How to improve your React.js code quality
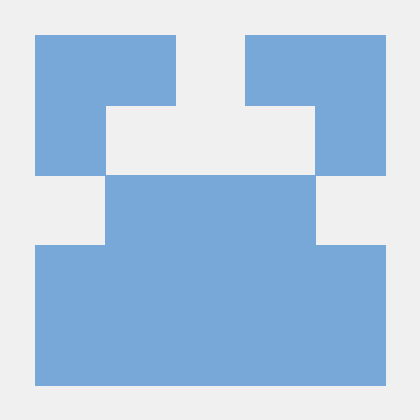
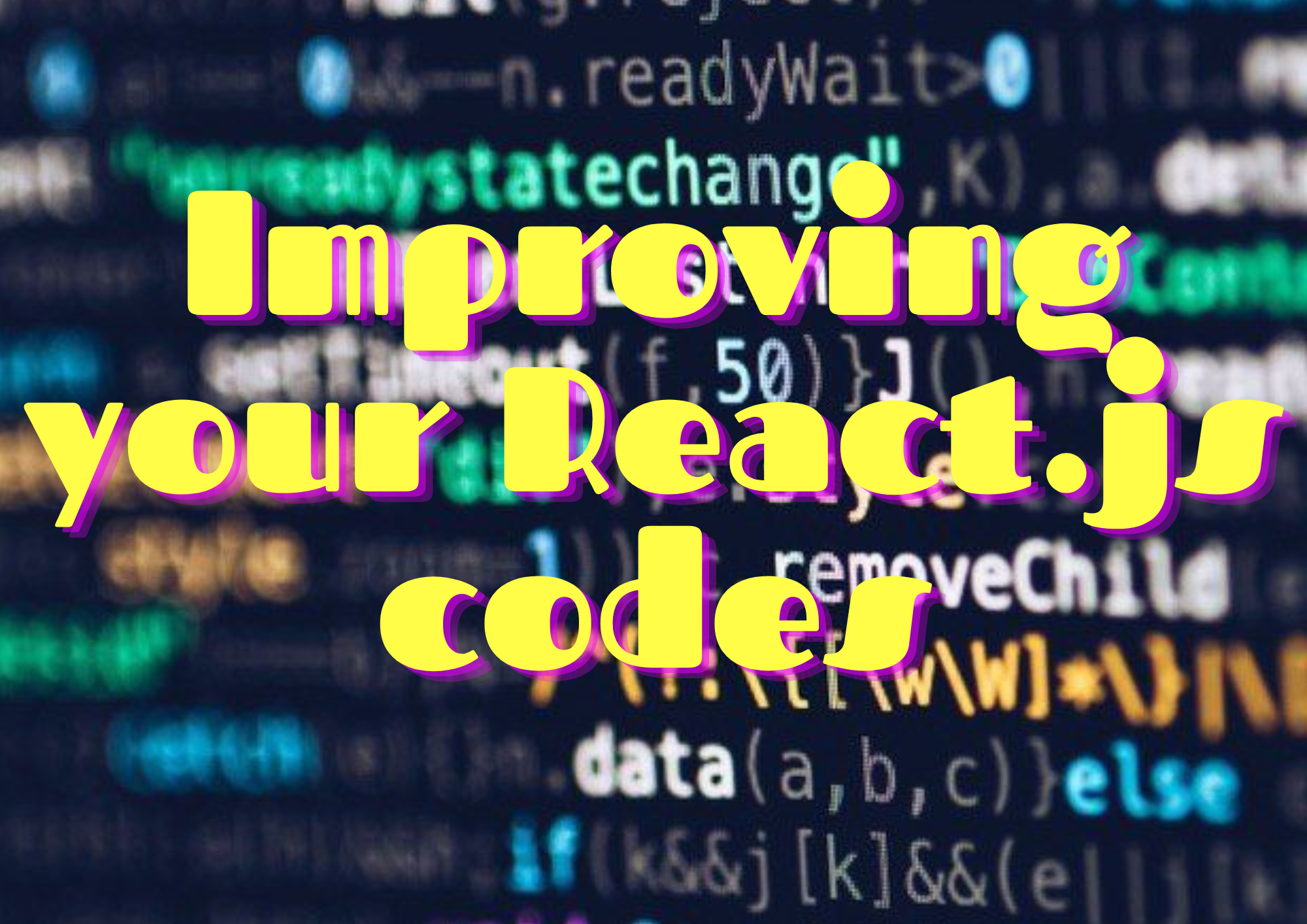
Introduction
You must have probably been told, or have read somewhere( maybe on Twitter) that a codebase with clean and quality codes is the best to work on.
Also, there are very good and important reasons why you should write codes which are very easy to understand and self-explanatory(which you will see in the course of this article).
So, in this article, you will learn how to optimise your react codebase and make it more readable and efficient. You will also learn how to improve your React website's performance and increase its speed.
Prerequisites
To better understand this topic, you must
Have a code editor(preferably VScode)
Have a basic understanding of JavaScript and React.js
Why improve code quality?
When writing codes, it is important to write them in a way that someone else can look at your codes and understand immediately what your codebase is all about.
Apart from that, it saves the time which would have been spent searching for a part in your codebase for you to make a correction or addition.
