Demystifying JavaScript's Single-Threaded Nature: Exploring setTimeout and Escaping Callback Hell
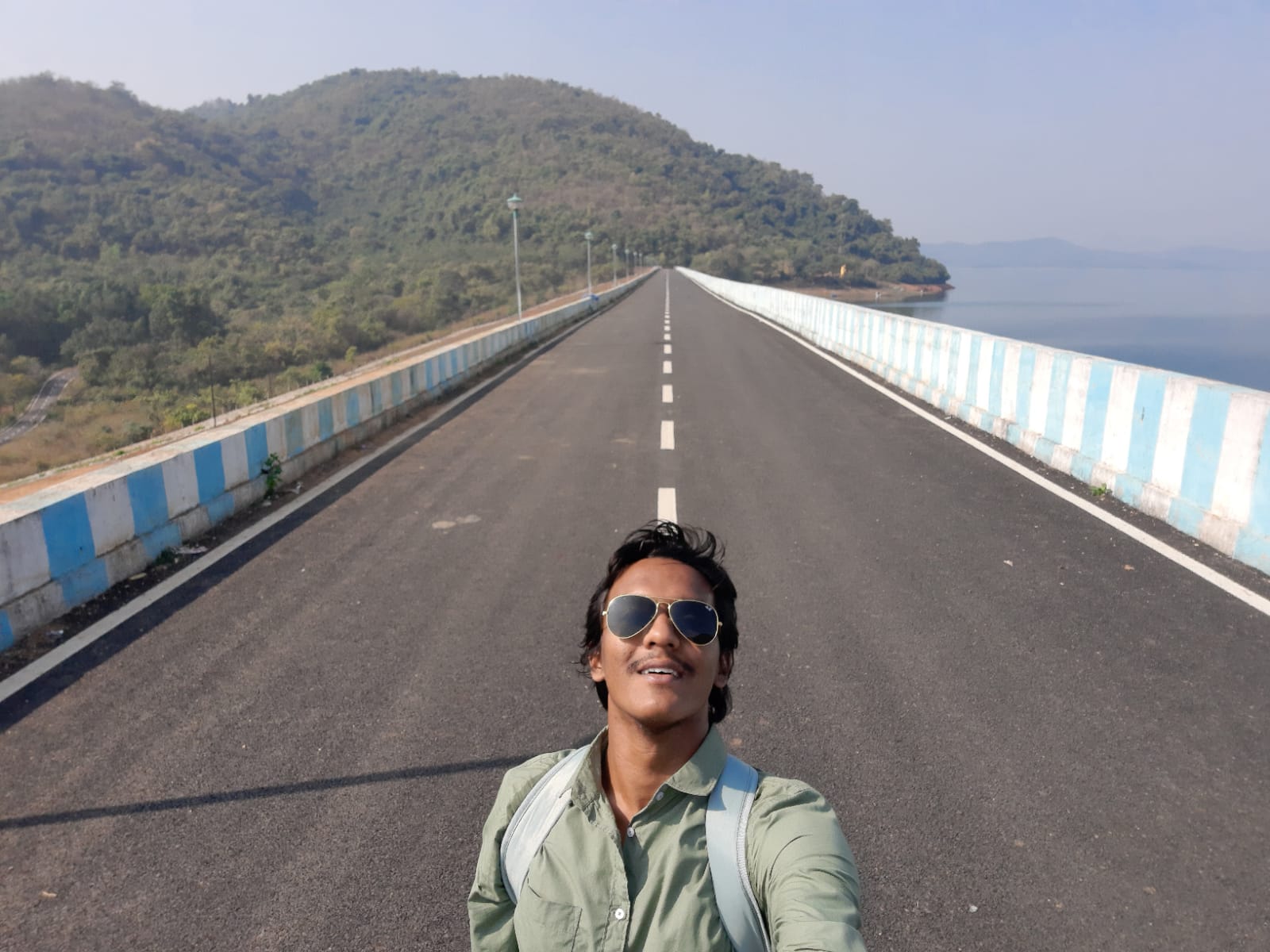
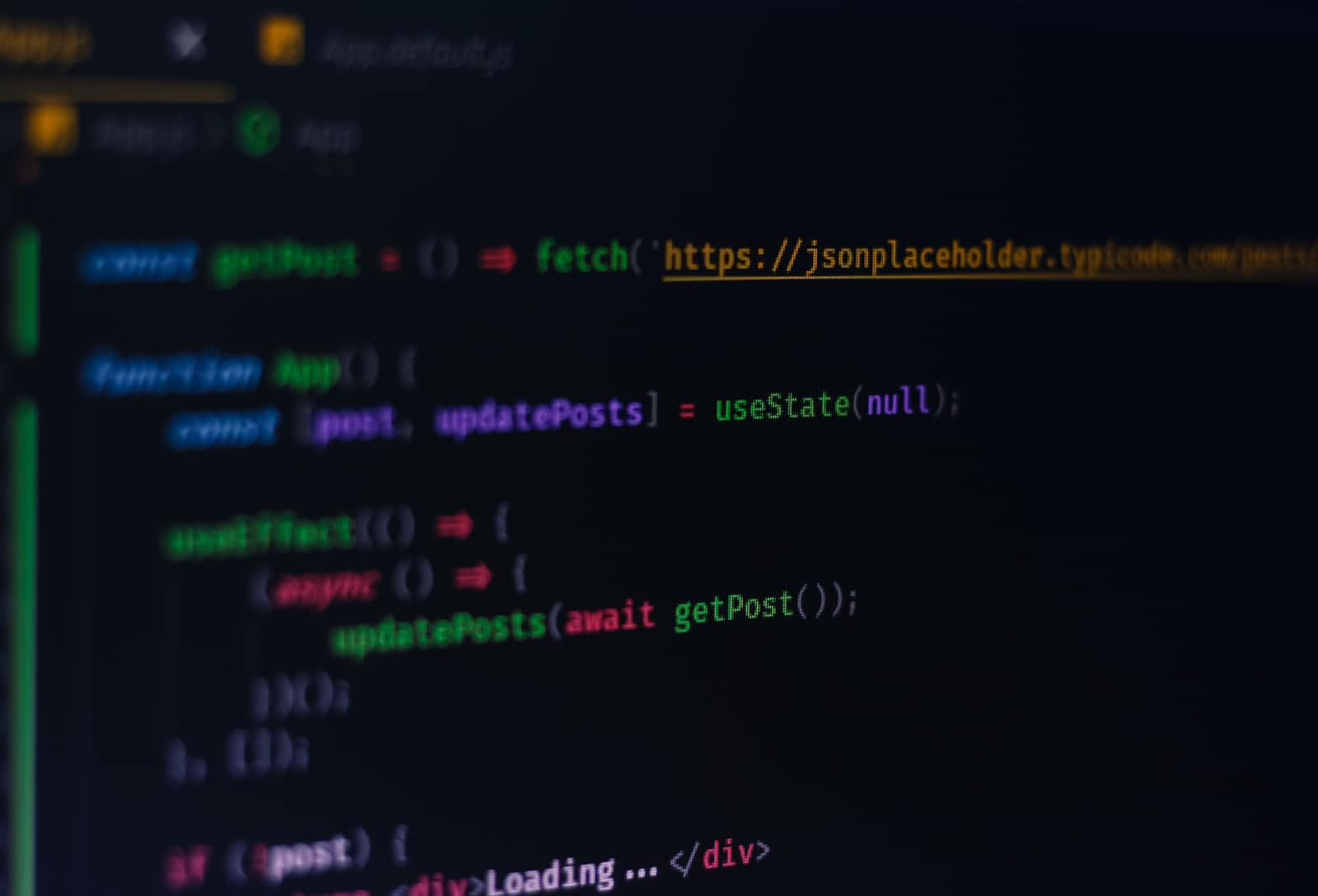
Introduction:
JavaScript, a versatile and widely-used programming language, is often praised for its asynchronous and non-blocking behaviour. One of its key characteristics is its single-threaded execution model, which means that it processes one task at a time. However, seemingly contradictory to this model, we have functions like setTimeout
that allows us to perform tasks after a specified delay. Moreover, we'll delve into the notorious "callback hell" and discover strategies to escape its complexity.
Understanding JavaScript's Single-Threaded Execution:
JavaScript's single-threaded execution does not mean it can't perform multiple tasks simultaneously. It simply means that it sequentially executes tasks, handling one task at a time. This single thread is responsible for executing the code, handling events, and managing asynchronous operations.
Exploring the Magic of setTimeout:
Mechanism of setTimeout:
Despite being single-threaded, JavaScript leverages an event loop to manage asynchronous operations. The setTimeout
function is a prime example of this mechanism. When setTimeout
is called, it sets a timer and schedules the specified function to be executed after the timer expires. However, during the wait, JavaScript's event loop remains active, allowing other tasks to be processed.
Concurrent Execution with setTimeout:
The apparent "concurrent" execution of multiple setTimeout
calls are achieved by leveraging the event loop. While each setTimeout
callback is executed one after the other, the event loop ensures that other tasks can be interleaved in between.
Escaping Callback Hell:
What is Callback Hell?
