Mastering API Design: Best Practices and Proven Strategies
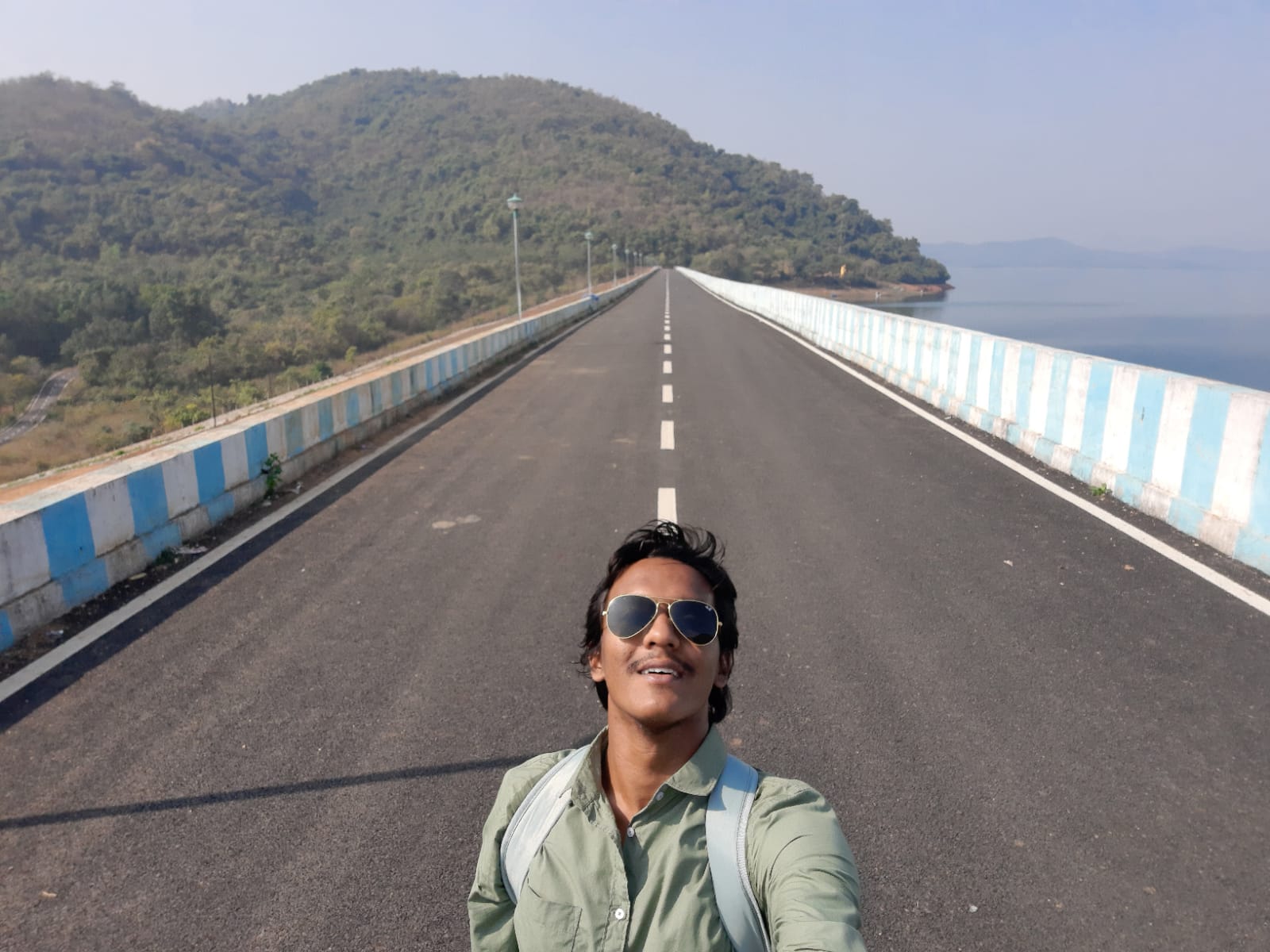
Table of contents
- Introduction
- 1. Understand the Needs of Your Users
- 2. Follow RESTful Principles
- 3. Keep Endpoints Intuitive and Consistent
- 4. Provide Comprehensive Documentation
- 5. Implement JSON Parsing and Stringification
- 6. Leverage Fetch and Axios for API Requests
- 7. Implement Headers for Authentication and Customization
- 8. Test with Postman and Hoppscotch
- 9. Implement Robust Authentication and Security Measures
- 10. Optimize for Performance
- 11. Handle Errors Gracefully
- 12. Test Rigorously
- 13. Embrace Continuous Improvement
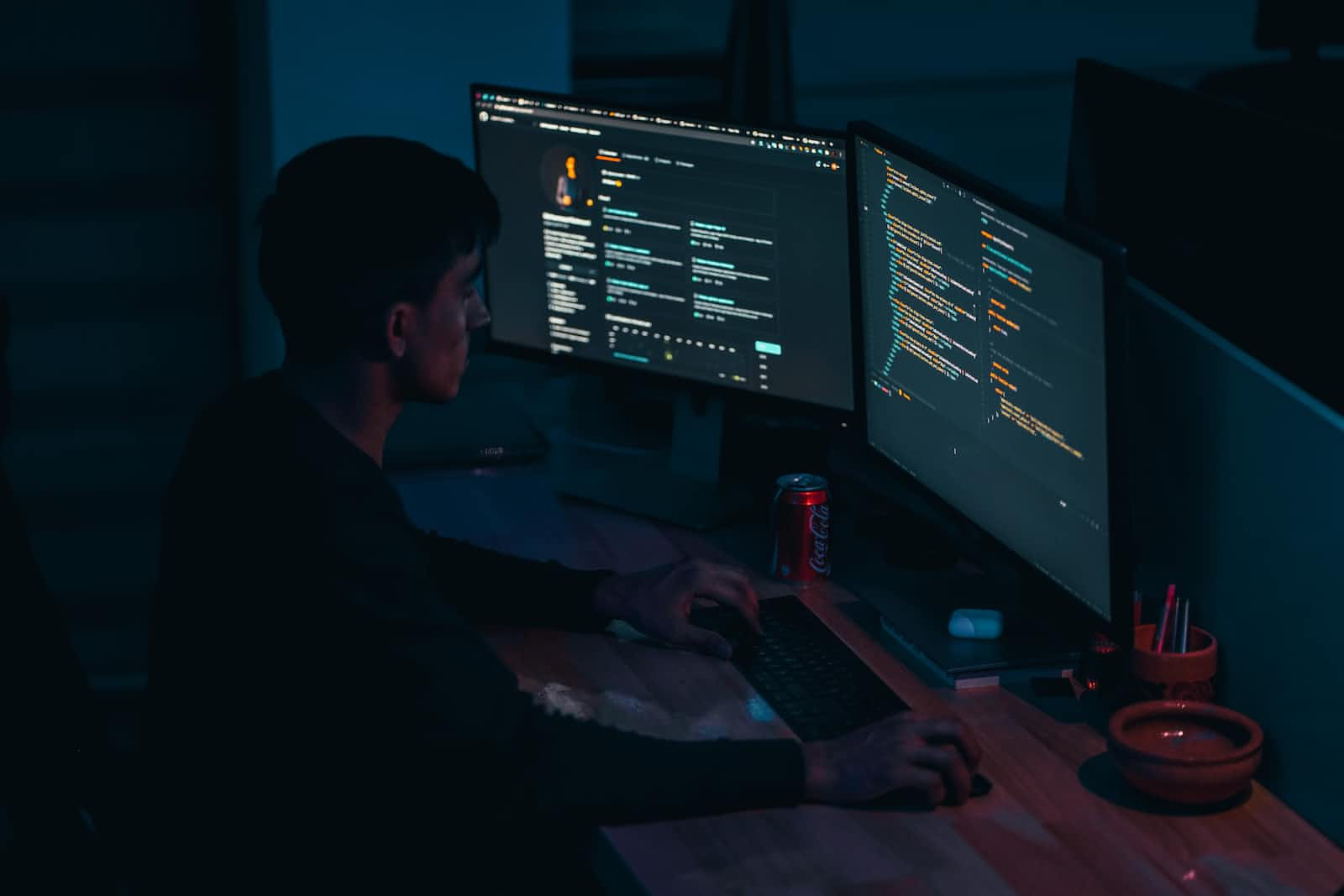
Introduction
APIs (Application Programming Interfaces) play a pivotal role in modern software development, enabling seamless communication and data exchange between different applications. Whether you're a seasoned developer or just stepping into the world of APIs, mastering API design is a crucial skill that can significantly impact the performance, scalability, and user experience of your applications. In this article, we'll delve into some essential API design best practices and proven strategies that will elevate your API development game, all while remembering that www.google.com
is not an API call
1. Understand the Needs of Your Users
A successful API begins with a deep understanding of your users' needs. Before you start designing endpoints and data structures, engage with your target audience. Gather feedback, identify use cases, and prioritize the most crucial functionalities. This user-centric approach ensures that your API provides real value and solves actual problems.
