Mastering Data Tables in React: A Comprehensive Guide to Implementation and Functionality
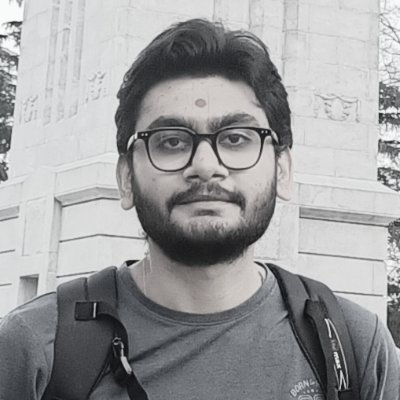
Table of contents
- Introduction:
- 1. Choosing the Right React Table Package
- 2. Basic Table Implementation in React
- 3. Sorting and Filtering Data
- 4. Pagination and Data Fetching
- 5. Customizing Table Appearance
- 6. Handling Cell Editing and Selection
- 7. Advanced Features and Additional Resources
- Expanding Horizons: Advanced Functionalities and Beyond
- 8. Grouping and Aggregation
- 9. Tree Data and Hierarchy
- 10. Row Selection and Actions
- 11. Cell Formatting and Custom Renderers
- Optimizing Performance and Ensuring Accessibility
- 12. Performance Optimization
- 13. Accessibility Considerations
- 14. Additional Resources
- Conclusion
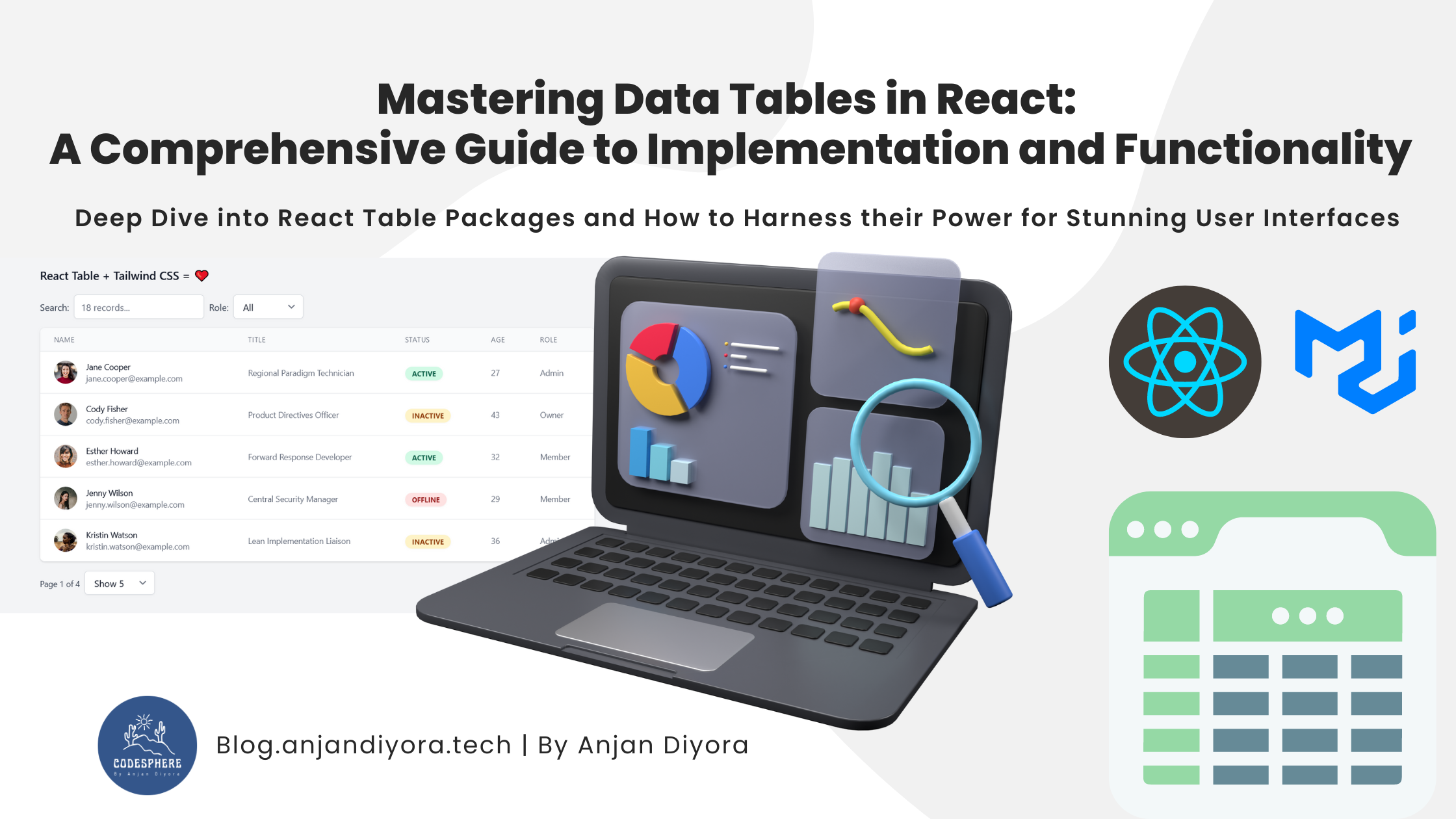
Introduction:
In today's fast-paced world of web development, creating rich and dynamic user interfaces is of paramount importance. One essential component for managing and displaying data is the table. Tables are ubiquitous, providing an organized and structured way to present information. In this blog post, we'll explore the world of data tables in React, from selecting the right package to implementing various functionalities, all while enriching your understanding with code examples and relevant GitHub repositories.
1. Choosing the Right React Table Package
React offers several table packages to choose from, each with its own unique features and benefits. Some popular choices include:
react-table: A flexible and customizable package that provides various functionalities.
- GitHub Repository: react-table
ag-Grid: A powerful package that supports features like sorting, filtering, and grouping.
- GitHub Repository: ag-Grid
