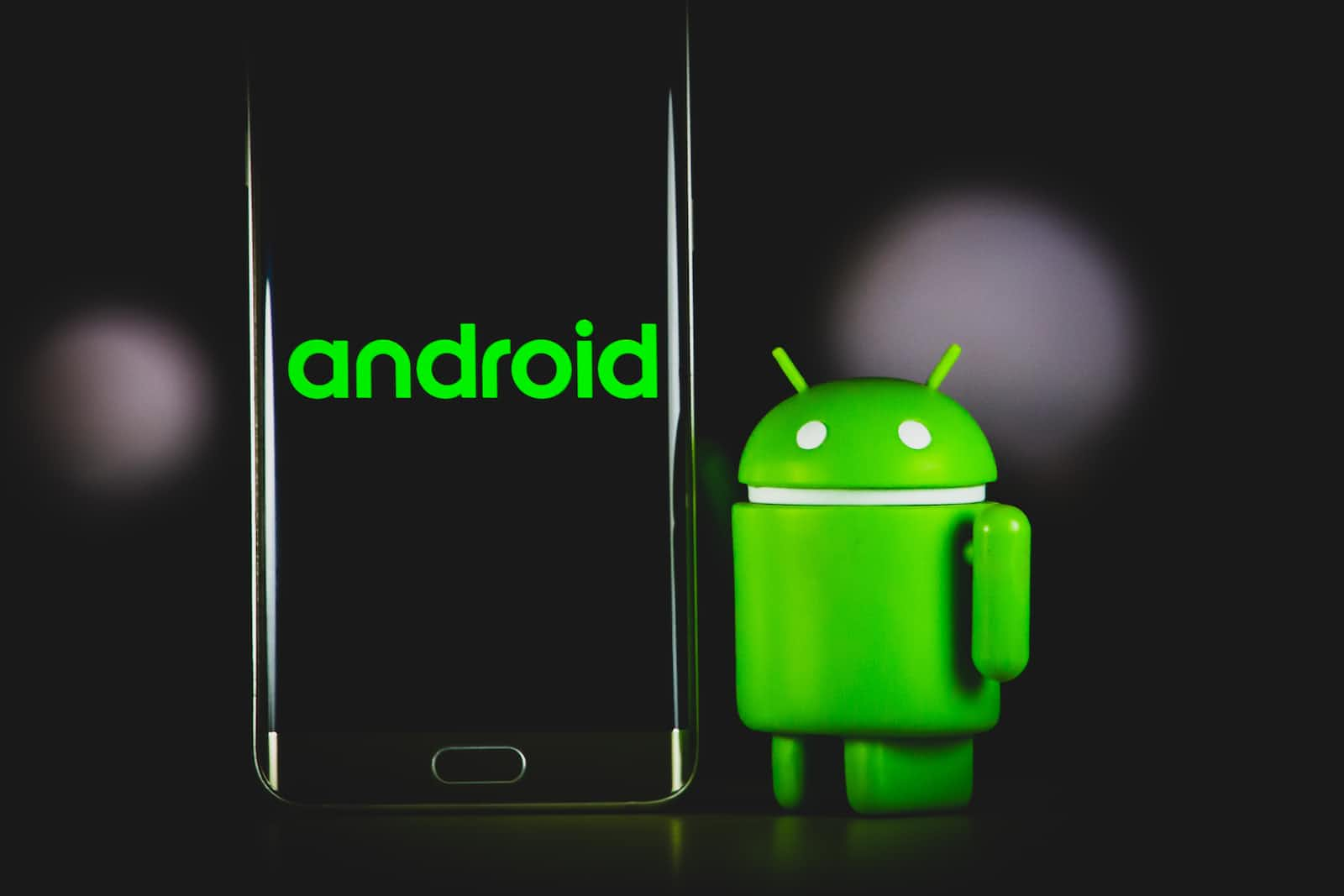
Introduction to activities in Android
Activities are part of the basic component of the Android application. Users cannot interact with an application on their mobile device without an activity. Activities form the bedrock upon which our mobile application is built. It consists of the layout, text fields, images, and different UI elements which the user can interact with.
