Shell Scripting Challenge: Day 04 and 05
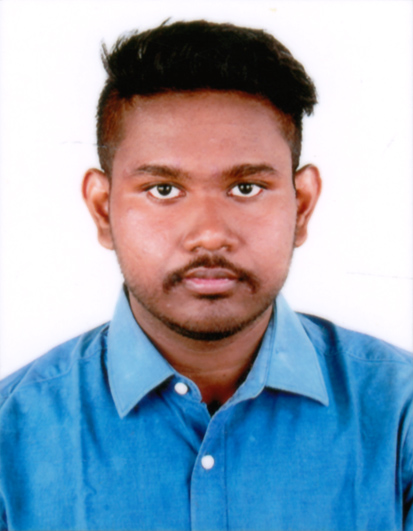
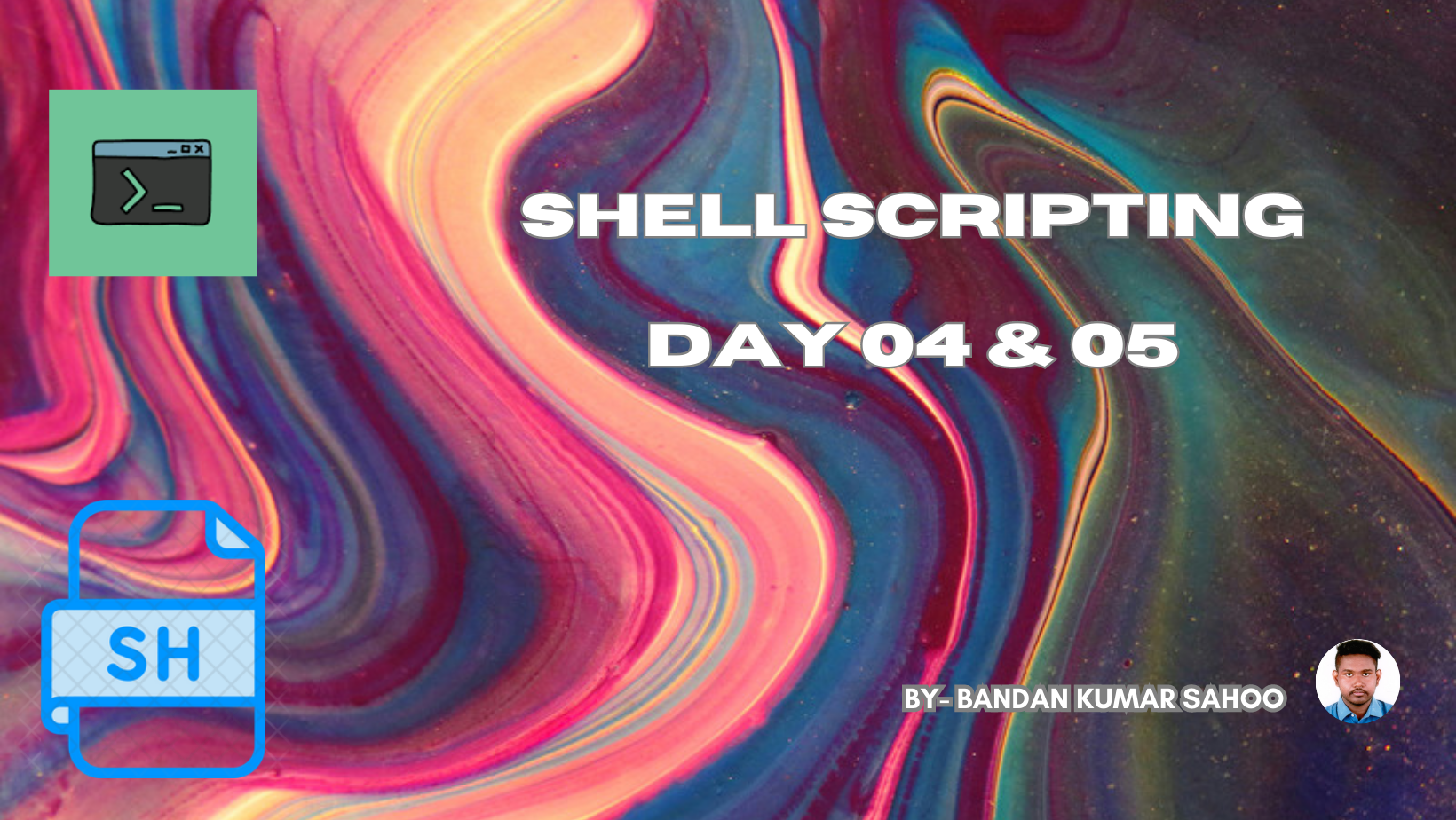
Welcome to the Bash Scripting Challenge - Day 4! This challenge is designed to test your Bash scripting skills and problem-solving abilities in the context of process monitoring and management.
