Python Libraries for DevOps: Streamlining Development and Operations ๐
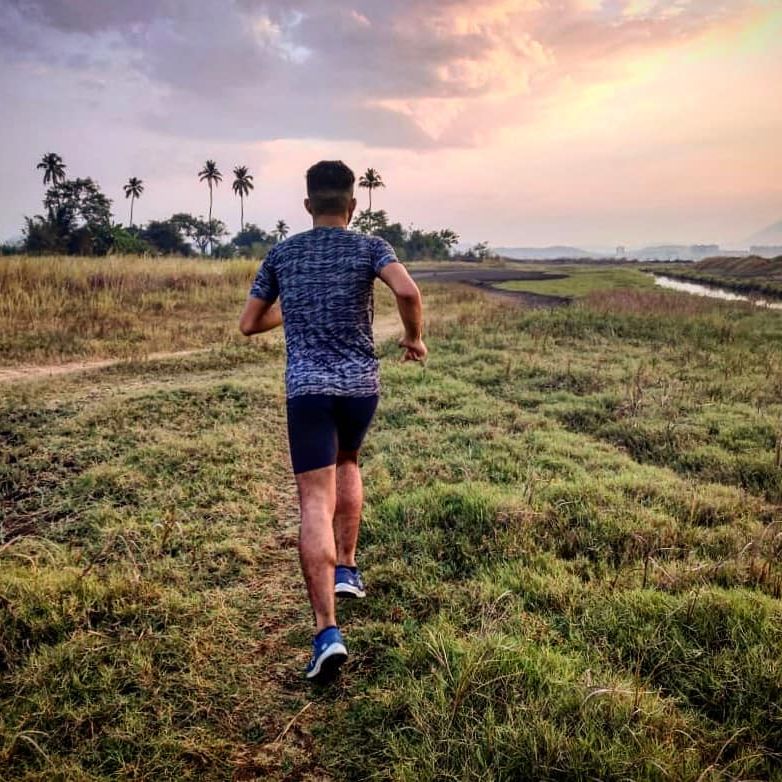
Introduction: In the ever-evolving landscape of software development and operations, DevOps practices have gained significant traction. DevOps aims to bridge the gap between development and IT operations, promoting collaboration, automation, and faster software delivery. Python, with its simplicity and versatility, has become a popular choice among DevOps professionals. In this blog, we'll explore some essential Python libraries that empower DevOps teams to streamline their workflows, automate processes, and enhance overall efficiency. Let's dive in! ๐ ๏ธ๐ง
Fabric (โ๏ธ): Fabric is a robust library that enables remote execution and automation of tasks. DevOps engineers can use it to perform tasks such as deploying applications, managing servers, and executing scripts across multiple servers simultaneously. With its SSH support and simple API, Fabric simplifies server management and configuration.
Example Code:
from fabric import Connection def deploy_app(): with Connection('server_host') as conn: conn.run('git pull origin master') conn.run('docker-compose up -d')
Ansible (๐): Ansible is a powerful automation tool widely used in DevOps. While it's not a pure Python library, Ansible leverages Python for its configuration files and modules. It automates provisioning, configuration management, and application deployment. Ansible's "playbooks" written in YAML are easy to understand and maintain.
Example Playbook:
--- - name: Deploy Web App hosts: webservers tasks: - name: Clone Git Repository git: repo: https://github.com/example/web-app.git dest: /var/www/web-app
JenkinsAPI (๐ฆ): JenkinsAPI provides a Pythonic way to interact with the Jenkins Continuous Integration server. It allows you to create, configure, and manage Jenkins jobs programmatically, enabling better integration of Jenkins into your DevOps pipelines.
Example Code:
from jenkinsapi.jenkins import Jenkins jenkins = Jenkins('http://jenkins_server_url') job = jenkins.get_job('my_job') job.invoke(build_params={'param1': 'value1'})
Docker SDK (๐ณ): Docker has revolutionized containerization, and the Docker SDK for Python empowers DevOps engineers to interact with Docker containers programmatically. It provides an interface to manage containers, images, networks, and volumes, making it easier to automate container workflows.
Example Code:
import docker client = docker.from_env() container = client.containers.run('nginx', detach=True)
Paramiko (๐): Paramiko is a Python library that facilitates secure SSH connections and provides support for SFTP. It's an excellent choice for automating tasks that require secure communication with remote servers.
Example Code:
import paramiko ssh = paramiko.SSHClient() ssh.connect('server_host', username='user', password='password') stdin, stdout, stderr = ssh.exec_command('ls')
Stay tuned for Part 2, where we'll explore more Python libraries for DevOps and provide you with code snippets to simplify your DevOps workflows. Keep exploring, automating, and innovating with Python in your DevOps journey! ๐๐
Subscribe to my newsletter
Read articles from Ronil Rodrigues directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
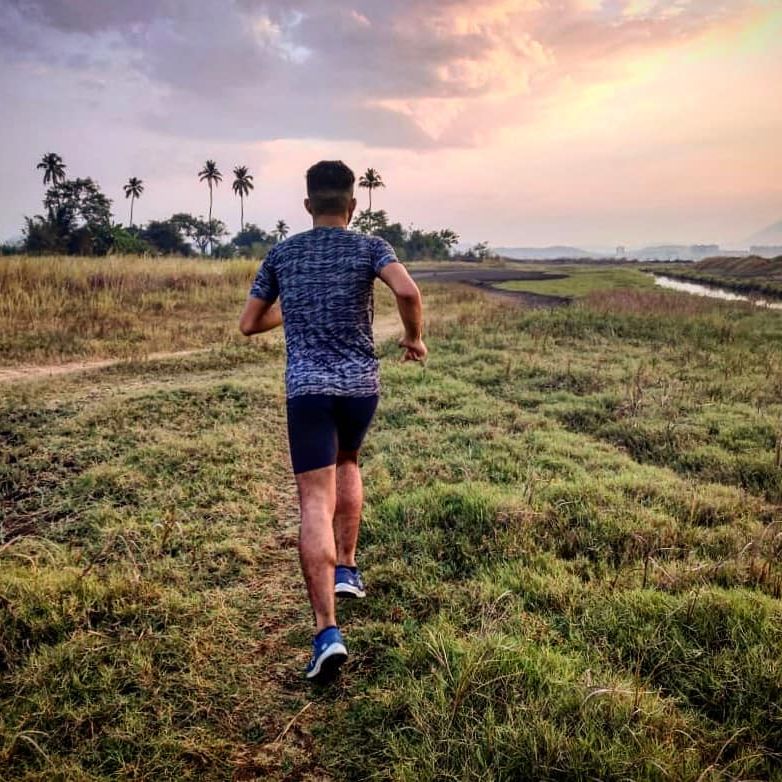
Ronil Rodrigues
Ronil Rodrigues
Cloud enthusiast who runs towards cloud!!