Exploring JavaScript Loops: for...of and forEach Demystified
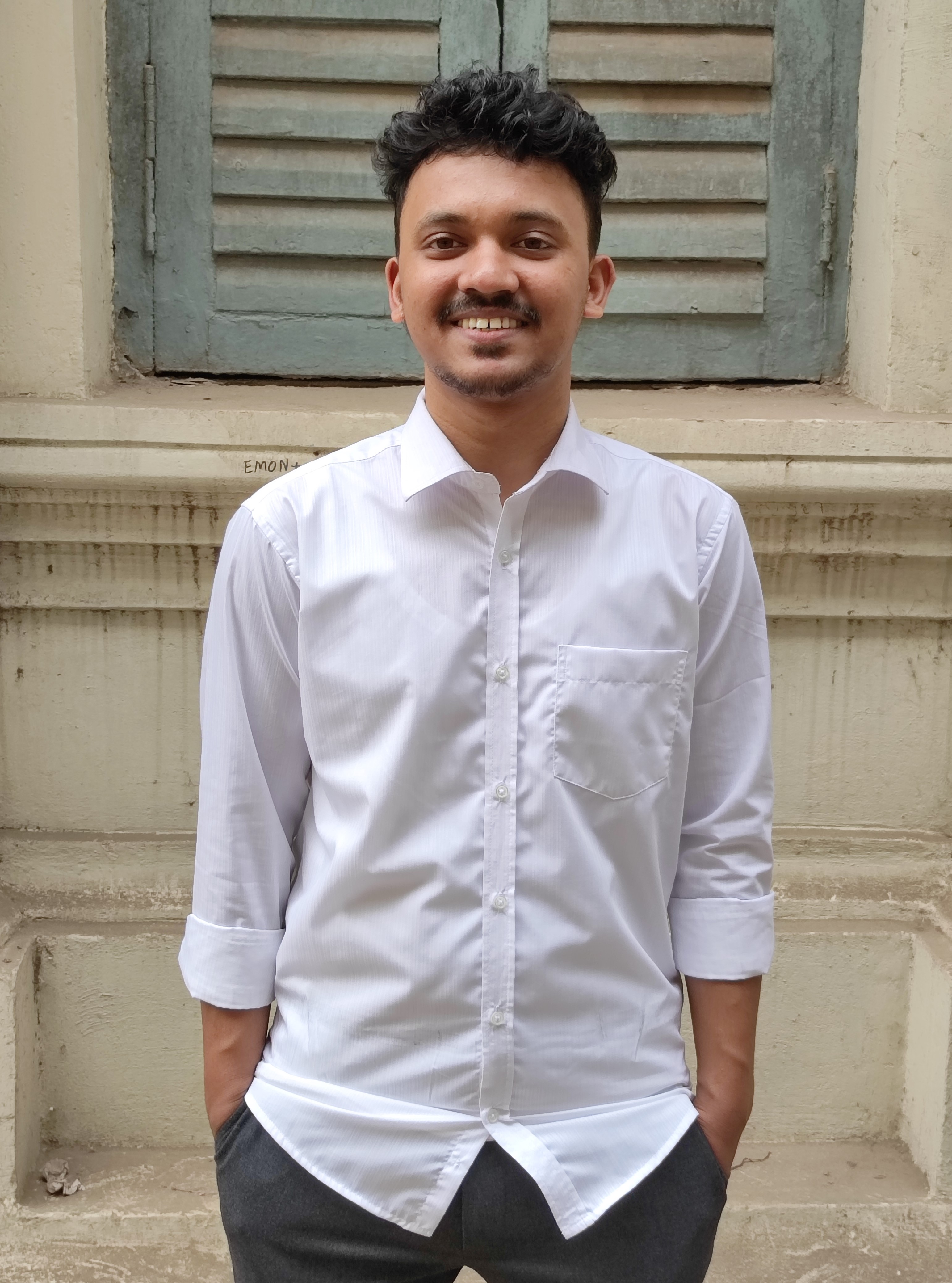
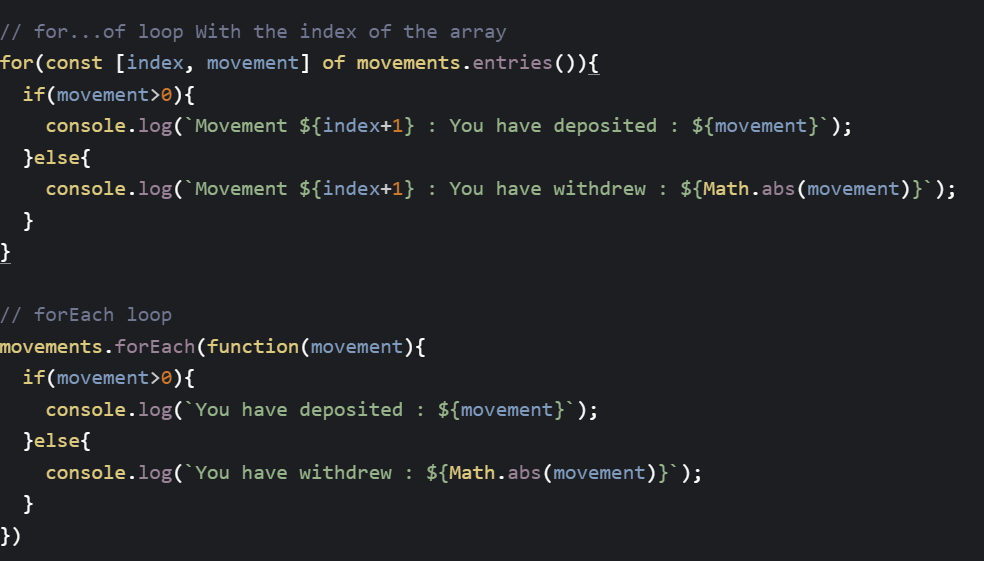
Introduction:
JavaScript, as a versatile programming language, offers several ways to iterate through arrays, and today we'll delve into two powerful looping mechanisms: for...of
and forEach
. These loops are essential tools in a developer's toolkit, enabling elegant and concise ways to process array elements. In this article, we'll explore both loops and provide insights into their working.
