Power of Scope in JavaScript: Why Every Developer Should Master It
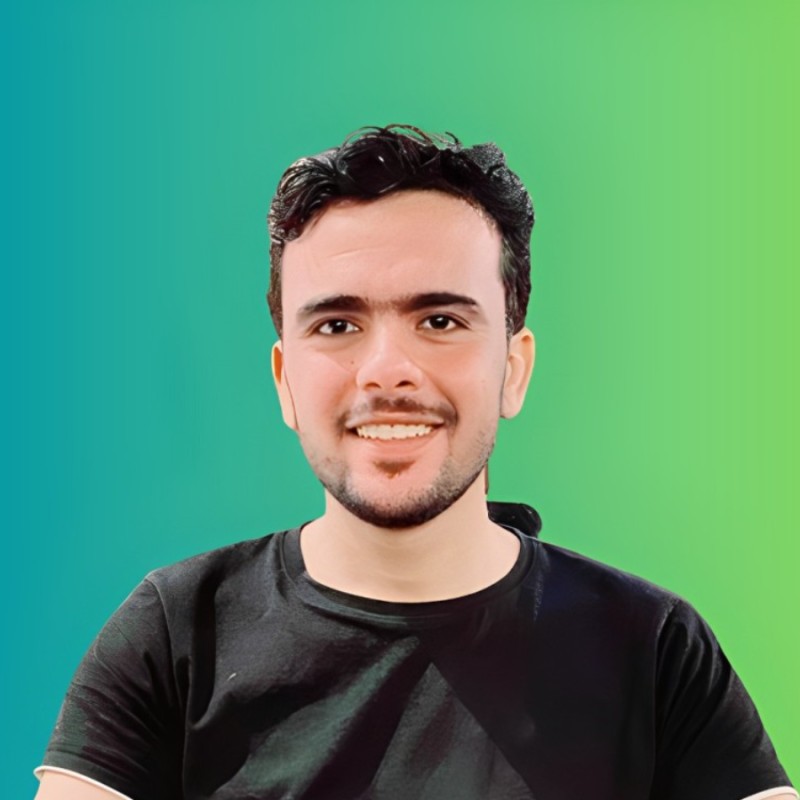
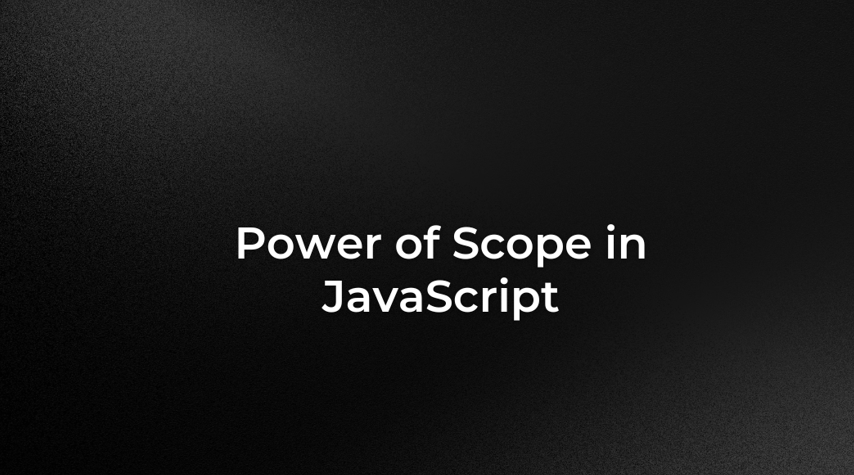
Introduction
As developers, our journey into the JavaScript realm begins with understanding its foundational concepts, and one of the most crucial of these concepts is “scope.” In this article, we will delve into the world of JavaScript scope, exploring its significance and highlighting why every developer should strive to master it.
Scope in JavaScript
Scope refers to the context in which variables are declared, accessed, and modified in a JavaScript program. It defines the accessibility and lifetime of a variable within a given portion of the code. JavaScript employs two main types of scope: global scope and local (function) scope.
Global Scope: Variables declared in the global scope are accessible throughout the entire program, making them readily available for any part of the code. However, this widespread accessibility can lead to unintended consequences, such as variable name clashes or security vulnerabilities.
Local (Function) Scope: Variables declared within a function have a limited scope, existing only within the confines of that function. This encapsulation ensures that variables do not interfere with other parts of the program and promotes better code organization and maintenance.
Why Scope Matters
Variable Isolation: Understanding and utilizing local scope ensures that variables do not unintentionally affect other parts of the codebase. This separation of concerns reduces bugs and makes code easier to debug and maintain.
Code Modularity: Embracing scope encourages the creation of modular and reusable code. By isolating functionality within functions and controlling variable access, developers can create independent units that can be easily integrated into various parts of an application.
