Python for Beginners Part-1

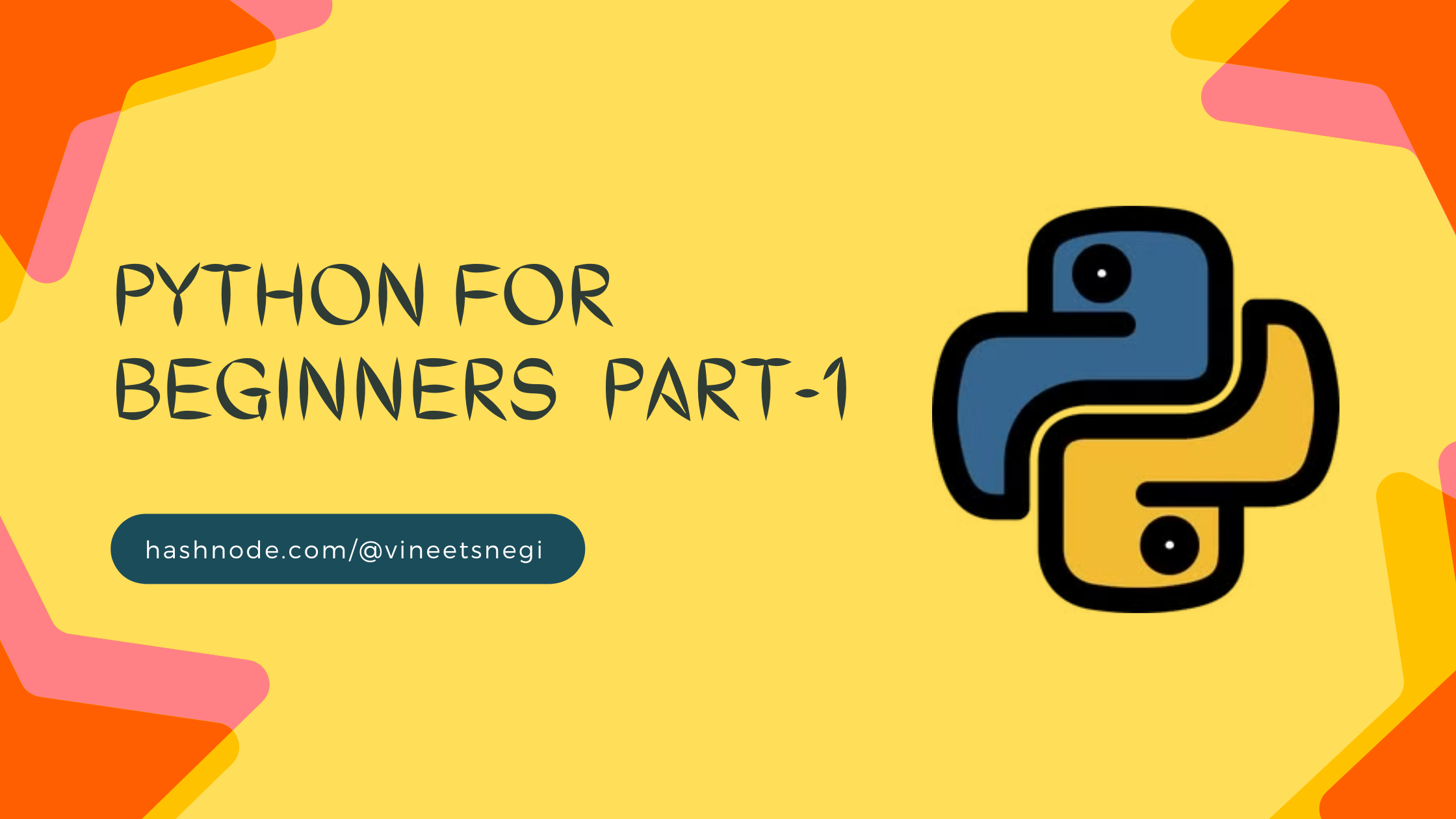
1. Why Learn Python In The First Place
Python is a powerful programming language yet extremely simple to learn. It is built around the philosophy of minimal code to get the same work done.
Let's get an intuition of the same using an example:
1.1) Hello Readers
program in C++ and Java
// C++ CODE
#include <iostream>
int main() {
std::cout << "Hello Readers!";
return 0;
}
// JAVA CODE
class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, Readers!");
}
}
1.2) Hello Readers
program in Python
print ("Hello Readers!")
Yes, that's about it. We got the same output in a single line of code. On the contrary, that same output needed required 5 lines of code in C++ and Java. Due to this compact and versatile nature of Python programming language, it finds its way into complex domains like Machine Learning, Data Science, Big Data, AI, etc.