Writing clean quality codes which follow standard procedures shows how high your skill set is as a developer.
Now that you know the importance of writing quality codes, let's see how you can improve your React codebase.
How to improve your React.js code quality
Several things should be considered for a React codebase to have quality codes. These are:
Comments
Single Responsibility Principle (SRP)
Indentation
File Structure
Variable naming
Comments:
A well-commented codebase is always the best to understand and navigate. If your codes are well commented on, at first glance you, or an outsider, even if they do not have basic programming knowledge, will be able to summarise and have a basic understanding of what your codebase does.
Here is a basic example:
A plain code
const addFunction = (num) => {
const randomNumber = Math.floor(Math.random() * 5);
const totalNumber = randomNumber + num;
return totalNumber;
}
VS
Commented code
//This function generates a random number and adds it to the function's parameter
const addFunction = (num) => {
//randomNumber generates a random number with range of 0 to 5 and approximates it
const randomNumber = Math.floor(Math.random() * 5);
//Addition of the random number and function parameter
const totalNumber = randomNumber + num;
return totalNumber;
}
Now, looking at the two examples, you will notice that the second code block gives you a basic idea of what it does even before you go through the actual codes. Anyone can come to this second code block and have a basic understanding of what it does as opposed to the first one. That is how powerful code comments are.
Single Responsibility Principle (SRP):
This is a concept gotten from object-oriented programming. Concerning React, the principle states that a component should not have more than one function.
This helps to improve code readability and organisation. When you discover that a particular component is already getting too large, it is always advised to break it down into smaller components. This will help make sure that a component in your codebase is not cluttered and unnecessarily large.
To further drive home the point, check out the example below:
A large component
import react from 'react'
const Data = [
{
name: 'Andrew',
age: '22'
},
{
name: 'Jane',
age: '20'
},
]
const MainFunction = () => {
return (
<div>
<div>
<h1>This is a list of users</h1>
{/*Mapping through the array*/}
{
Data.map((item, index) => {
<div key={index}>
<h1>{item.name}</h1>
<h1>{item.age}</h1>
</div>
})
}
</div>
</div>
)
}
export default MainFunction
VS
Smaller components
import react from 'react'
const Card = ({name, age}) => {
return (
<div>
<h1>{name}</h1>
<h1>{age}</h1>
</div>
)
}
export const Data = [
{
name: 'Andrew',
age: '22'
},
{
name: 'Jane',
age: '20'
},
]
import react from 'react'
import {Data} from './src/Data'
import Card from './component/card'
const MainFunction = () => {
return (
<div>
<div>
<h1>This is a list of users</h1>
{/*Mapping through the array*/}
{
Data.map((item, index) => {
<Card key={index} name={item.name} age={item.age} />
})
}
</div>
</div>
)
}
The examples above show how important the SRP is. The first block of code has a large component that does three things:
It holds the data used by the app
It maps through the data
It renders a subcomponent which uses the information inside the data
On the other hand, the second code example splits the MainFunction into three parts that handle one responsibility each. Notice how readable the MainFunction is after the improvement.
File Structure:
The way you arrange your files and folders is very important and determines the level of organization of your codebase. For large projects, buttons, cards, carousels, accordions, and all other smaller components which are used across the codebase are best arranged in the components folder. Hooks, types and interfaces(for TypeScript users) and global state should also have their folders for easier navigation of the codebase.
But file structure depends on the project size. When working with a small project, let's say a landing page, you are not expected to split the components like buttons and cards into their separate folders as they(buttons, cards etc.) are not being used across many pages. The key point here is to evaluate the size of the project; does it need more than 2 pages, is a function(s) needed across multiple pages, etc and base your file structure on that.
Variable naming:
While this has always been emphasized by most senior devs, most newbies often don't understand the importance of correct variable naming.
The golden rule for variable naming suggests that you should always name your variables, and by extension, your functions, according to the work they were created for or the reason why they exist.
Let's see an example for a better understanding:
//variable 1
const firstVar = 10;
console.log(`James is ${firstVar} old`)
//variable 2
const age = 10
console.log(`James is ${age} old`)
Now, looking at the two variables above, you can easily tell what the second variable does and why it exists based on its name, compared to the first variable which tells us nothing. The same applies to functions:
//first function
const function1 = (firstParam, secondParam) => {
return `Hi, My name is ${firstParam} and i am ${secondParam} years old`
}
//Second function
const intro = (name, age) => {
return `Hi, My name is ${name} and i am ${age} years old`
}
Conclusion
Now, you have learned the various ways in which you can improve your react.js codebase and code quality. Apply them and witness your codebase transform.
Thanks for readingš¤
Subscribe to my newsletter
Read articles from Divine Udise directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
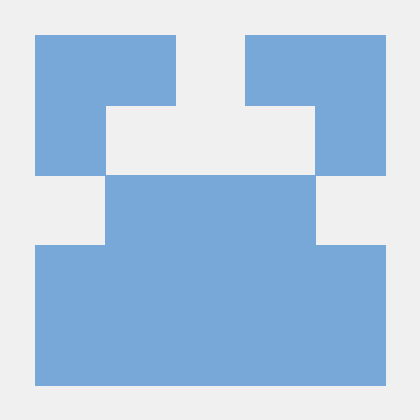