Python Data Types and Data Structures for DevOps ๐๐งฑ
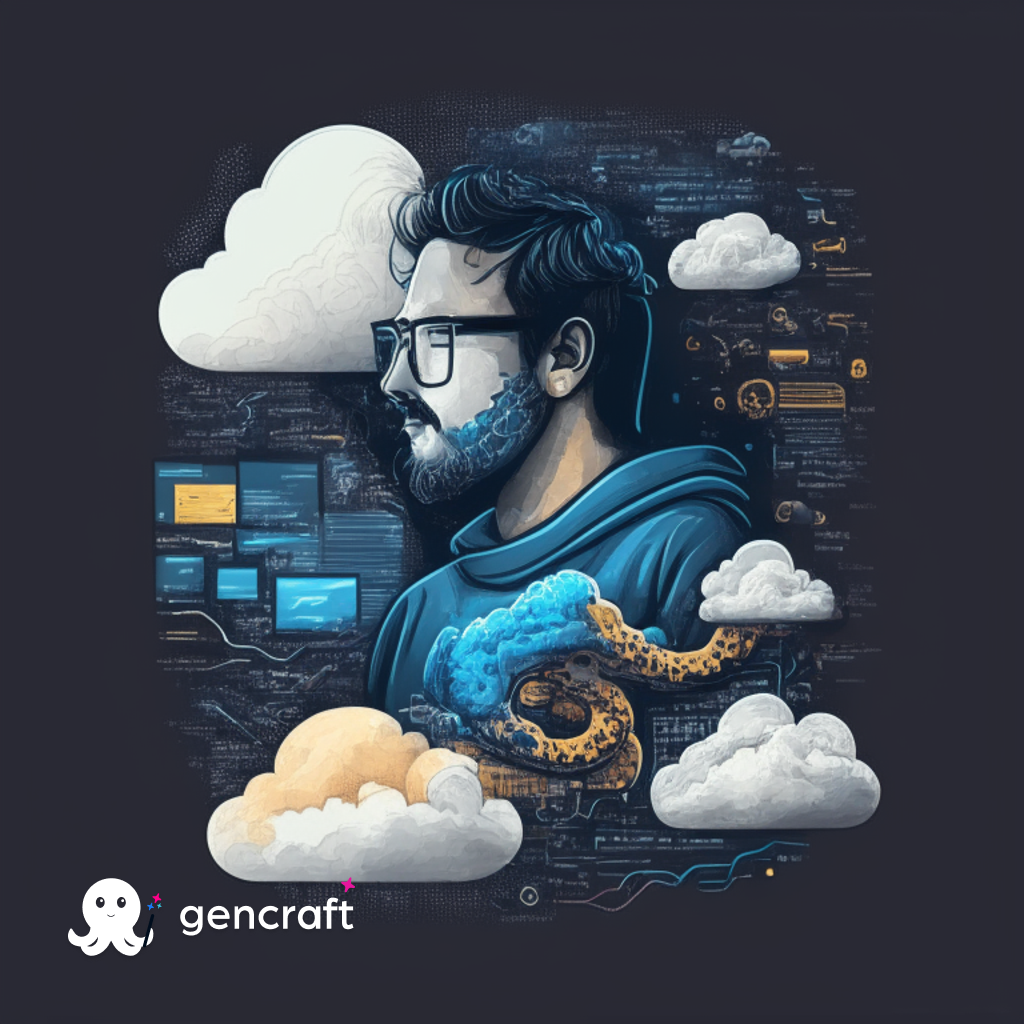

๐ Hello DevOps Adventurers! A new day, a new challenge. Inspired by the amazing Shubham Londhe and his #90daysofdevops journey, let's dive into the world of Python data types and data structures. Let's unravel the magic behind coding! ๐ปโจ
Understanding Data Types: Your Building Blocks
Data types are like the ingredients that make up your coding recipe. They help us organize and work with different kinds of data. In Python, everything's like a magical object, and data types are the spells that define them. Here's a quick look at some data types:
Numeric ๐งฎ: Integers, complex numbers, and floating-point numbers.
Sequential ๐: Strings (words and text), lists (ordered collections), and tuples (kind of like lists but unchangeable).
Boolean ๐ฆ: Represents either
True
orFalse
.Set ๐ฎ: A group of items without duplicates, in no particular order.
Dictionary ๐: Store stuff like a map - with key-value pairs.
Want to know a data type? Just use the type()
function. For instance, if you have my_variable
, type type(my_variable)
.