Callback hell, also known as the "pyramid of doom," is a situation where deeply nested callbacks make the code unreadable, difficult to maintain, and prone to errors. This occurs when dealing with asynchronous operations and multiple layers of callbacks.
Strategies to Avoid Callback Hell:
1. Use Promises:
Promises provide a cleaner and more structured way to handle asynchronous operations. They allow you to chain operations and handle errors more elegantly.
async function fetchData() {
try {
const data = await fetchSomeData();
const processedData = await processData(data);
return processedData;
} catch (error) {
console.error('An error occurred:', error);
}
}
2. Embrace Async/Await:
Async/await syntax simplifies asynchronous code even further by making it look more like synchronous code. It enhances readability and maintainability.
Async Functions
: Anasync
function is a special type of function that is defined using theasync
keyword before the function keyword. It allows you to use theawait
keyword within the function body to pause the execution of the function until an asynchronous operation completes.
async function fetchData() {
// Asynchronous operations using await
const data = await fetchSomeData();
const processedData = await processData(data);
return processedData;
}
2.Awaiting Promises
: The await
keyword can be used only inside an async
function and is used to pause the execution of the function until the awaited Promise is resolved. When the Promise is resolved, the value it resolves with is returned from the await
expression.
async function exampleAsyncFunction() {
const result = await somePromiseFunction();
console.log(result);
}
3.Concurrent Execution
: While await
makes asynchronous code appear synchronous within a single function, it doesn't change the single-threaded nature of JavaScript. Multiple async
functions can still run concurrently, and you can use them to orchestrate complex asynchronous workflows.
async function fetchAndProcessData() {
const [data1, data2] = await Promise.all([fetchData1(), fetchData2()]);
const combinedData = processData(data1, data2);
return combinedData;
}
4.Error Handling
: You can handle errors in async/await
functions using try/catch
blocks, just like you would in synchronous code.
async function fetchData() {
try {
const data = await fetchSomeData();
const processedData = await processData(data);
return processedData;
} catch (error) {
console.error('An error occurred:', error);
}
}
4.Chaining Async Functions
: async/await
can also be used in combination with Promise chaining to create more sophisticated asynchronous sequences.
async function fetchDataAndProcess() {
const data = await fetchSomeData();
return process(data);
}
async function main() {
try {
const result = await fetchDataAndProcess();
console.log(result);
} catch (error) {
console.error('An error occurred:', error);
}
}
main();
3. Modularize Code:
Break down your code into smaller, reusable functions. This not only helps in avoiding callback nesting but also improves code organization.
// todoService.js - Manages the to-do items
const todos = [];
function addTodo(todo) {
todos.push(todo);
}
function getTodos() {
return todos;
}
export { addTodo, getTodos };
// ui.js - Handles UI interactions
import { addTodo, getTodos } from './todoService';
function displayTodos() {
const todos = getTodos();
// Display todos in the UI
}
// app.js - Main application logic
import { addTodo } from './todoService';
import { displayTodos } from './ui';
addTodo('Buy groceries');
displayTodos();
In this example, we've modularized the code into three separate modules: todoService
, ui
, and app
. Each module handles a specific aspect of the application, such as managing to-do items, UI interactions, and the main application logic.
By modularizing the code, we've achieved better organization, improved readability, and the ability to work on different aspects of the application independently.
4. Use Libraries:
Leveraging libraries like Async.js and Bluebird can provide you with a toolkit to manage asynchronous operations effectively and avoid callback hell. These libraries introduce structured patterns and utilities that simplify the flow of your code, leading to cleaner, more maintainable, and less error-prone code.
Async.js:
Async.js is a popular library that provides a set of powerful functions to handle asynchronous operations. It allows you to manage tasks in series or parallel, control flow, and handle errors gracefully. Some key functions include async.series
, async.parallel
, and async.waterfall
.
Example usage of async.series
:
const async = require('async');
async.series([
function(callback) {
// Task 1
callback(null, 'Result 1');
},
function(callback) {
// Task 2
callback(null, 'Result 2');
}
], function(err, results) {
if (err) {
console.error('An error occurred:', err);
} else {
console.log('Results:', results);
}
});
Bluebird :
Bluebird is a Promise library that enhances the native Promise implementation with additional features and utilities. It offers advanced error handling, cancellation, and performance optimization. Bluebird's Promise.map
and Promise.each
are particularly useful for managing arrays of asynchronous operations.
