Event Bubbling and Controlling Propagation in JavaScript
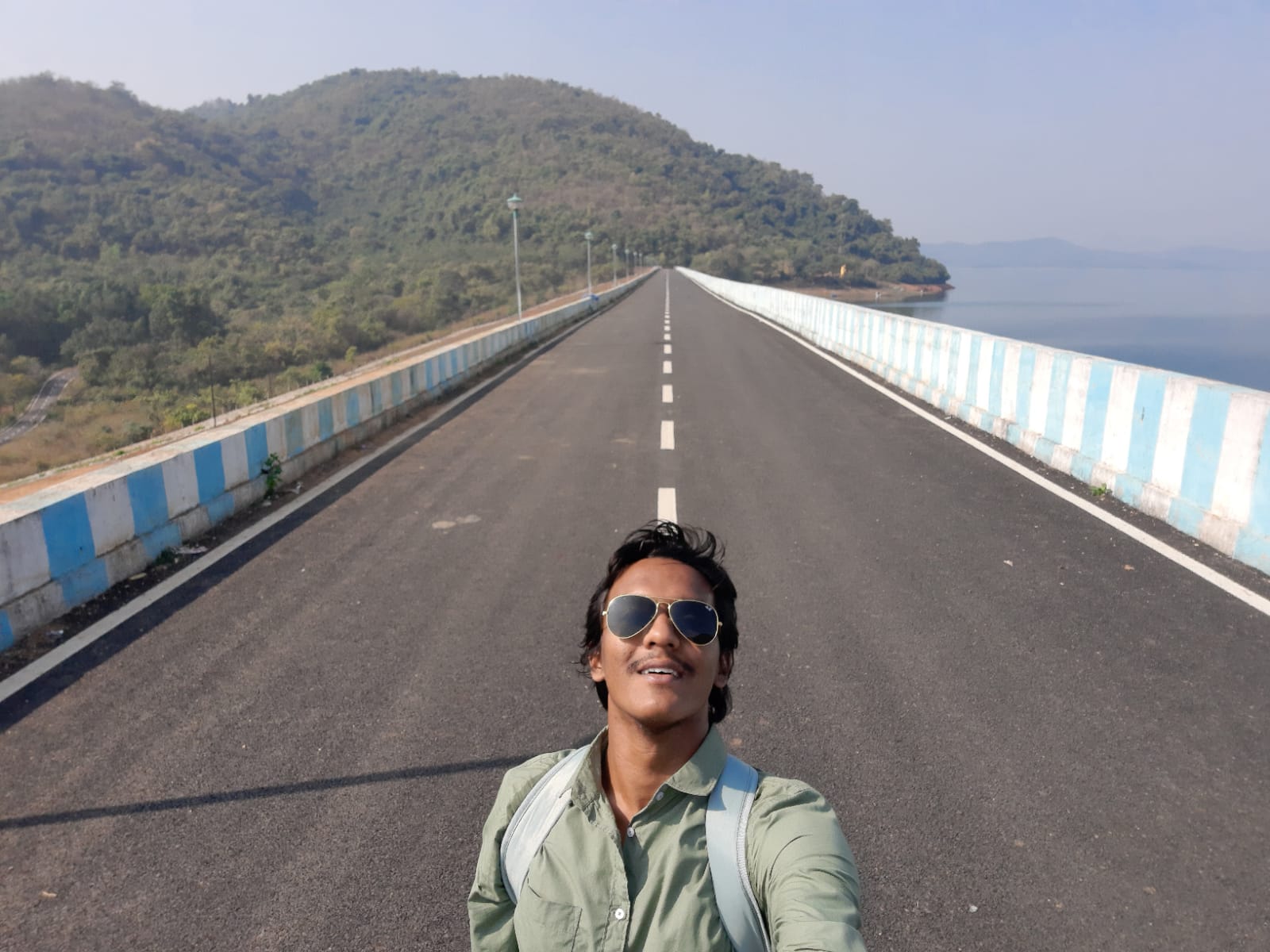
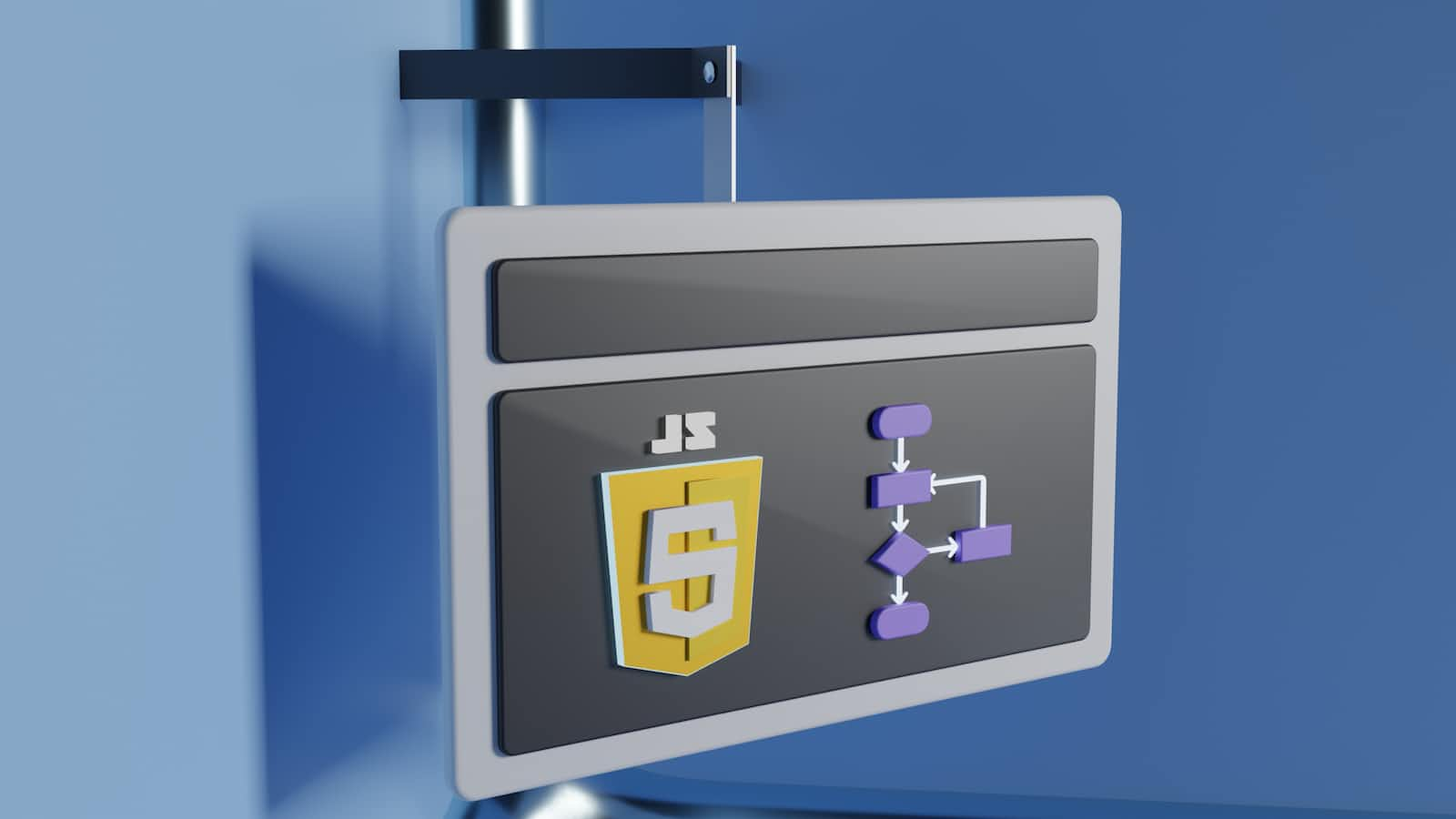
Introduction
Event handling is a fundamental aspect of modern web development, enabling dynamic and interactive user experiences. At the heart of event handling lies the concept of "event bubbling," a critical mechanism that dictates how events traverse the Document Object Model (DOM). In this article, we'll embark on a journey to thoroughly understand event bubbling, explore its significance, and delve into various methods for effectively controlling event propagation using JavaScript.
1. Understanding Event Bubbling:
Event bubbling is a foundational behaviour within the DOM where an event triggered on a nested element propagates upwards through its ancestor elements. This propagation forms the backbone of event handling across web applications. As an event occurs, it ripples through the DOM tree, triggering event handlers on each ancestor element until the root of the document is reached.
Event bubbling simplifies event management by allowing you to attach event listeners to a common ancestor element instead of every individual child element. This results in cleaner, more maintainable code and a reduction in memory overhead. Consider the following HTML structure.
<div id="outer">
<div id="inner">
<button id="button">Click me!</button>
</div>
</div>
When the "Click me!" button is clicked, the event will propagate from the innermost element (the button) up to the outermost element (the outer <div>
).