Data Structures: Your Coding Tools
Think of data structures as your coding toolbox. They help you organize your stuff efficiently. Python makes these tools user-friendly. Here are a few essential ones:
Lists: Your Collection Bins
๐ฆ Ordered collections.
๐จ Mix of different items.
๐ Create, modify, access with ease.
# Creating a list
fruits = ["apple", "banana", "orange", "grape"]
# Accessing elements
print(fruits[0]) # Output: apple
# Modifying elements
fruits[1] = "pear"
print(fruits) # Output: ['apple', 'pear', 'orange', 'grape']
# Appending an element
fruits.append("kiwi")
print(fruits) # Output: ['apple', 'pear', 'orange', 'grape', 'kiwi']
Tuples: The Immutable Helpers
๐ง Like lists, but frozen.
๐ Elements can't change.
๐งค Ensures your data's integrity.
# Creating a tuple
coordinates = (3, 4)
# Accessing elements
x = coordinates[0]
y = coordinates[1]
print(f"x: {x}, y: {y}") # Output: x: 3, y: 4
Dictionaries: Your Magical Maps
๐บ๏ธ Key-value pairs for data.
๐ Fast retrieval based on keys.
๐ฎ Manage configurations like a pro.
# Creating a dictionary
student = {
"name": "Alice",
"age": 20,
"major": "Computer Science"
}
# Accessing values
print(student["name"]) # Output: Alice
# Modifying values
student["age"] = 21
print(student) # Output: {'name': 'Alice', 'age': 21, 'major': 'Computer Science'}
# Adding a new key-value pair
student["gpa"] = 3.8
print(student) # Output: {'name': 'Alice', 'age': 21, 'major': 'Computer Scien
Task 1: Data Types Unveiled
Data types are like the building blocks of programming. They help us organize and work with different kinds of data. Let's break down the differences between three crucial data types: lists, tuples, and sets.
List: Your Versatile Collection
Definition: An ordered collection of items that can be of any type.
Key Characteristics:
Items can be added, modified, and removed.
Enclosed in square brackets
[ ]
.
Tuple: Your Immutable Friend
Definition: An ordered collection of items that is unchangeable once created.
Key Characteristics:
Similar to lists but uses parentheses
( )
.Provides data integrity, perfect for constants.
Set: Your No-Duplicate Zone
Definition: An unordered collection of unique items.
Key Characteristics:
Cannot contain duplicate values.
Created using curly braces
{ }
.
Your Hands-On: Create examples for each data type and take screenshots to showcase your understanding.
Task 2: Favorite Tools Dictionary
Let's harness the power of dictionaries to organize and retrieve data efficiently. Here's a pre-made dictionary with your favorite DevOps tools. Your task is to use dictionary methods to retrieve tools using their numeric keys.
Your Hands-On: Write code that prints out your favorite DevOps tool by using its numeric key. For instance, print(fav_tools[2])
should display "Git."
codefav_tools = {
1: "Linux",
2: "Git",
3: "Docker",
4: "Kubernetes",
5: "Terraform",
6: "Ansible",
7: "Chef"
}
# Replace the 'key' with your favorite tool's corresponding key
favorite_tool_key = 2
# Using dictionary methods to print your favorite tool
if favorite_tool_key in fav_tools:
favorite_tool = fav_tools[favorite_tool_key]
print("My favorite tool is:", favorite_tool)
else:
print("Favorite tool not found in the dictionary.")
In this example, I've used the key 2
to represent "Git" as your favorite tool. You can replace the favorite_tool_key
value with the key corresponding to your actual favorite tool to print it using the dictionary.
Task 3: Cloud Providers Adventure
Let's play with lists and add Digital Ocean to our list of cloud service providers. Then, let's sort the list alphabetically.
cloud_providers = ["AWS", "GCP", "Azure"]
new_provider = "Digital Ocean"
# Adding Digital Ocean to the list
cloud_providers.append(new_provider)
# Sorting the list in alphabetical order
cloud_providers.sort()
print("Updated cloud providers list:", cloud_providers)
When you run this program, it will add "Digital Ocean" to the list and then sort the list so that the output will be:
cloud providers list: ['AWS', 'Azure', 'Digital Ocean', 'GCP']
Your Code Journey:
Use the
append()
method to add "Digital Ocean" tocloud_providers
.Use the
sort()
method to alphabetically arrange the providers.
Your Tools:
append()
adds an item to the end of the list.sort()
arranges items in alphabetical or numerical order.
