Understanding the Façade Design Pattern in C#
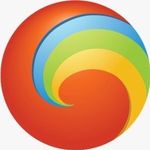
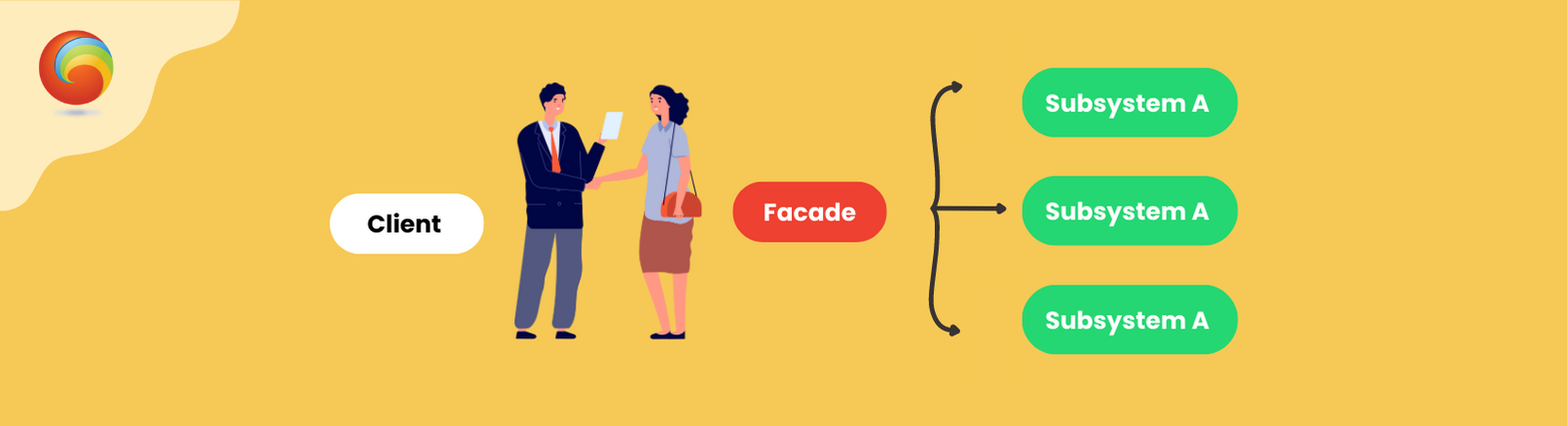
In software development, managing complex systems can be a daunting task. As systems grow larger and more intricate, it becomes challenging to maintain a clear and understandable codebase. This is where design patterns come to the rescue.
One such pattern is the Façade Design Pattern, which provides a simple interface to a complex subsystem, making it easier to use and understand.
In this blog post, we will explore the Façade Design Pattern in C# and see how it simplifies complex systems.
What is the Façade Design Pattern?
The Façade Design Pattern is a structural design pattern that provides a unified interface to a set of interfaces in a subsystem. It acts as a high-level interface that simplifies the use of complex subsystems by providing a single-entry point. The pattern encapsulates the complexity of the subsystem and provides clients with a straightforward interface to interact with.
When to Use the Façade Design Pattern?
The Façade Design Pattern in C# is beneficial when working with complex systems or libraries. It is particularly useful in the following scenarios:
Simplifying complex subsystems: When a system has a large number of components with intricate relationships, the Façade Design Pattern can provide a simplified interface for client code.
Decoupling clients from subsystems: By introducing a Façade, client code is decoupled from the detailed implementation of subsystems, allowing for easier maintenance and code evolution.
Providing a higher-level interface: The Façade Design Pattern can abstract away low-level details and provide a higher-level interface tailored to the specific needs of the clients.
Understanding the Structure of the Façade Design Pattern
The Façade Design Pattern consists of three main components:
Façade: This is the central class that provides a simplified interface to the subsystem. It delegates client requests to appropriate objects within the subsystem.
Subsystem: The subsystem represents a set of classes or components that work together to perform a specific task. The Façade interacts with the subsystem on behalf of the client.
Client: The client code interacts with the Façade to perform operations on the subsystem. It does not need to be aware of the complex internal structure of the subsystem.
Implementing the Façade Design Pattern in C#
To implement the Façade Design Pattern in C#, follow these steps:
Identify a complex subsystem that could benefit from a simplified interface.
Create a Façade class that encapsulates the subsystem and provides a simple interface to the clients.
Define methods in the Façade class that delegate calls to appropriate subsystem objects.
Clients can now interact with the subsystem through the Façade, without needing to understand its internal details.
Here’s a simple example of the Façade Design Pattern in C#:
In this diagram, we have three main components:
Client: Represents the class that interacts with the facade.
Façade: Acts as an interface to the subsystems and simplifies their usage. It encapsulates the creation and management of the subsystem objects.
Subsystem: Represents the complex operations that are encapsulated by the facade. It may consist of multiple classes or have a single class with multiple methods.
To study more about Façade Design along with the code for the above-mentioned example click here!
Here we explore the concept of the Façade Design Pattern in C# and learn how it can improve the maintainability and readability of our code. By adopting this pattern, developers can create more modular, decoupled, and easy-to-understand software systems.
Thank you for reading!
Subscribe to my newsletter
Read articles from Swabhav Techlabs directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
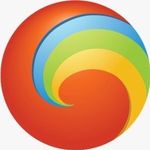
Swabhav Techlabs
Swabhav Techlabs
Swabhav Techlabs is a tech talent solutions company. We like to: Create value through talent and product creation. Build a community of people passionate about problem-solving and programming. Accelerate growing companies by building high-performing engineering teams for them. Ways We Create Value: Product Creation: Building a MVP by understanding the company vision, product purpose, selecting the right teck, chalking a roadmap, finding the best people & mentoring them. Talent Creation: Planning crazy ambitious hires and pulling them off by conducting skill gap analysis, working out the future openings, finding & mentoring them. Scouting and Mentor Young Product Teams: Developing your talent workforce of young tech graduates with our team of TA to scout and mentors to teach your tech stack. Scouting on Demand: Working on your live requirements to find senior engineering talent