Exploring Dynamic Carousels and Dropdowns in HTML and JavaScript
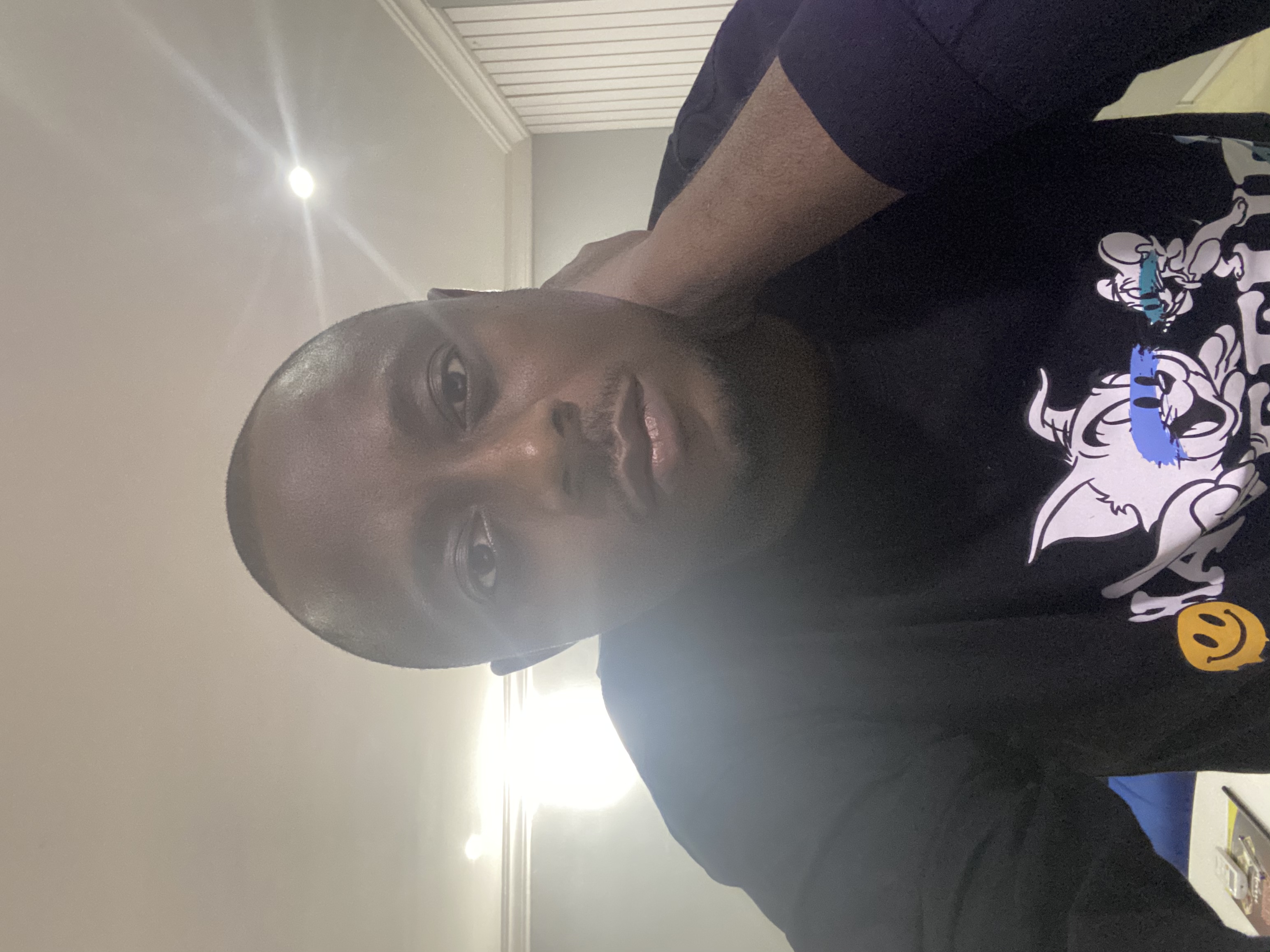
Table of contents
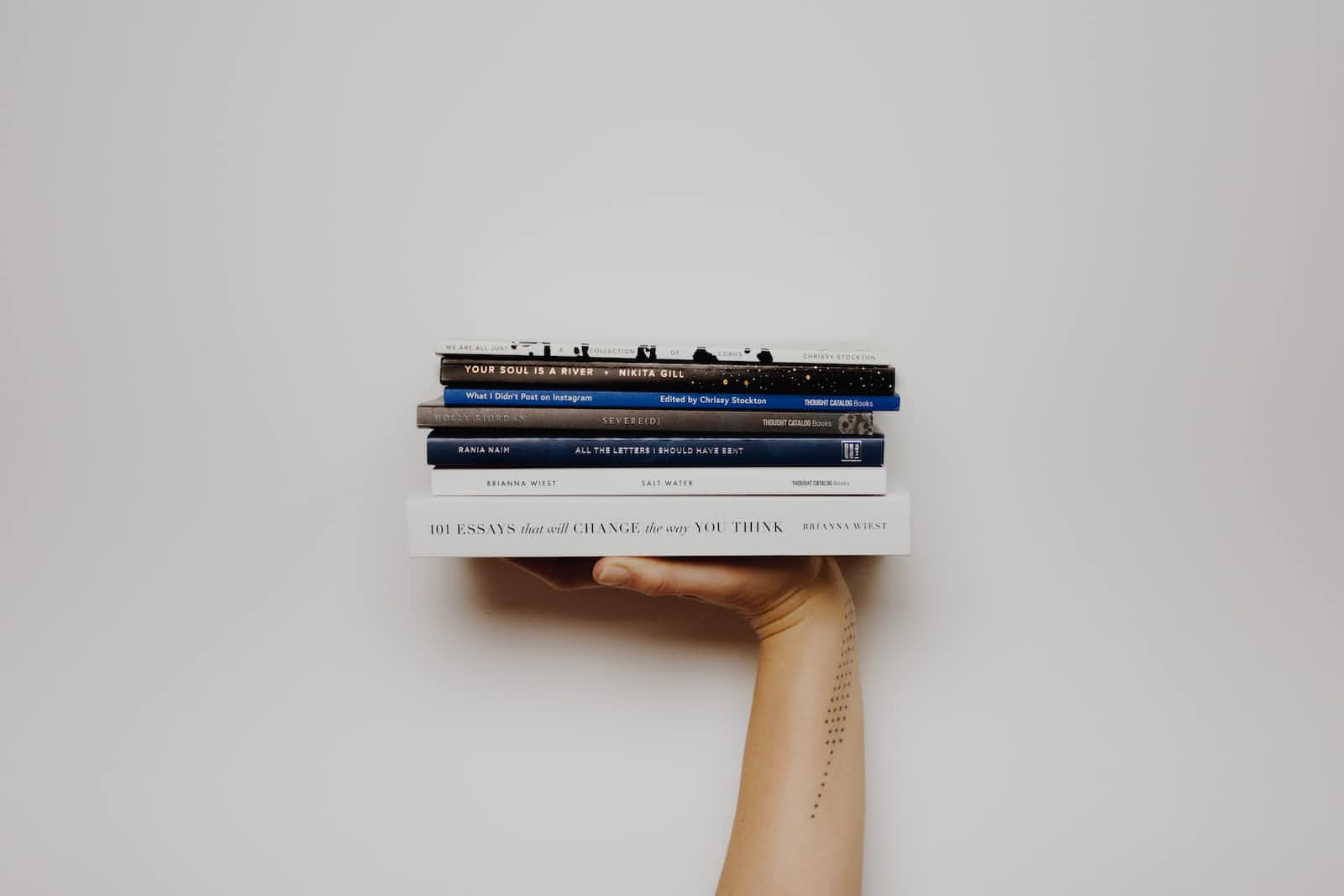
Introduction:
In today's rapidly evolving web development landscape, creating engaging and interactive user interfaces has become a key aspect of building successful websites. In this blog post, we're going to explore how to implement dynamic content carousels and responsive dropdown menus using HTML, CSS, and JavaScript. We'll provide in-depth code samples and explanations to guide you through the process of adding these captivating features to your web projects.
Table of Contents:
Understanding the Basics:
Exploring the importance of dynamic UI elements
A brief overview of HTML, CSS, and JavaScript roles
Building a Content Carousel:
Creating the HTML Structure: Let's start by structuring the HTML for our carousel. We'll use a container to hold the carousel items and indicators for navigation.