Scenario
You are tasked with writing a Bash script that efficiently monitors a specific process on a Linux system. The script's primary goal is to ensure the process is always running, and if it unexpectedly stops, it should be restarted automatically.
Task
Process Selection:
- The script should accept a command-line argument to specify the target process to monitor. For example:
./monitor_process.sh <process_name>
.
- The script should accept a command-line argument to specify the target process to monitor. For example:
Process Existence Check:
Implement a function that checks if the specified process is currently running on the system.
If the process is running, print a message indicating its presence.
Restarting the Process:
If the process is not running, implement a function that attempts to restart the process automatically.
Print a message indicating the attempt to restart the process.
Ensure the script does not enter an infinite loop while restarting the process. Limit the number of restart attempts.
Automation:
- Provide instructions on how to schedule the script to run at regular intervals using a cron job or any other appropriate scheduling method.
Documentation:
Include clear and concise comments in the script to explain its logic and functionality.
Write a separate document describing the purpose of the script, how to use it, and any specific considerations.
Bonus:
- Implement a notification mechanism (e.g., email, Slack message) to alert administrators if the process requires manual intervention after a certain number of restart attempts.
Remember to test your script thoroughly on a test system before deploying it to a production environment. Ensure it does not interfere with critical processes or cause any unintended side effects. Consider edge cases and potential race conditions during process monitoring and restarting.
Answer
#!/bin/bash
process_name=$1
#process existence check
function check_process_running() {
if pgrep -x "$process_name" >/dev/null; then
echo "The process $process_name is running."
return 0
else
echo "The process $process_name is not running."
return 1
fi
}
#restart process
max_attempts=3
current_attempt=1
function restart_process() {
echo "Restarting $process_name..."
systemctl restart "$process_name"
}
while true; do
if check_process_running; then
break
fi
if [ "$current_attempt" -le "$max_attempts" ]; then
restart_process
current_attempt=$((current_attempt + 1))
else
echo "Max restart attempts reached. Exiting..."
echo "Please try to restart the process manually."
exit 1
fi
done
Monitoring Metrics Script with Sleep Mechanism
Challenge Description
This project aims to create a Bash script that monitors system metrics like CPU usage, memory usage, and disk space usage. The script will provide a user-friendly interface, allow continuous monitoring with a specified sleep interval, and extend its capabilities to monitor specific services like Nginx.
Tasks
Task 1: Implementing Basic Metrics Monitoring
Write a Bash script that monitors the CPU usage, memory usage, and disk space usage of the system. The script should display these metrics in a clear and organized manner, allowing users to interpret the data easily. The script should use the top, free, and df commands to fetch the metrics.
Task 2: User-Friendly Interface
Enhance the script by providing a user-friendly interface that allows users to interact with the script through the terminal. Display a simple menu with options to view the metrics and an option to exit the script.
Task 3: Continuous Monitoring with Sleep
Introduce a loop in the script to allow continuous monitoring until the user chooses to exit. After displaying the metrics, add a "sleep" mechanism to pause the monitoring for a specified interval before displaying the metrics again. Allow the user to specify the sleep interval.