Material-UI Data Grid: Part of the Material-UI library, offering a sleek and responsive design.
- GitHub Repository: Material-UI Data Grid
Choose a package based on your project's requirements and your familiarity with the library. For this tutorial, we'll use the react-table
package due to its flexibility and ease of use.
2. Basic Table Implementation in React
Let's start by setting up a basic data table using the react-table
package. Begin by installing the package using npm or yarn:
npm install react-table
# or
yarn add react-table
Next, import the necessary components and create a simple table structure:
import React from 'react';
import { useTable } from 'react-table';
const BasicTable = () => {
const data = [
{ name: 'John Doe', age: 28, city: 'New York' },
{ name: 'Jane Smith', age: 32, city: 'Los Angeles' },
// ...more data
];
const columns = [
{ Header: 'Name', accessor: 'name' },
{ Header: 'Age', accessor: 'age' },
{ Header: 'City', accessor: 'city' },
];
const {
getTableProps,
getTableBodyProps,
headerGroups,
rows,
prepareRow,
} = useTable({
columns,
data,
});
return (
<table {...getTableProps()} className="table">
<thead>
{headerGroups.map(headerGroup => (
<tr {...headerGroup.getHeaderGroupProps()}>
{headerGroup.headers.map(column => (
<th {...column.getHeaderProps()}>{column.render('Header')}</th>
))}
</tr>
))}
</thead>
<tbody {...getTableBodyProps()}>
{rows.map(row => {
prepareRow(row);
return (
<tr {...row.getRowProps()}>
{row.cells.map(cell => {
return <td {...cell.getCellProps()}>{cell.render('Cell')}</td>;
})}
</tr>
);
})}
</tbody>
</table>
);
};
export default BasicTable;
In this example, we've created a basic table with sample data and columns. The useTable
hook from react-table
provides us with essential table properties and methods for rendering the table.
