Passing props between parent and child components in ReactJS bidirectionally.
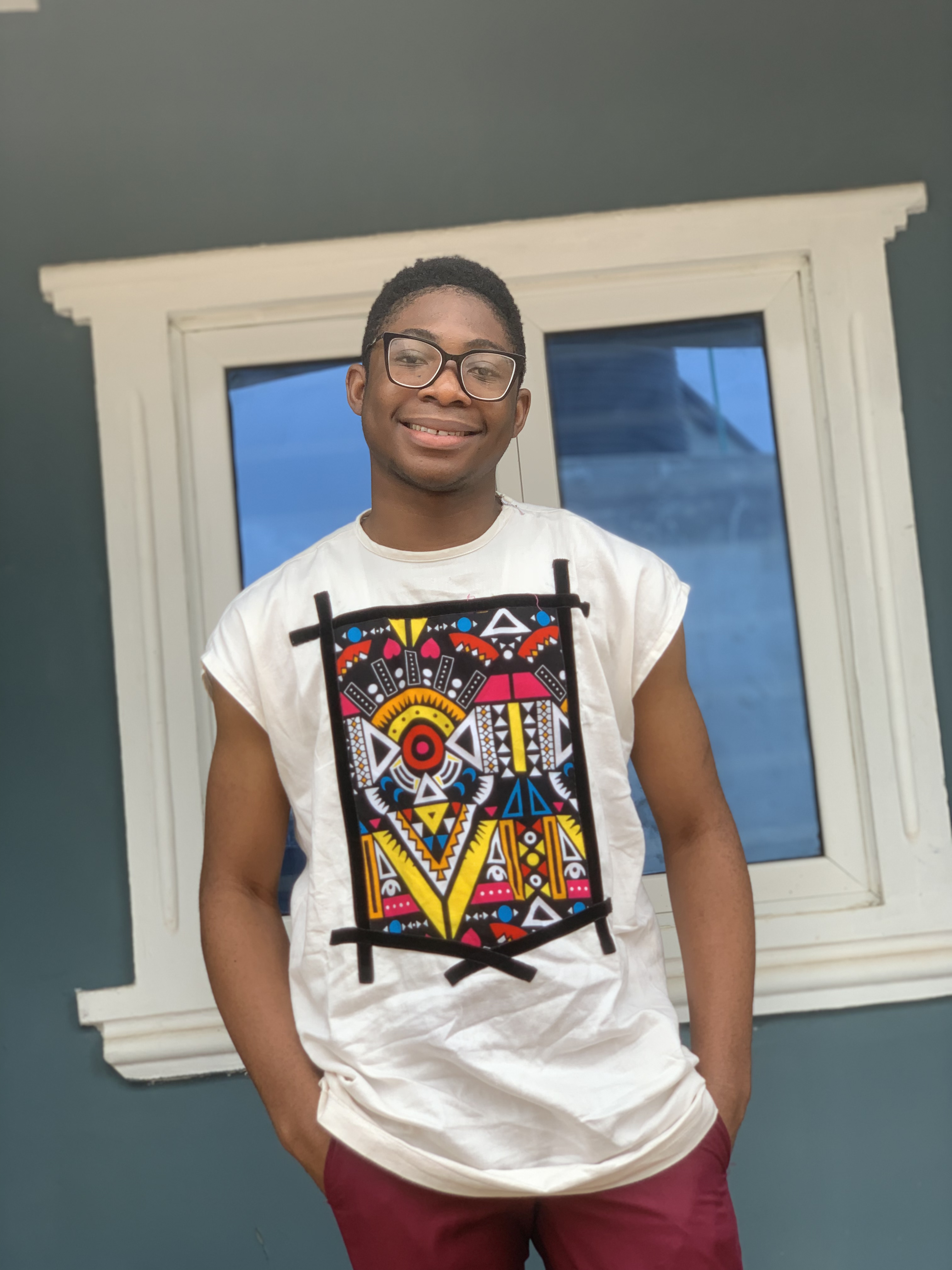
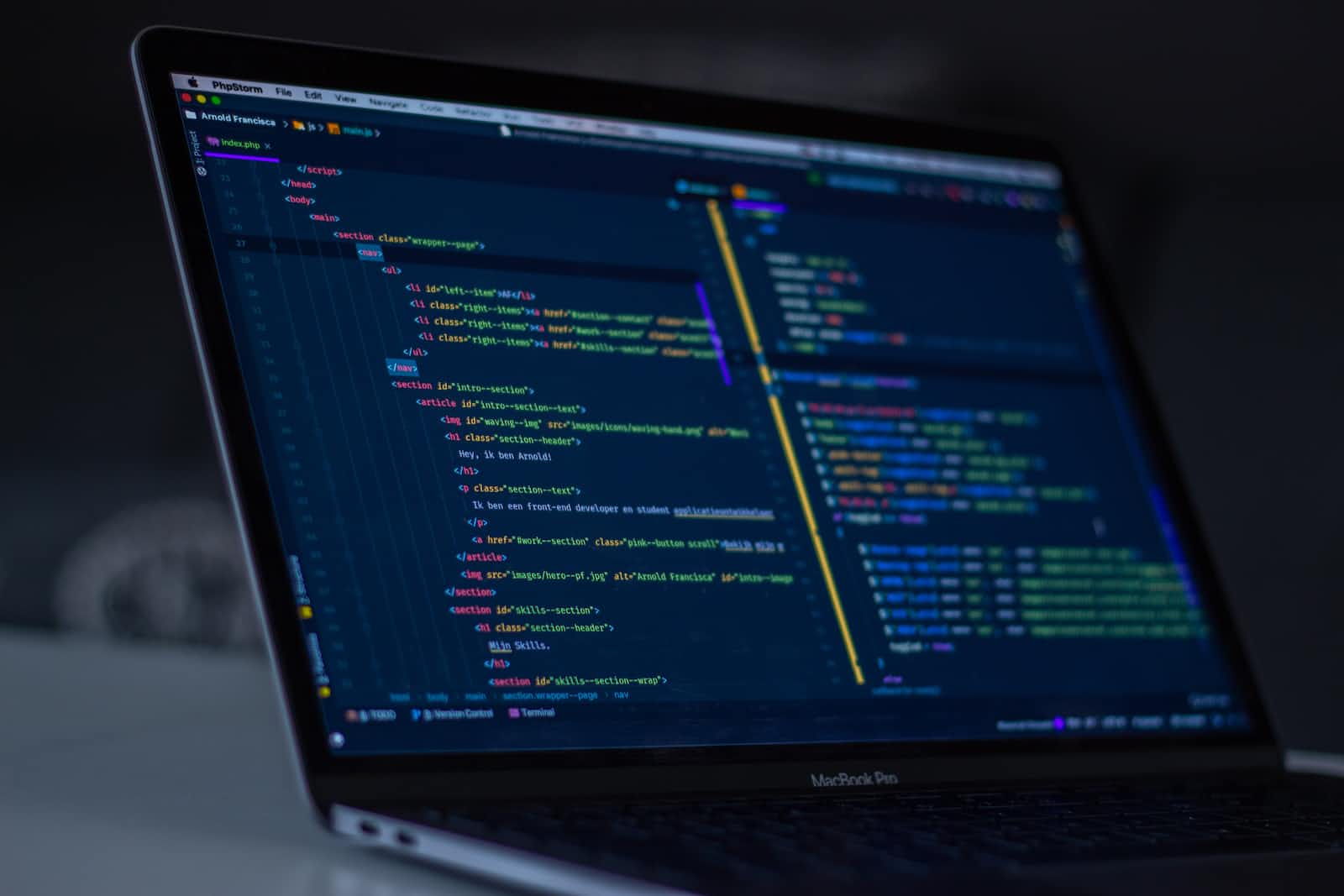
What are Props?