2. The Event Object:
Before we explore methods for controlling event propagation, let's become acquainted with the Event object. The Event object contains essential information about the event, such as the event type, the target element that triggered the event, and additional properties that offer insights into the event's context.
When an event handler is invoked, it receives an Event object as a parameter. This object can be used to access various properties and methods related to the event. For example:
document.getElementById('button').addEventListener('click', function (event) {
console.log('Event type:', event.type);
console.log('Target element:', event.target);
});
3. Methods for Event Bubbling:
stopPropagation(): The
stopPropagation()
method allows you to prevent an event from continuing its propagation up the DOM tree. It essentially halts the event's journey, ensuring that subsequent ancestor elements will not be notified of the event. This can be particularly useful when you want to isolate the event's impact on a specific element.Here's an example illustrating the usage of
stopPropagation()
:const parent = document.getElementById('parent'); const child = document.getElementById('child'); parent.addEventListener('click', function (event) { console.log('Parent clicked'); }); child.addEventListener('click', function (event) { console.log('Child clicked'); event.stopPropagation(); // Prevent propagation to the parent });
In this scenario, clicking the child element will only trigger the child's event handler and the parent's event handler will not be invoked.
stopImmediatePropagation(): While
stopPropagation()
prevents further propagation of the event,stopImmediatePropagation()
goes a step further by not only halting the current event's propagation but also preventing other listeners of the same event type from executing. This method is particularly useful when you need fine-grained control over event propagation.Consider the following example:
const button = document.getElementById('button'); button.addEventListener('click', function (event) { console.log('First listener'); }); button.addEventListener('click', function (event) { console.log('Second listener'); event.stopImmediatePropagation(); // Halt propagation and subsequent listeners }); button.addEventListener('click', function (event) { console.log('Third listener'); // This listener will not execute });
In this case, clicking the button triggers the first and second listeners, but the third listener is skipped due to
stopImmediatePropagation()
.Capture Phase: Event propagation involves two phases: the capture phase and the bubbling phase. By default, event listeners are attached to the bubbling phase. However, you can choose to capture events during the capture phase, which occurs before the bubbling phase. This can be achieved by setting the third parameter of the
addEventListener()
method totrue
.Let's illustrate the capture phase with an example:
const parent = document.getElementById('parent'); const child = document.getElementById('child'); parent.addEventListener( 'click', function (event) { console.log('Parent (Capture phase)'); }, true // Enable capture phase ); child.addEventListener('click', function (event) { console.log('Child clicked'); });
Here, when you click the child element, the parent's event listener is triggered during the capture phase, followed by the child's event listener during the bubbling phase.
Event Delegation: Efficient Event Handling with Bubbling: Event delegation is a powerful technique that leverages event bubbling to manage events efficiently, especially for elements dynamically added to the DOM. Instead of attaching an event listener to each element, you attach a single event listener to a common ancestor. As events bubble up to the ancestor, you can examine the event's target and decide how to handle it based on its properties.
Consider the following example involving a list of items:
<ul id="item-list"> <li>Item 1</li> <li>Item 2</li> <li>Item 3</li> </ul>
const itemList = document.getElementById('item-list'); itemList.addEventListener('click', function (event) { if (event.target.tagName === 'LI') { console.log('Clicked on:', event.target.textContent); } });
Here, a single event listener on the
<ul>
element handles clicks on its child<li>
elements. When you click on an item, the event bubbles up, and the event listener checks if the clicked element is an<li>
, logging the clicked item's text content.
4. Common Use Cases:
Understanding event bubbling and propagation is essential in various real-world scenarios. Here are a few common use cases:
Preventing Modals from Closing: When working with models or pop-up dialogues, you may want to prevent them from closing when users interact with their contents. By stopping event propagation within the modal, you ensure that clicks inside the modal do not reach the modal's close button or overlay.
Interactive Components: Building interactive components like dropdown menus, tooltips, and accordions often involves managing events for multiple elements. Event delegation simplifies the process by allowing you to handle events on a single parent element, regardless of the number of interactive elements within it.
5. Best Practices:
As you dive deeper into event bubbling and propagation, keep these best practices in mind:
Use Event Delegation: Embrace event delegation, especially for scenarios involving dynamically generated or frequently changing elements. By attaching a single event listener to a common ancestor, you ensure efficient event handling and reduce the complexity of your code.
Strategically Use Propagation Control Methods: While methods like
stopPropagation()
andstopImmediatePropagation()
offers granular control over event propagation, use them judiciously. Overusing these methods can lead to unintended consequences and hinder the natural flow of event handling.