The Array: Tracking Financial Movements
To illustrate these loops, let's consider a scenario where you're tracking financial movements in an array named movements
.
const movements = [200, 450, -400, 3000, -650, -130, 70, 1300];
The for...of Loop:
The for...of
loop simplifies iterating over arrays by directly accessing the values within them. It's especially handy when you don't need to track the index explicitly.
for (const movement of movements) {
if (movement > 0) {
console.log(`You have deposited: ${movement}`);
} else {
console.log(`You have withdrawn: ${Math.abs(movement)}`);
}
}
The for...of Loop with Index:
If you need both the value and the index, the for...of
loop can be enhanced using the entries()
method.
for (const [index, movement] of movements.entries()) {
if (movement > 0) {
console.log(`Movement ${index + 1}: You have deposited: ${movement}`);
} else {
console.log(`Movement ${index + 1}: You have withdrawn: ${Math.abs(movement)}`);
}
}
The forEach Loop:
The forEach
loop is a built-in array method that executes a provided function once for each array element.
movements.forEach(function (movement) {
if (movement > 0) {
console.log(`You have deposited: ${movement}`);
} else {
console.log(`You have withdrawn: ${Math.abs(movement)}`);
}
});
The forEach Loop with Index:
You can also use forEach
with three parameters: the current value, the index, and the entire array.
movements.forEach(function (movement, index, arr) {
if (movement > 0) {
console.log(`Movement ${index + 1}: You have deposited: ${movement}`);
} else {
console.log(`Movement ${index + 1}: You have withdrawn: ${Math.abs(movement)}`);
}
});
Understanding the Loops:
Both for...of
and forEach
loops offer concise ways to iterate through arrays, but they have some differences in terms of use cases and functionalities.
for...of
is great for simple iterations where you don't need to track the index.forEach
provides more flexibility, especially when you need to perform a specific action for each element.
Connect with Me:
LinkedIn: linkedin.com/in/farhanishtiyak
GitHub: github.com/farhanishtiyak
Hashnode: hashnode.com/@farhanishtiyak
Twitter: twitter.com/FarhanIshtiyak
Conclusion:
Mastering looping mechanisms is crucial for any JavaScript developer. The for...of
and forEach
loops simplify array iteration and empower you to manipulate data efficiently. By understanding how these loops work, you'll be better equipped to tackle various challenges in your coding journey. Happy coding! ๐
Subscribe to my newsletter
Read articles from Farhan Ishtiyak directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
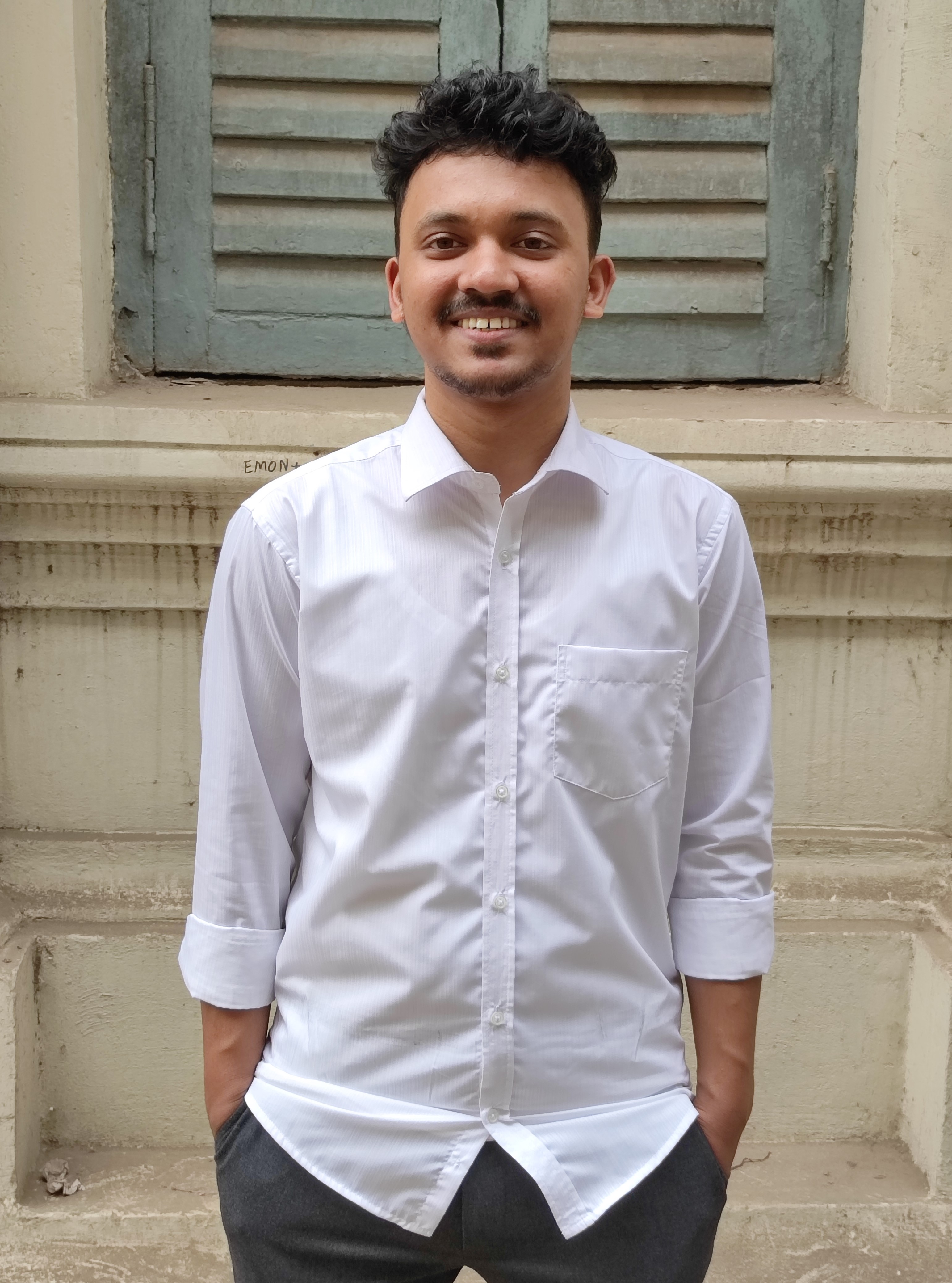
Farhan Ishtiyak
Farhan Ishtiyak
