Introduction to C Programming

Table of contents
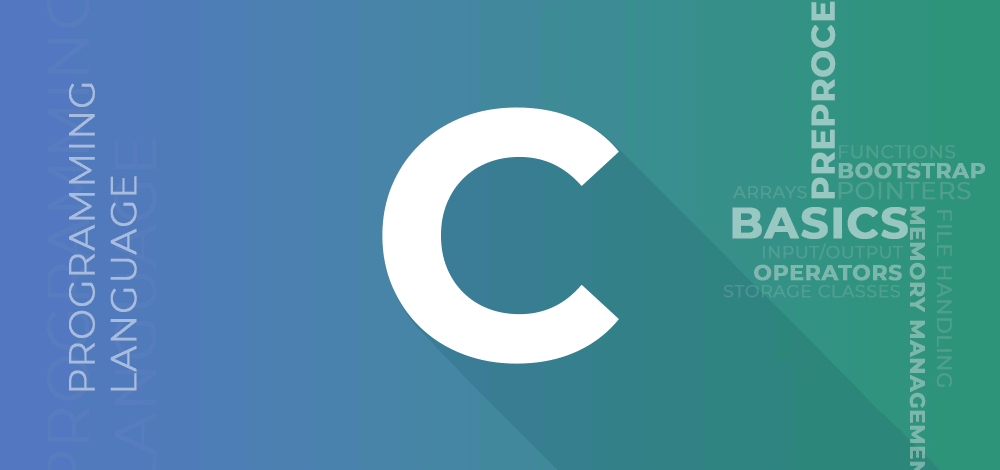
Introduction to C Programming
Computers
History
Earlier C language was called B language and was developed at Bell Labs( obviously B from Bell ). Then they made some improvements in the language and changed its name from B to C.
During the 1980s, C gradually gained popularity.
UNIX, Linux and Windows operating systems are written in C and C++
What is Program and why are we so-called programmers?
We use software applications like Chrome or Youtube to name a few! on our devices like smartphones, laptops, tablets, etc. every day. All these software consists of one or more programs.
Think of a program as a set of instructions that do some well-defined task.
Let's Simplify it
In our situation, we're not telling the computer to make a sandwich but to do something else, like adding two numbers together.
Programmers code these instructions in a programming language like C, C++.
How does an application run on your computer?
When you open an app, the computer puts the app's instructions in the RAM and lets the app take control. The app runs, gets information from you, shows you results, and finally stops running and gives control back to the computer.
Information
Now these instructions and data we provide to the computer are stored in RAM in the form of binary which consists of 0s and 1s. Each 0 or 1 represents a bit.
Bits
The most fundamental unit of a modern computer is the binary digit or bit. A bit is either on or off. One (1) represents on, while zero (0) represents off.
Bytes
The fundamental addressable unit of RAM is the byte. One byte consists of 8 bits.
Bit Value (this is 1 Byte) | Decimal Value |
This is how it is stored in Machine | This is in human Language |
27 26 25 24 23 22 21 20 | |
0 0 0 0 0 0 0 0 | 0 |
0 0 0 0 0 0 0 1 | 20 = 1 |
0 0 0 0 0 0 1 0 | 21 \= 2 |
0 0 0 0 0 0 1 1 | 21+ 20 \= 3 |
0 0 0 0 0 1 0 0 | 22 \= 4 |
... | ... |
0 0 1 1 1 0 0 0 | 25+24 +23 \= 56 |
... | ... |
1 1 1 1 1 1 1 1 | 27+26+25+24 +23+22+ 21+ 20 \= 255 |
One byte can store any one of 256 (28) possible values from 0 to 255 in the form of a bit string:
Hexadecimal
Hexadecimal is a numbering system with base 16. It can be used to represent large numbers with fewer digits. In this system, there are 16 symbols or possible digit values from 0 to 9, followed by six alphabetic characters -- A, B, C, D, E and F. The characters A through F denote the values that correspond to the decimal values 10 through 15 respectively. We use the 0x prefix to identify a number as hexadecimal (rather than decimal - base 10).
