Sending Emails with Node.js and Nodemailer: A Complete Guide
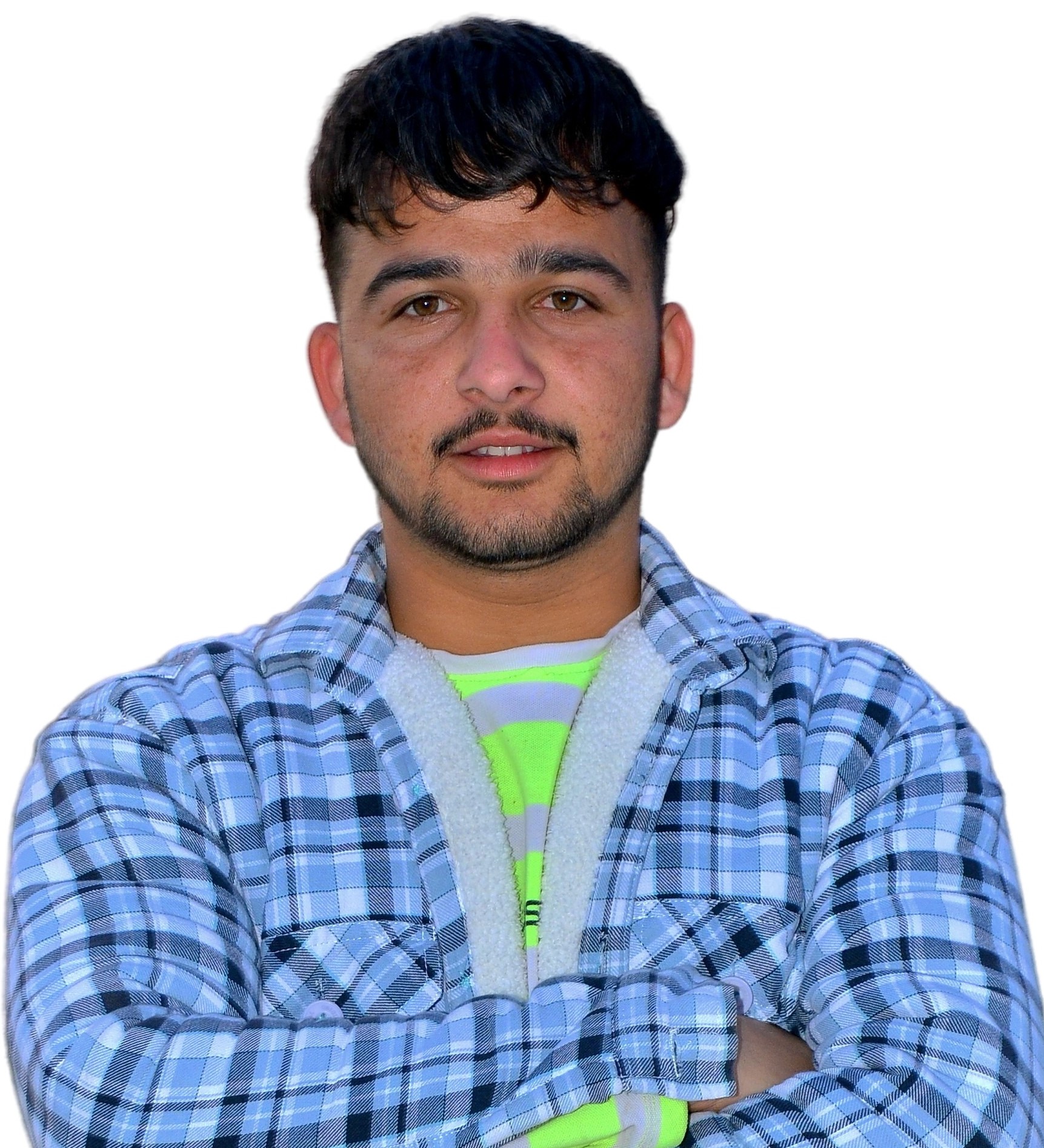

Introduction
Sending emails programmatically is an essential feature for many web applications. Whether it's sending account verification emails, notifications, or updates, the ability to send emails from your Node.js application is crucial. In this comprehensive guide, we'll explore how to use Nodemailer, a powerful and popular Node.js module, to send emails using the Gmail service with OAuth2 authentication. By the end of this blog, you'll have a solid understanding of how to integrate email functionality into your projects.