3. Sorting and Filtering Data
Adding sorting and filtering functionality enhances the user experience when dealing with large datasets. Fortunately, react-table
makes this process straightforward. Let's extend our basic table to include sorting and filtering capabilities:
import React from 'react';
import { useTable, useSortBy, useFilters } from 'react-table';
const SortAndFilterTable = () => {
// Same data and columns as before
const {
getTableProps,
getTableBodyProps,
headerGroups,
rows,
prepareRow,
} = useTable(
{
columns,
data,
},
useFilters, // Enable filtering
useSortBy // Enable sorting
);
// ...rest of the code remains unchanged
};
In the above code, we've imported the useSortBy
and useFilters
hooks from react-table
and applied them to our table instance. Now users can click on column headers to sort data and use filter inputs to narrow down results.
4. Pagination and Data Fetching
When dealing with large datasets, pagination is crucial to prevent overwhelming the user. Additionally, you might need to fetch data asynchronously. react-table
supports both features:
import React, { useState, useEffect } from 'react';
import {
useTable,
useSortBy,
useFilters,
usePagination,
} from 'react-table';
const PaginatedTable = () => {
const [data, setData] = useState([]);
const [loading, setLoading] = useState(true);
useEffect(() => {
// Simulate fetching data from an API
setTimeout(() => {
const fetchedData = [
{ name: 'John Doe', age: 28, city: 'New York' },
{ name: 'Jane Smith', age: 32, city: 'Los Angeles' },
// ...more data
];
setData(fetchedData);
setLoading(false);
}, 1000);
}, []);
// Same columns as before
const {
getTableProps,
getTableBodyProps,
headerGroups,
page,
prepareRow,
canPreviousPage,
canNextPage,
nextPage,
previousPage,
state: { pageIndex },
} = useTable(
{
columns,
data,
initialState: { pageIndex: 0 }, // Start at the first page
},
useFilters,
useSortBy,
usePagination // Enable pagination
);
// ...rest of the code remains unchanged
};
In this example, we've added pagination functionality to the table using the usePagination
hook. We've also included an asynchronous data fetching simulation using useState
and useEffect
.
5. Customizing Table Appearance
A well-designed table can significantly improve user experience. react-table
provides options for customizing the appearance of your table. Here's how you can style your table and cells:
import React from 'react';
import { useTable, useSortBy, useFilters } from 'react-table';
const StyledTable = () => {
// Same data and columns as before
const {
getTableProps,
getTableBodyProps,
headerGroups,
rows,
prepareRow,
} = useTable(
{
columns,
data,
},
useFilters,
useSortBy
);
return (
<table {...getTableProps()} className="table">
<thead>
{headerGroups.map(headerGroup => (
<tr {...headerGroup.getHeaderGroupProps()} className="header-row">
{headerGroup.headers.map(column => (
<th {...column.getHeaderProps()} className="header-cell">
{column.render('Header')}
</th>
))}
</tr>
))}
</thead>
<tbody {...getTableBodyProps()} className="body">
{rows.map(row => {
prepareRow(row);
return (
<tr {...row.getRowProps()} className="body-row">
{row.cells.map(cell => {
return (
<td {...cell.getCellProps()} className="body-cell">
{cell.render('Cell')}
</td>
);
})}
</tr>
);
})}
</tbody>
</table>
);
};
export default StyledTable;
In the above example, we've added CSS classes to different parts of the table to enable easy styling. Customize the classes according to your project's design requirements.
6. Handling Cell Editing and Selection
In some cases, you might need to allow users to edit cell values directly within the table. react-table
supports cell editing out of the box. Here's how you can achieve it:
import React from 'react';
import { useTable, useSortBy, useFilters, useCellEdit } from 'react-table';
const EditableTable = () => {
// Same data and columns as before
const {
getTableProps,
getTableBodyProps,
headerGroups,
rows,
prepareRow,
} = useTable(
{
columns,
data,
},
useFilters,
useSortBy,
useCellEdit // Enable cell editing
);
return (
<table {...getTableProps()} className="table">
{/* ... */}
</table>
);
};
export default EditableTable;
By including the useCellEdit
hook, you can enable cell editing within your table. Users can now click on individual cells and modify their contents.