Performance Optimization: Properly managing scope can lead to performance gains. Local scope allows JavaScript engines to optimize memory usage and enhance execution speed by deallocating resources as soon as they are no longer needed.
Security Enhancement: Global variables are susceptible to security vulnerabilities, as they can be accessed and manipulated by any part of the code. Utilizing local scope helps prevent unauthorized data tampering and enhances the overall security of the application.
Collaboration and Readability: When working on collaborative projects, understanding scope promotes better communication and collaboration among team members. Code that follows well-defined scope rules is more readable and less prone to misunderstandings.
Mastering JavaScript Scope
To master JavaScript scope, developers should focus on the following.
- Global scope
Global scope is a fundamental aspect of JavaScript that defines the accessibility and visibility of variables and functions across the entire codebase.
In JavaScript, variables declared outside of any function or block are considered to be in the global scope. This means they can be accessed from anywhere within the codebase, including other functions, blocks, or files. While global scope offers convenience, it can lead to unintended consequences if not used carefully.
Consider the following example:
var globalVar = 'I am a global variable';
function myFunction() {
console.log(globalVar);
}
myFunction(); // Outputs: I am a global variable
danger of Global Scope
Naming Conflicts: When multiple variables or functions share the same name in different parts of the codebase, it can lead to naming conflicts and unexpected behavior. This is particularly problematic in large projects with multiple developers.
Unintended Modifications: Variables in the global scope are accessible from anywhere, which means they can be unintentionally modified by different parts of the code. This can lead to bugs that are difficult to diagnose and fix.
Security Risks: Global variables are susceptible to security vulnerabilities. Sensitive data stored in global variables can be accessed or modified by malicious code, compromising the security of the application.
Maintenance Challenges: As the codebase grows, managing and tracking global variables becomes increasingly difficult. Debugging and maintaining code with numerous global variables can be time-consuming and error-prone.
Best Practices for Global Scope
Limit Global Variables: Minimize the use of global variables whenever possible. Instead, use local scope within functions to encapsulate data and functionality.
Namespacing: Group related variables and functions under a common object to avoid naming conflicts. This practice, known as namespacing, can help organize the global scope and prevent clashes with other parts of the codebase.
Use
const
andlet
: Prefer usingconst
orlet
overvar
when declaring global variables.const
ensures that the variable's value remains unchanged, whilelet
allows for reassignment if necessary.Modularize Code: Organize your code into modules or separate files to encapsulate functionality and reduce the reliance on global variables.
Global Constants: If you need global constants, declare them using uppercase letters to indicate their immutability and importance.
2. Function Scope:
Function scope is a fundamental concept in JavaScript that defines the accessibility and visibility of variables within the context of a function. Understanding function scope is crucial for writing clean, maintainable, and bug-free code. Let’s explore the intricacies of function scope and its impact on the way we structure and manage our JavaScript programs.
In JavaScript, variables declared within a function have function scope, meaning they are accessible only within the scope of that function. This isolation ensures that variables defined inside a function do not interfere with variables outside the function, promoting encapsulation and reducing the chances of naming conflicts.
Consider the following example:
function myFunction() {
var localVar = 'I am a local variable';
console.log(localVar);
}
// localVar is not accessible here
3. Block Scope and the power of let
and const
:
In the evolution of JavaScript, the introduction of ES6 (ECMAScript 2015) marked a significant milestone with the inclusion of block scope through the let
and const
keywords. Prior to ES6, JavaScript had only function scope and global scope, which could sometimes lead to unexpected variable behavior and clashes. Block scope brought a new level of control and predictability to how variables are accessed and managed within different blocks of code.
what is block scope?
Block scope essentially confines the scope of a variable to the block (a pair of curly braces {}
) in which it is defined. This block can be a loop, a conditional statement, or any other set of curly braces. Before the introduction of block scope, variables defined within loops or if statements had a broader scope, leading to potential issues when these variables were accessed outside the intended block.
With block scope, variables declared using let
and const
are isolated to the specific block in which they are defined. This isolation prevents unintended variable leakage and conflicts, contributing to cleaner and more reliable code.
The let Keyword
The let
keyword allows developers to declare variables with block scope. Variables declared using let
are accessible only within the block where they are defined. Once the block is exited, the variable's scope is terminated, and the variable is no longer accessible.
Example:
function exampleFunction() {
if (true) {
let x = 10;
console.log(x); // Output: 10
}
// console.log(x); // Error: x is not defined
}
The const
Keyword
Similar to let
, the const
keyword also introduces block scope. However, const
has an additional characteristic: it creates variables that are read-only and cannot be reassigned after their initial value is assigned. This immutability can be particularly useful for creating constants or preventing accidental variable reassignment.
Example:
function exampleFunction() {
if (true) {
const y = 20;
console.log(y); // Output: 20
// y = 30; // Error: Assignment to constant variable
}
}
Benefits of Block Scope
Variable Safety: Block scope minimizes the chances of variable conflicts and unintended variable modification, as variables are limited to the specific block in which they are defined.