Moreover, by getting proficient in Python programming language in addition to some specific libraries and skills, it's not wrong to say that you can apply to entry-level jobs like: -
Data Analyst
Python Developer
Backend Developer
And if you are someone with some experience on hand then you can apply to: -
DevOps Engineer
Data Scientist
MLOps Engineer
AI Scientist, etc.
2. Getting Started With print()
Function
2.1) Printing without the quotes
Assume you want to print the numerical output of a mathematical expression. For this, simply enter the values in the print function without the quotes. Let's look at an example of a numeric sum of 10 + 3
print(10+3)
So it returns the numerical sum of the two values; 13
2.2) Printing with the quotes
Assume you want to output the same value you entered into the print function. Simply insert the values in the print function within the quotations to do this. Consider this example sum of 10+ 3
print("10 + 3")
So it returns the expression 10 + 3
as the output. So, it's kinda right to say that anything put inside the quotations will be treated as a string by Python.
3) Mathematical Operators
Python supports all 4 general mathematical operators.
3.1) Addition
The
+
symbol denotes addition.It returns the
sum
of two or more numbers
print(4 + 5) #9
print(2.5 + 2.5) #5.0
3.2) Subtraction
The
-
symbol denotes subtraction.It returns the
difference
between two or more numbers
print(8 - 5) #3
print(7.0 - 5.5) #1.5
3.3) Multiplication
The
*
symbol denotes multiplication.It returns the
product
of two or more numbers
print(2 * 8) #16
print(9 * 0.5) #4.5
3.4) Division
The
/
symbol denotes division.It returns the
quotient
of two numbers.
print(10 / 2) #5.0
print(10 // 2) #5
print(12 / 0.3) #40.0
4) Variables
A Python variable is a designated memory location used for storing values. We can also visualize variables to be empty containers that store user-defined values or information. Let us now initialize a variable named player_name
and give it a value Messi
.
player_name = "Messi"
t_number = 10
When the variable is initialized, it means that it has been allocated some space within the memory. And when it's given a value like Messi
, it gets stored in the memory location. Here the equals to sign ( = )
is called as Assignment Operator
as it is used to assign values to variables.
Note: Rules to follow when naming Python Variables
Variable names should only contain alpha-numeric characters and underscores (A-z, 0-9, and _ ).
Variable names are case-sensitive (Personal advice is to use lowercase only).
Variable names cannot start with numbers.
4.1) Expressions
A valid combination of numbers, variables, and operators is called an expression.
(7 * 4) + (67 - 2) = a
x + 45 = 67
x / y = 876
The conventional order of solving a mathematical expression always follows the BODMAS RULE:
Bracket(B)
Order(O)
Division(D)
Multiplication(M)
Addition(A)
Subtraction(S)
Here's a step-by-step evaluation of an expression:
(7 * 4) + (67 - 2) - (88 / 4)
(28) + (65) - (22)
93 - 22
71
5) Data Types
Programming languages require that every user input be classified into a certain datatype for computers to understand it and act on it.
Some of the commonly used built-in datatypes are:
String
Integer
Float
Boolean
5.1) String (denoted by str
)
A string is defined as a continuous sequence of letters, numbers, and alphanumeric characters enclosed within quotes. This can include:
Capital Letters ( "A – Z" )
Small Letters ( "a – z" )
Digits ( "0 – 9" )
Special Characters ("~ ! @ # % ^ . ?,")
Space (" ")
Examples:-
"Hello, How was the day?"
"09038898"
5.2) Integer (denoted by int
)
An integer is defined as any whole number (positive / negative / 0) without any fractional or decimal parts.
This can include:
Positive Numbers (88, 34, 3, 21, 123)
Negative Numbers (-123, -34, -567, -76)
Positive nor Negative ( 0 )
5.3) Float (denoted by int
)
Any numeric value with a fractional part or decimal point comes under the float datatype.
This can include:
Positive Decimal Numbers (67.56, 367.56)
Negative Decimal Numbers (-13.98, -534.87)
5.4) Boolean (denoted by bool
)
In a broad sense, everything that can hold one of two possible values is called a Boolean.
This can include:
True or False
On or Off
0 or 1
Yes or No
Note: Python programming language recognizes True
and False
as the boolean values.
6) Order of Execution
Python always executes programs in a line-by-line sequence of order of code in a program.
name ='Vineet'
city ='Delhi'
occupation ='Student'
print(city)
print(grade)
grade=10
Python returned the output
"Delhi"
as the variable & its value was initialized before the corresponding print() function was called.Python returned a
"NameError"
because the variable"grade"
had not yet been created when we attempted to print it.
7) Continuous Variable Updation
In Python, a variable's value can be modified or updated dynamically based on the requirement of the program.
a = 456
print(a)
a = a + 100
# a += 100 is also same
print(a)
In the above example, variable 'a' initially held the value 456
. When 100
is added to 'a', its value gets updated to 556
. Now, if the variable is used hereafter at any point in the code, it returns the value 556
8) Taking Inputs From Users
To take inputs from users we can make use of the input()
function.
