How to make a Timer in Python
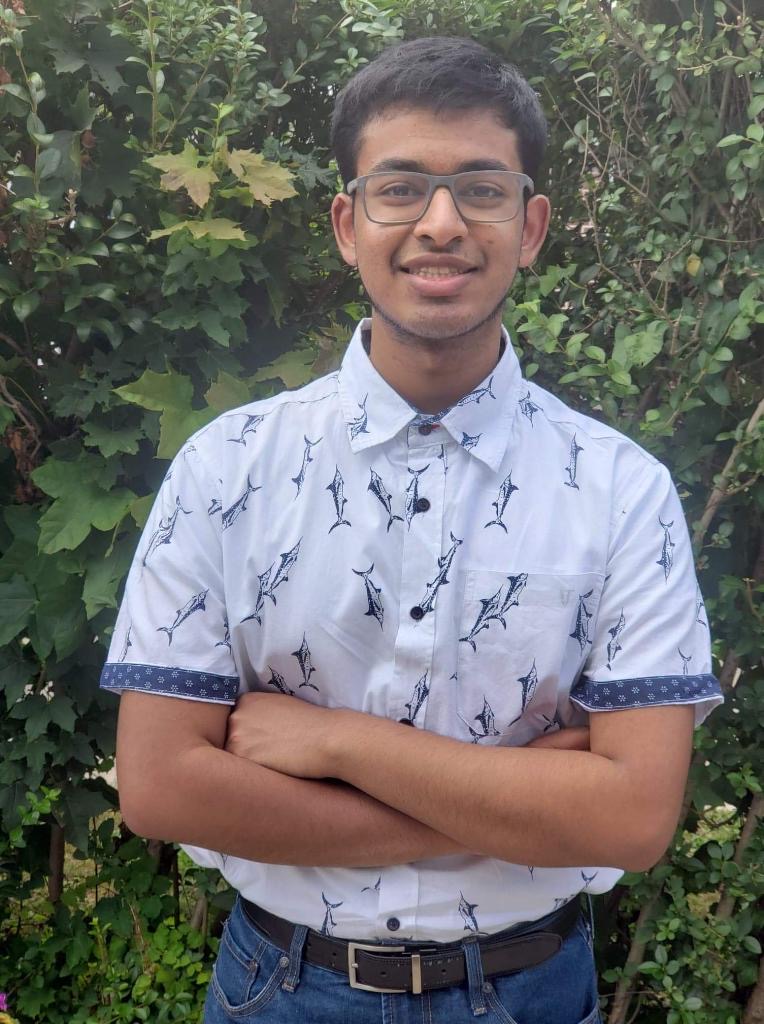
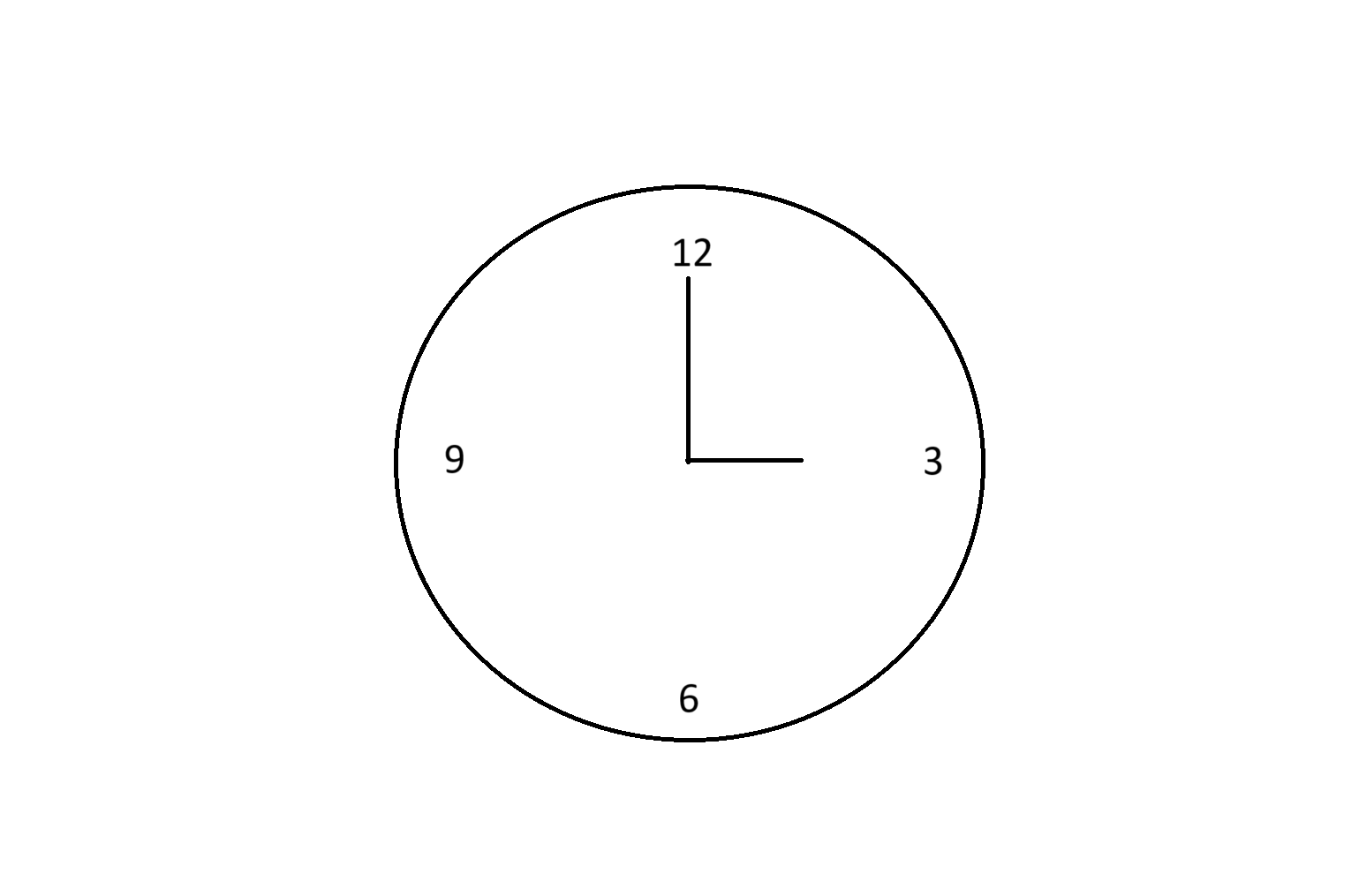
Overview
This article will explain how to write a Python script to create a countdown timer by using the "datetime" module. An optional module that is also used in this script is "time" which creates a delay between executing consecutive commands.
Materials
Computer
Python
- Python Modules: "datetime", "time". Both "datetime" and "time" are built-in Python modules. No additional packages are needed to download to use these modules.
Terminal, Command Prompt, Powershell or IDE
How the "datetime" Module Works
After importing the "datetime" module, one command that can be executed is "datetime.datetime.now()" which gives the current date and time by the present hour, minute, seconds and milliseconds.
import datetime
print(datetime.datetime.now())
"datetime" can also take in integer values for the year, month, day, hour, minute, seconds, and milliseconds values:
datetime.datetime(insert_year, insert_month, insert_day, insert_hour, insert_minute, insert_seconds, insert_milliseconds)
Methodology of Making the Timer
Since "datetime.datetime.now()" gives the current date and time, another variable using "datetime" can be created to take in integer values for a certain date and time. Within a while loop and an if statement, the Python script can constantly execute the "datetime.datetime.now()" command and compare the current date and time has reached the given time. This script shows a variable will be created to take in integers for the date and time, except for milliseconds.
Procedure
Step 1: Importing Modules
Import the modules "datetime" and "time".
#!/usr/bin/env python3
import datetime, time
Step 2: Make a Function
Create a function. The function name in this script is called timer:
def timer():
Step 3: Make While Loops to Take Integer Values
Within the function, there are many six-while loops. Each of the while loops takes in the year, month, day, hour, minute and seconds parameters for the given time, to ensure a realistic integer value is passed for every variable.
Explanations of the While Loops
When making the while loop, while is set to True to make it always run when a given input is not acceptable. Within the while loop, executes a try and except block. It will execute the try block by first asking for a variable. Depending on the variable, the if statement it evaluates differs to make sure the input is valid. If it is valid, then it will break out of the while loop to prevent it from executing the same while loop. If there is a scenario where non-integer data types are passed for the year, month, day, hour, minute, and second, then it will create a "ValueError" in Python. By specifying the "except ValueError:" this block will be executed if a non-integer data type was passed in the try block.