Prerequisites
Before diving into the code, make sure you have the following prerequisites in place:
A Gmail account: You'll need a Gmail account to send emails using the Gmail service.
Node.js: Make sure you have Node.js installed on your system to run the Node.js application.
Nodemailer: Install Nodemailer, a Node.js module, by running
npm install nodemailer
in your project directory.
The Code: Sending Emails with Nodemailer
Let's breakdown the code snippet which demonstrates how to set up a Node.js email transporter using Nodemailer:-
// Import the required modules
const nodemailer = require("nodemailer");
// Create a transporter
let transporter = nodemailer.createTransport({
service: "gmail",
auth: {
type: "OAuth2",
user: process.env.EMAIL_USER,
pass: process.env.EMAIL_PASSWORD,
clientId: process.env.OAUTH_CLIENTID,
clientSecret: process.env.OAUTH_CLIENT_SECRET,
refreshToken: process.env.OAUTH_REFRESH_TOKEN,
},
});
// Function to send emails
function send_mail(otp, mail_value, name_value) {
var mailOptions = {
from: process.env.EMAIL_USER,
to: mail_value,
subject: "Email for Verification",
text: `Hello ${name_value}
You registered an account on PiperChat. Here is your OTP for verification: ${otp}
Kind Regards, Sunil Kumar`,
};
// Send the email
transporter.sendMail(mailOptions, (error, info) => {
if (error) {
console.error("Error sending email:", error);
} else {
console.log("Email sent:", info.response);
}
});
}
Understanding the code
Let's explore the key components of the code snippet:-
Transporter Configuration: The
nodemailer.createTransport()
function is used to create a transporter object. Here, we configure the transporter to use the Gmail service for sending emails, and we use 0Auth2 authentication for enhanced security.send_mail Function:- This function takes three parameters:
otp
(One-Time Password),mail_value
(recipient's email address), andname_value
(recipient's name). Inside the function, we define amailOptions
is an object that includes the sender's email address, the recipient's email address, the subject, and the email body (which includes the OTP and a friendly message).Sending the Emails:- We use the
transporter.sendMail()
method to send the email using the specifiedmailOptions
. The code also includes error handling to log any errors that might occur during the email-sending process.
Putting All It Together
To use this code in your project, follow these steps:
Make sure you have the required environment variables set up (EMAIL_USER, EMAIL_PASSWORD, OAUTH_CLIENTID, OAUTH_CLIENT_SECRET, OAUTH_REFRESH_TOKEN). These are sensitive credentials, so handle them securely.
Integrate the
send_mail
function into your project. You can call this function whenever you need to send an email. For example, you can use it to send verification emails during user registration.Test the email functionality in your application. Verify that emails are being sent successfully and that any errors are properly handled.
Conclusion
By following this guide, you've learned how to use Nodemailer to send emails from your Node.js application using the Gmail service and OAuth2 authentication. This knowledge can be incredibly valuable for adding email functionality to your projects, enhancing user experiences, and improving communication with your users.
Remember to handle your email credentials and tokens securely and consider error handling to ensure your email-sending process is robust. Now that you have the tools, go ahead and integrate email functionality into your projects with confidence!
This article was inspired by a project I created that uses Nodemailer.