<div class="carousel-container"> <div class="carousel-content"> <!-- Content items go here --> </div> <div class="carousel-indicators"> <!-- Indicators go here --> </div> </div>
Styling the Carousel: Next, we'll style the carousel to create a visually appealing display. We'll ensure that the content items are stacked horizontally and aligned properly.
.carousel-container { position: relative; overflow: hidden; /* Additional styling properties */ } .carousel-content { display: flex; transition: transform 0.5s ease-in-out; /* Additional styling properties */ }
Implementing Auto-Scrolling: To automatically scroll through the content items, we'll use JavaScript to update the carousel's position at regular intervals.
const carouselContent = document.querySelector('.carousel-content'); let currentIndex = 0; function autoScroll() { currentIndex = (currentIndex + 1) % totalItems; const translateValue = -currentIndex * itemWidth; carouselContent.style.transform = `translateX(${translateValue}px)`; } setInterval(autoScroll, 3000); // Auto scroll every 3 seconds
Adding Interactive Indicators: Indicators provide users with navigation options. We'll use JavaScript to highlight the active indicator when a content item is displayed.
const indicators = document.querySelectorAll('.carousel-indicators div'); indicators.forEach((indicator, index) => { indicator.addEventListener('click', () => { currentIndex = index; const translateValue = -currentIndex * itemWidth; carouselContent.style.transform = `translateX(${translateValue}px)`; updateIndicators(); }); });
Bringing it All Together: By combining HTML, CSS, and JavaScript, you can create a fully functional content carousel that automatically scrolls and responds to user interactions.
Crafting a Responsive Dropdown Menu:
The Dropdown Concept: Understand the concept of dropdown menus and their role in enhancing user experience.
The HTML and CSS Foundation: Begin by constructing the HTML structure for the dropdown menu and applying basic styles for aesthetics.
<div class="dropdown"> <button class="dropdown-toggle">Select an Option</button> <div class="dropdown-content"> <!-- Dropdown options go here --> </div> </div>
.dropdown { position: relative; display: inline-block; /* Additional styling properties */ } .dropdown-content { display: none; position: absolute; /* Additional styling properties */ }
Creating the JavaScript Logic: Utilize JavaScript to toggle the visibility of the dropdown content when the user clicks the toggle button.
const dropdownToggle = document.querySelector('.dropdown-toggle'); const dropdownContent = document.querySelector('.dropdown-content'); dropdownToggle.addEventListener('click', () => { dropdownContent.style.display = dropdownContent.style.display === 'none' ? 'block' : 'none'; });
Managing Dropdown Behavior: Implement additional JavaScript logic to close the dropdown when users click outside of it.
document.addEventListener('click', (event) => { if (!dropdown.contains(event.target)) { dropdownContent.style.display = 'none'; } });
Enhancing User Experience: Elevate the user experience by adding icons and subtle animations to the dropdown menu.
Conclusion/Complete code with addons:
Recap the process of implementing dynamic content carousels and responsive dropdown menus.
Emphasize the impact of these features on user engagement and interaction.
Encourage readers to experiment with the provided code samples and incorporate these techniques into their projects.