Note: The input()
function by default categorizes every line of user input as a string
datatype unless the user explicitly mentions a datatype.
user_name = input("Enter your User Name: ")
print("Welcome, your user name is", username, "!")
9) Working With Strings
9.1) String Concatenation
Adding one or more strings together is called string concatenation.
We've already seen and used concatenations in the prior Section 8: Taking Inputs From Users.
However, let's take a look at another intuitive example
monument = "The" + " " + "Taj" + " " + "Mahal"
print(monument)
9.2) String Repetition
We use the multiplication symbol or (*)
asterisk symbol to make repeat a certain part of a string repeat "n" numbers of times.
Example 1:
text = "Hi Everyone!\n" * 10
# the \n is called a newline or linebreak character, makes it print
# on a new line.
print(text)
Example 2:
text = "SUbscribe to get my latest blog posts"
new_text = ("*" * 5) + text + ("*" * 5)
print(new_text)
9.3) String Indexing
The positions of characters in a string, which always begins at 0 upwards
can be used to access and pull out specific characters from the string. The positions of these characters are referred to as an index.
Example 1:
planet_name = "Earth"
first_letter = planet_name[0]
second_letter = planet_name[1]
last_letter = planet_name[4]
print(first_letter)
print(second_letter)
print(last_letter)
Example 2 (Negative Indexing):
password = "1234!@#$"
last_letter = password[-1]
second_last_letter = password[-2]
third_last_letter = password[-3]
fourth_last_letter = password[-4]
print(last_letter)
print(second_last_letter)
print(third_last_letter)
print(fourth_last_letter)
9.4) String Slicing
String slicing is the process of extracting a specific section of a string using indexing.
SYNTAX: variable_name[start_index : end_index]
NOTE: The end_index is not included in the output, meaning that Python will stop at the end_index - 1st position.
Example:
text = "We are learning Python"
part_text = text[5:10]
print(part_text)
9.5) Slicing from Start
If a start index is not specified, then Python takes the default start value as index 0
.
Example:
text = "We are learning Python"
part_text = text[:10]
print(part_text)
9.6) Slicing to End
If an end index is not specified, then Python goes to the end of the string.
Example:
text = "We are learning Python"
part_text = text[8:]
print(part_text)
Note: If neither the start nor end index
is given then, python starts from the beginning and goes till the end of the string.
10) Type Conversions
Type conversion, often known as type-casting, is the process of converting a value from one datatype to another.
Let us now make use of the type()
function to check the datatypes of some entries.
print(type("Have a Nice Day"))
print(type(3.14))
print(type(897))
print(type(True))
10.1) String datatype to Integer datatype
We use the int()
function to convert valid string data to integers.
Example 1:
user_otp = "346315"
update_otp = int(user_otp)
print(type(user_otp))
print(user_otp)
print(type(update_otp))
print(update_otp)
Example 2: Invalid Integer Conversion
number = "Hundred"
convert_num = int(number)
print(convert_num)
Example 3: Adding two numbers
We will first examine what happens if the values are not converted to the int
datatype.
first_no = input("Enter First Number: ")
second_no = input("Enter Second Number: ")
sum = first_no + second_no
print(sum)
first_no = int(input("Enter First Number: "))
second_no = int(input("Enter Second Number: "))
sum = first_no + second_no
print(sum)
Case 1: When we did not convert the input values to integer datatype, python by default considered it to be strings. And when we added them, it concatenated the two strings and returned the output
2424.
Case 2: When we converted the input values to integer datatype, we got the expected sum of two numbers as Python treated them as integers and returned the output
48.
10.2) Commonly used type conversions
int()
: Converts to integer datatypefloat()
: Converts to float datatypestr()
: Converts to a string datatypebool()
: Converts to boolean datatype
In Part 1 of this blog, we've covered all of the fundamental must-know topics for getting started with Python programming for data science.
Do look out for the upcoming Part 2, in which I will be covering these topics in depth:
Lists
Loops & Control Statements
Functions
Until then take care, Happy Learning!
Thanks for Reading!
Subscribe to my newsletter
Read articles from Vineet Singh Negi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vineet Singh Negi
Vineet Singh Negi
Hi there! I'm Vineet Singh Negi and I have just started writing blogs. I'm passionate about DevOps and Machine Learning exploring all the different technologies involved in the process. I am constantly seeking new opportunities to expand my skill set and am actively working on various projects to improve my knowledge. On my Hashnode blogs, you'll find a mix of tutorials and insights as I navigate the world of DevOps and Machine Learning. I will be sharing everything I learn along the way.