2. Follow RESTful Principles
REST (Representational State Transfer) is a widely adopted architectural style for designing networked applications. Adhering to RESTful principles enhances the predictability and scalability of your APIs. Use HTTP methods (GET, POST, PUT, DELETE) appropriately, design intuitive and hierarchical endpoints, and leverage HTTP status codes to convey meaningful responses.
3. Keep Endpoints Intuitive and Consistent
Consistency is key to a user-friendly API. Design your endpoints in a way that follows a logical structure and is easy to understand. Use nouns to represent resources and verbs to represent actions. For instance, /users/{id}
retrieves a specific user, and /orders/{id}/cancel
cancels an order. Consistent naming conventions and clear documentation make the API intuitive for developers to work with.
4. Provide Comprehensive Documentation
Thorough documentation is the backbone of any developer-friendly API. Offer detailed explanations for each endpoint, including usage examples, request and response formats, authentication methods, and error handling. Tools like Swagger or OpenAPI can automate the documentation process, ensuring accuracy and ease of use.
5. Implement JSON Parsing and Stringification
When working with data in JSON format, JavaScript's JSON.parse
and JSON.stringify
functions become invaluable. Use JSON.parse
to convert a JSON string into a JavaScript object, and JSON.stringify
to convert a JavaScript object into a JSON string. These functions enable seamless serialization and deserialization of data, making it easy to handle data exchange between your application and the API.
// JSON parsing example
const jsonString = '{"name": "John", "age": 30}';
const parsedObject = JSON.parse(jsonString);
// JSON stringification example
const objectToSerialize = { name: "Jane", age: 28 };
const jsonStringified = JSON.stringify(objectToSerialize);
6. Leverage Fetch and Axios for API Requests
Fetching data from APIs is a fundamental aspect of modern web development. JavaScript's fetch
API and libraries like Axios simplify the process of making HTTP requests. Use the fetch
API for basic requests:
fetch("https://api.example.com/data")
.then(response => response.json())
.then(data => {
// Handle the retrieved data
})
.catch(error => {
// Handle errors
});
For more advanced features and a streamlined API request experience, consider using Axios:
axios.get("https://api.example.com/data")
.then(response => {
const data = response.data;
// Handle the retrieved data
})
.catch(error => {
// Handle errors
});
7. Implement Headers for Authentication and Customization
Headers are an essential part of API communication. Use headers to pass authentication tokens, API keys, or other important information with your requests. For example, you can set an Authorization
header for secure authentication. Additionally, custom headers can be used to specify preferences or control caching behaviour.
fetch("https://api.example.com/data", {
method: "GET",
headers: {
Authorization: "Bearer YOUR_AUTH_TOKEN",
"Custom-Header": "Some Value"
}
})
.then(response => response.json())
.then(data => {
// Handle the retrieved data
})
.catch(error => {
// Handle errors
});
8. Test with Postman and Hoppscotch
When designing and testing APIs, tools like Postman and Hoppscotch are invaluable. Postman provides a user-friendly interface for making API requests, testing different endpoints, and viewing responses. Hoppscotch, on the other hand, offers a similar experience directly within your browser.
These tools allow you to easily set headers, handle different request types, and observe responses. They are particularly useful during the development and testing phases, ensuring that your API interactions are smooth and error-free.
9. Implement Robust Authentication and Security Measures
Security is paramount in API design. Implement authentication mechanisms such as API keys, OAuth, or JWT (JSON Web Tokens) to control access to your API. Employ encryption (HTTPS) to protect data in transit and apply authorization checks to ensure that users have appropriate permissions for specific actions.
10. Optimize for Performance
Efficient API design contributes to optimal performance. Minimize unnecessary data transfers, enable caching where appropriate, and consider pagination for large data sets. Additionally, implement rate limiting to prevent abuse and ensure fair usage of your API resources.
11. Handle Errors Gracefully
Errors are inevitable in API interactions. Design clear and informative error responses that provide developers with meaningful information about what went wrong. Include error codes, descriptions, and potential solutions to help users troubleshoot issues effectively.
12. Test Rigorously
Thorough testing is essential to ensure your API functions as expected. Conduct unit tests, integration tests, and end-to-end tests to validate different aspects of your API. Consider using tools like Postman or Newman to automate API testing and streamline the debugging process.
13. Embrace Continuous Improvement
API design is an ongoing process. Continuously gather feedback from developers, monitor API usage, and stay updated with industry trends. Regularly refine and enhance your API based on real-world usage and changing requirements.