<!DOCTYPE html>
<html>
<head>
<title>Styled Header and Content</title>
<link href="https://fonts.googleapis.com/icon?family=Material+Icons" rel="stylesheet">
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/4.7.0/css/font-awesome.min.css">
<style>
body {
margin: 0;
padding: 0;
font-family: Arial, sans-serif;
}
header {
background-color: #333;
color: white;
padding: 14px;
text-align: center;
position: sticky;
top: 0;
z-index: 100;
/* Ensure header stays on top of content */
}
h1 {
margin: 0;
font-size: 36px;
}
nav {
padding: 0;
display: flex;
justify-content: space-between;
margin-top: 0px;
}
.logo {
display: flex;
justify-content: center;
align-items: center;
}
.rightme {
display: flex;
flex-direction: row;
justify-content: space-between;
gap: 15px;
}
.butt {
padding: 10px;
border-radius: 5px;
}
.texty {
border: 1px solid black;
}
.footers {
background-color: #333;
color: white;
position: fixed;
bottom: 0;
z-index: 999;
/* Ensure header stays on top of content */
width: 100%;
height: 74px;
}
.goat {
display: flex;
flex-direction: row;
justify-content: space-between;
padding: 20px;
}
.bottomflex {
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
gap: 5px;
font-size: 14px;
}
.content {
padding: 5px;
text-align: center;
margin-top: 5px;
/* Add margin to the top of content to allow space for the sticky header */
margin-bottom: 100px;
/* Add margin to the bottom of content */
overflow-y: auto;
/* Add vertical scroll if content overflows */
}
#brownmenu {
position: fixed;
bottom: 74px;
left: 0;
width: 55%;
height: 100%;
background-color: #333;
display: flex;
flex-direction: column;
z-index: 999;
/* border-top-left-radius: 10px;
border-top-right-radius: 10px; */
gap: 10px;
}
.gugu {
display: none;
z-index: 999;
}
.whiter {
color: white;
}
.linker {
text-decoration: none;
cursor: pointer;
}
/* Style for the overlay */
.overlay {
display: none;
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
background-color: rgba(0, 0, 0, 0.6);
z-index: 998;
}
.type1 {
height: 60px;
border: 0px solid red;
display: flex;
flex-direction: row;
justify-content: space-between;
align-items: center;
gap: 5px;
}
.widthme1 {
/* width: 35px; */
/* height: 100%; */
border: 0px solid green;
display: flex;
align-items: center;
justify-content: center;
font-size: 26px;
overflow: hidden;
/* Hide horizontal overflow */
cursor: pointer;
padding: 10px;
}
.widthme3 {
/* width: 35px; */
/* height: 100%; */
border: 0px solid pink;
display: flex;
align-items: center;
justify-content: center;
font-size: 26px;
overflow: hidden;
/* Hide horizontal overflow */
cursor: pointer;
padding: 10px;
}
.widthme2 {
border: 0px solid black;
display: flex;
overflow-x: auto;
/* Enable horizontal scrolling */
scroll-behavior: smooth;
/* Smooth scrolling behavior */
flex-direction: row;
align-items: center;
/* width: auto; */
padding: 10px;
gap: 10px;
white-space: nowrap;
}
.boxy1 {
display: flex;
flex-direction: row;
justify-content: center;
align-items: center;
height: 20px;
border: 1px solid blue;
gap: 5px;
padding: 10px;
font-size: 16px;
border-radius: 5px;
white-space: nowrap;
}
.type2 {
/* position: relative; */
height: 180px;
border: 0px solid red;
display: flex;
overflow: hidden; /* Hide overflow */
scroll-behavior: smooth;
flex-direction: row;
align-items: center;
gap: 10px;
white-space: nowrap;
width: 100%;
}
.mbah {
border: 1px solid blue;
height: 100%;
width: 300px;
border-radius: 10px;
flex: 0 0 auto;
}
.indicators {
display: flex;
justify-content: center;
/* position: absolute; */
/* bottom: 10px;
left: 0; */
width: 100%;
margin-top: 30px;
margin-bottom: 30px;
}
.indicator {
width: 10px;
height: 10px;
border-radius: 50%;
background-color: gray;
margin: 0 5px;
cursor: pointer;
}
.indicator.active {
background-color: black;
}
.type3 {
border: 0px solid red;
display: flex;
flex-direction: row;
justify-content: space-between;
align-items: center;
gap: 5px;
}
.boxy12 {
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
height: 80px; /* Set the desired height */
width: 80px; /* Set the desired width */
border: 1px solid blue;
padding: 10px;