Subscribe to my newsletter
Read articles from Sunil Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
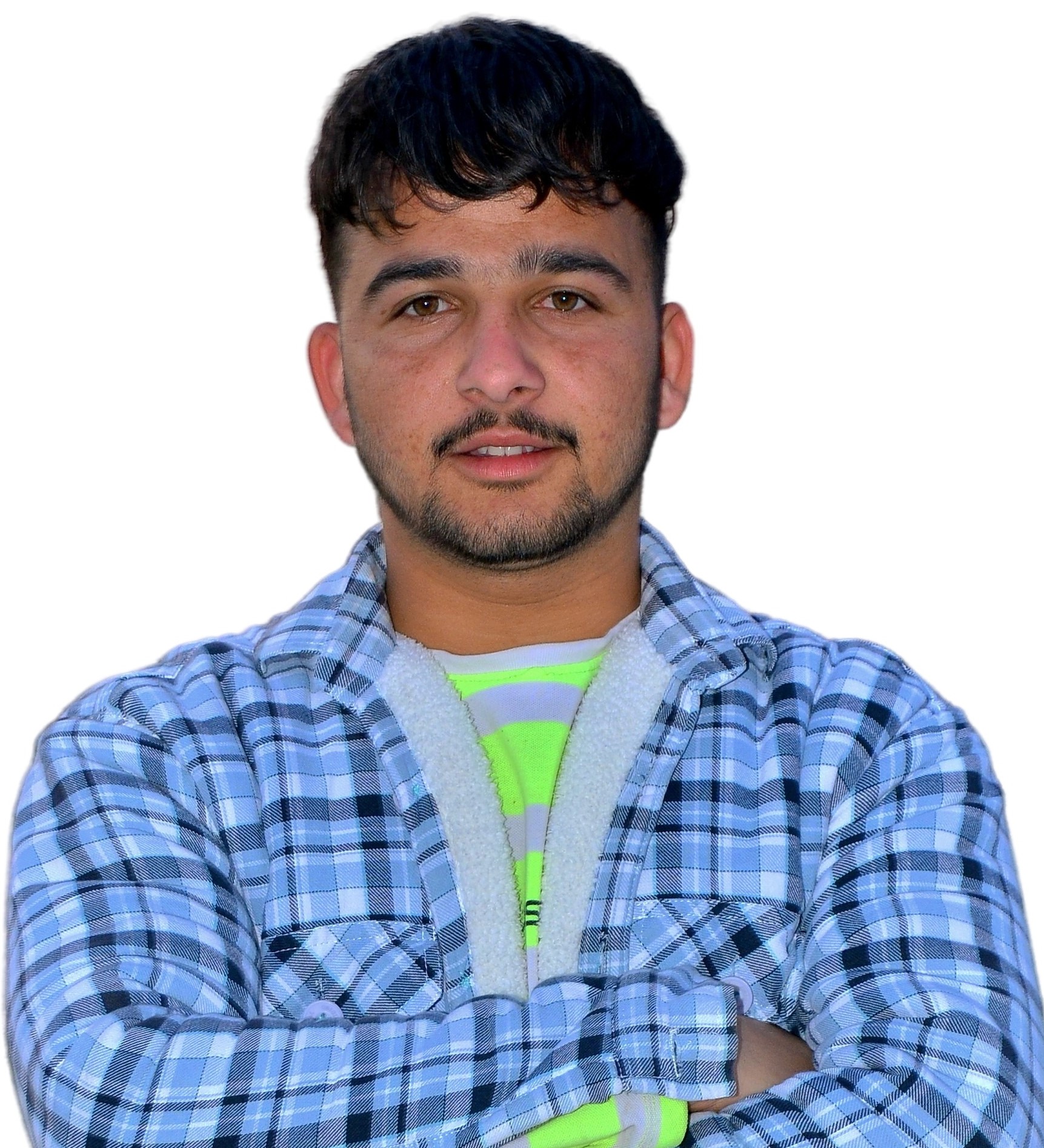
Sunil Kumar
Sunil Kumar
Teaching myself how to code