Every mobile application you open, the first screen you see is an activity. In Android Studio activities are created using the Java or Kotlin programming language.
Activities are linked through intents, that are used for communication and navigation between different activities. Intents can be used to start a new activity, pass data between activities, or even trigger actions in other components of the app.
This article will provide a detailed explanation of activities, their importance, and activity life cycle along with its methods.
What is an activity
Activity is more like a container for one or more multiple screens in your app. It's not just seen as a screen but also as a unit that users interact with in your application.
Activities contain information about whether a user is currently interacting with the screen or if the activity is in the background.
It also serves as an entry point for your app. We can have multiple screens in an application that are bundled together into an activity.
For example, A user profile page activity can contain other screens like information details of the user.
However, Jetpack Compose has made everything easier. Using the jetpack compose UI we can have only one main activity with multiple screens while staying in the single main activity.
The main activity in Jetpack Compose serves as the entry point of our application. The main characteristic of activities in Android is the LIFECYCLE which means at some point our activity is created, destroyed, paused, etc.
Importance of activities in Android.
The importance of activities cannot be overemphasized as they are the bedrock or building block of every mobile application.
Activities serve as a container that houses UI elements with which the user can interact. Activities also play a vital role in the organization of our mobile applications. They inherit specific functionalities making it easier to manage your application code base.
In terms of Multitasking and App Switching, activities allow Android users to multitask by switching between different apps seamlessly.
Activities make it simpler to test and debug particular application components in isolation, which makes it easier to find problems and quickly fix them.
Basic components of an activity
Layout XML: the layout XML file represents the user interface of an activity. These files contain view-groups (linear layout, constraint layout) and views such as buttons, images, app bars, etc.
The XML file defines the structure and positioning of these components which the user will interact with like clicking a button to open a new activity.
However, in Jetpack Compose you can define and structure the features of your app inside the main.activity.kt file.
Activity class: An activity class comprising a single screen with a user interface is represented by an instance(object) of the Activity class in Android.
To create a custom activity you must create a subclass of the Activity class and override its lifecycle methods and other crucial methods to the application's needs.
Life cycle methods: these methods perform various behaviors in activity and can be overridden in different stages. The methods are:`onCreate()`,
onStart()
,onResume()
,onPause()
,onStop()
,onDestroy()
, etc.User interface components: this represents the user interface of an activity i.e. how the app looks on the screen to the user. UI can be programmed in Java/Kotlin or using the XML layout. We also have the jetpack compose UI which is now used for building Android applications.
Intents: interaction between other activities and other app components is made possible through intent. Intents can be used to initiate an action from one activity to another or from an application to another app component.
For example, suppose you want to set your profile photo in a messaging app. In that case, an intent can be used to open your gallery or camera application to select a photo for upload or snap using my phone camera.
Back stack: activities are stacked on each other. When an activity is started, they are placed on a stack called the back stack. This enables the user to return to previous activities by hitting the back button.
Navigation: activities in Android can be started, finished, and used for data transfers between different parts of the application. They manage the navigation flow of your Android application.
Manifest file: every activity in Android must be declared in the androidManifest.xml file. This file consists of the app configuration and also it provides essential information about the app's components, like the services, app icon, app name, intent filters, etc.
Activity callbacks: An activity can receive a callback result from other activities. For instance, an activity can receive a callback result when it is executed with the startActivityForResult().
Overview of the Android activity life cycle
The Android activity lifecycle represents a series of events and states that an Android activity goes through from creation to destruction.
A deep understanding of the activity life cycle is very crucial in handling user interaction and experience in an Android application. The activity life cycle consists of the following states and corresponding call-back methods
onCreate()
onStart()
onResume()
onPause()
onStop()
onRestart()
onDestroy()
It is vital to note that the activity lifecycle is closely related to tasks and back stack concepts in Android.
Whenever a new Activity is initiated, it is placed on a stack and the Activities are removed from the stack when a user navigates back.
States of an activity
Let's now delve into the different states of activity and their respective life cycle methods.
onCreate(): when the activity is created the onCreate() method is called and we move on to the created life cycle. In the onCreate() method we can initialize our variables, views, and other UI content of our activity.
