Conditional Statement And Loops In Shell Script
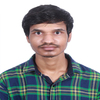
Conditional Statement
-> Conditional Statement is used to perform a certain task based on some conditions.
IF-ELSE
#!/bin/bash
read -p "Enter Your Age - " age
if [ $age -lt 18 ]
then
echo "You can not vote"
else
echo "You can vote"
fi
Output -
ELIF
#!/bin/bash
read -p "Enter Your Marks - " marks
if [ $marks -gt 40 ] && [ $marks -lt 60 ]
then
echo "You got 2nd Division"
elif [ $marks -ge 60 ]
then
echo "You got 1st Division"
else
echo "You are fail"
fi
Output -
CASE
-> CASE is similar to the switch case in other programming language.
Example -
#!/bin/bash
echo "Select One Option - "
echo "a = To see the current date"
echo "b = To see the list of files in current directory"
read choice
case $choice in
a) date;; # We have to use ;; at the last of the command
b) ls;;
*) echo "Please Enter Valid Input"
esac # We have to end the case with esac
Output -
We can use multiple commands as -
#!/bin/bash
echo "Select One Option - "
echo "a = To see the current date"
echo "b = To see the list of files in current directory"
read choice
case $choice in
a)
echo "Hello , We are going to print date"
date
echo "Date is printed"
;;
b) ls;;
*) echo "Please Enter Valid Input"
esac
Output -
Logical Operators
-> We are going to see about &&, || and ! operator.
&&
-> It is a logical AND operator. Here all the conditions must have to be true then only the result will be true else it will be false.
#!/bin/bash
read -p "Enter your age " age
read -p "Enter your country " country
if [[ $age -ge 18 ]] && [[ $country == "India" ]] # In case of string we use "==" and in case of numeric we use "-eq"
then
echo "You can vote in India"
else
echo "You can not vote in India"
fi
Output -
||
-> It is a logical OR operator. Here if any of the conditions is true then the result will be true. If all conditions are false then only the result will be false.
#!/bin/bash
read -p "Enter your age " age
read -p "Enter your country " country
if [[ $age -ge 18 ]] || [[ $country == "India" ]] # In case of string we use "==" and in case of numeric we use "-eq"
then
echo "You can vote in India"
else
echo "You can not vote in India"
fi
Output -
Loops
For Loop - If we have to perform repetitive tasks then we can use the loop.
#!/bin/bash for i in 1 2 3 4 5 do echo "print $i" done
Output -
-> Other way to use For Loop
#!/bin/bash
for i in {1..5}
do
echo "print $i"
done
Output -
Read the values from a File using For Loop -
Example- filename = name.txt
Bhaskar
Mehta
Raju
#!/bin/bash
file=/home/ubuntu/test.txt
for i in $(cat $file)
do
echo "Name is $i"
done
Output -
Read the Values From Array Using For Loop
#!/bin/bash
myArray=(1 2 45 65 "Bhaskar")
arrayLength=${#myArray[*]}
echo "Length of the Array is $arrayLength"
for (( i=0; i<$arrayLength; i++ ))
do
echo "Value is - ${myArray[i]}"
done
Output -
While Loop
-> It will run till the condition is true
#!/bin/bash
count=0
sum=10
while [[ $sum -ge $count ]]
do
echo "Count is - $count"
let count++
done
Output -
Until Loop
-> It is just the opposite of While Loop. i.e. It will run till the condition is false
#!/bin/bash
count=10
sum=5
until [[ $count -lt $sum ]]
do
echo "Count is $count"
let count--
done
Output -
Infinite Loop Using While Loop
-> If we have to run the commands infinite times then we can use this.
#!/bin/bash
while true
do
echo "Hello"
sleep 2s # Every 2s it will print Hello
done
Output -
Infinite Loop Using For Loop
#!/bin/bash
for (( ;; ))
do
echo "Hello"
sleep 2s # Every 2s it will print Hello
done
Subscribe to my newsletter
Read articles from Bhaskar Mehta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
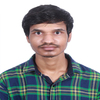
Bhaskar Mehta
Bhaskar Mehta
I am DevOps Engineer who works on DevOps tools like Docker, Kubernetes, Terraform, Git, GitHub, Jenkins and AWS services.