Predictability: With block scope, it’s easier to understand where a variable is accessible and where it is not. This improves code predictability and reduces debugging time.
Performance Optimization: Block-scoped variables have a shorter lifetime, which can lead to better memory management and performance optimization by allowing JavaScript engines to release resources sooner.
Code Clarity: By using
let
andconst
within specific blocks, developers signal their intent about where a variable should be used, making the codebase more readable and maintainable.
Conclusion
In the dynamic landscape of web development, a solid grasp of JavaScript’s scope is paramount for any developer aspiring to write efficient, maintainable, and secure code. As we conclude our exploration of JavaScript scope, let’s recap the key takeaways and emphasize its significance in shaping the way we build applications.
Here’s a quick recap of what we’ve covered:
Global Scope and Its Implications: Global scope provides universal access to variables and functions, allowing data to be shared across the entire codebase. However, this convenience comes with risks, including naming conflicts, unintended modifications, security vulnerabilities, and maintenance challenges. Adopting best practices like limiting global variables, using proper naming conventions, and modularizing code can mitigate these risks and promote cleaner, more organized development.
Function Scope and Encapsulation: Function scope confines variables within the scope of a function, promoting encapsulation and preventing interference with external code. This separation of concerns improves code predictability, prevents naming clashes, and fosters modular design. Understanding closures, which arise from function scope, empowers developers to create powerful and efficient solutions by maintaining access to variables beyond the function’s execution.
Block Scope and the Power of let and const: ES6 introduced block scope through the
let
andconst
keywords, enabling fine-grained control over variable accessibility within blocks of code. Block scope enhances variable safety, predictability, performance optimization, and code clarity. By embracinglet
andconst
, developers can avoid variable leakage, improve memory management, and create more understandable code.
Subscribe to my newsletter
Read articles from Amr Mohamed directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
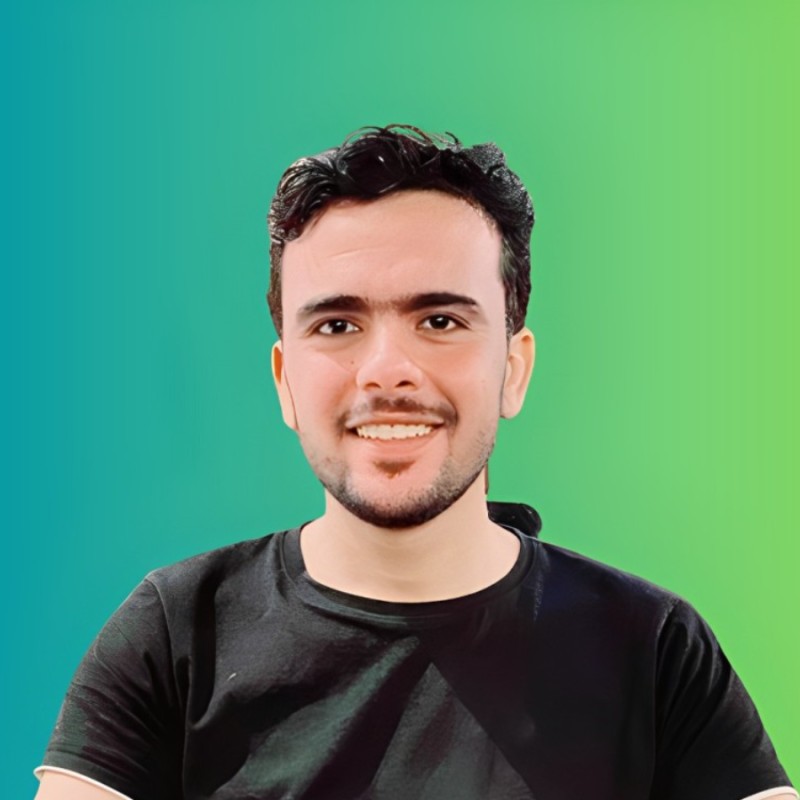
Amr Mohamed
Amr Mohamed
I am a dedicated and experienced Full Stack Developer with three years of expertise in software development. Throughout my career, I have successfully worked across various stages of development, specializing in both frontend and backend technologies. I have a strong proficiency in utilizing cloud services, particularly AWS, to create scalable and efficient solutions. I excel in collaborative environments and thrive as a proactive team member, leveraging my programming skills to solve complex business problems. I am driven by challenging projects and strive to deliver exceptional results. My focus on code quality ensures bug-free applications, optimal performance, and long-term maintainability. With a proven track record of delivering high-quality software solutions, I am eager to contribute my technical proficiency and problem-solving abilities to your organization. I am excited about opportunities to leverage my skills and make a significant impact in driving innovation and fostering growth.