Example usage of Bluebird's Promise.map
:
const Promise = require('bluebird');
const data = [1, 2, 3, 4];
Promise.map(data, async (item) => {
const result = await processItem(item);
return result;
})
.then(results => {
console.log('Results:', results);
})
.catch(error => {
console.error('An error occurred:', error);
});
Conclusion:
JavaScript's single-threaded nature does not prevent it from handling asynchronous tasks effectively. The setTimeout
function, powered by the event loop, allows tasks to be scheduled and interleaved, creating a sense of concurrency. To steer clear of callback hell, developers can adopt strategies like using Promises, async/await, modularization, and libraries designed for managing asynchronous code. By mastering these concepts, developers can write cleaner, more maintainable, and less error-prone JavaScript code.
So, there you have it โ a deeper dive into JavaScript's single-threaded nature, the fascinating world of setTimeout
, and techniques to rescue yourself from the clutches of callback hell. Happy coding! ๐
Subscribe to my newsletter
Read articles from Debasmit Biswal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
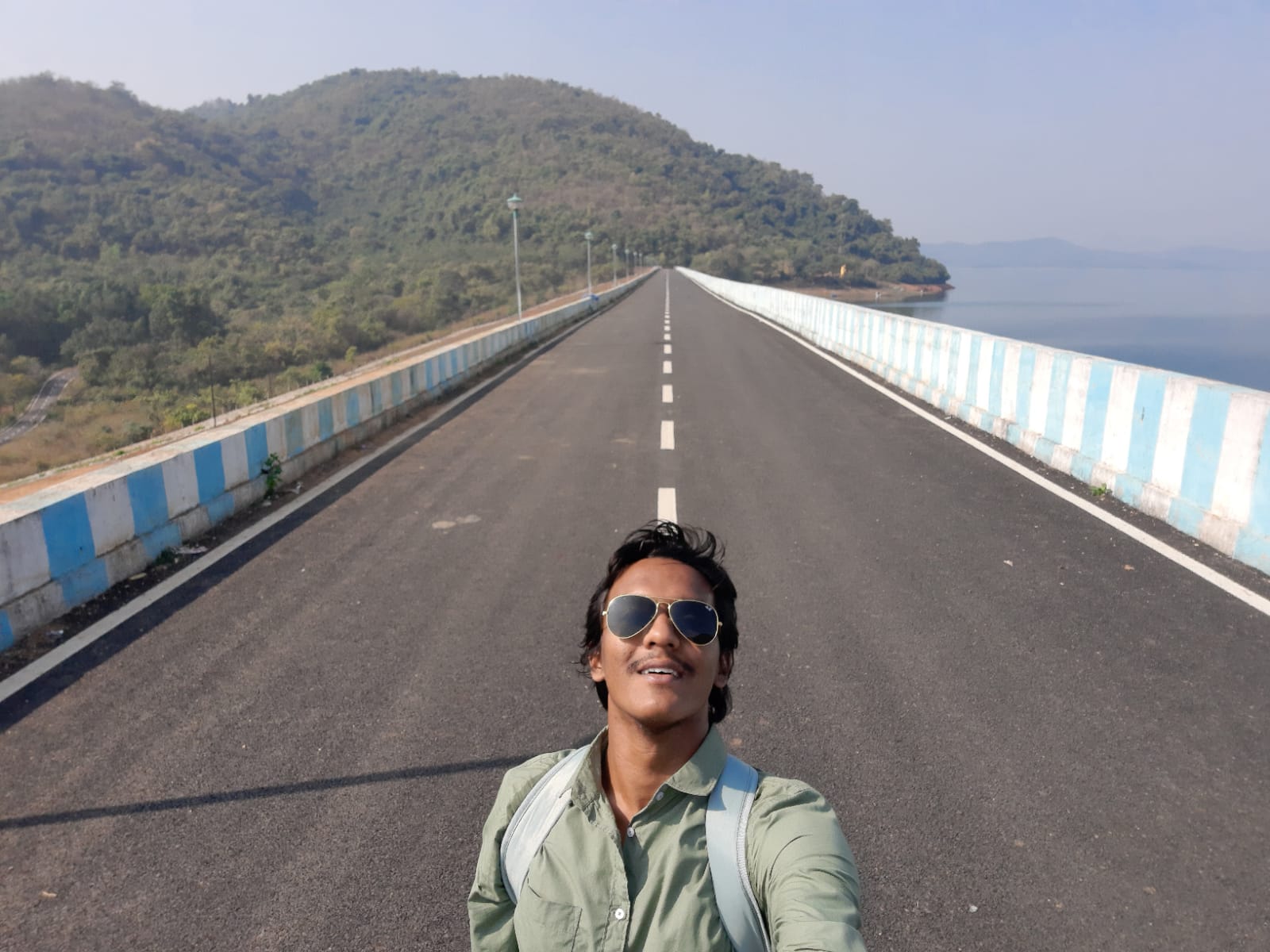
Debasmit Biswal
Debasmit Biswal
I am currently open for SDE roles