Task 4: Monitoring a Specific Service (e.g., Nginx)
Extend the script to monitor a specific service like Nginx. Check if the service is running and display its status. If it is not running, provide an option for the user to start the service. Use the systemctl or appropriate command to check and control the service.
Task 5: Allow User to Choose a Different Service
Modify the script to give the user the option to monitor a different service of their choice. Prompt the user to enter the name of the service they want to monitor, and display its status accordingly.
Task 6: Error Handling
Implement error handling in the script to handle scenarios where commands fail or inputs are invalid. Display meaningful error messages to guide users on what went wrong and how to fix it.
Task 7: Documentation
Add comments within the script to explain the purpose of each function, variable, and section of the code. Provide a clear and concise README file explaining how to use the script, the available options, and the purpose of the script.
Remember, the main goal of this challenge is to utilize various monitoring commands, implement a user-friendly interface, and create a script that is easy to understand and use.
Feel free to explore and research relevant commands and syntax while completing the tasks. Enjoy monitoring your system metrics effortlessly.
Answer
#!/bin/bash
# Interactive Menu
Interact_menu() {
echo "Choose from tthe following to view items:"
echo "1. System usage metrics"
echo "2. Monitor Nginx Service"
echo "3. Monitor a Different Service"
echo "4. exit"
}
#system metrics
system_usages()
{
echo "1. CPU Usage"
top -bn 1 | grep '%Cpu' | awk '{print $2}'
echo "2. Memory Usage"
free -h
echo "3. Disk Space"
df -h
}
# Function to check and start Nginx service
monitor_nginx_service() {
# Check if Nginx is running
if systemctl is-active --quiet nginx; then
echo "Nginx service is running."
else
echo "Nginx service is not running."
read -p "Do you want to start Nginx? (y/n): " choice
if [[ $choice == "y" ]]; then
sudo systemctl start nginx
echo "Nginx service has been started."
fi
fi
}
# Function to monitor a specific service
monitor_specific_service() {
read -p "Enter the name of the service to monitor: " service_name
if systemctl is-active --quiet "$service_name"; then
echo "$service_name service is running."
else
echo "$service_name service is not running."
fi
}
# Function for error handling
handle_error() {
echo "Error: $1"
exit 1
}
# Clear the screen before the loop starts
clear
# Main script
while true; do
Interact_menu
read -p "Enter your choice: " choice
echo
case $choice in
1) system_usages ;;
2) monitor_nginx_service ;;
3) monitor_specific_service ;;
4) exit 0 ;;
*) handle_error "Invalid choice. Please enter a valid option." ;;
esac
echo
read -p "Press Enter to continue..." -t 3
echo
done
Output
Link to Shell scripting day 05 task - https://bandandevopsjourney.hashnode.dev/shell-scripting-challenge-day-05
Thanks for reading my article. Have a nice day.
You can follow me on LinkedIn for my daily updates:- linkedin.com/in/bandan-kumar-sahoo-131412203
Subscribe to my newsletter
Read articles from Bandan Sahoo directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
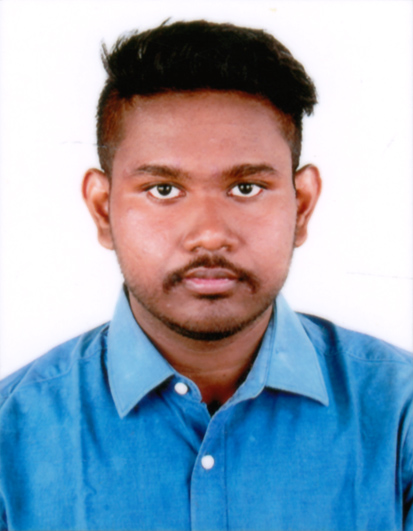
Bandan Sahoo
Bandan Sahoo
I like to explore the technology in DevOps area where I write blog about my learning each day on the tools that is mostly used in Industries for DevOps practices. You can go through my blogs and reach me out in LinkedIn for any suggestions.