๐ Now, go forth and conquer these tasks! Remember, the journey to becoming a DevOps wizard is all about learning, practicing, and having fun along the way. Share your progress with the #90daysofdevops community and let's rock this together! ๐ช๐จโ๐ป #PythonWonders #DataMastery
๐ข Stay in the โ๏ธ cloud loop and blast off into the ๐ DevOps universe! Join me on Hashnode, where I share my latest insights and articles about all things cloud and DevOps. Let's connect on LinkedIn (https://www.linkedin.com/in/nirmal-mahale-605565215/) and explore the boundless possibilities of the tech cosmos together. ๐ And hey, if you want to peek under the hood of my projects, hop on over to my GitHub (https://github.com/nirmal7030). ๐ ๏ธ
Subscribe to my newsletter
Read articles from Nirmal Mahale directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
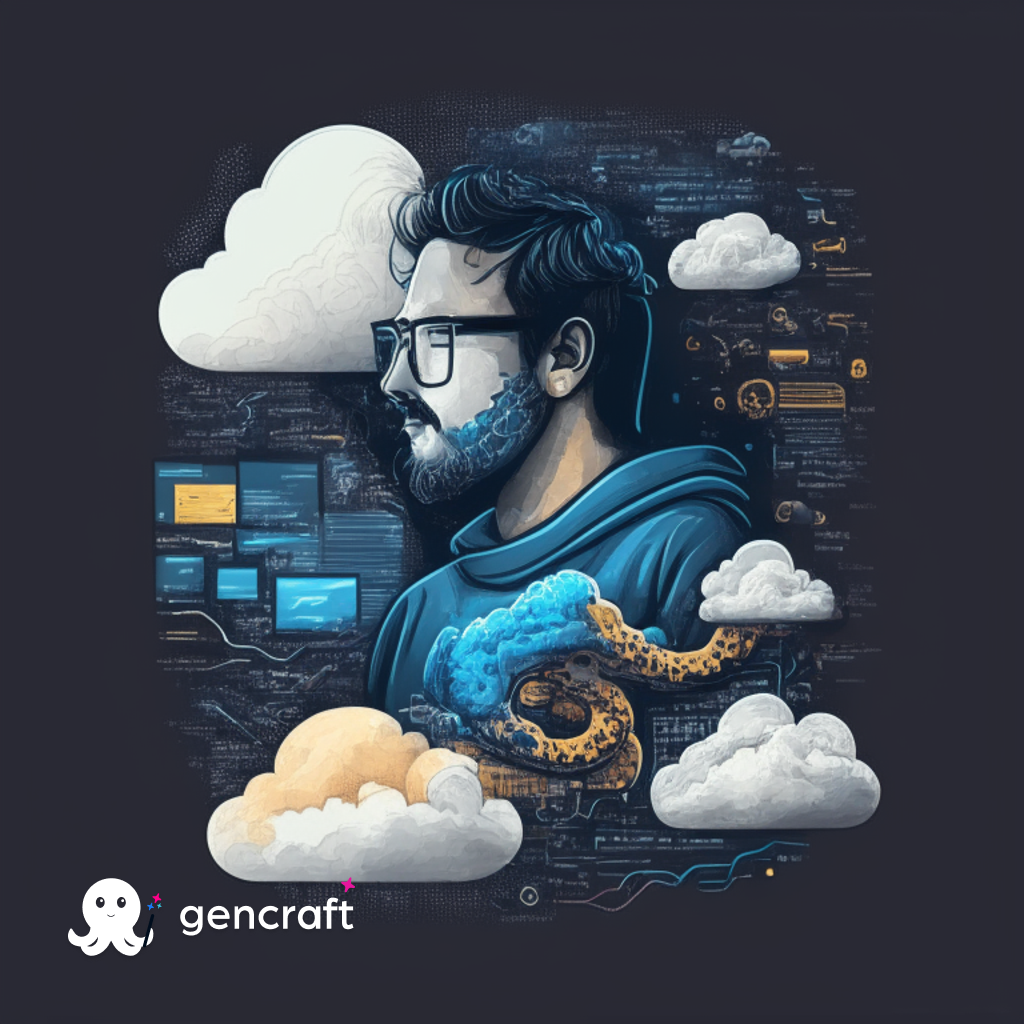
Nirmal Mahale
Nirmal Mahale
Passionate about Python, Cloud Computing, and DevOps. Architecting robust cloud solutions, mastering AWS & Azure, and automating with cutting-edge DevOps tools. Join me on this journey of innovation and collaboration. Let's shape the future together!