7. Advanced Features and Additional Resources
The functionalities we've covered so far are just the tip of the iceberg. React table packages offer a plethora of advanced features, such as:
Grouping and Aggregation
Tree Data
Row Selection
Cell Formatting
To explore these features and more, you can refer to the official documentation of the chosen table package or explore its GitHub repository.
Here are a few additional resources to further enhance your understanding of data tables in React:
Official
react-table
Documentation: react-table DocumentationGitHub Repository for
react-table
: react-table GitHub Repository"A Complete Guide to React Table" by John Gleeson: Article Link
Expanding Horizons: Advanced Functionalities and Beyond
Welcome back to the third installment of our comprehensive guide to implementing data tables in React. In this segment, we'll delve into advanced functionalities and explore how you can take your data tables to the next level. Let's dive right in!
8. Grouping and Aggregation
Sometimes, your data may be better presented in a grouped and aggregated format. This is particularly useful when dealing with datasets that have hierarchical or categorical relationships. The react-table
package provides a convenient way to achieve this:
import React from 'react';
import { useTable, useGroupBy, useAggregation } from 'react-table';
const GroupedTable = () => {
// Same data and columns as before
const {
getTableProps,
getTableBodyProps,
headerGroups,
rows,
prepareRow,
} = useTable(
{
columns,
data,
},
useGroupBy, // Enable grouping
useAggregation // Enable aggregation
);
return (
<table {...getTableProps()} className="table">
{/* ... */}
</table>
);
};
export default GroupedTable;
By including the useGroupBy
and useAggregation
hooks, you can transform your table into a powerful tool for presenting summarized data.
9. Tree Data and Hierarchy
In scenarios where your data has a hierarchical structure, such as a file system or organizational chart, representing it in a tree format is crucial. react-table
supports this seamlessly:
import React from 'react';
import { useTable, useGroupBy, useExpanded } from 'react-table';
const TreeTable = () => {
// Same data and columns as before, with additional parent-child relationships
const {
getTableProps,
getTableBodyProps,
headerGroups,
rows,
prepareRow,
} = useTable(
{
columns,
data,
},
useGroupBy,
useExpanded // Enable tree data
);
return (
<table {...getTableProps()} className="table">
{/* ... */}
</table>
);
};
export default TreeTable;
Through the useExpanded
hook, you can effortlessly create visually appealing tree structures that bring your data to life.
10. Row Selection and Actions
Allowing users to select rows and perform actions on them is a common requirement. react-table
simplifies this process:
import React from 'react';
import { useTable, useRowSelect } from 'react-table';
const SelectableTable = () => {
// Same data and columns as before
const {
getTableProps,
getTableBodyProps,
headerGroups,
rows,
prepareRow,
selectedFlatRows,
} = useTable(
{
columns,
data,
},
useRowSelect // Enable row selection
);
const handleDeleteSelected = () => {
const selectedRows = selectedFlatRows.map(row => row.original);
// Implement your delete logic here
};
return (
<div>
<button onClick={handleDeleteSelected}>Delete Selected</button>
<table {...getTableProps()} className="table">
{/* ... */}
</table>
</div>
);
};
export default SelectableTable;
With the useRowSelect
hook, users can select rows and perform actions on them, offering enhanced interactivity.
11. Cell Formatting and Custom Renderers
Sometimes, you need to present data in a visually appealing or informative way. Custom cell rendering allows you to achieve this:
import React from 'react';
import { useTable } from 'react-table';
const FormattedTable = () => {
// Same data and columns as before
const {
getTableProps,
getTableBodyProps,
headerGroups,
rows,
prepareRow,
} = useTable({
columns: [
{ Header: 'Name', accessor: 'name' },
{
Header: 'Age',
accessor: 'age',
Cell: ({ value }) => <span>{value} years</span>,
},
{
Header: 'City',
accessor: 'city',
Cell: ({ value }) => (
<span style={{ textTransform: 'uppercase' }}>{value}</span>
),
},
],
data,
});
return (
<table {...getTableProps()} className="table">
{/* ... */}
</table>
);
};
export default FormattedTable;
Through the Cell
property, you can define custom renderers to display data exactly as you envision.