border-radius: 5px;
flex: 0 0 auto;
}
.widthme1a {
/* width: 35px; */
/* height: 100%; */
border: 0px solid green;
display: flex;
align-items: center;
justify-content: center;
font-size: 26px;
overflow: hidden;
/* Hide horizontal overflow */
cursor: pointer;
padding: 10px;
}
.widthme3a {
/* width: 35px; */
/* height: 100%; */
border: 0px solid pink;
display: flex;
align-items: center;
justify-content: center;
font-size: 26px;
overflow: hidden;
/* Hide horizontal overflow */
cursor: pointer;
padding: 10px;
}
.widthme2a {
border: 0px solid black;
display: flex;
overflow-x: auto;
/* Enable horizontal scrolling */
scroll-behavior: smooth;
/* Smooth scrolling behavior */
flex-direction: row;
align-items: center;
/* width: auto; */
padding: 10px;
gap: 10px;
white-space: nowrap;
}
.widthme2a .boxy12 i {
font-size: 28px; /* Adjust the icon size as needed */
}
.boxy12 > div {
text-align: center;
white-space: normal; /* Allow text to wrap to the next line */
max-width: 100%; /* Allow the content to expand to the available width */
}
/*
.dropme{
height: 30px;
width: 130px;
border: 1px solid red;
border-radius: 5px;
display: flex;
flex-direction: row;
justify-content: space-between;
align-items: center;
padding: 0 20px;
}
.dropme i {
font-size: 24px;
} */
.dropme {
position: relative;
/* display: inline-block; */
cursor: pointer;
}
.dropme-toggle {
height: 30px;
width: 130px;
border: 1px solid red;
border-radius: 5px;
display: flex;
flex-direction: row;
justify-content: space-between;
align-items: center;
padding: 0 20px;
}
.dropme i {
font-size: 24px; /* Adjust the icon size as needed */
}
.dropdown-content {
display: none;
position: absolute;
background-color: white;
border: 1px solid gray;
border-radius: 5px;
min-width: 130px;
z-index: 1;
margin-top: 10px; /* Add margin to create space between dropme-toggle and dropdown-content */
padding: 10px; /* Add padding for better appearance */
}
.dropme:hover .dropdown-content {
display: block;
}
.dropdown-item {
padding: 10px 15px;
cursor: pointer;
}
.dropdown-item:hover {
background-color: #f1f1f1;
}
</style>
</head>
<body>
<header>
<nav>
<div class="logo">
<div>Logo</div>
</div>
<div class="rightme">
<div><button class="butt"><i class="fa fa-credit-card"></i></button></div>
<div><button class="butt">Login </button></div>
<div><button class="butt">Register </button></div>
</div>
</nav>
</header>
<div class="content">
<div class="type1">
<div class="widthme1" onclick="scrollss()">
<i class="fa fa-angle-left"></i>
</div>
<div class="widthme2" id="scrollContainer">
<div class="boxy1">
<div><i class="fa fa-home"></i></div>
<div>Home</div>
</div>
<div class="boxy1">
<div><i class="fa fa-video-camera"></i></div>
<div>Live Events</div>
</div>
<div class="boxy1">
<div><i class="fa fa-history"></i></div>
<div>Upcoming</div>
</div>
<div class="boxy1">
<div><i class="fa fa-trophy"></i></div>
<div>Outrights</div>
</div>
<div class="boxy1">
<div><i class="fa fa-gear"></i></div>
<div>Odds Format - Decimal</div>
</div>
<div class="boxy1">
<div><i class="fa fa-home"></i></div>
<div>Home</div>
</div>
<div class="boxy1">
<div><i class="fa fa-video-camera"></i></div>
<div>Live Events</div>
</div>
<div class="boxy1">
<div><i class="fa fa-history"></i></div>
<div>Upcoming</div>
</div>
<div class="boxy1">
<div><i class="fa fa-trophy"></i></div>
<div>Outrights</div>
</div>
<div class="boxy1">
<div><i class="fa fa-gear"></i></div>
<div>Odds Format - Decimal</div>
</div>
</div>
<div class="widthme3" onclick="scrollRight()">
<i class="fa fa-angle-right"></i>
</div>
</div>
<div class="type2" id="carousel">
<div class="mbah"></div>
<div class="mbah"></div>
<div class="mbah"></div>
<div class="mbah"></div>
<div class="mbah"></div>
</div>
<div class="indicators" id="indicators"></div>
<div class="type3">
<div class="widthme1a" onclick="scrollssa()">
<i class="fa fa-angle-left"></i>
</div>
<div class="widthme2a" id="scrollContainera">
<div class="boxy12">
<div><i class="fa fa-home"></i></div>
<div>Live Stream</div>
</div>
<div class="boxy12">
<div><i class="fa fa-video-camera"></i></div>
<div>Football</div>
