Simplify Form Handling with React Hook Form: A Comprehensive Guide

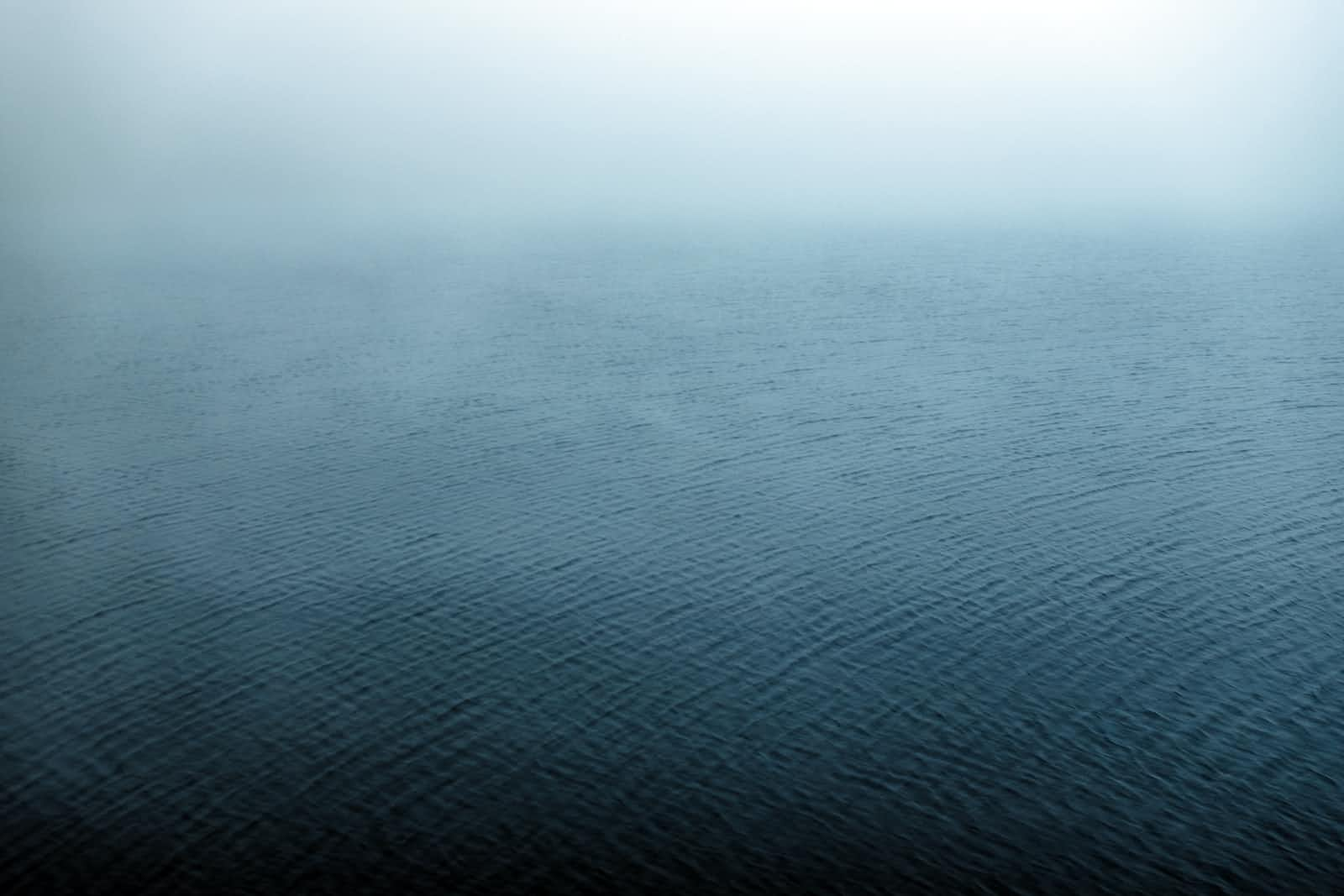
When it comes to crafting interactive web applications, forms play a pivotal role in gathering user data. However, managing form state, validation, and submission can often be intricate and time-consuming. Enter React Hook Form – an invaluable library designed to streamline form management in React applications, making the entire process efficient and hassle-free.
Introduction to React Hook Form
Simplifying form handling has never been easier, thanks to React Hook Form. This powerful library offers a straightforward and effective approach to managing forms, from simple data collection to complex multi-step processes. By implementing React Hook Form, developers can ensure seamless form interactions without the typical headaches associated with form development.
Getting Started with React Hook Form
To embark on your journey with React Hook Form, it's essential to install the library in your project. Utilize the following command:
npm install react-hook-form
This installation lays the foundation for intuitive form management in your React application.
A Primer on Basic Usage
Let's dive into a succinct example of how React Hook Form transforms form creation:
import React from 'react';
import { useForm } from 'react-hook-form';
function MyForm() {
const { register, handleSubmit } = useForm();
const onSubmit = (data) => {
console.log(data); // Capturing and logging form data
};
return (
<form onSubmit={handleSubmit(onSubmit)}>
<input {...register('firstName')} name="firstName" placeholder="First Name" />
<input {...register('lastName')} name="lastName" placeholder="Last Name" />
<button type="submit">Submit</button>
</form>
);
}
export default MyForm;
In this example, the register
function associates input fields with the form state, while handleSubmit
managing form submissions. The captured data is then logged for further processing.
Streamlined Validation with React Hook Form
The library greatly simplifies form validation, making it effortless to implement rules using the register
function. Consider this email validation example:
import React from 'react';
import { useForm } from 'react-hook-form';
function MyForm() {
const { register, handleSubmit, formState: { errors } } = useForm();
const onSubmit = (data) => {
console.log(data);
};
return (
<form onSubmit={handleSubmit(onSubmit)}>
<input {...register('email', { required: 'Email is required'} name="email" placeholder="Email" />
{errors.email && <p>{errors.email.message}</p>}
<button type="submit">Submit</button>
</form>
);
}
export default MyForm;
By utilizing the pattern
option, email inputs are validated against a specific format, and error messages are displayed as needed.
Conclusion: Elevate Your Form Handling with React Hook Form
React Hook Form stands as a pivotal tool in simplifying form handling within React applications. With its user-friendly API and built-in validation capabilities, it empowers developers to focus on crafting exceptional user experiences, unburdened by intricate form intricacies. Whether you're creating a basic contact form or a multifaceted data entry system, React Hook Form streamlines the process and saves valuable development time.
This blog post has showcased React Hook Form's prowess through clear examples and guidance. As you delve into its integration within your projects, remember that the official documentation is your comprehensive resource for advanced usage and deeper insights.
Embrace the world of hassle-free form handling by implementing React Hook Form and enjoying the advantages of streamlined development. Happy coding!
Subscribe to my newsletter
Read articles from Yaseer Tasleem directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Yaseer Tasleem
Yaseer Tasleem
Front-End Developer