Day 14: Python Data Types and Data Structures for DevOps

Table of contents
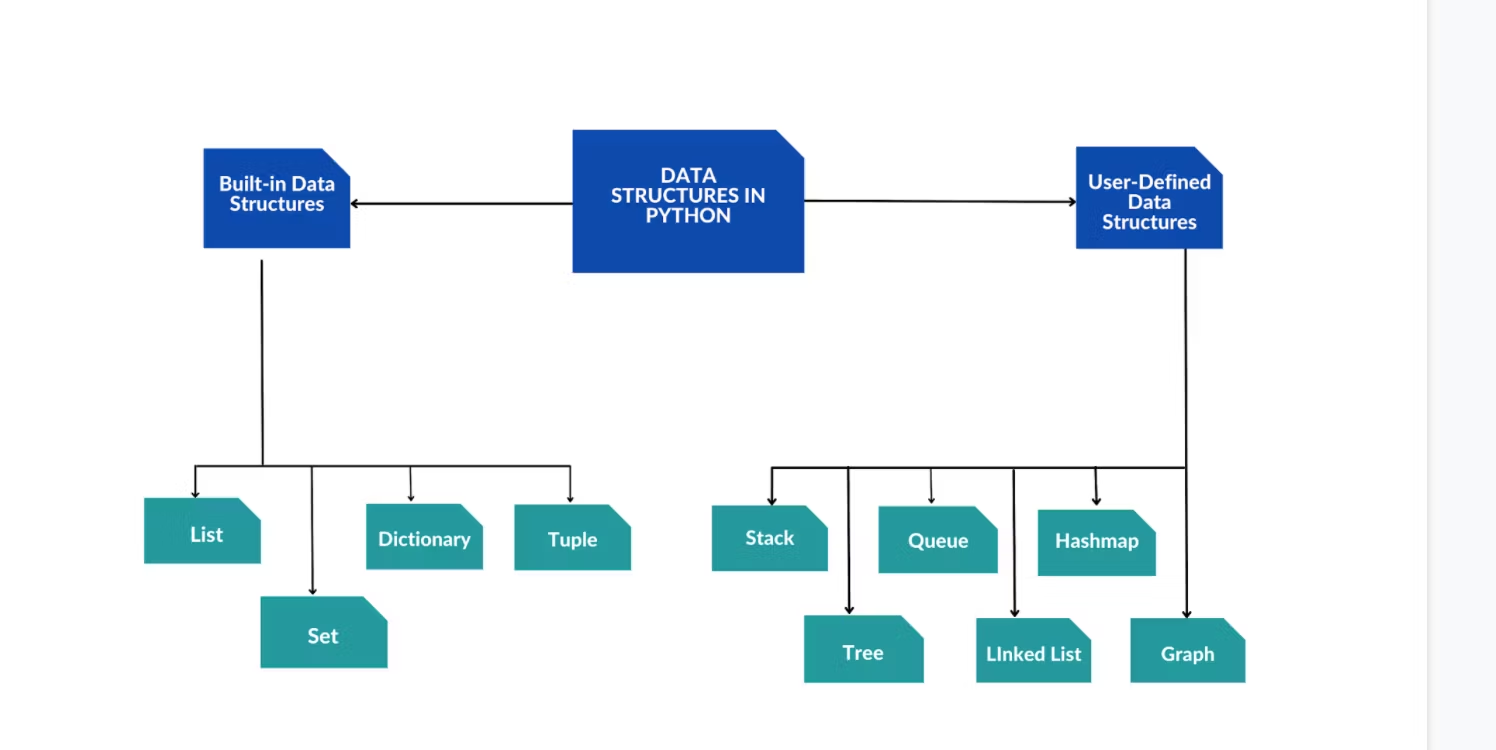
Data Types
Data types are the classification or categorization of data items. It represents the kind of value that tells what operations can be performed on a particular data.
If you want to know about data types in python. Please go through the link below:
https://moiz-journeytodevops.hashnode.dev/day13-basics-of-python-for-devops
Data Structures
Data structures in programming are used to organize and store collections of data in an efficient and meaningful way. Python offers a variety of built-in data structures that serve different purposes. Here are some of the most common ones:
Lists:
Lists are ordered collections of items that can be of different data types.
They are mutable, meaning you can add, remove, and modify elements after creation.
Example:
my_list = [1, "hello", 3.14]
Tuples:
Tuples are similar to lists, but they are immutable, meaning their contents cannot be changed after creation.
They are often used to represent fixed collections of elements.
Example:
my_tuple = (1, "hello", 3.14)
Sets:
Sets are unordered collections of unique elements.
They are useful for tasks that require uniqueness, such as removing duplicates from a list.
Example:
my_set = {1, 2, 3}
Dictionaries:
Dictionaries are collections of key-value pairs.
They provide fast access to values using their associated keys.
Example:
my_dict = {"name": "Alice", "age": 25}
These data structures serve various purposes and are chosen based on the specific requirements of your program, such as the need for fast access, efficient insertion and deletion, uniqueness, and immutability.
Tasks
Give the Difference between List, Tuple, and Set. Do Hands-on and put screenshots as per your understanding.
Here are the key differences between List, Tuple, and Set:
Feature | List | Tuple | Set |
Order | Ordered | Ordered | Unordered |
Mutability | Mutable | Immutable | Mutable |
Elements | Can be duplicate | Can be duplicate | Unique |
Syntax | [1, 2, 3] | (1, 2, 3) | {1, 2, 3} |
Use cases | General-purpose | Fixed collection | Uniqueness check |
Indexing | Available | Available | Not available |
Modification | Add, remove, modify | Not allowed | Add, remove |
1. List :
Let's create a list of cloud service providers and modify it by adding and changing elements.
2. Tuple:
Let's create a tuple of fruits and try to modify it (which will result in an error).
3. Set:
Let's create a set of numbers and demonstrate how duplicates are automatically removed.
Create below Dictionary and use Dictionary methods to print your favourite tool just by using the keys of the Dictionary.
fav_tools = { 1:"Linux", 2:"Git", 3:"Docker", 4:"Kubernetes", 5:"Terraform", 6:"Ansible", 7:"Chef" }
Happy Learning!
Subscribe to my newsletter
Read articles from Moiz Asif directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