While Loop to Validate the Year
while True:
try:
insert_year = int(input("Enter the year number: "))
if insert_year >= 0:
break
except ValueError:
print("Not a real year number. ", end="")
While Loop to Validate the Month
while True:
try:
insert_month = int(input("Enter the month number: "))
if insert_month >= 1 and insert_month <= 12:
break
except ValueError:
print("Not a real month number. ", end="")
While Loop to Validate the Day
while True:
try:
insert_day = int(input("Enter the day number: "))
if insert_month == 1 or insert_month == 3 or insert_month == 5 or insert_month == 7 or insert_month == 8 or insert_month == 10 or insert_month == 12:
if insert_day >= 1 and insert_day <= 31:
break
elif insert_month == 2:
if insert_year % 400 == 0:
if insert_day >=1 and insert_day <= 29:
break
elif insert_year % 4 == 0 and insert_year % 100 != 0:
if insert_day >=1 and insert_day <= 29:
break
else:
if insert_day >=1 and insert_day <= 28:
break
else:
if insert_day >=1 and insert_day <= 30:
break
except ValueError:
print("Not a real month number. ", end="")
The while loop code for the day is longer than the other while loops to ensure, that the number passed for the day is correct for each month, including if it is a leap year or not for February.
While Loop to Validate the Hour
while True:
try:
insert_hour = int(input("Enter the hour number: "))
if insert_hour >= 0 and insert_hour <= 23:
break
except ValueError:
print("Not a real hour number. ", end="")
The hour variable takes in an integer variable in the military time format.
While Loop to Validate the Minute
while True:
try:
insert_minute = int(input("Enter the minute number: "))
if insert_minute >= 0 and insert_minute <= 59:
break
except ValueError:
print("Not a real minute number. ", end="")
While Loop to Validate the Seconds
while True:
try:
insert_seconds = int(input("Enter the amount of seconds: "))
if insert_seconds >= 0 and insert_seconds <= 59:
break
except ValueError:
print("Not a real second number. ", end="")
While Loop to Check the Time
The last while loop checks if "datetime.datetime.now()" has passed the given time. All the values from the previous while loops are entered into the variable called "inserted_time". If "datetime.datetime.now()" is greater than the "inserted_time" then it will break through the while loop. Otherwise, the else statement will be executed within the while loop will keep running until the if statement is true.
A new variable called "difference_in_time" takes the difference between the inserted time and the current time to find the total amount of time in seconds the timer will run.
The "time" module has been imported to run the "time.sleep()" command to prevent a delay in executing the next consecutive command. Another if and else statement is placed within the else statement for Python to sleep. The script will wait 10 seconds to execute the next command or either execute the next command in less than 10 seconds since the timer will eventually count down below 10 seconds. Since the while loop will always run until the if statement is satisfied, it always executes commands and updates numerical values in the else statement, including the "difference_in_time" variable. When "difference_in_time" is less than 10, signifies there are less than 10 seconds left. Then the second else statement will run and the if statement will execute and break out of the while loop.
inserted_time = datetime.datetime(insert_year, insert_month, insert_day, insert_hour, insert_minute, insert_seconds)
print("The timer will stop when it reaches this date and time:", inserted_time)
while True:
if datetime.datetime.now() > inserted_time:
break
else:
print("Current time:", datetime.datetime.now())
print("Time is still going...")
difference_in_time = inserted_time - datetime.datetime.now()
print("Time left: ", difference_in_time)
if (difference_in_time.total_seconds()) > 10:
time.sleep(10)
print("10 seconds has passed.")
else:
time.sleep(difference_in_time.total_seconds())
print("Less than 10 seconds has passed.")
print("Program Finished.")
