Encryption and Emojis!
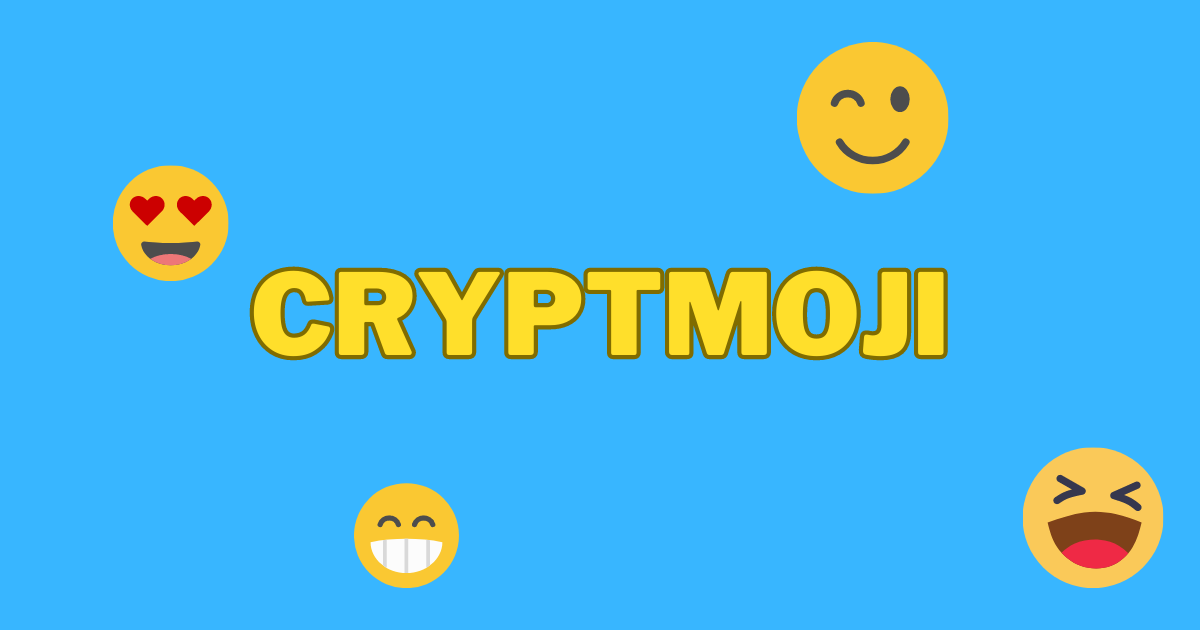
Almost nine months ago, I published my 3rd Python library - Cryptmoji. You may have come across a ton of cryptography libraries on PyPI. Many may be relatively safer, but I aimed to use "Caesar Cipher" and "mapping" to make a fun tool to learn cryptography. To install Cryptmoji, run the following:
pip install cryptmoji
How to use Cryptmoji
Encryption
To encrypt text, we use the encrypt()
function.
>>> from cryptmoji import encrypt
>>> text = "H3LL0 W0RLD"
>>> encrypted = encrypt(text)
'๐พ๐๐๐๐๐๐๐๐๐๐บ'
The output is much different than the regular encryption algorithms, isn't it?
Decryption
To decrypt text, we use the decrypt()
function.
>>> from cryptmoji import decrypt
>>> encrypted = "๐พ๐๐๐๐๐๐๐๐๐๐บ"
>>> decrypt(encrypted)
'H3LL0 W0RLD'
And Voila! We have our string back!
using the Key
parameter
As you can see, the output starts to become predictable after some time. So we need another parameter to shuffle the characters. This Parameter is key
. This will drastically change the encrypted string.
For example:
>>> from cryptmoji import encrypt, decrypt
>>> key = "HI_M0M"
>>> encrypted = encrypt("H3LL0 W0RLD", key=key)
>>> encrypted
'๐๐ฒ๐ฎ๐๐๐ฃ๐ข๐ฏ๐ด๐๐ช'
>>> decrypt(encrypted, key=key)
'H3LL0 W0RLD'
For more details, refer to the documentation and GitHub repo.
Subscribe to my newsletter
Read articles from Siddhesh Agarwal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Siddhesh Agarwal
Siddhesh Agarwal
I am a CSE Student from Coimbatore, India.