๐ Hello, World! I'm Farhan Ishtiyak, a passionate and self-taught computer science and engineering student hailing from Jagannath University, Dhaka, Bangladesh. As an avid learner and enthusiast in the world of technology, I constantly seek to expand my horizons and challenge myself to reach new heights. ๐ Education: I am currently pursuing my degree in Computer Science and Engineering, where I have been exposed to a wide range of subjects, from algorithms and data structures to software engineering and artificial intelligence. My academic journey has honed my problem-solving skills and nurtured my curiosity for exploring cutting-edge technologies. ๐ป Competitive Programming: One of my true passions lies in the realm of competitive programming. Solving complex algorithmic problems and optimizing solutions is like solving puzzles that keep my mind sharp and creative. Participating in coding competitions has not only refined my coding abilities but has also instilled in me the value of perseverance and teamwork. ๐ Web Development: Alongside competitive programming, I am deeply intrigued by web development. The power of creating interactive and user-friendly websites and applications excites me. I have dabbled in various web technologies, such as HTML, CSS, JavaScript, and frameworks like React and Node.js, to build projects that showcase my abilities and passion for coding. ๐ Building Global Connections: Beyond the realms of academics and coding, I believe in the power of connecting with like-minded individuals across the globe. I am a firm believer in the spirit of community and continuous learning. Through various online learning platforms, I aim to engage with people from diverse backgrounds, share knowledge, and collaborate on innovative projects that make a positive impact on the world. ๐ Learning Never Stops: In the vast ocean of technology, I understand that there is always more to learn. Embracing the ever-evolving nature of the field, I am committed to staying updated with the latest trends and developments. I love exploring new frameworks, attending tech conferences, and being part of coding communities to exchange ideas and stay at the forefront of innovation. ๐ญ Looking Ahead: As I journey through the world of computer science and engineering, I aspire to contribute to open-source projects, create meaningful applications, and use my skills to solve real-world challenges. I am open to exciting opportunities and collaborations that allow me to grow both professionally and personally. ๐ซ Get in Touch: I'm always eager to connect with fellow learners, developers, and tech enthusiasts worldwide. Feel free to reach out to me via email or connect with me on various social platforms. Let's inspire and learn together on this incredible technological journey!