Final Script
All the snippets of code shown above are implemented within the timer function:
#!/usr/bin/env python3
# Completed Script
import datetime, time
def timer():
while True:
try:
insert_year = int(input("Enter the year number: "))
if insert_year >= 0:
break
except ValueError:
print("Not a real year number. ", end="")
while True:
try:
insert_month = int(input("Enter the month number: "))
if insert_month >= 1 and insert_month <= 12:
break
except ValueError:
print("Not a real month number. ", end="")
while True:
try:
insert_day = int(input("Enter the day number: "))
if insert_month == 1 or insert_month == 3 or insert_month == 5 or insert_month == 7 or insert_month == 8 or insert_month == 10 or insert_month == 12:
if insert_day >= 1 and insert_day <= 31:
break
elif insert_month == 2:
if insert_year % 400 == 0:
if insert_day >=1 and insert_day <= 29:
break
elif insert_year % 4 == 0 and insert_year % 100 != 0:
if insert_day >=1 and insert_day <= 29:
break
else:
if insert_day >=1 and insert_day <= 28:
break
else:
if insert_day >=1 and insert_day <= 30:
break
except ValueError:
print("Not a real month number. ", end="")
while True:
try:
insert_hour = int(input("Enter the hour number: "))
if insert_hour >= 0 and insert_hour <= 23:
break
except ValueError:
print("Not a real hour number. ", end="")
while True:
try:
insert_minute = int(input("Enter the minute number: "))
if insert_minute >= 0 and insert_minute <= 59:
break
except ValueError:
print("Not a real minute number. ", end="")
while True:
try:
insert_seconds = int(input("Enter the amount of seconds: "))
if insert_seconds >= 0 and insert_seconds <= 59:
break
except ValueError:
print("Not a real second number. ", end="")
inserted_time = datetime.datetime(insert_year, insert_month, insert_day, insert_hour, insert_minute, insert_seconds)
print("The timer will stop when it reaches this date and time:", inserted_time)
while True:
if datetime.datetime.now() > inserted_time:
break
else:
print("Current time:", datetime.datetime.now())
print("Time is still going...")
difference_in_time = inserted_time - datetime.datetime.now()
print("Time left: ", difference_in_time)
if (difference_in_time.total_seconds()) > 10:
time.sleep(10)
else:
time.sleep(difference_in_time.total_seconds())
print("Less than 10 seconds has passed.")
print("Program Finished.")
timer()
Demonstration
Below shows when the script is executed, it asks for a time and the timer runs until that given time is reached.
Source Code
https://github.com/AndrewDass1/SCRIPTS/tree/main/Python/Make%20a%20Timer
Subscribe to my newsletter
Read articles from Andrew Dass directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
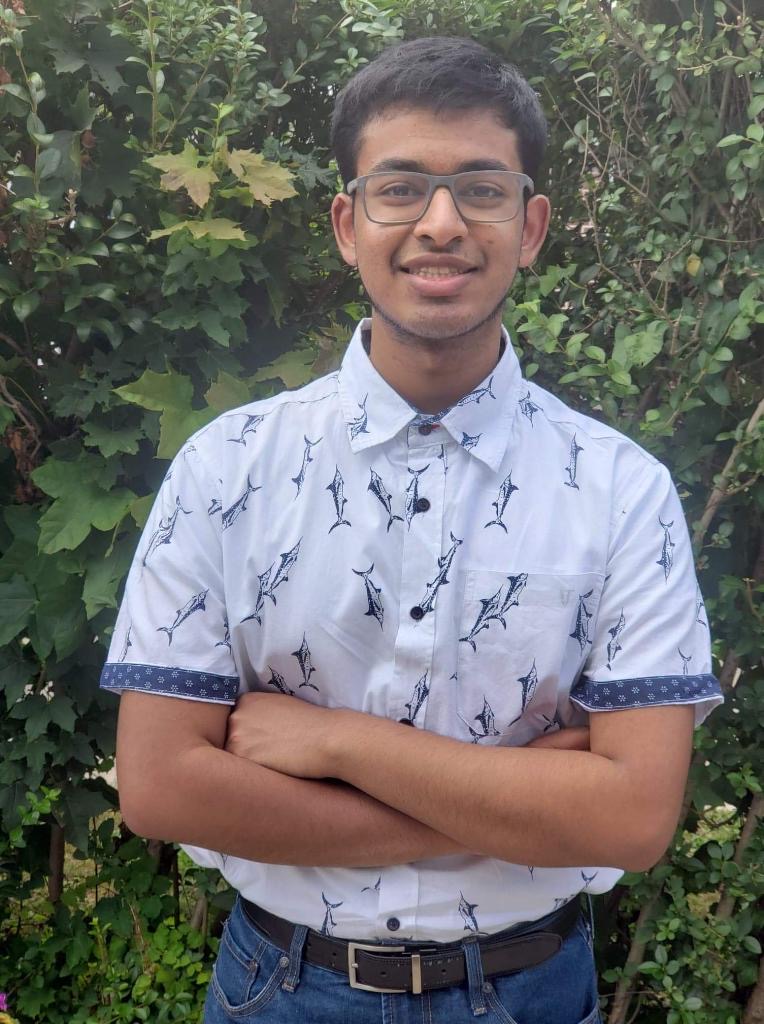
Andrew Dass
Andrew Dass
On my free time, I like to learn more about hardware, software and different technologies. I publish articles about my recent learnings or the projects I have done.