Just like its name props refer to the term properties, props help to provide dynamic values to a component. With props you can change the values or content of your code whenever you reuse a component. It makes working with ReactJS flexible.
As interesting as this sounds, there is a little downside to it, props are read-only, that is, they can't be modified by the receiving component. It is intended to be a one-way mechanism that is it can only be sent in one direction - Parent component to Child component.
However, it is possible to move in the opposite flow, which will also be covered in this article. We will be looking at the following :
Passing props from parent to child component
Passing props from child to parent component (not recommended)
Introduction to Contex
Passing props from Parent to Child Component
When passing props from parent to child component, the prop is defined in the parent component where the child component is rendered and can then be passed as properties to the child component. Here is what it looks like:
Passing the prop (Parent Component)
// ParentComponent.js
import React from 'react';
import ChildComponent from './ChildComponent';
function ParentComponent() {
const message = "Welcome to Olufikayomi's Blog";
return <ChildComponent messageProp={message} />;
}
export default ParentComponent;
In the above code (Parent component), the prop being passed is the "Welcome to Olufikayomi's Blog" stored in the message variable, the variable is then passed as the messageProp property value.
Receiving the prop (Child Component)
// ChildComponent.js
import React from 'react';
function ChildComponent(props) {
return <p>{props.messageProp}</p>;
}
export default ChildComponent;
In the above code (Child Component), the props keyword is passed as a parameter in the function, to access the prop sent props.messageProp is used.
In the above example, we just passed a prop from the parent component to the child component successfully ๐๐
Passing props from child to parent component (not recommended)
Although not recommended, it is very much possible to pass values from a child component to a parent component. The main idea is to pass a function as the prop's value. Here is an example :
Receiving the prop (Parent Component)
//ParentComponent.js
import ChildComponent from "../components/ChildComponent";
import {useState} from "react";
function ParentComponent() {
const [comment, setComment] = useState("unsubscribed");
function HandleComment(message) {
setComment(message);
}
return (
<>
<ChildComponent commentProp={HandleComment} />
<p>{comment}</p>
</>
);
}
export default ParentComponent;
In the above code (Parent component), the prop value being passed is the HandleComment function that receives a message parameter, which is then saved in the comment variable*,* with the help of the useState hook. In the <p> tag the value of the comment is displayed.
Right now no changes have been made to the comment, so it currently displays the initial value of the state "unsubscribed".
Sending the prop (Child Component)
// ChildComponent.js
import React from "react";
function ChildComponent(props) {
function HandleSubscription() {
props.commentProp("subscribe");
}
return <button onClick={HandleSubscription}>Subscribe</button>;
}
export default ChildComponent;
In the above code (Child Component), there is a subscribe button that runs an HandleSubscription function when clicked. In the function, the commentProp prop is called and the string "subscribe" is passed.
NOTE: The only reason it's being called as a method is that the value passed to the prop is a function, the function also receives a parameter- message, in this example, when the function runs the **string "**subscribe" is what is passed as the parameter of the HandleComment function.
In the above example, we just passed a prop from the child component to the parent component successfully ๐๐
Elevating State Management: Context API and Redux Efficiently Sharing Data Across React Components
When it comes to managing and sharing data across components in a React application, two popular options stand out: the Context API and Redux. These tools provide powerful mechanisms for maintaining state and facilitating communication between components, making your application more organized and efficient. The Context API offers a built-in way to share states between components without the need for prop drilling, while Redux provides a centralized store for state management, enabling a predictable state container. Both approaches have their strengths and are widely adopted in the React community. Stay tuned for my next article, where we'll dive deeper into the Context API and explore how it can streamline your data management in React applications.