</div>
<div class="boxy12">
<div><i class="fa fa-history"></i></div>
<div>Basketball</div>
</div>
<div class="boxy12">
<div><i class="fa fa-trophy"></i></div>
<div>Tennis</div>
</div>
<div class="boxy12">
<div><i class="fa fa-gear"></i></div>
<div>MMA</div>
</div>
<div class="boxy12">
<div><i class="fa fa-home"></i></div>
<div>American Football</div>
</div>
<div class="boxy12">
<div><i class="fa fa-video-camera"></i></div>
<div>Baseball</div>
</div>
<div class="boxy12">
<div><i class="fa fa-history"></i></div>
<div>Ice Hockey</div>
</div>
<div class="boxy12">
<div><i class="fa fa-trophy"></i></div>
<div>Table Tennis</div>
</div>
<div class="boxy12">
<div><i class="fa fa-gear"></i></div>
<div>League of Legends</div>
</div>
</div>
<div class="widthme3a" onclick="scrollRighta()">
<i class="fa fa-angle-right"></i>
</div>
</div>
<hr>
<!-- <div class="dropme">
<i class="fa fa-filter"></i>
<span>Next Hour</span>
<i class="fa fa-angle-down"></i>
</div> -->
<div class="dropme" onclick="toggleDropdown()">
<div class="dropme-toggle">
<i class="fa fa-filter"></i> <!-- Add the class "fa-toggle" here -->
<span id="selectedItem">Next Hour</span>
<i class="fa fa-angle-down fa-toggle"></i>
</div>
<div class="dropdown-content" id="dropdownContent">
<div class="dropdown-item" onclick="selectDropdownItem('Next Hour')">Next Hour</div>
<div class="dropdown-item" onclick="selectDropdownItem('Today')">Today</div>
<div class="dropdown-item" onclick="selectDropdownItem('Tomorrow')">Tomorrow</div>
<div class="dropdown-item" onclick="selectDropdownItem('Next Week')">Next Week</div>
<div class="dropdown-item" onclick="selectDropdownItem('Custom')">Custom</div>
<div class="dropdown-item" onclick="selectDropdownItem('All')">All</div>
</div>
</div>
<!-- Your content goes here -->
<!-- ... -->
</div>
<div class="footers">
<div class="goat">
<a class="bottomflex whiter linker mack" id="button1" onclick="menuclick()">
<span><i class="fa fa-align-justify" style="font-size:16px"></i></span>
<span> Menu </span>
</a>
<a class="bottomflex">
<span><i class="fa fa-bookmark" style="font-size:16px"></i></span>
<span> MyBets </span>
</a>
<a class="bottomflex">
<span><i class="fa fa-gamepad" style="font-size:16px"></i></span>
<span> BetSlip </span>
</a>
<a class="bottomflex">
<span><i class="fa fa-lastfm-square" style="font-size:16px"></i></span>
<span> Casino </span>
</a>
<a class="bottomflex">
<span><i class="fa fa-wechat" style="font-size:16px"></i></span>
<span> Chat </span>
</a>
</div>
</div>
<!-- Overlay element -->
<div class="overlay" id="overlay"></div>
<div class="gugu">
<div id="brownmenu">
</div>
</div>
<script>
function menuclick() {
const gugu = document.querySelector(".gugu");
const overlay = document.querySelector(".overlay"); // Get the overlay element
const body = document.body;
if (gugu.style.display === "" || gugu.style.display === "none") {
gugu.style.display = "block";
overlay.style.display = "block"; // Show the overlay
body.style.overflow = "hidden";
} else {
gugu.style.display = "none";
overlay.style.display = "none"; // Hide the overlay
body.style.overflow = "auto";
}
}
// Add click event listener to the document
document.addEventListener("click", function (event) {
const gugu = document.querySelector(".gugu");
const mackButton = document.querySelector(".mack");
const overlay = document.querySelector(".overlay"); // Get the overlay element
// Check if the click target is not within gugu or mackButton
if (!gugu.contains(event.target) && !mackButton.contains(event.target)) {
gugu.style.display = "none";
document.body.style.overflow = "auto";
overlay.style.display = "none"; // Hide the overlay
}
});
function scrollss() {
scrollContainer.scrollLeft -= 20; // Adjust the scrolling amount as needed
}
function scrollRight() {
scrollContainer.scrollLeft += 20; // Adjust the scrolling amount as needed
}
// New code to handle hiding/showing navigation arrows
const scrollContainer = document.getElementById("scrollContainer");
const widthme1 = document.querySelector(".widthme1");
const widthme3 = document.querySelector(".widthme3");
