Introduction to C Programming

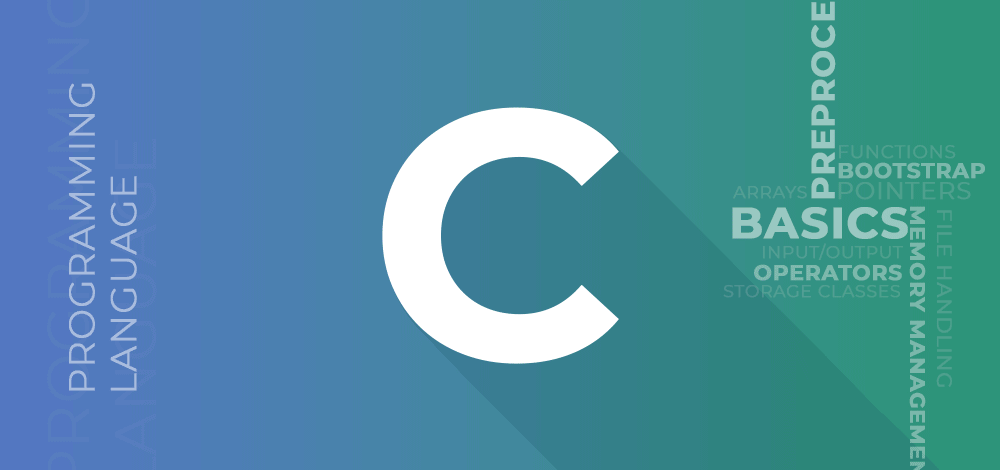
Previously we did the Introduction to the Programming Language where we learned about the basic functionality of computers, learned how information is stored and how compilers play an important role in converting our human readable code to machine language which is Binary.
Types
Introduction
Everything that we write gets converted into 0's and 1's (binary).
For example:
65 is stored as 01000001 in the computer
A is also stored as 01000001 in the computer
Wait, hold on a second! If every single thing we write gets turned into 0s and 1's by the compiler, how on earth does the computer figure out if a binary represents an integer or an alphabet? That's mind-boggling!
For that, the Typed Programming languages use a type system to interpret the bit streams in memory.
C is also a typed programming language.
A type is a rule that defines how to store values in memory and which operations are admissible on those values.
So, there are four most common types in the C language.
Arithmetic Types
The four most common types or data types of the C language for performing arithmetic calculations are:
char
int
float
double
A char
occupies 1 byte and can store a small integer value, a single character or a single symbol:
Now int like 1, 2, and 3 can be represented as 0 and 1's easily like we learned last time but characters and symbols have no intrinsic binary representation. So we use collating sequences like ASCII which associates each character and symbol with a unique integer value.
For example:
A is associated with 65 which has a binary value of 01000001.
Now you might think both 65 and A have the same binary value how does the computer distinguish between both? Then read this again.
An int
occupies one word and can store an integer value. In a 32-bit environment, an int
occupies 4 bytes:
A float
typically occupies 4 bytes and can store a single-precision, floating-point number:
A double
typically occupies 8 bytes and can store a double-precision, floating-point number:
Think of these types like a box which has a definite size and tells you what is inside.
When I say int, I know the box can hold a number like 1, 2, 3, ..100, and the biggest number it can hold is 2,147,483,647.
2,147,483,647 This is quite a big number but in today's world, we might need to store bigger values. For that, we can also adjust the size of our data types by using Size Specifiers.
Size Specifiers
Size specifiers adjust the size of the int
and double
types.
int
Type Size Specifiers
Specifying the size of an int ensures that the type contains a minimum number of bits. The three specifiers are:
short
long
long long
A short int
(or simply, a short
) contains at least 16 bits:
A long int
(or simply, a long
) contains at least 32 bits:
A long long int
(or simply, a long long
) contains at least 64 bits:
The size of a simple int
is no less than the size of a short
.
double
Type Size Specifier
The size of a long double
depends on the environment and is typically at least 64 bits:
Specifying the long double
type only ensures that it contains at least as many bits as a double
. The C language does not require a long double
to contain a minimum number of bits.
Value Ranges
The number of bytes allocated for a type determines the range of values that that type can store.
Integral Types
The ranges of values for the integral types are shown below. Ranges for some types depend on the execution environment:
Type | Size | Min | Max |
char | 8 bits | -128 | 127 |
char | 8 bits | 0 | 255 |
short | \>= 16 bits | -32,768 | 32,767 |
int | 2 bytes | -32,768 | 32,767 |
int | 4 bytes | -2,147,483,648 | 2,147,483,647 |
long | \>= 32 bits | -2,147,483,648 | 2,147,483,647 |
long long | \>= 64 bits | -9,233,372,036,854,775,808 | 9,233,372,036,854,775,807 |
Floating-Point Types
The limits on a float and double depends on the execution environment:
Type | Size | Significant | Min Exponent | Max Exponent |
float | minimum | 6 | -37 | 37 |
float | typical | 6 | -37 | 37 |
double | minimum | 10 | -37 | 37 |
double | typical | 15 | -307 | 307 |
long double | typical | 15 | -307 | 307 |
NOTE
Both the number of significant digits and the range of the exponent are limited. The limits on the exponent are in base 10.
Variable Declarations
Variables are containers for storing data values, like numbers and characters. The type identifies the properties of the variable.
To create a variable, specify the type and assign it a value:
Syntax
type variableName = value;
Where type is one of C types (such as int
), and variableName is the name of the variable (such as x or myName). The equal sign is used to assign a value to the variable.
We conclude the declaration with a semi-colon, making it a complete statement.
For example:
char children;
int nPages;
float cashFare;
const double pi = 3.14159265;
// const means that you can't change the value of variable pi.
// if we try to do somthing like this
pi = 4;
// this is going to give error as
//I am trying to change unmodifiable value
You can also declare a variable without assigning the value, and assign the value later:
Example
// Declare a variable
int myNum;
// Assign a value to the variable
myNum = 15;
Multiple Declarations
We may group the identifiers of variables that share the same type within a single declaration by separating the identifiers by commas.
For example,
char children, digit;
int nPages, nBooks, nRooms;
float cashFare, height, weight;
double loan, mortgage;
Subscribe to my newsletter
Read articles from Pratham Garg directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Pratham Garg
Pratham Garg
I am a student learning Computer Programming 👨💻.