Retrieving and Displaying Weather Data using Python and the OpenWeatherMap API
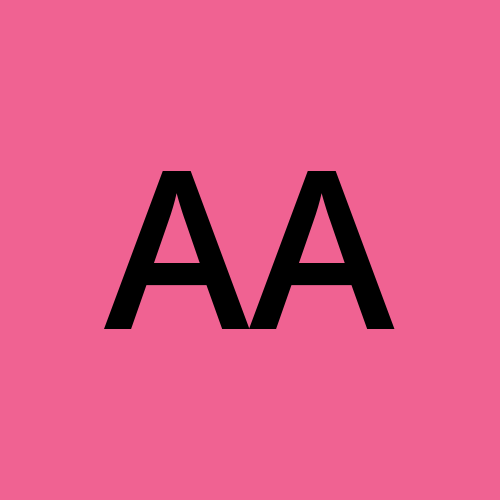
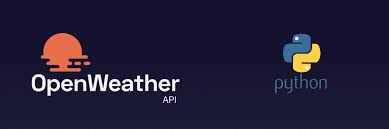
We will demonstrate how to use Python, along with the requests
library, to fetch and display weather data from the OpenWeatherMap API. This solution can be beneficial for creating weather-related applications or integrating weather information into various projects.
Problem Statement:
How to retrieve real-time weather data for a specific location and display it to users in a readable format.
Solution:
We'll use Python's requests
library to make an HTTP GET request to the OpenWeatherMap API, which will provide us with the weather information for a given city. We'll then parse the JSON response and extract relevant data to display.
import requests
def get_weather_data(city_name, api_key):
base_url = "http://api.openweathermap.org/data/2.5/weather"
params = {
"q": city_name,
"appid": api_key,
"units": "metric" # Use metric units for temperature
}
response = requests.get(base_url, params=params)
return response.json()
def display_weather_data(weather_data):
temperature = weather_data["main"]["temp"]
weather_description = weather_data["weather"][0]["description"]
print(f"Temperature: {temperature}°C")
print(f"Weather: {weather_description}")
if __name__ == "__main__":
city = "New York" # Replace with the desired city
api_key = "YOUR_API_KEY" # Replace with your OpenWeatherMap API key
data = get_weather_data(city, api_key)
display_weather_data(data)
Explanation:
We import the
requests
library to handle HTTP requests.The
get_weather_data
function takes a city name and an API key as parameters, constructs the API request URL, and retrieves the weather data in JSON format.The
display_weather_data
function takes the weather data JSON and extracts relevant information such as temperature and weather description.In the
main
section, we specify the desired city and API key, call the functions, and display the weather information.
Conclusion:
The solution demonstrates how to use Python and the requests
library to fetch and display weather data from the OpenWeatherMap API.
Subscribe to my newsletter
Read articles from Adekunle Adefokun directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
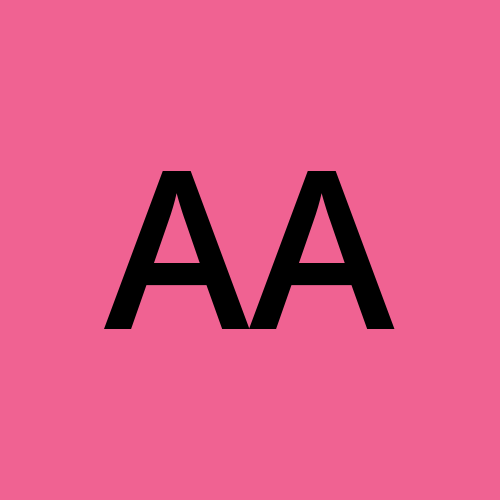
Adekunle Adefokun
Adekunle Adefokun
I am a software engineer with experience in product management and design.