Synchronous and Asynchronous Functions in JavaScript: A Comprehensive Guide
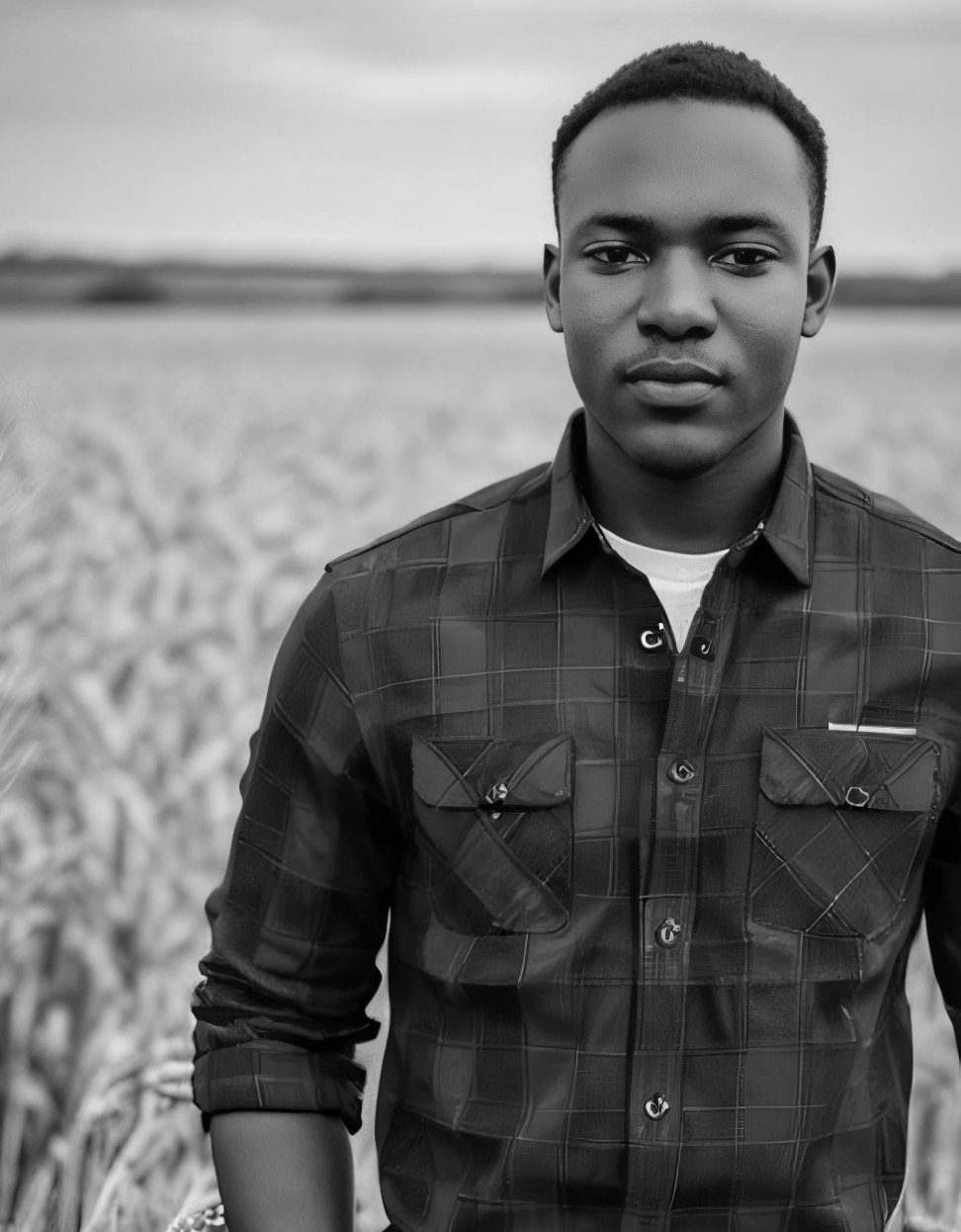
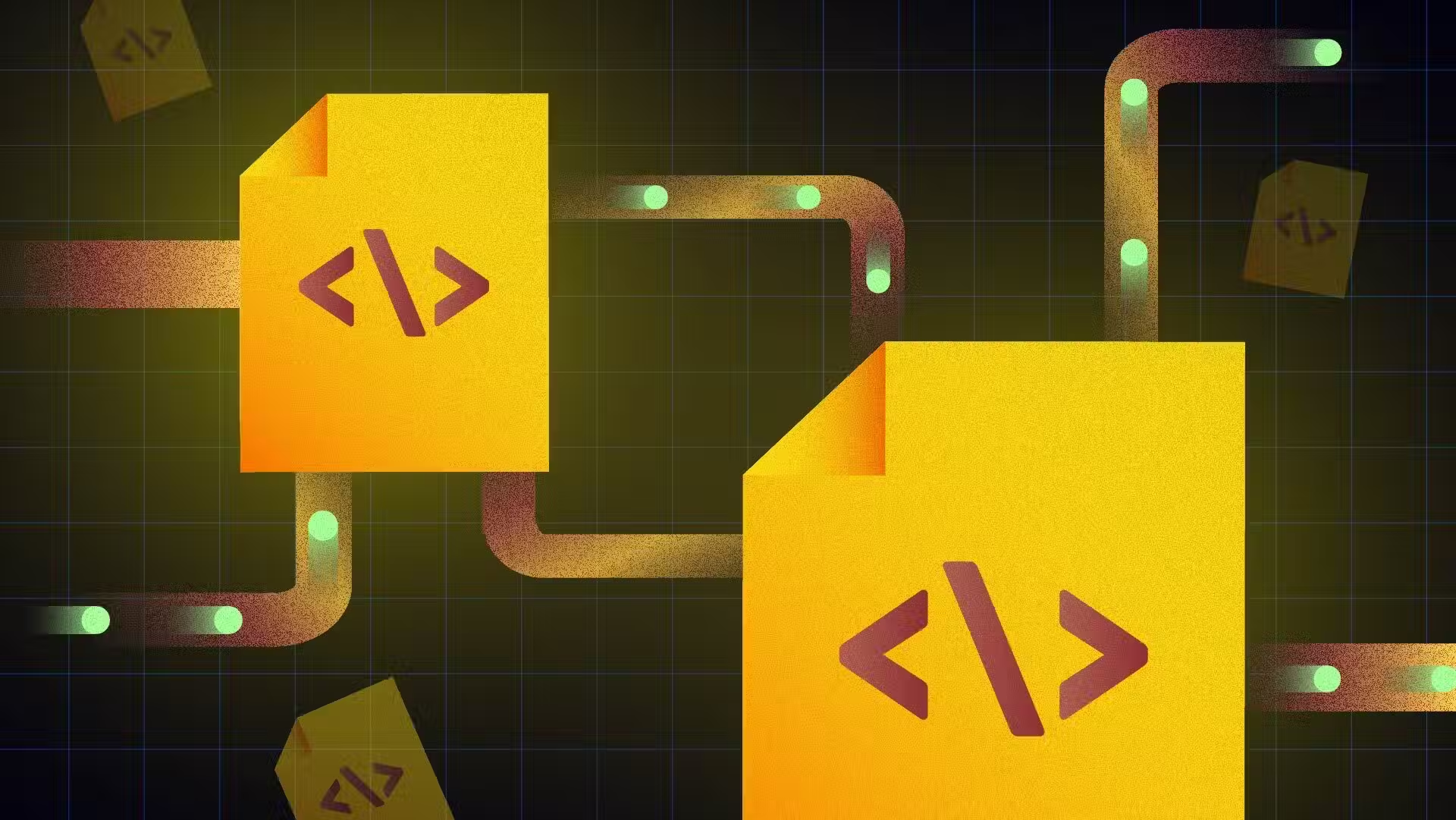
JavaScript is a versatile programming language, it is a very essential aspect of web development and right now it is one of the most used programming languages worldwide. In the world of javascript programming, two fundamental concepts stand out: synchronous and asynchronous functions. Understanding their differences, capabilities, pitfalls, and applications is important for any developer aiming to create efficient, responsive, and user-friendly applications. In this comprehensive guide, we will dive deep into the world of synchronous and asynchronous functions in JavaScript, exploring their definitions, differences, examples, common pitfalls, and the significance they hold in modern programming.
Prerequisites
For the reader to be able to grasp a full understanding of this article,
You need to understand the basics of javascript and how it works.
You also need a code editor (preferably Visual Studio Code)
Lastly, A browser (Chrome or Firefox will work).
Understanding Synchronous and Asynchronous Functions.
Synchronous Functions
What are Synchronous Functions?
Synchronous functions are functions that execute one after another in the order they appear in the code. Each function blocks the execution thread until it completes, preventing the next function from running until the current function finishes. Synchronous functions are straightforward and also easy to reason about, making them suitable for tasks that don't involve external operations that take time.