Subscribe to my newsletter
Read articles from Olufikayomi Jetawo directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
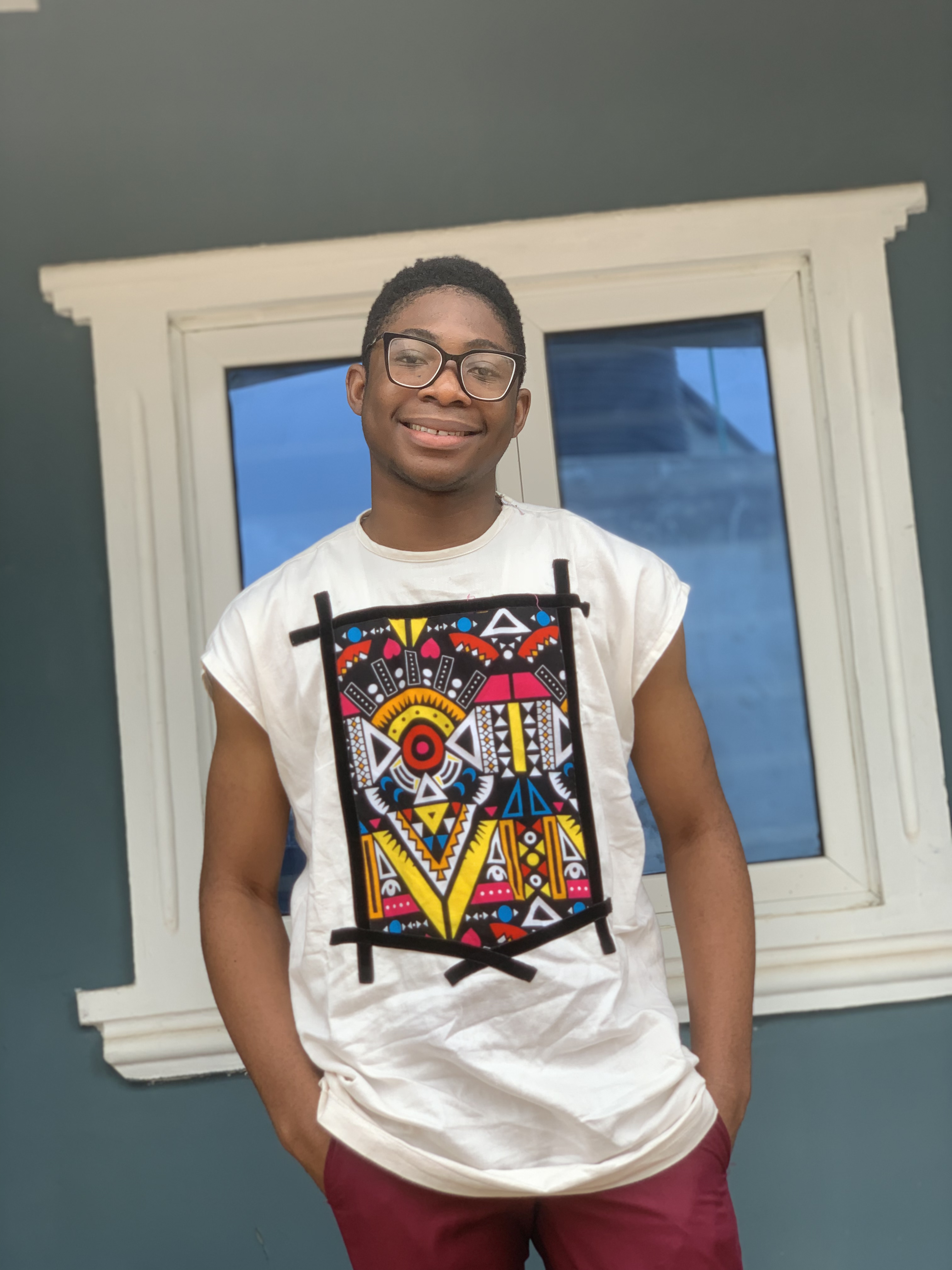
Olufikayomi Jetawo
Olufikayomi Jetawo
I am a Full-stack Developer, with four (4) years of experience, passionate about building beautiful, functional and user-friendly websites.