Break, Continue and More Commands In Shell Script
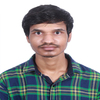
Break
-> Suppose we want that our loop should stop running when certain conditions meet then we use Break.
Example - Suppose we want to find a particular number from a list of numbers and if that number is found then we should come out of the loop.
#!/bin/bash
no=6
for i in 1 2 3 4 5 6 7
do
if [[ $no -eq $i ]]
then
echo " $no No is Present"
break
fi
done
Output -
Continue
Suppose a situation comes where if a condition is met then we do not want to perform the operation and want to move forward then we can use continue.
Example - Suppose we have a list of numbers from 1 to 10. We want that only even number should be printed then we can use continue for that -
#!/bin/bash
for i in 1 2 3 4 5 6 7 8 9 10
do
if [[ $i%2 -ne 0 ]]
then
continue
else
echo "Number is $i"
fi
done
Output -
Some more commands
exit -> It is used to stop the script at a point
Ex -
#!/bin/bash
if [[ $# -eq 0 ]]
then
echo "Please Enter Some arguments"
exit 1
fi
echo "First Argument is $1"
echo "Second Argument is $2"
-> Here it is dependent on the Arguments, if we don't provide then our script will not run.
Output -
-> To see the exit status - $?
-> When our script fails to execute then the status code we get is a non-zero value-
-> When our script sun successfully then we get zero value
basename - Strip Dirctory info and only give filename
dirname - Strip filename info and only give Directory path
realpath - Gives us a full path of a file. It will give full path of location were we are.
if [[ -d folder_name ]] -> Check if directory exist
if [[ ! -d folder_name ]] -> Check if directory not exist
if [[ -f file_name ]] -> Check if file exist
#!/bin/bash
FILEPATH="/home/ubuntu/dir1/dir2/dir3/file1.txt"
if [[ -f $FILEPATH ]]
then
echo "File exists"
else
echo "File not exists"
fi
if [[ ! -f file_name ]] -\> Check if file not exist
Bash Variable
RANDOM
- It generates integer between 0 and 32767
UID
- It give the User ID of the user Logged In.
For root user, id will be zero
-> We can write a script to check if the User is logged in as a root or not-
#!/bin/bash
if [[ $UID -eq 0 ]]
then
echo "User is logged in as a root"
else
echo "User is not a root user"
fi
Output -
Redirection In Shell Script
-> We can redirect the output of a commands in other file
Threre are two ways in which we can redirect
1) Using ">" - If we use this then when we enter a new content in a file then earlier content will be deleted.
-> We have created a file which is all_file.txt and storing the output of ls command.
-> We can see that we have all the file list in all_file.txt file
-> Now when we storing the output of echo command in this file then we can see that our previous data got lost.
2) Using ">>" - If we want to persist all the content in this file then we can use this.
/dev/null
Suppose we do not want to print the output of a command on a terminal and do not want to store in a file then we can use &> /dev/null.
Example - Suppose if we do not use /dev/null then
-> When we use /dev/null
Logger
-> if we want to maintain the logging for our script then we can use logger in our script.
-> We can find logs under /var/logs/messages
Example - logger "Hello Bhaskar"
set -x
-> To enable the debugging in the script, we can use set -x in the start of the script.
set -e
-> If the script fails then it will exit and stop running the script
Run the Script In the Background
-> When we run the script then it captures the terminal and that time we can not perform other task.
-> To avoid this situation we can run this script in background,
-> To do this we have to use nohup command.
Example - nohup ./script_name &
Note - First chnage the permission of the file
-> Here it will create a nohup.out file and store the output of the script.
#!/bin/bash
for i in 1 2 3 4 5 6
do
echo "Number is $i"
sleep 3s
done
Output -
Subscribe to my newsletter
Read articles from Bhaskar Mehta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
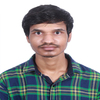
Bhaskar Mehta
Bhaskar Mehta
I am DevOps Engineer who works on DevOps tools like Docker, Kubernetes, Terraform, Git, GitHub, Jenkins and AWS services.