- Synchronous Function example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Synchronous Func</title>
</head>
<body>
<div class="container">
<h2>The area of the rectangle is: <span class="output"> </span></h2>
</div>
<script src="./app.js"></script>
</body>
</html>
const output = document.querySelector('.output');
function calculateRectangleArea(width, height) {
return width * height;
}
function main() {
const width = parseFloat(prompt("Enter the width of the rectangle:"));
const height = parseFloat(prompt("Enter the height of the rectangle:"));
const area = calculateRectangleArea(width, height);
output.textContent = area;
}
main();
In the code blocks provided above:
The html code defines a simple container so we can output the result to the browser.
The
calculateRectangleArea
function calculates the area of a rectangle which is the multiplication of the provided width and height.The declared
main
function uses the inbuilt javascriptprompt
function to ask the user to input the width and height of the rectangle.The user's input is converted to numbers using
parseFloat
to ensure accurate calculations.The
calculateRectangleArea
function is called with the user's inputted width and height, and the calculated area is stored in thearea
variable.Then the calculated area is appended to the output element so it can be displayed on the browser
This program runs synchronously. When the prompt
function is called, the program waits for the user to provide input before moving on. Similarly, the program calculates the area and outputs the result in order.
This simple example demonstrates how synchronous functions in JavaScript allow the program to wait for user input and function execution to complete before moving on to the next step.
Advantages of using synchronous functions:
Synchronous functions, while often considered less flexible than asynchronous functions, offer several benefits in specific areas. Here are some advantages of using synchronous functions:
Simplicity and Readability: Synchronous code is often simpler to write and easier to understand, especially for beginners and when dealing with straightforward tasks. The linear flow of execution mirrors the natural progression of the task, making the code more intuitive and readable.
Predictable Execution: Synchronous functions execute one after another in a predictable order, making it easier to reason about the program's behavior. This predictability simplifies debugging and troubleshooting.
Sequential Logic: Synchronous functions are well-suited for tasks that require a strict sequential order of operations, such as mathematical calculations, procedural data processing, or tasks with strict dependencies.
Common Pitfalls and Challenges:
Execution blocking: Synchronous programming can lead to blocking the entire program when a task takes a long time to complete. This can result in unresponsiveness and a poor user experience. To fix this, it is recommended that you move time-consuming tasks, such as network requests or complex calculations, to separate threads or processes.
Scalability: The scalability of an application may also be affected by synchronous functions. The number of synchronous operations that must be performed can grow along with the number of simultaneous users. This may cause the application to get congested and unable to handle increased traffic.
Difficult to debug: Debugging synchronous functions can be challenging since it might be difficult to identify the root cause of an issue when the calling thread is blocked.
Asynchronous Functions
What are Asynchronous Functions?
Asynchronous functions, in opposite to Synchronous functions, enable other code to run while the function is in progress. They are particularly useful when dealing with time-consuming tasks, such as data fetching, file Input/Output, or network requests. Asynchronous functions return Promises or use callbacks to handle the eventual completion of the operation. This non-blocking behavior ensures that applications remain responsive even when dealing with operations that may take time.
- Example of an Asynchronous Function:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Async Func</title>
</head>
<body>
<div class="container">
<h2 class="output"></h2>
</div>
<script src="./app.js"></script>
</body>
</html>
const output = document.querySelector('.output');
function fetchData(callback) {
setTimeout(() => {
const data = ['apple', 'banana', 'cherry'];
callback(data);
}, 1000); // Imitating fetching data with a delay of 1 second
}
function main() {
output.textContent = `Fetching data...` ;
fetchData((data) => {
output.textContent =`Your Data: ${data}`;
});
}
main();
In the code blocks provided above:
The html code defines a simple container so we can output the result to the browser.
The
fetchData
function imitates the process of fetching data from an external source usingsetTimeout
to delay the process by 1000 milliseconds.The
main
function updates a message on the browser to indicate data fetching and upon the end of thesetTimeout
function which is 1 second, Themain
function callsfetchData
and provides an inline callback function. This callback is executed once the data is "fetched.", it replaces the message with the data array that was fetched.
The program does not wait for the data to be fetched; it continues executing other tasks. The setTimeout
function creates a 1-second delay, and the provided callback is programmed to execute immediately after the delay. This is a simple demonstration of how asynchronous functions allow non-blocking execution in JavaScript, making it possible to perform tasks like data fetching, handling events, and more without blocking the main program flow.