At this point, the user does not see anything on the screen after the onCreated state the activity will start to move to the started state. So onStart() will be called when that is complete
OnStart(): an activity is considered to be started when visible to the user, but at this point, the user still can't interact with it.
This can be compared with a theater with the curtains open up initially and we can already see the actors and the actress from the play but the play itself is not starting yet. However, after the start state, it will move to the resume state.
onResume(): when an activity is in the resume state it means it's in the foreground where the user can interact with it and that's where it will stay until the user eventually moves away from it.
From the diagram above, the activity will block the state until something is happening.
onPause(): As soon as another activity comes in the foreground the life cycle moves to the on pause()state. It doesn't necessarily mean it must be an activity that comes in the foreground, it can be a dialog pop-up or any other UI.
At this point, all the resources the activity needs will be kept in memory because the user might still come back to the activity which is very likely if they see a dialog. It could also be that they navigate through different screens of their application where they might decide to click the back button and come back to the previous activity and if that happens the activity that is in the pause state comes back into the foreground.
When the user returns to the activity the lifecycle will move back to the resume state and it is in the foreground until the user moves away again.
onStop(): when the user navigates from one activity to another activity then the onStop cycle will be called and the life cycle state will move to the onStop state. e.g. when a user moves from the home page screen of an application to the profile page screen.
note: when an activity is in the stop state it is not visible to the user, if it is in the pause state the user could be seeing a dialog and the actual activity that is paused.
onRestart(): this is when a user comes back to an app that was minimized and that' was hidden. After that onStart will be called again so that the activity will be made invisible to the user and then resume where the user can finally interact again.
onDestroy(): here the activity is finishing or being destroyed by the system. It means you intentionally closed the activity or the user is currently active on the activity and then they clicked the back button. The current activity will be destroyed.
There is another case where the life cycle will reach the destroyed state and that is also called the configuration changes i.e. when some kind of global configuration changes.
Example with Activity States
An example is the screen rotation. When the user rotates the device that means the layout, of the activity also needs to be loaded from the resources. Maybe there is a specific landscape layout so everything will be set up from the very beginning.
In that case, the whole activity will be recreated and the life cycle will start from the onCreate as soon as the device is in landscape mode.
package com.example.firstcompose
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.tooling.preview.Preview
import com.example.firstcompose.ui.theme.FirstComposeTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
println("onCreate")
setContent {
FirstComposeTheme {
// A surface container using the 'background' color from the theme
Surface(modifier = Modifier.fillMaxSize(), color = MaterialTheme.colorScheme.background) {
Greeting("Android")
}
}
}
}
override fun onStart() {
super.onStart()
println("onStart")
}
override fun onResume() {
super.onResume()
println("onResume")
}
override fun onPause() {
super.onPause()
println("onPause")
}
override fun onStop() {
super.onStop()
println("onStop")
}
override fun onDestroy() {
super.onDestroy()
println("onDestroy")
}
override fun onRestart() {
super.onRestart()
println("onRestart")
}
}
@Composable
fun Greeting(name: String, modifier: Modifier = Modifier) {
Text(
text = "Hello $name!",
modifier = modifier
)
}
@Preview(showBackground = true)
@Composable
fun GreetingPreview() {
FirstComposeTheme {
Greeting("Android")
}
}
When you launch the app on Android studio Go to your log cat and filter for system.out
The onCreate(), onStart(), onResume() functions are called once the app launches.
When you minimize the app the onPause() and onStop() state is called onPause() because you move into the background and onStop() because it completely gets invisible to the user.
When you get back to the activity onRestart(), onStart() and onResume() is called.
When the user closes the app completely onPause(), onStop(),onDestroy() state is called.
Conclusion
In this article, we have comprehensively learned about activities and the activity lifecycle in Android.
It is important to understand this concept as an Android developer, as it is crucial in creating robust and seamless mobile applications.
Activities serve as the bedrock upon which the app's user interface is built facilitating an effortless user experience. The knowledge of activities and their life cycle enables developers to build user-friendly applications.
Subscribe to my newsletter
Read articles from Etugbo Judith directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Etugbo Judith
Etugbo Judith
I'm a Technical writer passionate about breaking down complex concepts into clear, concise and engaging content.