Creating dynamic head tags in React using react-helmet
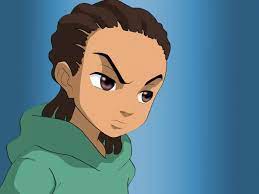
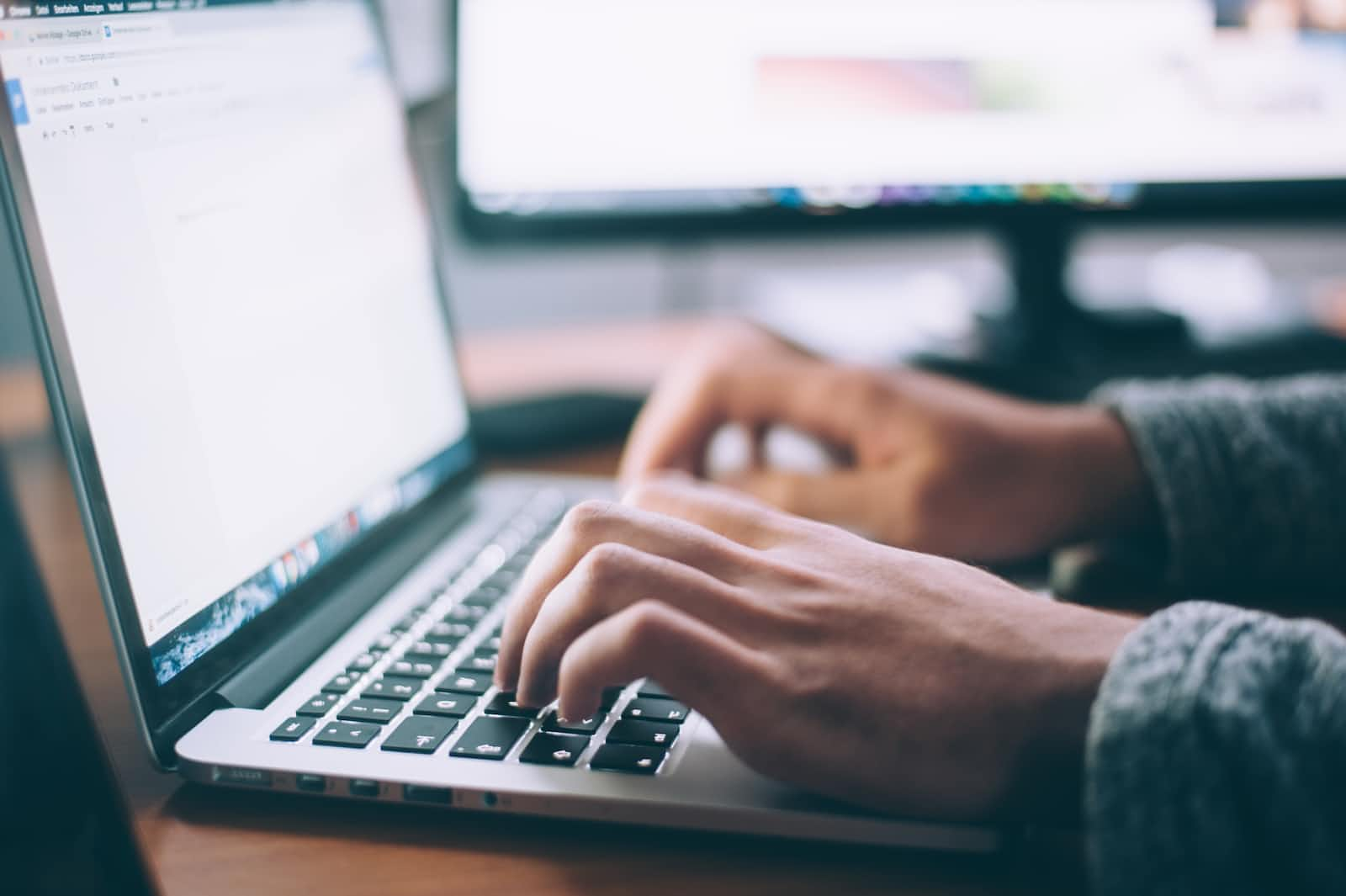
When building modern web applications, it is crucial to work on the <head>
section of your pages. The <head>
section of an HTML document contains metadata like <title>
and other important information that helps search engines, social media platforms and sometimes users understand your site correctly. The majority of modern JavaScript frameworks, including React, employ SPA (Single Page Applications) architecture. In this approach, a single HTML page serves as the foundation, and content is subsequently added or removed using JavaScript. To simulate the experience of a multi-page application developers make use of react-router
a JavaScript framework responsible for handling routing. However, it's important to note that all these manipulations occur within the <body> tag, rendering it impossible to dynamically alter the content within the <head> section throughout these routes. One tool we can use to manage the <head>
tags for each route is the react-helmet
library.