Advantages of using Asynchronous functions:
Asynchronous functions offer several benefits, particularly in cases where responsiveness, scalability, and efficient resource utilization are essential. Here are some advantages of using asynchronous functions:
Improved Responsiveness: Asynchronous functions prevent blocking the main thread, allowing the program to remain responsive even when performing time-consuming tasks. This is crucial for applications with user interfaces that need to remain interactive while handling background operations.
Concurrency and Parallelism: Asynchronous functions enable concurrent execution of multiple tasks without waiting for each one to complete. This leads to improved performance, effective use of resources, and the ability to handle multiple requests at the same time.
Scalability: Asynchronous programming is well-suited for scalable applications that need to handle numerous simultaneous users or processes. It allows the application to handle incoming requests without getting bogged down by lengthy operations.
Enhanced User Experience: Applications that use asynchronous functions can deliver a smoother and more responsive user experience, as tasks that might otherwise cause delays can be offloaded to the background.
Common Pitfalls and Challenges:
Callback Hell: Callback hell, commonly referred to as the "pyramid of doom," is a condition in asynchronous programming when numerous nested callback functions make the code challenging to read, maintain, and comprehend. This happens when several connected asynchronous actions exist, resulting in highly nested indentation and diminished code clarity.
Race conditions: Race conditions are errors that happen when multiple functions attempt to access the same data at the same time. Asynchronous functions might result in race conditions. Two asynchronous functions trying to update the same variable simultaneously may result in this.
Complex code: If you're not aware of how they operate, asynchronous functions can complicate your code. Because of this, it could be challenging to maintain your code and troubleshoot it when something goes wrong.
Differences between Synchronous and Asynchronous Functions
The main difference between synchronous and asynchronous functions lies in how they handle execution and waiting. Synchronous functions block the execution thread until they are complete, while asynchronous functions allow other code to run concurrently. Tasks are carried out progressively by synchronous functions, blocking the program until they are all completed. They provide simplicity and predictability, yet they can also result in inactions popularly called "hangs" during laborious tasks.
Asynchronous functions, on the other hand, operate concurrently and improve responsiveness by working on tasks in the background. They ensure a positive user experience, effectively manage complicated activities like network requests and maximize resource consumption. Due to callbacks or promise chains, they complicate error handling and control flow, nevertheless. Whether sequential logic or concurrent execution is required will determine whether to use synchronous or asynchronous functions. Asynchronous functions excel in responsiveness and resource efficiency, making them perfect for current applications, while synchronous functions are best for simple tasks.
Balancing Synchronous and Asynchronous Operations
Synchronous and asynchronous operations are two types of operations that can be used in programming. Synchronous operations block the thread that is executing them, while asynchronous operations do not. This means that synchronous operations can make an application unresponsive, while asynchronous operations can improve responsiveness.
Synchronous functions are ideal for linear, step-by-step processes like simple calculations and local data operations, while asynchronous functions are crucial for managing time-consuming tasks like handling data fetching from external resources and creating web applications that can do multiple things at once and adapt to dynamic events.
Careful consideration of the nature of your task and its potential impact on the overall program flow will guide your choice between synchronous and asynchronous approaches.
When you're writing code, you need to decide which type of operation to use for each task. Here are a few things to keep in mind:
Is the operation time-critical? If the operation needs to be completed as quickly as possible, it should be synchronous.
Does the operation need to be able to handle a lot of concurrent requests? If the operation needs to be able to handle a lot of requests at the same time, it should be asynchronous.
Can the operation be broken down into smaller tasks? If the operation can be broken down into smaller tasks, each task can be executed asynchronously.
In general, it's best to use asynchronous operations whenever possible. This will improve the responsiveness of your application and make it more scalable. However, there are some cases where synchronous operations are necessary. For instance, a time-critical operation should be synchronous.
Here are some tips for balancing synchronous and asynchronous operations:
Use asynchronous operations for tasks that don't need to be executed synchronously.
Use synchronous operations for tasks that are time-critical or that need to ensure the results are available before the next function is called.
Use a combination of synchronous and asynchronous operations to improve the performance and scalability of your application.
Test your code thoroughly to make sure that it is handling asynchronous operations correctly.
By following these tips, you can balance synchronous and asynchronous operations in your code to improve the performance, scalability, and responsiveness of your application.
Conclusion
Building effective, responsive, and maintainable applications requires a knowledge of the subtle differences between synchronous and asynchronous functions in the world of programming.