Conclusion
In the dynamic landscape of web development, understanding event bubbling and propagation is a pivotal skill. By grasping these concepts and mastering the methods for controlling event propagation, you gain the ability to craft seamless and responsive web applications. Armed with this knowledge, you can navigate the intricacies of event handling in JavaScript with confidence, ensuring a richer user experience and a more efficient codebase.
Subscribe to my newsletter
Read articles from Debasmit Biswal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
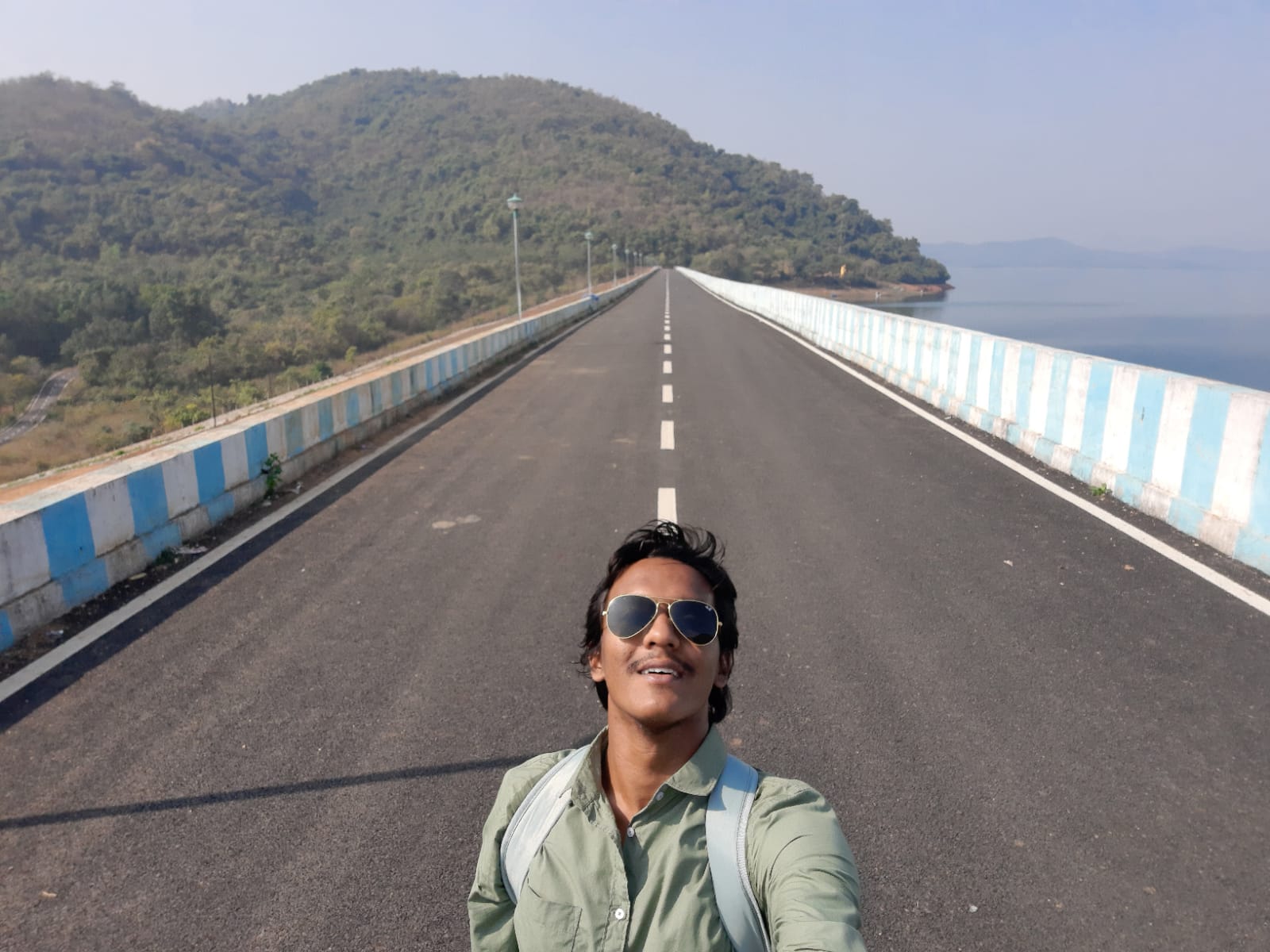
Debasmit Biswal
Debasmit Biswal
I am currently open for SDE roles