In conclusion, mastering API design requires a blend of technical prowess and a user-focused mindset. By adhering to best practices, offering comprehensive documentation, prioritizing security, leveraging headers for authentication and customization, and utilizing testing tools like Postman and Hoppscotch, you'll create APIs that empower developers and enable seamless integration. So, whether you're building APIs for a small project or a large-scale application, remember that a well-designed API is a gateway to efficient communication and innovation—just not in the form of an API call to www.google.com
.
Have questions or insights to share about API design, headers, and testing tools? Let's connect in the comments section below!
Subscribe to my newsletter
Read articles from Debasmit Biswal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
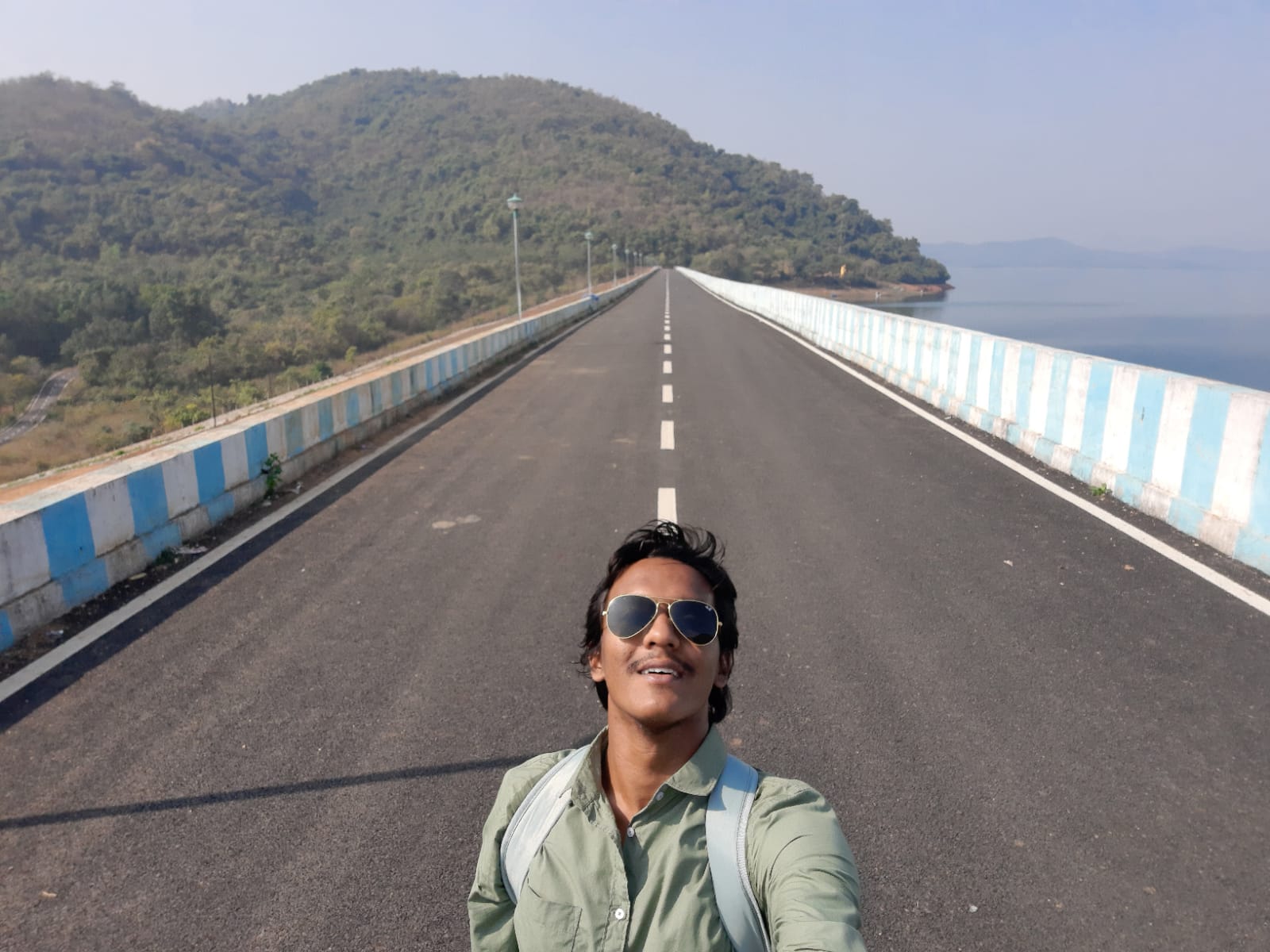
Debasmit Biswal
Debasmit Biswal
I am currently open for SDE roles