Bit Value | Hexadecimal Value | Decimal Value |
00000000 | 0x00 | 0 |
00000001 | 0x01 | 1 |
00000010 | 0x02 | 2 |
00000011 | 0x03 | 3 |
00000100 | 0x04 | 4 |
... | ... | |
00111000 | 0x38 | 56 |
... | ... | |
11111111 | 0xFF | 255 |
For example, the hexadecimal value 0x5C is equivalent to the 8-bit value 010111002, which is equivalent to the decimal value 92.
Addresses
Each byte of RAM has a unique address. Addressing starts at zero and is sequential, and ends at the address equal to the size of RAM less 1 unit.
For example, 4 Gigabytes of RAM
consists of 32 (= 4 * 8) Gigabits
starts at a low address of 0x00000000
ends at a high address of 0xFFFFFFFF
NOTE:
Each byte, and not each bit, has its own address. lThat’s why we say that RAM is byte addressable.
Segmentation Faults ( you will face it while coding)
The information stored in RAM consists of information that serves different purposes.
We are expected to read and write data, but not to execute it. Similarly, we are expected to execute program instructions but not to write them. So, certain architectures assign the data => read and write permissions, while some assign instructions => read and execute permissions.
Such a permissions system helps trap errors while a program is executing. An attempt to execute data or to overwrite an instruction reports an error. Clearly, the access has been to the wrong segment. We call such errors a segmentation fault.
Compilers
Introduction
Now the computer only understands things in bits and bytes.
Human language that we read can be converted to bits and bytes.
For Example:
1 is equal to 00000001.
A is equal to 64 in decimal which is 01000001 in binary.
The C compiler
Now whatever instructions we write and data we provide in programming language need to get converted to Computer language. The compiler does that for us.
Our very first C program
Let us write a program that displays the phrase "This is C" and name our source file hello.c. Source files written in the C language end with the extension .c.
/* My first program // comments introducing the source file
hello.c
*/
#include <stdio.h> // information about the printf identifier
int main(void) // the starting point of the program
{
printf("This is C"); // send output to the screen
return 0; // return control to the operating system
}
Linux
The C compiler that ships with Linux operating systems is called gcc.
To create a binary code version of our source code, enter the following command in command line:
gcc hello.c
hello.c -------> gcc --------> a.out
(source code) (compiler) (executable file)
By default, the gcc compiler produces an output file named a.out*.*
a.out contains all of the machine language instructions needed to execute the program.
To execute these machine language instructions, enter the command:
a.out
The output of the executed binary will display:
This is C
Documentation
We put comments to self-document our source code and enhance its readability. Comments are important in the writing of any program.
C supports two styles: multi-line and inline.
C compilers ignore all comments.
Multi-Line Comments
/* My first program
hello.c */
Inline Comments
int main(void) // the starting point of the program
Indentation
#include <stdio.h> // information about the printf identifier
int main(void) // the starting point of the program
{
printf("This is C"); // send output to the screen
return 0; // return control to the operating system
}
By indenting the printf("This is C")
statement and placing it on a separate line, we show that printf("This is C")
is part of something larger, which we have called int main(void)
Program Startup
Every C program includes a clause like int main(void)
. Program execution starts at this line. We call it the program's entry point.
int main(void) // program startup
{
return 0; // return to operating system
}
When the users or we load the executable code into RAM (a.out or hello.exe), the operating system transfers control to this entry point. The last statement (return 0;) before the closing brace transfers control back to the operating system.
Program Output
The following statement outputs "This is C"
to the standard output device (the screen).
printf("This is C");
The line before int main(void)
includes information that tells the compiler that printf
is a valid identifier.
#include <stdio.h> // information about the printf identifier
Case Sensitivity
The C programming language is case-sensitive.
If we change the identifier printf()
to PRINTF(). The compiler will report a syntax error.
Subscribe to my newsletter
Read articles from Pratham Garg directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Pratham Garg
Pratham Garg
I am a student learning Computer Programming 👨💻.