widthme1.style.display = "none";
scrollContainer.addEventListener("scroll", function () {
const atStart = scrollContainer.scrollLeft === 0;
const contentWidth = scrollContainer.scrollWidth;
const containerWidth = scrollContainer.clientWidth;
const atEnd = scrollContainer.scrollLeft + containerWidth >= contentWidth;
widthme1.style.display = atStart ? "none" : "block";
widthme3.style.display = atEnd ? "none" : "block";
});
//second carousel
// Replicate scrolling functionality for type3
function scrollssa() {
scrollContainera.scrollLeft -= 20; // Adjust the scrolling amount as needed
}
function scrollRighta() {
scrollContainera.scrollLeft += 20; // Adjust the scrolling amount as needed
}
const scrollContainera = document.getElementById("scrollContainera");
const widthme1a = document.querySelector(".widthme1a");
const widthme3a = document.querySelector(".widthme3a");
widthme1a.style.display = "none";
scrollContainera.addEventListener("scroll", function () {
const atStart = scrollContainera.scrollLeft === 0;
const contentWidth = scrollContainera.scrollWidth;
const containerWidth = scrollContainera.clientWidth;
const atEnd = scrollContainera.scrollLeft + containerWidth >= contentWidth;
widthme1a.style.display = atStart ? "none" : "block";
widthme3a.style.display = atEnd ? "none" : "block";
});
</script>
<script>
const carousel = document.getElementById("carousel");
const indicatorsContainer = document.getElementById("indicators");
const mbahItems = document.querySelectorAll(".mbah");
const indicatorCount = mbahItems.length;
let currentSlide = 0;
function updateIndicators() {
const indicators = indicatorsContainer.querySelectorAll(".indicator");
indicators.forEach((indicator, index) => {
if (index === currentSlide) {
indicator.classList.add("active");
} else {
indicator.classList.remove("active");
}
});
}
function scrollToSlide(slideIndex) {
const scrollPosition = slideIndex * (mbahItems[0].offsetWidth + 10); // Include gap
carousel.scroll({
left: scrollPosition,
behavior: "smooth"
});
currentSlide = slideIndex;
updateIndicators();
}
function autoScroll() {
currentSlide = (currentSlide + 1) % indicatorCount;
scrollToSlide(currentSlide);
}
// Create indicators
for (let i = 0; i < indicatorCount; i++) {
const indicator = document.createElement("div");
indicator.className = "indicator";
indicator.addEventListener("click", () => {
scrollToSlide(i);
});
indicatorsContainer.appendChild(indicator);
}
updateIndicators();
setInterval(autoScroll, 3000); // Auto scroll every 3 seconds
</script>
<script>
// Hide dropdown content when the page loads
const dropdownContent = document.getElementById("dropdownContent");
const dropdownToggle = document.querySelector(".dropme i.fa-toggle");
dropdownContent.style.display = "none";
function toggleDropdown() {
if (dropdownContent.style.display === "block") {
dropdownContent.style.display = "none";
dropdownToggle.classList.remove("fa-angle-up");
dropdownToggle.classList.add("fa-angle-down");
} else {
dropdownContent.style.display = "block";
dropdownToggle.classList.remove("fa-angle-down");
dropdownToggle.classList.add("fa-angle-up");
}
}
function selectDropdownItem(item) {
const selectedItem = document.getElementById("selectedItem");
selectedItem.textContent = item;
toggleDropdown();
}
document.addEventListener("click", function(event) {
const dropme = document.querySelector(".dropme");
if (!dropme.contains(event.target) && !dropdownContent.contains(event.target)) {
dropdownContent.style.display = "none";
dropdownToggle.classList.remove("fa-angle-up");
dropdownToggle.classList.add("fa-angle-down");
}
});
</script>
</body>
</html>
In Closing:
By following the detailed code samples and explanations in this blog post, you now have the tools and knowledge to create engaging content carousels and user-friendly dropdown menus. These elements can significantly enhance the interactivity and usability of your website, providing a more enjoyable experience for your visitors. As you continue your journey in web development, consider exploring other dynamic UI elements to further elevate your web projects.
