Mastering Local Notifications in Flutter: A Comprehensive Guide on How To Implement and Customize
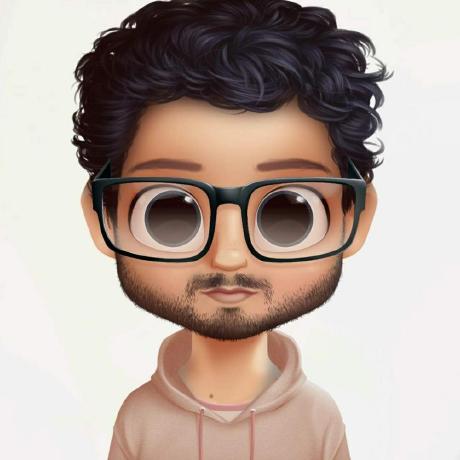
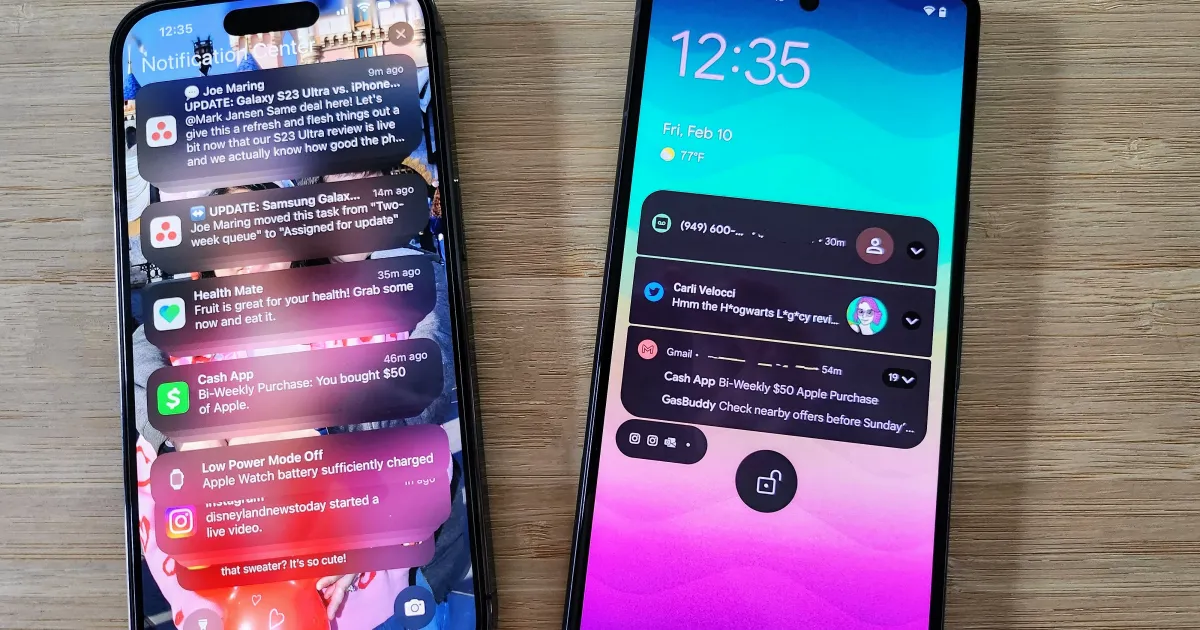
Nowadays finding an app that doesn't have a push notification feature is rare. We'll learn here how to have local push notifications in our app.
First thing first, we have to add the flutter_local_notifications plugin to our project.
flutter pub add flutter_local_notifications
Then we'll create a local_notification_service file and add our necessary codes there to have a separation of UI and logic code for better readability and maintainability.
import 'package:flutter_local_notifications/flutter_local_notifications.dart';
class LocalNotificationService {
static final flutterLocalNotificationsPlugin =
FlutterLocalNotificationsPlugin();
int notificationId = 1;
static Future<void> init() async {
// Initialize native android notification
const AndroidInitializationSettings initializationSettingsAndroid =
AndroidInitializationSettings('@mipmap/ic_launcher');
// Initialize native Ios Notifications
const DarwinInitializationSettings initializationSettingsIOS =
DarwinInitializationSettings();
const InitializationSettings initializationSettings =
InitializationSettings(
android: initializationSettingsAndroid,
iOS: initializationSettingsIOS,
);
await flutterLocalNotificationsPlugin.initialize(
initializationSettings,
);
}
}
We'll call the init method from our main function.
void main() async {
WidgetsFlutterBinding.ensureInitialized();
// our init method
await LocalNotificationService.init();
runApp(const MyApp());
}
We'll now implement the showNotification*() method for our specific platform, the method will take a title and a message* parameter.
class LocalNotificationService {
...
NotificationDetails getNotificationDetailsAndroid(
String title, String value) {
const AndroidNotificationDetails androidNotificationDetails =
AndroidNotificationDetails('channel_id', 'Channel Name',
channelDescription: 'Channel Description',
importance: Importance.max,
priority: Priority.high,
ticker: 'ticker');
const NotificationDetails notificationDetails =
NotificationDetails(android: androidNotificationDetails);
return notificationDetails;
}
NotificationDetails getNotificationDetailsIos(String title, String value) {
final DarwinNotificationDetails iOSPlatformChannelSpecifics =
DarwinNotificationDetails(
presentAlert:
true, // Present an alert when the notification is displayed and the application is in the foreground (only from iOS 10 onwards)
presentBadge:
true, // Present the badge number when the notification is displayed and the application is in the foreground (only from iOS 10 onwards)
presentSound:
true, // Play a sound when the notification is displayed and the application is in the foreground (only from iOS 10 onwards)
// sound: String?, // Specifics the file path to play (only from iOS 10 onwards)
// badgeNumber: int?, // The application's icon badge number
// attachments: List<IOSNotificationAttachment>?, // (only from iOS 10 onwards)
subtitle: value, //Secondary description (only from iOS 10 onwards)
threadIdentifier: title, // (only from iOS 10 onwards)
);
final NotificationDetails notificationDetails =
NotificationDetails(iOS: iOSPlatformChannelSpecifics);
return notificationDetails;
}
Future<void> showNotification(
{required String title, required String description}) async {
final notificationDetails = Platform.isAndroid
? getNotificationDetailsAndroid(title, description)
: getNotificationDetailsIos(title, description);
await flutterLocalNotificationsPlugin.show(
notificationId,
title,
description,
notificationDetails,
payload: 'Not present',
);
}
}
Now we can call the showNotification() method anywhere from our app to show a local notification.
Subscribe to my newsletter
Read articles from Masnun Siam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
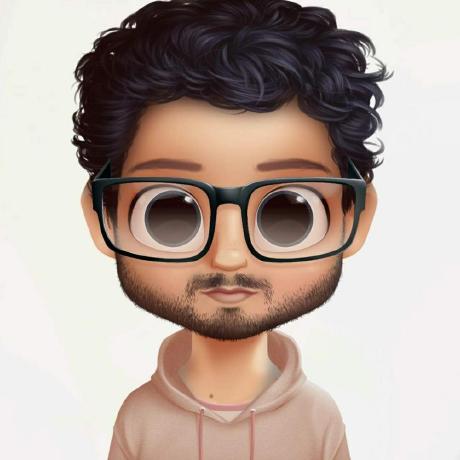
Masnun Siam
Masnun Siam
I am a Flutter developer with 3 years of experience. I am currently working at Binary Fusion.