Simple calculations, procedural chores, and local data processing are all well-suited for synchronous functions and their step-by-step execution. They offer predictability and a clear flow of events, but they can also become unresponsive, restrict concurrency, and make managing shared resources difficult.
But when it comes to managing time-consuming tasks like network requests, file I/O, and user interactions, asynchronous functions are preferred. They preserve responsiveness and enable more effective resource use by allowing processes to perform concurrently without interrupting the main thread. They complicate code organization, error handling, and debugging, too. So the most essential thing to understand is knowing about both functions and when necessary to use them.
Subscribe to my newsletter
Read articles from Omotosho Toheeb directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
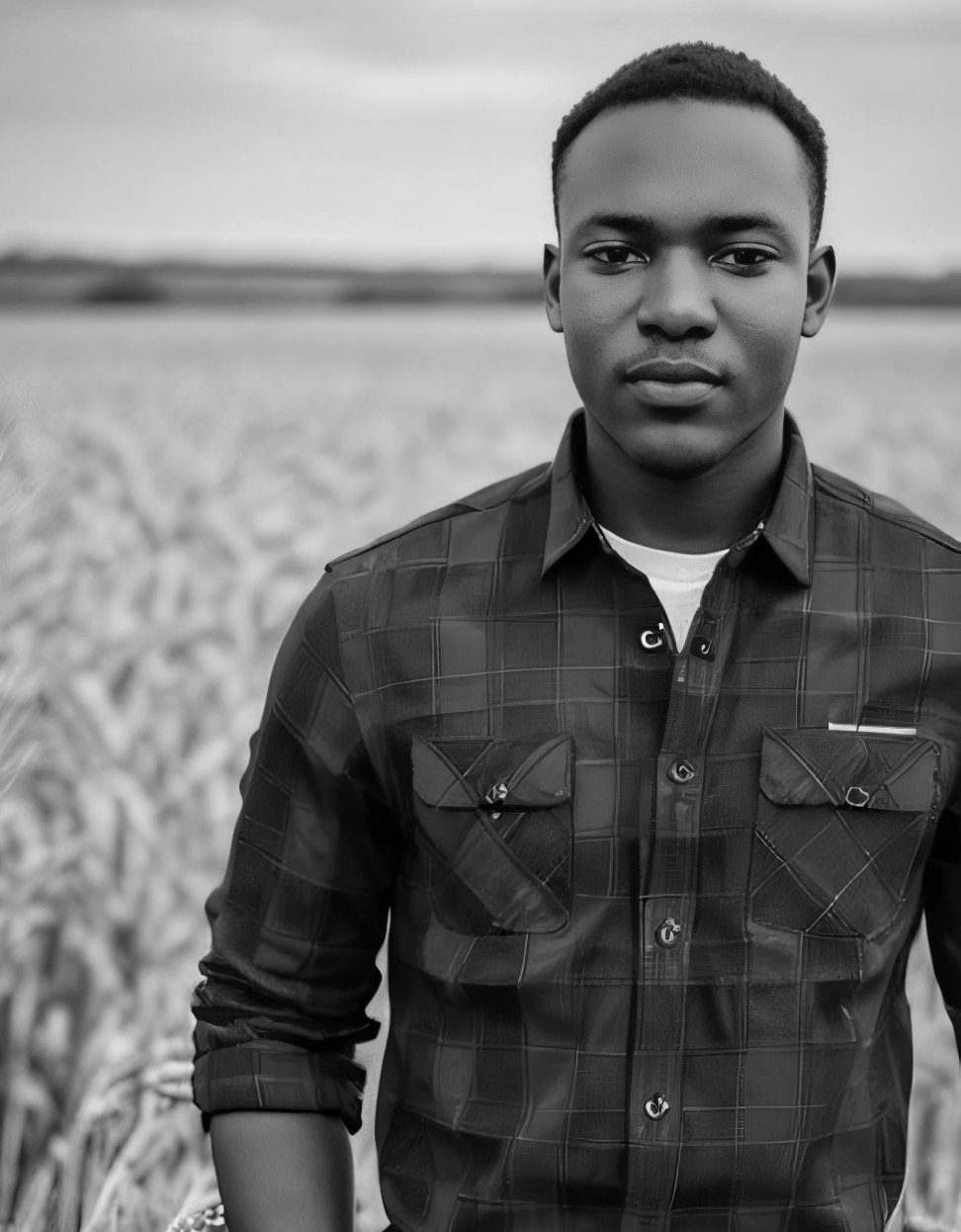
Omotosho Toheeb
Omotosho Toheeb
Front-End dev