Subscribe to my newsletter
Read articles from Ogunuyo Ogheneruemu B directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
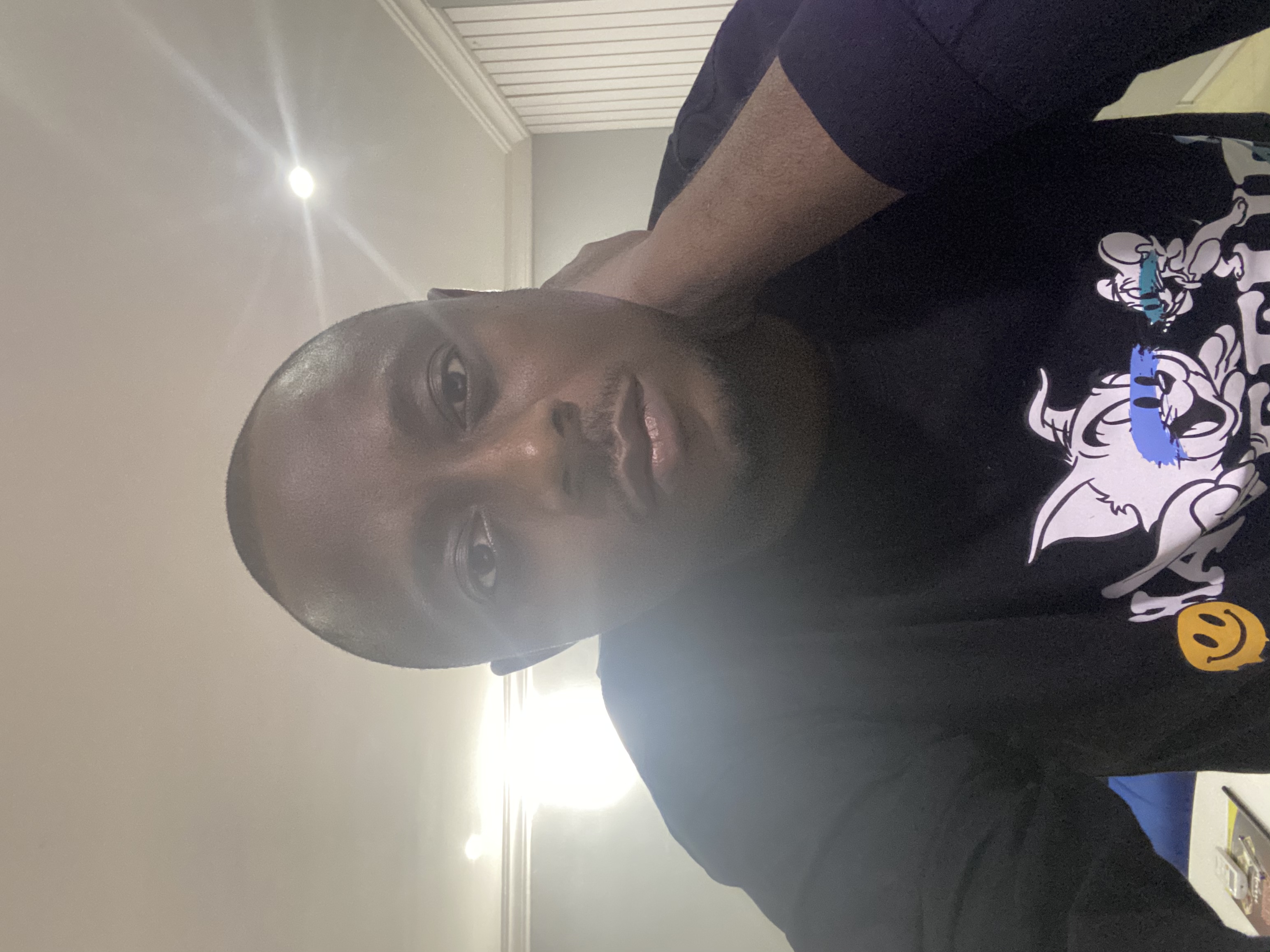
Ogunuyo Ogheneruemu B
Ogunuyo Ogheneruemu B
I'm Ogunuyo Ogheneruemu Brown, a senior software developer. I specialize in DApp apps, fintech solutions, nursing web apps, fitness platforms, and e-commerce systems. Throughout my career, I've delivered successful projects, showcasing strong technical skills and problem-solving abilities. I create secure and user-friendly fintech innovations. Outside work, I enjoy coding, swimming, and playing football. I'm an avid reader and fitness enthusiast. Music inspires me. I'm committed to continuous growth and creating impactful software solutions. Let's connect and collaborate to make a lasting impact in software development.