What is react-helmet
react-helmet
is a popular third-party library for managing and updating the content of the <head>
section of your web pages dynamically. It provides a convenient and declarative way to control the metadata, titles, styles, scripts, and other elements in the <head>
of your HTML documents. This library makes it easy to manage this information on a per-component basis, allowing you to create more personalized and optimized user experiences.
Getting Started
To use react-helmet
in your React application, you'll need to follow these steps
Install react-helmet
and react-router-dom
Install react-helmet
and react-router-dom
using a package manager like npm or yarn
npm install react-helmet react-router-dom
Create the page components
Create a component for each page and import in App.jsx
- For this example, I created three pages Home, About and Contact
// /App.jsx
import React from 'react';
import { BrowserRouter, Routes, Route } from 'react-router-dom';
import Home from './pages/Home';
import Contact from './pages/Contact';
import About from './pages/About';
function App() {
return (
<BrowserRouter>
<Routes>
<Route path="/" element={<Home />} />
<Route path="/contact" element={<Contact />} />
<Route path="/about" element={<About />} />
</Routes>
</BrowserRouter>
);
}
export default App;
PS: The code to this project is available here https://stackblitz.com/edit/vitejs-vite-zroh9s?file=src%2Fpages%2FHome.jsx
Use react-helmet
in your page component
Create a <Helmet>
component in all of your pages, whatever you write in here will be applied to the head of that page when you are on that
// /pages/Home.jsx
import React from 'react';
import { Helmet } from 'react-helmet';
export default function Home() {
return (
<div>
<Helmet>
<title>Home</title>
<meta name="description" content="Description for Home" />
</Helmet>
Home
</div>
)
}
Benefits of Using react-helmet
1. Declarative Approach: react-helmet
offers a declarative approach to managing the <head>
content. You can define the desired head tags directly within the components that require them, making your code more organized and easier to maintain.
2. SEO Optimization: Search engines use the information in the <head>
section to understand and rank your content. By dynamically updating metadata like titles and descriptions, you can improve the visibility and SEO ranking of your pages.
3. Social Media Sharing: Social media platforms use the information from the <head>
section to generate previews when users share links. With react-helmet
, you can control how your content appears on platforms like Facebook, Twitter, and LinkedIn.
4. Component-Specific Updates: Different components in your application might require unique metadata. react-helmet
enables you to set specific head tags for each component, tailoring the content to the user's context.
Conclusion
Managing the dynamic content of the <head>
section is crucial for creating well-optimized and visually appealing web applications. react-helmet
simplifies this process by providing an intuitive and declarative way to control the content of the <head>
tags within your React components. Whether you're optimizing for search engines, social media sharing, or creating a better user experience, react-helmet
makes it easy to dynamically update your <head>
section.
Subscribe to my newsletter
Read articles from Judge-Paul Ogebe directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
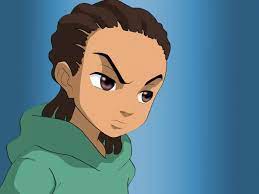
Judge-Paul Ogebe
Judge-Paul Ogebe
software dev trying a bit of everything, linux fanboy