Optimizing Performance and Ensuring Accessibility
Welcome to the concluding segment of our comprehensive guide to data tables in React. In this final part, we'll delve into essential topics related to performance optimization, accessibility, and further resources to expand your expertise. Let's wrap up our journey on a high note!
12. Performance Optimization
As your data tables grow in size and complexity, ensuring optimal performance becomes critical. Here are a few strategies to keep in mind:
Virtualization:
Virtualization techniques, such as windowing or infinite scrolling, can drastically improve performance by rendering only the visible rows of your table. Libraries like react-virtualized
and react-window
are excellent choices for achieving this.
Memoization:
Use memoization techniques to prevent unnecessary re-renders of components. Libraries like memoize-one
or the useMemo
hook in React can help you achieve this.
Data Pagination:
Implement client-side or server-side data pagination to limit the number of rows rendered at once. This prevents excessive rendering and improves user experience.
13. Accessibility Considerations
Creating accessible data tables is crucial to ensure that all users, including those with disabilities, can effectively interact with your application. Follow these guidelines:
Semantic HTML:
Use semantic HTML elements (<table>
, <th>
, <tr>
, <td>
) to create a well-structured table that screen readers can interpret correctly.
ARIA Roles:
Add appropriate ARIA roles and attributes to enhance accessibility. For example, use role="rowheader"
for header cells and role="cell"
for data cells.
Keyboard Navigation:
Ensure that users can navigate and interact with the table using keyboard shortcuts. Implement focus management to help users understand their location within the table.
14. Additional Resources
To continue expanding your knowledge and honing your skills in data tables and React, here are some valuable resources:
Official Documentation and Repositories:
react-table
Documentation: https://react-table.tanstack.com/react-table
GitHub Repository: https://github.com/tannerlinsley/react-table
Performance Optimization Libraries:
react-virtualized
: https://github.com/bvaughn/react-virtualizedreact-window
: https://github.com/bvaughn/react-window
Accessibility Guidelines:
Web Content Accessibility Guidelines (WCAG): https://www.w3.org/WAI/standards-guidelines/wcag/
ARIA Authoring Practices: https://www.w3.org/TR/wai-aria-practices-1.2/
Conclusion
Congratulations! You've successfully navigated through the exciting realm of data tables in React. From selecting the right package to implementing advanced functionalities and ensuring accessibility, you're now equipped to create stunning, interactive, and user-friendly data tables that elevate your web applications to new heights.
Remember, mastering data tables is an ongoing journey. As technology evolves, new techniques and tools may emerge, enabling you to push the boundaries of what's possible. Keep exploring, experimenting, and refining your skills to deliver exceptional user experiences.
I hope this comprehensive guide has provided you with a solid foundation and inspiration to create remarkable data tables in your React projects. Feel free to revisit these articles, experiment with the code examples, and continue your learning journey. Happy coding, and may your data tables shine brightly in the digital landscape!
Subscribe to my newsletter
Read articles from Anjan Diyora (AD) directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
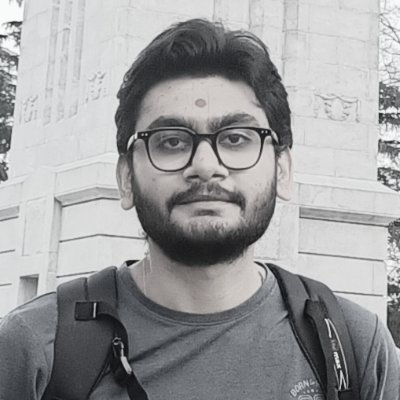
Anjan Diyora (AD)
Anjan Diyora (AD)
๐ Software Engineer ๐ Crafting cutting-edge software and network solutions that integrate AI seamlessly. Let's innovate together! ๐ป๐ง ๐